catalogue
Sentence classification in C language
Branch statement (select structure)
Sentence classification in C language
- Expression statement
- Function call statement
- Control statement
- Compound statement
- Empty statement
All statements in C language end with a semicolon (;).
- Expression statement:
int a = 10;
int b = 20;
int c = 0;
int c = a + b; // Expression statement
- Empty statement:
Nothing before the semicolon is an empty statement.
- Function call statement:
Using functions provided by C language or calling custom functions are function call statements.
Control statement
Control statements are used to control the execution flow of the program to realize various structural modes of the program. They are composed of specific statement definer. There are nine control statements in C language.
It can be divided into the following three categories:
- Conditional judgment statements are also called branch statements: if statements and switch statements;
- Circular execution statements: do while statement, while statement and for statement;
- Turn statements: break statements, goto statements, continue statements, and return statements.
Branch statement (select structure)
We have learned about branch statements in C language:
- if... else statement
- switch sentence
Explain the branch statement in detail
1, if... else statement
1. Grammatical structure
- Single branch
If (expression)
sentence;
If the expression is true, the statement is executed; if the expression is false, the statement is not executed (nothing is output).
- Double branch
If (expression)
Statement 1;
else
Statement 2;
If the expression is true, statement 1 is executed, and if the expression is false, statement 2 is executed.
Execute only one of the statements.
- Multi branch
If (expression 1)
Statement 1;
Else if (expression 2)
Statement 2;
else
Statement 3;
If expression 1 is true, statement 1 is executed.
If expression 1 is false and expression 2 is true, statement 2 is executed.
If both expression 1 and expression 2 are false, statement 3 is executed.
Only one of the three statements will execute.
In C language: 0 means false, and non-0 means true
2. Code demonstration:
- Single branch:
#include<stdio.h> int main() { int age = 0; scanf("%d", &age); if (age >= 18) printf("adult\n"); return 0; }
The expression is true:
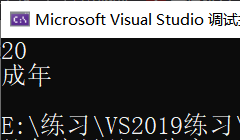
Expression is false:
- Double branch
#include<stdio.h> int main() { int age = 0; scanf("%d", &age); if (age >= 18) printf("adult\n"); else printf("under age\n"); return 0; }
The expression is true:
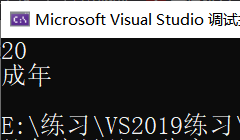
Expression is false:
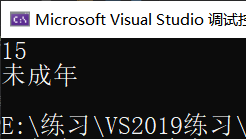
- Multi branch
#include<stdio.h> int main() { int age = 0; scanf("%d", &age); if (age < 18) printf("juvenile\n"); else if (age >= 18 && age < 30) printf("youth\n"); else if (age >= 30 && age < 50) printf("Prime of life\n"); else if (age >= 50 && age < 90) printf("old age\n"); else printf("God of Longevity\n"); return 0; }
Operation results:
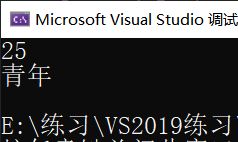
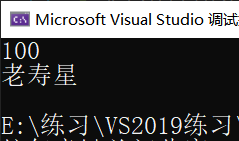
Simplified code:
#include<stdio.h> int main() { int age = 0; scanf("%d", &age); if (age < 18) printf("juvenile\n"); else if (age < 30) printf("youth\n"); else if (age < 50) printf("Prime of life\n"); else if (age < 90) printf("old age\n"); else printf("God of Longevity\n"); return 0; }
The first method is more readable.
3. Suspended else
#include <stdio.h> int main() { int a = 0; int b = 2; if (a == 1) if (b == 2) printf("hehe\n"); else printf("haha\n"); return 0; }
Operation results:
From the running results, we can see that nothing is printed after the program is executed.
Code analysis:
Because int a = 0, the expression 1 (a==1) is false, and the program will not execute the if (b = = 2) statement. But else matches if (b = = 2). It is executed only if expression 1 (a==1) is true If (b = = 2) statement, so nothing is output after the program is executed.
Else matching: else matches the nearest if.
Supplement: in C language:
- =Is an assignment operator
- ==Is an equal sign operator to determine whether the values are equal.
