We know that the bottleneck of a program is the database. We also know that the speed of memory is much faster than that of hard disk. When we need to retrieve the same data repeatedly, we request database or remote service again and again, which leads to a large amount of time spent on database query or remote method invocation, resulting in deterioration of program performance. This is the problem to be solved by data caching.
Spring cache support
Spring defines the technologies used by the org. spring framework. cache. Cache Manager and the org. spring framework. cache. Cache interfaces to unify different caches. Among them, Cache Manager is the abstract interface of various caching technologies provided by Spring. Cache interface contains various operations of caching (adding, deleting and obtaining caches, we usually do not deal with this interface directly).
1.Spring-supported Cache Manager
CacheManager | describe |
---|---|
SimpleCacheManager | Use a simple Collection to store caches, mainly for testing |
ConcurrentMapCacheManager | Use Concurrent Map to store caches |
NoOpCacheManager | Testing only, no caching is actually stored |
EhCacheCacheManager | Using EhCache as Caching Technology |
GuavaCacheManager | Use the Guava Cache of google guava as a caching technology (abandoned in version 1.5) |
HazelcastCacheManager | Using Hazelcast as Caching Technology |
JCacheCacheManager | Supporting the implementation of JCache (JSR-107) standard as a caching technology, such as Apache Commons JCS |
RedisCacheManager | Using redis as a caching technique |
For different caching technologies, different Cache Managers need to be implemented. Spring defines the implementation of Cache Manager as shown in the table.
CacheManager | describe |
---|---|
SimpleCacheManager | Use a simple Collection to store caches, mainly for testing |
ConcurrentMapCacheManager | Use Concurrent Map to store caches |
NoOpCacheManager | Testing only, no caching is actually stored |
EhCacheCacheManager | Using EhCache as Caching Technology |
GuavaCacheManager | Use the Guava Cache of google guava as a caching technology (abandoned in version 1.5) |
HazelcastCacheManager | Using Hazelcast as Caching Technology |
JCacheCacheManager | Supporting the implementation of JCache (JSR-107) standard as a caching technology, such as Apache Commons JCS |
RedisCacheManager | Using redis as a caching technique |
When we use Cache Manager of any implementation, we need to register the Bean of the implemented Cache Manager, for example:
@Bean public EhCacheCacheManager cacheManager(CacheManager ehCacheCacheManager) { return new EhCacheCacheManager(ehCacheCacheManager); }
Of course, there are many additional configurations for each caching technology, but configuring the cache manager is essential.
2. Nominal Cache Annotations
Spring provides four annotations to declare caching rules (another vivid example of using annotated AOP). These four annotations are shown in the table.
annotation | explain |
---|---|
@Cacheable | Before the method is executed, Spring first checks whether there is data in the cache, and if there is data, it returns the data directly to the cache; if not, it calls the method and puts the returned value into the cache. |
@CachePut | In any case, the return value of the method is put into the cache. @ CachePut's attributes are consistent with @Cacheable |
@CacheEvict | Delete one or more data from the cache |
@Caching | Through this annotation, multiple annotation strategies can be combined on one method. |
@ Cacheable, @CachePut, @CacheEvit all have value attributes, specifying the name of the cache to be used; key attributes specify the key for the storage of data in the cache.
3. Open Famous Cache Support
It's easy to turn on fame caching support by simply using the @EnableCaching annotation on the configuration class, such as:
@Configuration @EnableCaching public class AppConfig { }
Spring Boot support
The key to using caching technology in Spring is to configure Cache Manager, and Spring Boot automatically configures several Cache Manager implementations for us.
The automatic configuration of Spring Boot's Cache Manager is placed in the org. spring framework. boot. autoconfigure. cache package, as shown in the figure.
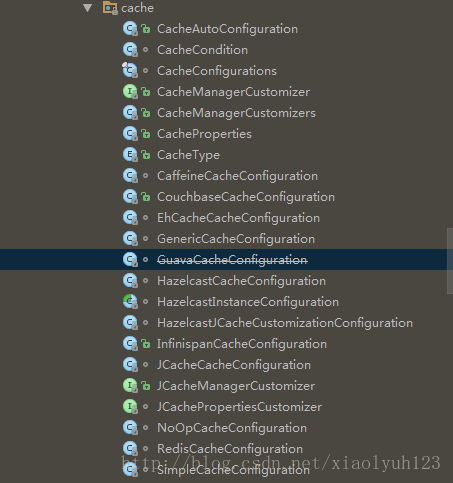
From the diagram, we can see that Spring Boot automatically configures EhCache Configuration (using EhCache), GenericCache Configuration (using Collection), Guava Cache Configuration (using Guava), Hazelcast Cache Configuration (using Hazelcast), Infinispan Cache Configuration (using Infinispan), JCache Cache Configuration (using JCache). NoOpCacheConfiguration (no storage), RedisCacheConfiguration (using Redis), SimpleCacheConfiguration (using Concurrent Map). Without any additional configuration, the default is SimpleCacheConfiguration, even with Concurrent MapCache Manager.
/* * Copyright 2012-2016 the original author or authors. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.springframework.boot.autoconfigure.cache; import java.util.List; import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean; import org.springframework.cache.CacheManager; import org.springframework.cache.concurrent.ConcurrentMapCacheManager; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Conditional; import org.springframework.context.annotation.Configuration; /** * Simplest cache configuration, usually used as a fallback. * * @author Stephane Nicoll * @since 1.3.0 */ @Configuration @ConditionalOnMissingBean(CacheManager.class) @Conditional(CacheCondition.class) class SimpleCacheConfiguration { private final CacheProperties cacheProperties; private final CacheManagerCustomizers customizerInvoker; SimpleCacheConfiguration(CacheProperties cacheProperties, CacheManagerCustomizers customizerInvoker) { this.cacheProperties = cacheProperties; this.customizerInvoker = customizerInvoker; } @Bean // Concurrent MapCache Manager is used by default public ConcurrentMapCacheManager cacheManager() { ConcurrentMapCacheManager cacheManager = new ConcurrentMapCacheManager(); List<String> cacheNames = this.cacheProperties.getCacheNames(); if (!cacheNames.isEmpty()) { cacheManager.setCacheNames(cacheNames); } return this.customizerInvoker.customize(cacheManager); } }
Spring Boot supports the configuration of caches with properties prefixed with "spring.cache".
spring.cache.type= # Optional generic, ehcache, hazelcast, infinispan, jcache, redis, guava, simple, none spring.cache.cache-names= # Create a cache name at program startup spring.cache.ehcache.config= # ehcache profile address spring.cache.hazelcast.config= # hazelcast configuration file address spring.cache.infinispan.config= # infinispan configuration file address spring.cache.jcache.config= # jcache configuration file address spring.cache.jcache.provider= #When multiple jcache implementations are in the classpath, specify the jcache implementation spring.cache.guava.spec= # guava specs
In Spring Book environment, using caching technology only needs to import dependency packages of related caching technology into the project, and use @EnableCaching to open caching support in configuration class.