Redis
1. Concept: redis is a high-performance NOSQL series Philippine relational database.
2. Comparison between NoSQL and relational database
-
Relational database: mysql, Oracle
1. Data is related
2. Data is stored on the hard disk file -
Non relational database (NoSQL): redis, hbase
1. No association between data: storage key:value
2. Data is stored in memory -
Generally, the data will be stored in the relational database, and the data of the relational database will be backed up and stored in the nosql database.
3. Download the installation directory
- redis.wnidow.conf : Profile
- redis-cli.exe : redis client
- redis-server.exe ; redis server
4. Command operation
1. Data structure of redis
*redis stores data in the format of key and value, in which key is a string and value has five different data structures.
-
Data structure of value:
1) string type
2) Hash type hash: map format
3) List type list: linkedlist format, supporting duplicate elements
4) Set type set: duplicate elements are not allowed
5) Ordered set type sortedset: duplicate elements are not allowed, but elements have order
2. string type
- Store: set key value
- Get: get key
- Delete: del key
3. Hash type hash
- Store: hset key field value
- obtain:
- hget key field: get the value corresponding to the specified field
- hgetall key: get all field s and value s
- Delete: hdel key field
4. List type list: you can add an element to the head (left) or tail (right) of the list
- Storage:
- lpush key value: add elements to the left table of the list
- rpush key value: add elements to the right table of the list
- obtain:
- lrange key start end: range get 0 - 1 get all
- Delete:
- lpop key: delete the leftmost element of the list and return the element
- rpop key: delete the rightmost element of the list and return the element
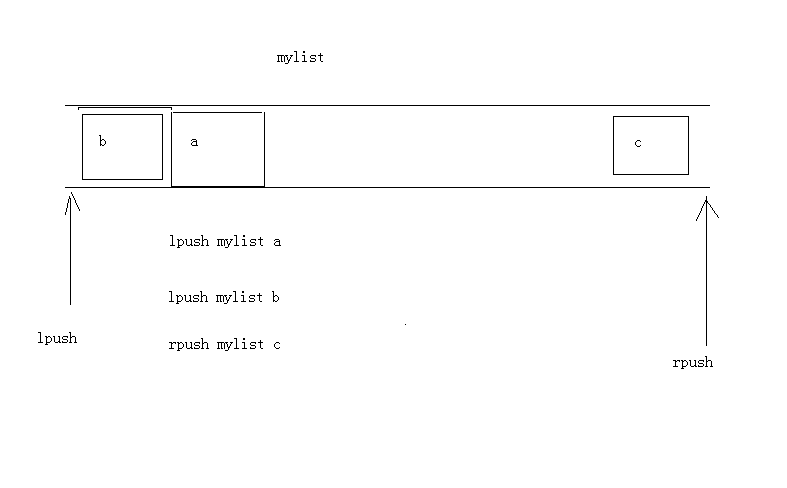
5. Set type set: duplicate elements are not allowed, and elements have no order
- Store: sadd key value
- Get: smembers key: get all elements in the set set
- Delete: srem key value: delete an element in the set set
6. Ordered set type sortedset: duplicate elements are not allowed, and elements have order
- Store: zadd key score value
- Get: zrange key start end
- Delete: zrem key value
7. General order
- Keys *: query all keys
- type key: get the type of value corresponding to the key
- del key: delete the specified key value
5. Data persistence
Persistence
-Redis is a memory database. When the redis server is restarted or the data is lost when the computer is restarted, the data in the redis memory can be persisted to the hard disk file.
-redis persistence mechanism:
1.RDB: the default mode, which does not need to be configured.
*In a certain interval, the change of key is detected, and then the data is persisted
1. Edit redis.window.conf file
# after 900 sec (15 min) if at least 1 key changed
save 900 1
# after 300 sec (5 min) if at least 10 keys changed
save 300 10
# after 60 sec if at least 10000 keys changed
save 60 10000
2. Restart the redis server and make the profile name
Command line: redis absolute path > redis- server.exe redis.window.conf
2.AOF: the way of logging, which can record the operation of each command, and can persist the data after each command operation.
