redis series articles:
redis series (1) - installation and startup
redis series (2) - syntax and commands
1. Brief description
The key value types supported by Redis are String character type, map hash type, list list list type, set collection type, sortedset ordered collection type.This article summarizes the use of these key value types and introduces the Keys command. Although the syntax is simple, it needs more practice because of the large number.
2. String Character Types
1. Assignment
Syntax: SET key value
127.0.0.1:6379> set test 123 OK
2. Value
Syntax: GET key
127.0.0.1:6379> get test "123"
3. Value and assign
Syntax: GETSET key value
127.0.0.1:6379> getset s2 222 "111" 127.0.0.1:6379> get s2 "222"
4. Set/Get Multiple Key Values
Grammar:
- MSET key value [key value ...]
- MGET key [key ...]
127.0.0.1:6379> mset k1 v1 k2 v2 k3 v3 OK 127.0.0.1:6379> get k1 "v1" 127.0.0.1:6379> mget k1 k3 1) "v1" 2) "v3"
5. Delete
Syntax: DEL key
127.0.0.1:6379> del test (integer) 1
6. Value increase or decrease
a. Incremental numbers
When the stored string is an integer, redis provides a useful command, INCR, that increments the current key value and returns the incremented value.
Syntax: INCR key
127.0.0.1:6379> incr num (integer) 1 127.0.0.1:6379> incr num (integer) 2 127.0.0.1:6379> incr num (integer) 3
b. Increase specified integer
Syntax: INCRBY key increment
127.0.0.1:6379> incrby num 2 (integer) 5 127.0.0.1:6379> incrby num 2 (integer) 7 127.0.0.1:6379> incrby num 2 (integer) 9
c. Decreasing values
Syntax: DECR key
127.0.0.1:6379> decr num (integer) 9 127.0.0.1:6379> decr num (integer) 8
d. Reduce specified integer
Syntax: DECRBY key decrement
127.0.0.1:6379> decr num (integer) 6 127.0.0.1:6379> decr num (integer) 5 127.0.0.1:6379> decrby num 3 (integer) 2 127.0.0.1:6379> decrby num 3 (integer) -1
7. Append value to tail
append appends the value to the end of the key value.If the key does not exist, set the value of the key to value, which is equivalent to set key value.The return value is the total length of the appended string.
Syntax: APPEND key value
127.0.0.1:6379> set str hello OK 127.0.0.1:6379> append str " world!" (integer) 12 127.0.0.1:6379> get str "hello world!"
8. Get String Length
The STRLEN command returns the length of the key value, or 0 if the key does not exist.
Syntax: STRLEN key
127.0.0.1:6379> strlen str (integer) 0 127.0.0.1:6379> set str hello OK 127.0.0.1:6379> strlen str (integer) 5
3. map hash type
In redis, map is also called hash.Assuming a User object is stored in Redis as JSON serialization, and a User object has attributes such as id, username, password, age, name, etc., the stored procedure is as follows:
Save, update: User object --> JSON (string) --> redis.
What should I do if the business only updates the age attribute, and the other attributes do not update?If the above method still causes waste of resources in transmission and processing, hash can solve this problem very well.
Introduction to redis hash
Hash is called hash type and provides mapping of fields and field values.Field values can only be of string type.
Other types such as hash type, collection type, etc. are not supported.The following:
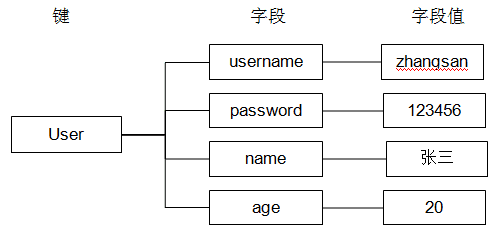
1. Assignment
The HSET command does not distinguish between an insert and a temporary update operation, and returns 1 when an insert operation is performed and 0 when an update operation is performed.
Set one field value at a time
Syntax: HSET key field value
127.0.0.1:6379> hset user username zhangesan (integer) 1
Setting multiple field values at once
Syntax: HMSET key field value [field value...]
127.0.0.1:6379> hmset user age 20 username lisi OK
Assignment when the field does not exist, similar to HSET, except that if the field exists, the command does nothing
Syntax: HSETNX key field value
127.0.0.1:6379> hsetnx user age 30 (integer) 0
If there is no age field in the user, set the age value to 30, otherwise do nothing.
