Write code ideas flow:
// 1. Privileges and Dependencies // 2. Layout file // 3. Generate entity bean classes // 4. Encapsulation tool class Retrofit + RxJava + OkHttp // 5. Building MVP Architecture // 6. Create an adapter // 7. Loading Layout Manager and Binding Adapter in Activity or Fragment
Design sketch:
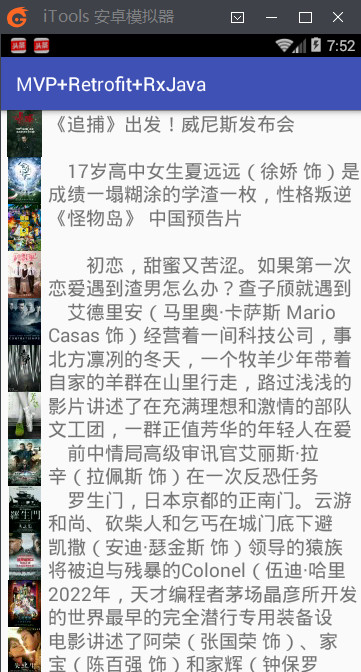
a. Adding Internet access:
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
b. Dependencies: (Dependencies with libs need to be imported into jar packages by themselves or added directly by themselves in online searches)
compile 'com.squareup.okhttp3:okhttp:3.9.0' compile 'io.reactivex:rxjava:1.0.14' compile 'io.reactivex:rxandroid:1.0.1' Compile'com.squareup.retrofit2: retrofit: 2.0.2'//Retrofit2 library file Compoile'com.squareup.retrofit2: converter-gson: 2.0.2'// Support Gson parsing Compoile'com.squareup.retrofit2: adapter-rxjava: 2.1.0'// Support for RxJava compile 'com.jcodecraeer:xrecyclerview:1.3.2' compile 'com.github.bumptech.glide:glide:3.7.0'
Layout file:
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.ccm.mvpretrofitrxjava.activity.MainActivity"> <com.jcodecraeer.xrecyclerview.XRecyclerView android:id="@+id/xrv" android:layout_width="match_parent" android:layout_height="match_parent"></com.jcodecraeer.xrecyclerview.XRecyclerView> </android.support.constraint.ConstraintLayout>
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="50dp" android:orientation="horizontal"> <ImageView android:id="@+id/iv_item" android:layout_width="50dp" android:layout_height="match_parent" /> <TextView android:id="@+id/tv_item" android:layout_width="match_parent" android:layout_height="match_parent" android:text="I am the text." android:textSize="20sp"/> </LinearLayout>
Generate entity bean classes:
public class Bean { private String msg; private RetEntity ret; private String code; public String getMsg() { return msg; } public void setMsg(String msg) { this.msg = msg; } public RetEntity getRet() { return ret; } public void setRet(RetEntity ret) { this.ret = ret; } public String getCode() { return code; } public void setCode(String code) { this.code = code; } public static class RetEntity { private AdvEntity adv; private int pnum; private int totalRecords; private int records; private int totalPnum; private List<?> bannerList; private List<ListEntity> list; public AdvEntity getAdv() { return adv; } public void setAdv(AdvEntity adv) { this.adv = adv; } public int getPnum() { return pnum; } public void setPnum(int pnum) { this.pnum = pnum; } public int getTotalRecords() { return totalRecords; } public void setTotalRecords(int totalRecords) { this.totalRecords = totalRecords; } public int getRecords() { return records; } public void setRecords(int records) { this.records = records; } public int getTotalPnum() { return totalPnum; } public void setTotalPnum(int totalPnum) { this.totalPnum = totalPnum; } public List<?> getBannerList() { return bannerList; } public void setBannerList(List<?> bannerList) { this.bannerList = bannerList; } public List<ListEntity> getList() { return list; } public void setList(List<ListEntity> list) { this.list = list; } public static class AdvEntity { private String imgURL; private String dataId; private String htmlURL; private String shareURL; private String title; public String getImgURL() { return imgURL; } public void setImgURL(String imgURL) { this.imgURL = imgURL; } public String getDataId() { return dataId; } public void setDataId(String dataId) { this.dataId = dataId; } public String getHtmlURL() { return htmlURL; } public void setHtmlURL(String htmlURL) { this.htmlURL = htmlURL; } public String getShareURL() { return shareURL; } public void setShareURL(String shareURL) { this.shareURL = shareURL; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } } public static class ListEntity { private int airTime; private String duration; private String loadtype; private int score; private String angleIcon; private String dataId; private String description; private String loadURL; private String shareURL; private String pic; private String title; private String roomId; public int getAirTime() { return airTime; } public void setAirTime(int airTime) { this.airTime = airTime; } public String getDuration() { return duration; } public void setDuration(String duration) { this.duration = duration; } public String getLoadtype() { return loadtype; } public void setLoadtype(String loadtype) { this.loadtype = loadtype; } public int getScore() { return score; } public void setScore(int score) { this.score = score; } public String getAngleIcon() { return angleIcon; } public void setAngleIcon(String angleIcon) { this.angleIcon = angleIcon; } public String getDataId() { return dataId; } public void setDataId(String dataId) { this.dataId = dataId; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } public String getLoadURL() { return loadURL; } public void setLoadURL(String loadURL) { this.loadURL = loadURL; } public String getShareURL() { return shareURL; } public void setShareURL(String shareURL) { this.shareURL = shareURL; } public String getPic() { return pic; } public void setPic(String pic) { this.pic = pic; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getRoomId() { return roomId; } public void setRoomId(String roomId) { this.roomId = roomId; } } } }
