shell programming alarm system
1. Requirements: use shell to customize various personalized alarm tools, but unified management and standardized management are needed2. Idea: specify a script package, including main program, subprogram, configuration file, mail engine, output log, etc
Main program: as the entrance of the whole script, it is the lifeblood of the whole system.
Configuration file: it is a control center, which is used to switch on and off each subprogram, and specify each associated log file.
Subprogram: real monitoring script, used to monitor various indicators.
Mail engine: it is implemented by a php program, which can define the server, sender and receiver of mail.
Output log: the whole monitoring system should have log output
Requirements: for different machines playing different roles, the same monitoring system should be deployed, but different configuration files should be customized.
Program architecture:
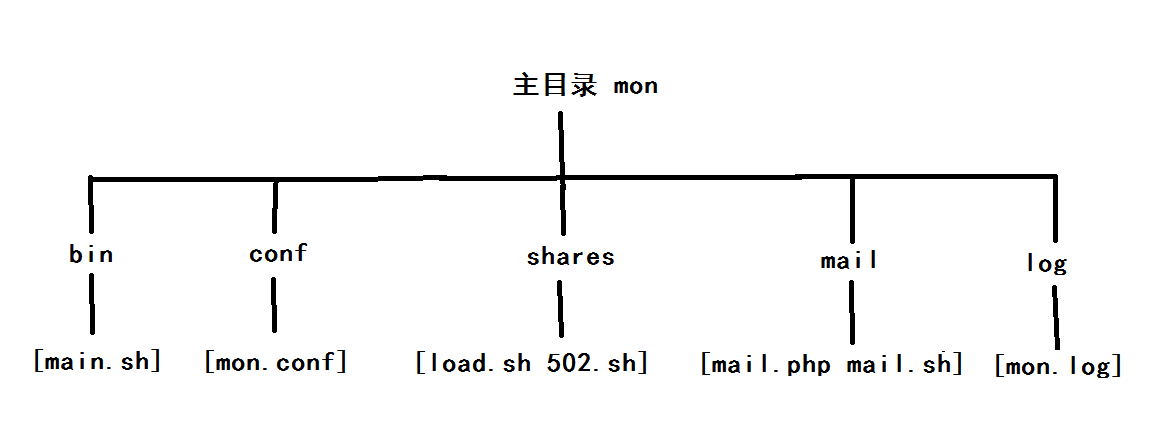
bin is the main program
Configuration file under conf
Under shares are various monitoring scripts
Mail engine under mail
3. Specific script
(1),main.sh
#!/bin/bash #main.sh #Whether to send mail export send=1 #Filter ip address export addr=`/sbin/ifconfig|grep -A1 'eth0'|grep addr: |awk '{print $2}'|awk -F : '{print $2}'` dir=`pwd` #Only the last level directory name is required last_dir=`echo $dir|awk -F '/' '{print $NF}'` #Ensure that the script is executed in the bin directory, so as to avoid that the monitoring script, mail and log cannot be found if [ $last_dir == "bin" ] || [ $last_dir == "bin/" ];then conf_file="../conf/mon.conf" else echo "you should cd bin dir" exit fi exec 1>>../log/mon.log 2>>../log/err.log #All servers need to monitor the load echo "`date +"%F %T"` load average" /bin/bash ../shares/load.sh #Check if monitoring is required in the configuration file 502 if grep -q 'to_mon_502=1' $conf_file;then export log=`grep 'logfile=' $conf_file |awk -F '=' '{print $2}' |sed 's/ //g'` /bin/bash ../shares/502.sh fi
(2) Configuration file mon.conf
(3)load.sh##to config the options of monitor ##cdb mainly defines the server address, port, user name and password of mysql #1 or 0, 1 is monitored, 0 is not monitored to_mon_cdb=0 cdb_ip=10.20.3.13 cdb_port=3315 cdb_user=username cdb_pass=passwd ##Httpd 1 is monitored, 0 is not monitored to_mon_httpd=0 ##php 1 is monitored, 0 is not monitored to_mon_php_socket=0 ##HTTP code 502 access log path needs to be defined to_mon_502=1 logfile=/data/log/www.example.com/access.log ##Request & count custom log path and domain name to_mon_request_count=0 req_log=/data/log/www.discuz.net/access.log domainname=www.discuz.net
#!/bin/bash ##load.sh load=`uptime |awk -F 'average:' '{print $2}'|cut -d ',' -f1|sed 's/ //g' |cut -d . -f1` if [ $load -gt 20 ] && [ $send -eq "1" ] then echo "$addr `date +%T` load is $load" >../log/load.tmp /bin/bash ../mail/mail.sh $addr\_load $load ../log/load.tmp fi echo "`date +%T` load is $load"
(4)502.sh
#!/bin/bash ##502.sh d=`date -d "-1 min" +%H:%M` c_502=`grep :$d: $log |grep '502'|wc -l` if [ $c_502 -gt 10 ] && [ $send == 1 ];then echo "$addr $d 502 count is $c_502">../log/502.tmp /bin/bash ../mail/mail.sh $addr\_502 $c_502 ../log/502.tmp fi echo "`date +%T` 502 $c_502"
(5)mail.php
