Continued Django 2.0 introductory tutorial section 3 This section describes how to vote in the foreground.
Build a simple form submission page
polls/templates/polls/detail.html
<h1>{{ question.question_text }}</h1> {% if error_message %}<p><strong>{{ error_message }}</strong></p>{% endif %} <form action="{%url 'polls:vote' question.id %}" method="post"> {% csrf_token %} {% for choice in question.choice_set.all %} <input id="choice{{ forloop.counter }}" type="radio" name="choice" value="{{ choice.id }}"> <label for="choice{{ forloop.counter }}">{{ choice.choice_text }}</label><br /> {% endfor %} <br /> <input type="submit" name="" id="" value="vote" /> </form>
Code resolution:
The url submitted by form form is {% url 'poll: vote' question. id%}, which means the vote method of accessing poll / views.py and taking the question id as a parameter.
Traverse the relevant options of the problem to show in the radio box
form forms submit data by post
Configure url
polls/urls.py
path('<int:question_id>/vote/', views.vote, name='vote'),
Screenshot of voting page http://127.0.0.1:8000/polls/1/ :
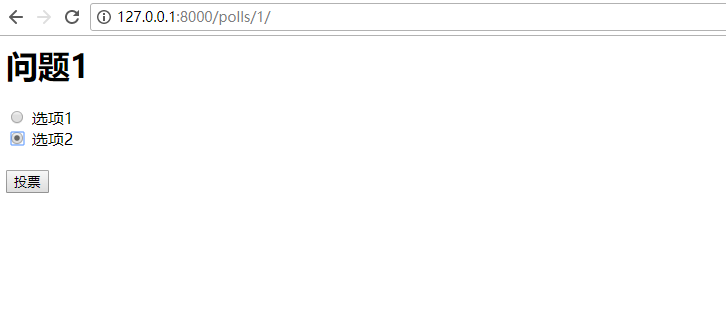
View layer processing submission results
polls/views.py
from django.shortcuts import render, get_object_or_404 from django.http import HttpResponse, HttpResponseRedirect from django.urls import reverse from .models import Question, Choice # ... def vote(request, question_id): question = get_object_or_404(Question, pk=question_id) try: selected_choice = question.choice_set.get(pk=request.POST['choice']) except (KeyError, Choice.DoesNotExist): return render(request, 'polls/detail.html', { 'question': question, 'error_message': "You must select an option", }) else: selected_choice.votes += 1 selected_choice.save() return HttpResponseRedirect(reverse('polls:results', args=(question.id,)))
Code resolution:
request.POST['choice '] receive data submitted by form page
Add 1 to the number of votes and update the database
Show voting results
polls/views.py
from django.shortcuts import render, get_object_or_404 # ... def results(request, question_id): question = get_object_or_404(Question, pk=question_id) return render(request, 'polls/results.html', {'question': question})
results.html
<h1>{{ question.question_text }}</h1> <ul> {% for choice in question.choice_set.all %} <li>{{ choice.choice_text }} -- {{choice.votes}}</li> {% endfor %} </ul> <a href="{% url 'polls:detail' question.id %}">Again?</a>
Submit results page http://127.0.0.1:8000/poll/1/results/
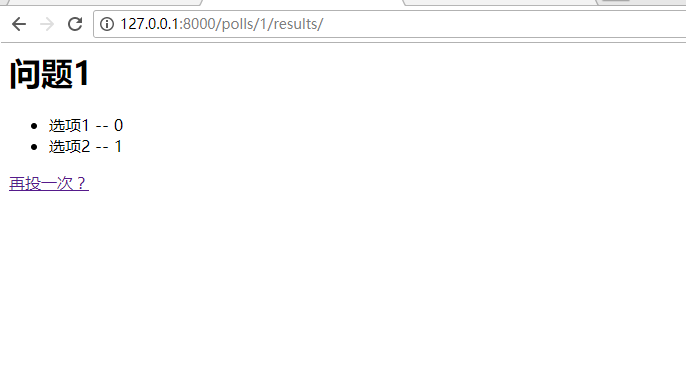
Optimize url and view writing
Another way of writing:
Replace the question? id with the primary key id
polls/urls.py
#_*_coding:utf8_*_ from django.urls import path from . import views app_name = 'polls' urlpatterns = [ path('', views.IndexView.as_view(), name='index'), path('<int:pk>/', views.DetailView.as_view(), name='detail'), path('<int:pk>/results/', views.ResultsView.as_view(), name='results'), path('<int:question_id>/vote/', views.vote, name='vote'), ]
It is more flexible to use < PK > instead of < question ﹣ ID >. < PD > represents the primary key
The corresponding view also needs to be modified to another writing method. The vote method remains the same, which is used to compare the differences between the two writing methods
polls/views.py
#_*_coding:utf8_*_ from django.shortcuts import render, get_object_or_404 from django.http import HttpResponseRedirect from django.urls import reverse from django.views import generic from .models import Question, Choice class IndexView(generic.ListView): template_name = 'polls/index.html' context_object_name = 'latest_question_list' def get_queryset(self): return Question.objects.order_by('-pub_date')[:5] class DetailView(generic.DetailView): model = Question template_name = 'polls/detail.html' class ResultsView(generic.DetailView): model = Question template_name = 'polls/results.html' def vote(request, question_id): # ...
The introductory tutorial will not explain the code in depth. You can roughly understand its function first, and then analyze it module by module
Source download
If you are interested in the Django 2.0 tutorial, please pay attention to my short book, which is continuously updated