2. Value
Get one field value at a time
Syntax: HGET key field
127.0.0.1:6379> hget user username "zhangesan"
Get multiple field values at once
Syntax: HMGET key filed[field...]
127.0.0.1:6379> hmget user age username 1) "20" 2) "lisi"
Get all field values
Syntax: HGETALL key
127.0.0.1:6379> hgetall user 1) "age" 2) "20" 3) "username" 4) "lisi"
3. Delete Field
One or more fields can be deleted, and the return value is the number of fields deleted.
Syntax: HDEL key field [field...]
127.0.0.1:6379> hdel user age (integer) 1 127.0.0.1:6379> hdel user age name (integer) 0 127.0.0.1:6379> hdel user age username (integer) 1
4. Increase Numbers
Syntax: HINCRBY key field increment
127.0.0.1:6379> hincrby user age 2 Increase user's age by 2 (integer) 22 127.0.0.1:6379> hget user age Get the age of the user "22"
5. Determine whether a field exists
Syntax: HEXISTS key field
127.0.0.1:6379> hexists user age See user Is there any age field (integer) 1 127.0.0.1:6379> hexists user name See user Is there any name field (integer) 0
6. Get only the field name or value
Grammar:
- HKEYS key
- HVALS key
127.0.0.1:6379> hmset user age 20 name lisi OK 127.0.0.1:6379> hkeys user 1) "age" 2) "name" 127.0.0.1:6379> hvals user 1) "20" 2) "lisi"
7. Get Number of Fields
Syntax: HLEN key
127.0.0.1:6379> hlen user (integer) 2
4. list List Type
The difference between Arraylist and linkedlist
- ArrayList uses arrays to store data with the following features: fast queries, slow growth and deletion
- LinkedList uses a two-way chain table to store data. It is characterized by fast growth and deletion, slow query, but fast query of data at both ends of the chain table.
The list of redis is stored in a chain table, so the operation of the list data type of redis is to operate on both ends of the list.
1. Add elements to both ends of the list
Add elements to the left of the list
Syntax: LPUSH key value [value...]
127.0.0.1:6379> lpush list1 1 2 3 (integer) 3
Add elements to the right of the list
127.0.0.1:6379> rpush list1 4 5 6 (integer) 3
2. View List
The LRANGE command is one of the most common commands for list types. Getting a fragment in a list returns all elements (including elements at both ends) between start and stop, with an index starting at 0.Indexes can be negative numbers, such as:'-1'represents the rightmost element.
Syntax: LRANGE key start stop
127.0.0.1:6379> lrange list1 0 2 1) "1" 2) "2" 3) "3" 127.0.0.1:6379> lrange list1 0 -1 1) "1" 2) "2" 3) "3" 4) "4" 5) "5" 6) "6"
3. Pop elements from both ends of the list
The LPOP command pops up an element from the left of the list in two steps:
Step 1: Remove the elements to the left of the load list from the list
Step 2: Return the removed element value.
Grammar:
- LPOP key
- RPOP key
127.0.0.1:6379> lpop list1 "1" 127.0.0.1:6379> rpop list1 "6"
4. Get the number of elements in the list
Syntax: LLEN key
127.0.0.1:6379> llen list1 (integer)4
5. Delete the value specified in the list
The LREM command deletes the elements in the list whose first count value is value, returning the actual number of elements deleted.This command will execute differently depending on the count value.
- When count > 0, LREM is deleted from the left of the list.
- When count <0, LREM is deleted from the right side of the list.
- When count=0, LREM deletes all elements with value.
Syntax: LREM key count value
127.0.0.1:6379> lrem list1 1 4 Remove elements with a value of 4 from the left (integer)1
6. Get/set the element value of the specified index
Gets the element value of the specified index
Syntax: LINDEX key index
127.0.0.1:6379> lindex list1 2 "5"
Set element value for specified index
Syntax: LSET key index value
127.0.0.1:6379> lset list1 2 4 OK 127.0.0.1:6379> lrange list1 0 -1 1) "2" 2) "3" 3) "4"
7. Keep only the fragments specified in the list
Specified scope is consistent with LRANGE
Syntax: LTRIM key start stop
127.0.0.1:6379> lrange list1 0 -1 1) "2" 2) "3" 3) "4" 127.0.0.1:6379> ltrim list1 0 1 OK 127.0.0.1:6379> lrange list1 0 -1 1) "4"
8. Insert an element into the list
This command first looks for pivot-valued elements from left to right in the list, then decides whether to insert a value before or after the element based on whether the second parameter is BEFORE or AFTER.