<?php class Smtp { /*Public Variables*/ var $smtp_port; var $time_out; var $host_name; var $log_file; var $relay_host; var $debug; var $auth; var $user; var $pass; /*Private Variables*/ var $sock; /*Constractor*/ function Smtp($relay_host="",$smtp_port = 25,$auth = false,$user,$pass) { $this->debug = FALSE; $this->smtp_port = $smtp_port; $this->relay_host = $relay_host; $this->time_out = 30; $this->auth = $auth; $this->user = $user; $this->pass = $pass; $this->host_name = "localhost"; $this->log_file = ""; $this->sock = FALSE; } /*Manin Function*/ function sendmail($to,$from,$subject = "",$body = "",$mailtype,$cc = "",$bcc = "",%addtional_headers = "") { $mail_from = $this->get_address($this->strip_comment($from)); $body = ereg_replace("(^|(\r\n))(\.)","\1.\3",$body); $header = "MIME-Version:1.0\r\n"; if($mailtype=="HTML"){ $header .= "Content-Type:text/html\r\n"; } $header .= "To: ".$to."\r\n"; if($cc != ""){ $header .= "Cc: ".$cc."\r\n";; } $header .= "From: $from<".from.">\r\n"; $header .= "Subject: ".$subject."\r\n"; $header .= $additional_headers; $header .= "Date: ".date("r")."\r\n"; $header .= "X-Mailer:By Redhat (PHP/".phpversion().")\r\r"; list($msec.$sec) = explode(" ",microtime()); $header .= "Message-ID:<".date("YmdHis",$sec).".".($msec*1000000).".".$mail_from.">\r\n"; $TO = explode(",",$this->strip_comment($to)); if($cc != ""){ $TO = array_merge($TO,explode(",",$this->strip_comment($cc))); } if($bcc != ""){ $TO = array_merge($TO,explode(",",$this->strip_comment($bcc))); } $sent = TRUE; foreach ($TO as $rcpt_to){ $rcpt_to = $this->get_address($rcpt_to); if(!$this->smtp_sockopen($rcpt_to)){ $this->log_write("Error: Cannot send email to ".$rcpt_to."\n"); $sent = FALSE; } fclose($this->sock); $this->log_write("Disconnected from remote host\n"); } return $sent; } /*Private Functions*/ function smtp_send($helo,$from,$to,$header,$body = "") { if(!$this->smtp_putcmd("HELO",$helo)){ return $this->smtp_error("sending HELO command"); } #auth if($this->auth){ if(!$this->smtp_putcmd("UTH LOGIN",base64_encode($this->user))){ return $this->smtp_error("sending HELO command"); } } # if(!$this->smtp_putcmd("MAIL","FROM:<".$from.">")){ return $this->smtp_error("sending MAIL FROM command"); } if(!$this->smtp_putcmd("RCPT","TO:<".$to.">")){ return $this->smtp_error("sending RCPT TO command"); } if(!$this->smtp_putcmd("DATA")){ return $this->smtp_error("sending DATA command"); } if(!$this->smtp_message($header.$body)){ return $this->smtp_error("sending message"); } if(!$this->smtp_eom()){ return $this->smtp_error("sending <CR><LF>.<CR><LF> [EOM]"); } if(!$this->smtp_putcmd("QUIT")){ return $this->smtp_error("sending QUIT command"); } return TRUE; } function smtp_sockopen($address) { if($this->relay_host == ""){ return $this->smtp_sockopen_mx($address); } else{ return $this->smtp_sockopen_relay(); } } function smtp_sockopen_relay() { $this->log_write("Trying to ".$this->relay_host.":".%this->smtp_port."\n"); $this->sock = @fsockopen($this->relay_host,$this->smtp_port,$errno,$errstr,$this->time_out); if(!($this->sock && $this->smtp_ok())){ $this->log_write("Error: Cannot connect to relay host".$this->relay_host."\n"); $this->log_write("Error: ".$errstr."(".$errno.")\n"); return FALSE; } $this->log_write("Connect to relay host".$this->relay_host."\n"); return TRUE; } function smtp_sockopen_mx($address) { $domain = ereg_replace("^.+@([^@]+)$","\1",$address); if(!@getmxrr($domain,$MXHOSTS)){ $this->log_write("Error : Cannot resolve MX \"".$domain."\"\n"); return FALSE; } foreach ($MXHOSTS as $host){ $this->log_write("Trying to ".$host.":".$this->smtp_port."\n"); $this->sock = @fsockopen($host,$this->smtp_port,$errno,$errstr,$this->time_out); if(!($this->sock && $this->smtp_ok())){ $this->log_write("Warning: Cannot connect to mx host".$host."\n"); $this->log_write("Error: ".$errstr."(".$errno.")\n"); continue; } $this->log_write("Connect to mx host".