Reference link: https://my.oschina.net/sdlvzg/blog/1590946
Reference link: https://blog.csdn.net/chengqiuming/article/details/83684952
1. What is the Future type?
Future is the interface for canceling the execution result of a specific Runnable or Callable task, querying whether it is completed, and obtaining the result. If necessary, you can get the execution result through the get method, which will block until the task returns the result.
Its interface is defined as follows:
public interface Future<V> { boolean cancel(boolean mayInterruptIfRunning); boolean isCancelled(); boolean isDone(); V get() throws InterruptedException, ExecutionException; V get(long timeout, TimeUnit unit) throws InterruptedException, ExecutionException, TimeoutException; }
2. It declares the following five methods:
-
The cancel method is used to cancel the task. If the task is cancelled successfully, it returns true. If the task is cancelled successfully, it returns false. The parameter mayInterruptIfRunning indicates whether to allow canceling tasks that are being executed but not completed. If true is set, tasks that are in the process of execution can be cancelled. If the task has been completed, this method will definitely return false no matter whether the mayInterruptIfRunning is true or false, that is, if the completed task is cancelled, it will return false; if the task is executing, if the mayInterruptIfRunning is set to true, it will return true; if the mayInterruptIfRunning is set to false, it will return false; if the task has not been executed, it will return false no matter whether the mayinterrupt If uptifrunning is true or false, it must return true.
-
The isCancelled method indicates whether the task has been cancelled successfully. If it has been cancelled successfully before the task completes normally, it returns true.
-
The isDone method indicates whether the task has been completed. If the task is completed, it returns true;
-
The get() method is used to get the execution result. This method will block until the task is completed;
-
get(long timeout, TimeUnit unit) is used to get the execution result. If the result has not been obtained within the specified time, it will return null directly.
3. In other words, Future provides three functions:
-
Judge whether the task is completed
-
Ability to interrupt tasks
-
Ability to get task execution results
4. Cases
4.1 write dynamic Task class, mainly to control any addition and modification through URL.
package com.example.power_spider.controller; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.scheduling.concurrent.ThreadPoolTaskScheduler; import org.springframework.scheduling.support.CronTrigger; import org.springframework.stereotype.Component; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.example.power_spider.utils.SchedulingRunnable; import java.util.concurrent.ScheduledFuture; import java.util.concurrent.TimeUnit; /** * @author Mr.Hao * @date 2020-03-27 */ @RestController @Component public class DynamicTaskController { @Autowired private ThreadPoolTaskScheduler threadPoolTaskScheduler; /** * * ThreadPoolTaskScheduler: Thread pool task scheduling class, which can open the thread pool for task scheduling. * ThreadPoolTaskScheduler.schedule()Method creates a scheduled future, * Two parameters need to be added in this method, Runnable (thread interface class) and CronTrigger (timed task trigger) * @return */ @Bean public ThreadPoolTaskScheduler threadPoolTaskScheduler() { ThreadPoolTaskScheduler executor = new ThreadPoolTaskScheduler(); return new ThreadPoolTaskScheduler(); } /** * There is a cancel in ScheduledFuture to stop the scheduled task. */ private ScheduledFuture<?> future; /** * Start task **/ @RequestMapping("/startTask") public String startCron() { future = threadPoolTaskScheduler.schedule(new SchedulingRunnable(), new CronTrigger("0/5 * * * * *")); System.out.println("DynamicTaskController.startCron()"); try{ //The get() method is used to get the execution result. This method will block until the task is completed; //get() of future sets the timeout Object o = future.get(3900, TimeUnit.MILLISECONDS); System.out.println("===>>> "+o); Object o1 = future.get(); System.out.println("===>>> "+o1); } catch ( Exception e){ System.out.println(e); } return "startTask"; } /** * Start this task **/ @RequestMapping("/stopTask") public String stopCron() { if (future != null) { future.cancel(true); } System.out.println("DynamicTaskController.stopCron()"); return "stopTask"; } /** * Change task interval, restart **/ @RequestMapping("/changeCron") public String changeCron() { stopCron();// Stop first, turn on future = threadPoolTaskScheduler.schedule(new SchedulingRunnable(), new CronTrigger("*/10 * * * * *")); System.out.println("DynamicTaskController.changeCron()"); return "changeCron"; } }
4.2 write a simple thread:
package com.example.power_spider.utils; import java.text.SimpleDateFormat; import java.util.Date; import cn.hutool.core.date.DateUtil; /** * @author Mr.Hao * @date 2020-03-27 */ public class SchedulingRunnable implements Runnable { @Override public void run() { Date date = DateUtil.date(); System.out.println("The time to execute the function is now:"+date); } }
4.3 note: there are several points to understand in the above classes:
- A class of ThreadPoolTaskScheduler: thread pool task scheduling class, which can open the thread pool for task scheduling.
- Thr eadPoolTaskScheduler.schedule () method will create a scheduled future. In this method, you need to add two parameters, Runnable (thread interface class) and CronTrigger (scheduled task trigger)
- There is a cancel in ScheduledFuture to stop the scheduled task.
effect:
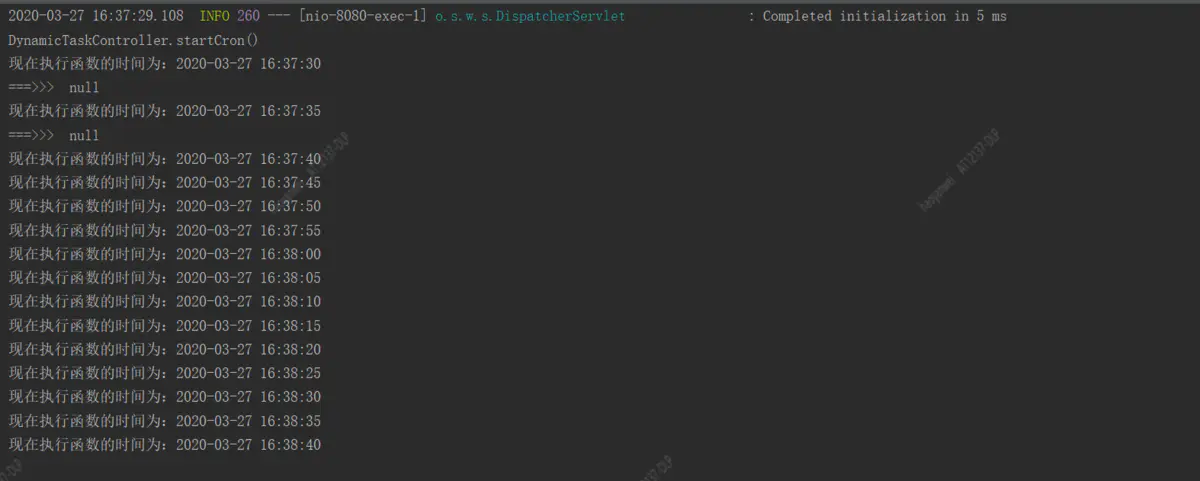
Author: HAO Yanwei
Link: https://www.jianshu.com/p/0eceb245f6c7
Source: Jianshu
The copyright belongs to the author. For commercial reprint, please contact the author for authorization. For non-commercial reprint, please indicate the source.