Syntax: LINSERT key BEFORE|AFTER pivot value
127.0.0.1:6379> lrange list1 0 -1 1) "4" 127.0.0.1:6379> linsert list1 after 4 5 (integer) 2 127.0.0.1:6379> lrange list1 0 -1 1) "4" 2) "5"
9. Transfer elements from one list to another
Syntax: RPOPLPUSH source destination
127.0.0.1:6379> rpoplpush list1 list2 "5" 127.0.0.1:6379> lrange list2 0 -1 1)"5" 127.0.0.1:6379> lrange list1 0 -1 1) "4"
5. set Collection Type
The difference between set and list:
- Set type: Unordered, non-repeatable
- List type: ordered, repeatable
1. Add/Remove Elements
Syntax: SADD key member [member...]
127.0.0.1:6379> sadd set1 a b c (integer)3 127.0.0.1:6379> sadd set1 a (integer)0
Syntax: SREM key memeber [member...]
127.0.0.1:6379> srem set1 c d d Not in collection, so only remove c (integer)1
2. Get all the elements in the collection
Syntax: SMEMBERS key
127.0.0.1:6379> smemebers set1 1)"b" 1)"a"
3. Determine if an element is in a set
Syntax: SISMEMBER key member
127.0.0.1:6379> sismember set1 a (integer)1 127.0.0.1:6379> sismember set1 h (integer)0
4. Operational commands
a. Difference set operation of sets A - B
A collection of elements belonging to A and not B.
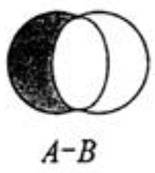
Syntax: SDIFF key [key...]
127.0.0.1:6379> sadd setA 1 2 3 (integer)3 127.0.0.1:6379> sadd setB 2 3 4 (integer)3 127.0.0.1:6379> sdiff setA setB 1)"1" 127.0.0.1:6379> sdiff setB setA 1)"4"
B. Intersection operation of sets A_B
A collection of elements belonging to A and B.
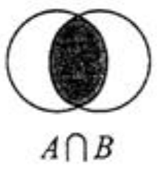
Syntax: SINTER key [key...]
127.0.0.1:6379> sinter setA setB 1)"2" 2)"3"
c. Union operation of sets A_B
A collection of elements belonging to A or B.
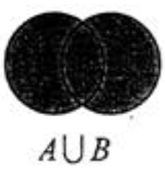
Syntax: SUNION key [key...]
127.0.0.1:6379> sunion setA setB 1) "1" 2) "2" 3) "3" 4) "4"
5. Get the number of elements in the collection
Syntax: SCARD key
127.0.0.1:6379> smembers setA 1) "1" 2) "2" 3) "3" 127.0.0.1:6379> scard setA (integer)3
6. Pop an element out of a collection
Note: Since the set is out of order, the SPOP command randomly selects an element from the set to pop up.
Syntax: SPOP key
127.0.0.1:6379> spop setA "1"
6. sortedset ordered set type
Sortedset, also known as zset, is an ordered set that can be sorted but unique.Sortedset differs from set in that it adds a score to the elements in the set and sorts them by that score.
1. Add Elements
Add an element and its fraction to an ordered set, and if the element already exists, replace the original fraction with a new fraction.The return value is the number of elements that are newly added to the collection and does not contain elements that previously existed.
Syntax: ZADD key score member [score member...]
127.0.0.1:6379> zadd scoreboard 80 zhangesan 89 lisi 94 wangwu (integer)3 127.0.0.1:6379> zadd scoreboard 97 lisi (integer)0
2. Get the fraction of an element
Syntax: ZSCORE key member
127.0.0.1:6379> zscore scoreboard lisi "97"
3. Delete Elements
Remove one or more members from the ordered set key, and nonexistent members will be ignored.
An error is returned when the key exists but is not an ordered set type.
Syntax: ZREM key member [member...]