$thost."\n"); return TRUE; } $this->log_write("Error: Cannot connect to any mx hosts(".implode(",",$MXHOSTS).")\N"); return FALSE; } function smtp_message($header,$body) { fputs($this->sock,$header."\r\n.\r\n"); $this->smtp_debug(".[EOM]\n"); return $this->smtp_ok(); } function smtp_ok() { $response = str_replace("\r\n","",fgets($this->sock,512)); $this->smtp_debug($response."\n"); if(!ereg("^[23]",$response)){ fputs($this->sock,"QUIT\r\n"); fgets($this->sock,512); $this->log_write("Error:Remote host returned \"".$response."\"\n"); return FALSE; } return TRUE; } function smtp_putcmd($cmd,$arg = "") { if($arg != ""){ if($cmd == "") $cmd =$arg; else $cmd=$cmd." ".$arg; } fputs($this->sock,$cmd."\r\n"); $this->smtp_debug("> ".$cmd."\n"); return $this->smtp_ok(); } function smtp_error($string) { $this->log_write("Error: Error occurred while ".$string.".\n"); return FALSE; } function log_write($message) { $this->smtp_debug($message); if($this->log_file == ""){ return TRUE; } $message = date("M d H:i:s ").get_current_user()."[".getmypid()."]:".$message; if(!@file_exits($this->log_file) || !($fp = @fopen($this->log_file,"a"))){ $this->smtp_debug("Warning: Cannot open log file \"".$this->log_file."\"\n"); return FALSE; } flock($fp,LOCK_EX); fputs($fp,$message); fclose($fp); return TRUE; } function strip_comment($address) { $comment = "\([^()]*\)"; while (ereg($comment,$address)){ $address = ereg_replace($comment,"",$address); } return $address; } function get_address($address) { $address = ereg_replace("([ \t\r\n])+","",$address); $address = ereg_replace("^.*<(.+)>.*$","\1",$address); return $address; } function smtp_debug($message) { if($this->debug){ echo $message; } } } $file = $argv[2]; $smtpserver = "smtp.qq.com";//SMTP server $smtpserverport = "25";//SMTP server port $smtpusermail = "123456@qq.com";//User mailbox of SMTP server $smtpemailto = "123456@163.com";//Feed water supply $smtpuser = "123456";//User account of SMTP server $smtppass = "123456";//User password for SMTP server $mailsubject = $argv[1];//Mail theme $mailbody = file_get_contents($file);//Mail content $mailtype = "HTML";//Message format (HTML/TXT), TXT is text message $smtp =new smtp($smtpserver,$smtpserverport,true,$smtpuser,$smtppass);//One of the truths here is to use authentication, otherwise authentication will not be used //$SMTP - > debug = true; / / display the debugging information sent $smpt->sendmail($smtpemailto,$smtpusermail,$mailsubject,$mailbody,$mailtype); ?>
Directly run php mail.php "mailbox subject" and "email text directory address" to send. Of course, PHP support is required
6.mail.sh
7. Monitoring disk scripts#!/bin/bash #mail.sh log=$1 t_s=`date +%s` t_s2=`date -d "2 hours ago" +%s` if [ ! -f /tmp/$log ] then echo $t_s2 > /tmp/$log fi t_s2=`tail -1 /tmp/$log|awk '{print $1}'` echo $t_s>>/tmp/$log v=$[$t_s-$t_s2] echo $v if [ $v -gt 3600 ] then /dir/to/php ../mail/mail.php "$1 $2" "$3" echo "0">/tmp/$log.txt else if[ ! -f /tmp/$log.txt ] then echo "0" > /tmp/$log.txt fi nu=`cat /tmp/$log.txt` nu2=$[$nu+1] echo $nu2>/tmp/$log.txt if [ $nu2 -gt 10 ] then /dir/to/php ../mail/mail.php "trouble continue 10 min $1 $2 " "$3" echo "0">/tmp/$log.txt fi fi
#!/bin/bash for r in `df -h|awk -F '[ %]+' '{print $5}'|grep -v Use` do if [ $r -gt 90 ] && [ $send -eq 1 ] then df -h >> ../log/disk.tmp echo "$addr `date +%T` disk useage is $r" >>../log/disk.tmp fi if [ -f ../log/disk.tmp ] then df -h >> ../log/disk.tmp /bin/bash ../mail/mail.sh $addr\_disk $r ../log/disk.tmp echo "`date +%T` disk useage is not ok" else echo "`date +%T` disk useage is ok" fi done