1. Edit redis.window.conf File appendonly no (close AOF) - > appendonly Yes (open AOF)
- appendfsync always: persist every operation
- appendfsync everysec: persistent every second
- appendfsync no: no persistence
Java client Jedis
- Jedis: a java tool for operating redis database
- Use steps:
1. Download Jedis jar package
2. Use
//1. Get connection
Jedis jedis=new Jedis("localhost",6379);
//2. Operation
jedis.set("username","zhangsan");
//3. Close the connection
jedis.close();
-Jedis operates on data structures in various redis
1) string type
set()
get()
/* string Data structure operation */ public static void test1(){ //1. Get connection Jedis jedis=new Jedis("localhost",6379);//Default value "localhost",6379 //2. Operation jedis.set("username","zhangsan"); String username = jedis.get("username"); System.out.println(username); //You can use the setex() method to store the key value that can specify the expiration time jedis.setex("actioncode",20,"hello");//Put a ctioncode:hello The key value pair is stored in redis and automatically deleted after 20 seconds //3. Close the connection jedis.close(); }
2) Hash type hash: map format
hset()
hget()
hgetAll()
/* hash Data structure operation */ public static void test2(){ //1. Get connection Jedis jedis=new Jedis("localhost",6379);//Default value "localhost",6379 //2. Operation jedis.hset("user","name","zhangsan"); jedis.hset("user","age","20"); jedis.hset("user","gender","man"); // String hget = jedis.hget("user", "gender"); // System.out.println(hget); Map<String, String> user = jedis.hgetAll("user"); Set<String> keySet = user.keySet(); for (String key:keySet){ String value = user.get(key); System.out.println(key+"::"+value); } //3. Close the connection jedis.close(); }
3) List type list: linkedlist format, supporting duplicate elements
lpush/rpush()
lpop/rpop()
lrange() start end: range get
/* list Data structure operation */ public static void test3(){ //1. Get connection Jedis jedis=new Jedis("localhost",6379);//Default value "localhost",6379 //2. Operation jedis.lpush("mylist","a","b","c"); jedis.rpush("mylist","a","b","c"); List<String> mylist = jedis.lrange("mylist", 0, -1); System.out.println(mylist); String c1 = jedis.lpop("mylist"); System.out.println(c1); String c2 = jedis.rpop("mylist"); System.out.println(c2); List<String> mylist1 = jedis.lrange("mylist", 0, -1); System.out.println(mylist1); //3. Close the connection jedis.close(); }
4) Set type set: duplicate elements are not allowed
sadd()
smembers()
/* set Data structure operation */ public static void test4(){ //1. Get connection Jedis jedis=new Jedis("localhost",6379);//Default value "localhost",6379 //2. Operation jedis.sadd("myset","java","php","c++"); Set<String> myset = jedis.smembers("myset"); System.out.println(myset); //3. Close the connection jedis.close(); }
5) Ordered set type sortedset: duplicate elements are not allowed, but elements have order
zadd()
zrange()
/* sortedset Data structure operation */ public static void test5(){ //1. Get connection Jedis jedis=new Jedis("localhost",6379);//Default value "localhost",6379 //2. Operation jedis.zadd("mysortedset",2,"Is it"); jedis.zadd("mysortedset",1,"Yasuo"); jedis.zadd("mysortedset",3,"Big bug"); Set<String> mysortedset = jedis.zrange("mysortedset", 0, -1); System.out.println(mysortedset); //3. Close the connection jedis.close(); }
-Jedis connection pool: JedisPool
*Use steps:
1. Create a JedisPool object
2. Get connection
3. Use
4. Close the connection and return it to the connection pool
/* Jedis Use of connection pools */ public static void test6(){ //0. Create a configuration object JedisPoolConfig config=new JedisPoolConfig(); config.setMaxTotal(50); config.setMaxIdle(10); //1. Create a Jedis connection pool object JedisPool jedisPool=new JedisPool(config,"localhost",6379); //2. Get connection Jedis jedis = jedisPool.getResource(); //3. Use jedis.set("haha","heihei"); String haha = jedis.get("haha"); System.out.println(haha); //4. Close the connection and return it to the connection pool jedis.close(); }
-Jedis connection pool utility class
JedisPoolUtils
/* Jedis Use of connection pool tool class */ public static void test8(){ //1. Use the Jedis connection pool tool class to get the connection object Jedis jedis = JedisPoolUtils.getJedis(); //2. Use jedis.set("hello","word"); String hello = jedis.get("hello"); System.out.println(hello); //3. Close and return the connection to the connection pool jedis.close(); }
...
Jedis case
Requirements:
1. Provide index.html Page with a drop-down list of provinces.
2. When the page is loaded, send an ajax request to load all provinces.
Note: use redis to cache some data that doesn't change frequently.
-
Once the data of the database changes, the cache needs to be updated.
-
The tables in the database need to add, delete, and modify. The redis cache data needs to be saved again
-
In the add / delete / modify method corresponding to service, delete the redis data.