127.0.0.1:6379> zrem scoreboard lisi (integer)1
4. Get a list of elements ranked in a range
a. Returns all elements (including elements at both ends) indexed from start to stop in the order from smallest to largest element fractions
Syntax: ZRANGE key start stop [WITHSCORES]
127.0.0.1:6379> zrange scoreboard 0 2 1)"zhangesan" 2)"wangwu" 3)"lisi"
b. Returns all elements (including elements at both ends) indexed from start to stop in the order of element fraction from large to small
Syntax: ZREVRANGE key start stop [WITHSCORES]
127.0.0.1:6379> zrevrange scoredboard 0 2 1)"lisi" 2)"wangwu" 3)"zhangesan"
If you need to get a fraction of an element, you can add the WITHSCORES parameter at the end of the command
127.0.0.1:6379> zrange scoreboard 0 1 WITHSCORES 1) "zhangsan" 2) "80" 3) "wangwu" 4) "94"
5. Get ranking of elements
a. From small to large
Syntax: ZRANK key member
127.0.0.1:6379> zrank scoreboard lisi (integer)0
b. From large to small
Syntax: ZREVRANK key member
127.0.0.1:6379> zrevrank scoreboard zhangsan (integer)1
6. Get the elements of the specified score range
Syntax: ZRANGEBYSCORE key min max [WITHSCORES][LIMIT offset count]
127.0.0.1:6379> zrangebyscore scoreboard 90 97 WITHSCORES 1) "wangwu" 2) "94" 3) "lisi" 4) "97" 127.0.0.1:6379> zrangebyscore scoreboard 70 100 limit 1 2 1) "wangwu" 2) "lisi"
7. Increase the fraction of an element
The return value is the changed score
Syntax: ZINCRBY key increment member
127.0.0.1:6379> zincrby scoreboard 4 lisi "101"
8. Get the number of elements in the collection
Syntax: ZCARD key
127.0.0.1:6379> zcard scoreboard (integer)3
9. Get the number of elements within the specified score range
Syntax: ZCOUNT key min max
127.0.0.1:6379> zcount scoreboard 80 90 (integer) 1
10. Delete elements by rank range
Syntax: ZREMRANGEBYRANK key start stop
127.0.0.1:6379> zremrangebyrank scoreboard 0 1 (integer)2 127.0.0.1:6379> zrange scoreboard 0 -1 1)"lisi"
11. Delete elements by score range
Syntax: ZREMRANGEBYSCORE key min max
127.0.0.1:6379> zadd scoreboard 84 zhangsan (integer)1 127.0.0.1:6379> zremrangebyscore scoreboard 80 100 (integer)1
7. Keys Command
1,keys
Returns all key s that satisfy a given pattern
127.0.0.1:6379> keys mylist* 1) "mylist" 2) "mylist5" 3) "mylist6" 4) "mylist7" 5) "mylist8"
2,exists
Confirm the existence of a key
Example: From the results, the key Hong Wan does not exist in the database, but the key age does exist
127.0.0.1:6379> exists HongWan (integer)0 127.0.0.1:6379> exists age (integer)1
3,del
Delete a key
127.0.0.1:6379> del age (integer)1 127.0.0.1:6379> exists age (integer)0
4,rename
Rename key
Example: Change age to age_new.
127.0.0.1:6379> keys * 1) "age" 127.0.0.1:6379> rename age age_new OK 127.0.0.1:6379>keys * 1) "age_new"
5,type
Type of return value
Example: Determine the type of value
127.0.0.1:6379> type addr string 127.0.0.1:6379> type myzset2 set 127.0.0.1:6379> type mylist list
6. Set the key's lifetime
redis are more often used as caches in practice, but cached data generally requires a lifetime, that is, data is destroyed after expiration.
EXPIRE key seconds sets the key's lifetime in seconds. The key is automatically deleted after how many seconds TTL key sets the remaining lifetime of the key PERSIST key Clear Lifetime PEXPIRE key milliseconds lifetime is set in milliseconds
Example:
127.0.0.1:6379> set test 1 Set up test Value of 1 OK 127.0.0.1:6379> get test Obtain test Value of "1" 127.0.0.1:6379> EXPIRE test 5 Set up test Lifetime is 5 seconds (integer)1 127.0.0.1:6379> TTL test See test Generation time of 1 second to delete (integer)1 127.0.0.1:6379> TTL test (integer) -2 127.0.0.1:6379> get test Obtain test Value of, deleted (nil)