Packaging tool class:
RequestApi interface
public interface RequestApi { public static final String BASE_URL = "http://api.svipmovie.com/front/"; //get and RxJava @GET("columns/getVideoList.do?catalogId=402834815584e463015584e539330016&pnum=2") Observable<Bean> getData(); @Streaming @POST("{fileName}") Call<ResponseBody> downloadFile(@Path("fileName") String fileName, @Header("Range") String range); @Streaming @POST("{fileName}") Call<ResponseBody> getFileLenght(@Path("fileName") String fileName); }
RetrofitUtils class:
public class RetrofitUtils { //Support RxJava public static RequestApi getNetDatas(){ OkHttpClient client = new OkHttpClient.Builder() .connectTimeout(5, TimeUnit.SECONDS) .readTimeout(5, TimeUnit.SECONDS) // .addNetworkInterceptor(new MyInterceptro()) .build(); Retrofit retrofit = new Retrofit.Builder() .addCallAdapterFactory(RxJavaCallAdapterFactory.create()) .addConverterFactory(GsonConverterFactory.create()) .client(client) .baseUrl(RequestApi.BASE_URL) .build(); RequestApi api = retrofit.create(RequestApi.class); return api; } public static RequestApi download(){ OkHttpClient client = new OkHttpClient.Builder() .connectTimeout(5, TimeUnit.SECONDS) .readTimeout(5, TimeUnit.SECONDS) //.addNetworkInterceptor(new MyInterceptro()) .build(); Retrofit retrofit = new Retrofit.Builder() .client(client) .baseUrl("http://10.0.2.2:8080/aaa/") .build(); RequestApi api = retrofit.create(RequestApi.class); return api; } }
view level:
public interface IRecyView { void showRecy(Bean bean); }
model level:
IRecyModel interface
public interface IRecyModel { void recy(Observer<Bean> observer); }RecyModel is the implementation class of IRecyViewpublic class RecyModel implements IRecyModel { @Override public void recy(Observer<Bean> observer) { //OkHttpUtils.getInstance().doGet("http://api.svipmovie.com/front/columns/getVideoList.do?catalogId=402834815584e463015584e539330016", callback); RetrofitUtils.getNetDatas().getData() .subscribeOn(Schedulers.io()) //Define the observer to execute in a subthread .observeOn(AndroidSchedulers.mainThread()) //Define observer execution in the main thread .subscribe(observer); } }presenter layer:
public class RecyPresenter { private IRecyView view; private IRecyModel model; public RecyPresenter(IRecyView view) { this.view = view; model = new RecyModel(); } public void showRecy(){ model.recy(new Observer<Bean>() { @Override public void onCompleted() { Log.i("I use retrofit+RxJava All right.", "onCompleted: "); } @Override public void onError(Throwable e) { Log.i("I use retrofit+RxJava All right.", "onError: "); } @Override public void onNext(Bean bean) { Log.i("I use retrofit+RxJava All right.", "onNext: "); view.showRecy(bean); } }); } //Untying public void onDestory(){ view = null; } }Adapter:
public class MyRecyAdapter extends RecyclerView.Adapter<MyRecyAdapter.MyViewHolder>{ private Context context; private Bean bean; public MyRecyAdapter(Context context, Bean bean) { this.context = context; this.bean = bean; } @Override public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View view = LayoutInflater.from(context).inflate(R.layout.recy_item, parent, false); MyViewHolder holder = new MyViewHolder(view); return holder; } @Override public void onBindViewHolder(MyViewHolder holder, int position) { Glide.with(context).load(bean.getRet().getList().get(position).getPic()).into(holder.iv_item); holder.tv_item.setText(bean.getRet().getList().get(position).getDescription()); } @Override public int getItemCount() { return bean.getRet().getList().size(); } static class MyViewHolder extends RecyclerView.ViewHolder{ ImageView iv_item; TextView tv_item; public MyViewHolder(View itemView) { super(itemView); iv_item = itemView.findViewById(R.id.iv_item); tv_item = itemView.findViewById(R.id.tv_item); } } }
MainActivity:
//1. Privileges and Dependencies //2. Layout file //3. Generate entity bean classes //4. Encapsulation tool class Retrofit + RxJava + OkHttp //5. Building MVP Architecture //6. Create an adapter //7. Loading Layout Manager and Binding Adapter in Activity or Fragment public class MainActivity extends AppCompatActivity implements IRecyView{ private XRecyclerView xrv; private RecyPresenter presenter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initView(); presenter = new RecyPresenter(this); presenter.showRecy(); } private void initView() { xrv = (XRecyclerView) findViewById(R.id.xrv); LinearLayoutManager manager = new LinearLayoutManager(this); manager.setOrientation(LinearLayoutManager.VERTICAL); xrv.setLayoutManager(manager); //Here's how to load more methods } @Override public void showRecy(Bean bean) { MyRecyAdapter myRecyAdapter = new MyRecyAdapter(this, bean); xrv.setAdapter(myRecyAdapter); } }