Adding {} can make this code clearer:
#include <stdio.h> int main() { int a = 0; int b = 2; if (a == 1) { if (b == 2) printf("hehe\n"); else printf("haha\n"); } return 0; }
4. Comparison of writing forms of if statements
If (expression) Expressions can be constants or variables
int age = 0;
scanf("%d", &age);
if (age)
{
}
If the condition holds (the expression is true), you should use code blocks (enclosed by {}) to execute multiple statements
#include<stdio.h> int main() { int age = 0; scanf("%d", &age); if (age >= 18) { printf("adult\n"); printf("Can fall in love\n"); } else { printf("under age\n"); } return 0; }
Operation results:
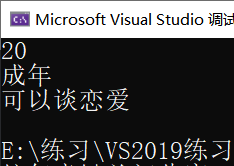
In this code, you can also not enlarge the parentheses after else. It is recommended to add braces no matter how many statements are after if, and there will be no error. If not, sometimes writing code is prone to error. (see personal habits).
Look at the following code:
#include<stdio.h> int main() { int a=0; scanf("%d", &a); //Code 1 if (a) { printf("hehe\n"); } printf("haha\n"); //Code 2 if (a) { printf("hehe\n"); } else { printf("haha\n"); } return 0; }
The execution result of code 1 is the same as that of code 2. A is 0 to print haha, and a is non-0 to print hehe.
However, we can find that code 2 is more readable than code 1.
5. Error prone point
- It's easy to make mistakes
If (expression) The expression is: judge whether the conditions are met, which is easy to write in the form of mathematics.
#include<stdio.h> int main() { int age = 0; scanf("%d", &age); //First: if (age >= 18 && age < 40) { printf("1:ha-ha\n"); } //Second: if (18 <= age < 40) { printf("2:ha-ha\n"); } return 0; }
The expression is true:
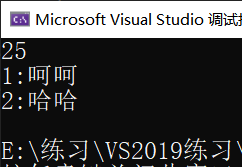
Seeing this, one may think that both pieces of code are correct. But are they all true?
Let's look at the expression that is false:
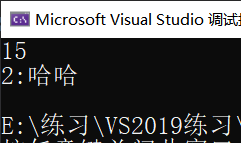
The second writing method has no grammatical errors, but it does not meet the requirements logically.
18 < = age < 40: calculate 18 < = 15 first (at this time, the entered age is 15), and the result is 0 (false); If 0 < 40 is calculated again, the result is 1 (true), so 2 will be printed: ha ha.
- Error prone point 2
If (expression) The expression is: judge whether the constant and variable values are equal. It is easy to write two equal signs into one equal sign.
#include<stdio.h> int main() { //Code 1 int num = 10; if (num == 5) { printf("hehe\n"); } //Code 2 int num = 10; if (5 == num) { printf("hehe\n"); } return 0; }
Sometimes when writing conditional judgment, it is easy to write the following:
#include<stdio.h> int main() { //Code 1 int num = 10; if (num = 5) { printf("hehe\n"); } return 0; }
The grammar is correct, but the meaning is different.
Code analysis: if (num = 5) assigns 5 to num, so the expression is always true. Print hehe.
It's not easy to find out what's wrong with this way of writing.
But if it is written as follows:
#include<stdio.h> int main() { //Code 2 int num = 10; if (5 = num) { printf("hehe\n"); } return 0; }
In code 2, if a = is omitted, the compiler will report an error, and it is easy to know where the error is.
Write if (expression). If the expression is to judge whether the constant and variable values are equal, it is recommended to write it in the form of if (constant = = variable). Example: if(5==num)
6. Understand the if... Else statement, do a question and feel the branch statement
Judge whether a number is odd or even.
#include<stdio.h> int main() { int num = 0; scanf("%d", &num); if (num % 2 == 1) { printf("%d It's an odd number\n", num); } else { printf("%d It's an even number\n", num); } return 0; }
Code analysis:
%Modulo operator. Is the remainder of the division of two integers.
Divide an integer by 2, the remainder of 0 is even, and the remainder of 1 is odd.
Operation results:
2, switch statement
The switch statement is also a branch statement. It is often used in the case of multiple branches.
Input 1, output Monday
Input 2, output Tuesday
Input 3, output Wednesday
Input 4, output Thursday
Input 5, output Friday
Input 6, output Saturday
Input 7, output Sunday
The form written as if... Else if... Else if is too complex, it must have a different grammatical form.
- switch syntax structure:
switch (integer expression)
{
Statement item;
}
- Statement item:
case integer constant expression:
sentence;
Note: there should be a space between case and integer constant expression.
case must be followed by an integer constant expression, which can be an integer or a character.
- break in switch statement
In the switch statement, we can't directly implement the branch. Only when we use it with break can we realize the real branch.
Execute case statement in switch:
- Switch (expression). When the expression is, the switch is when to start execution.
- If there is no break after the case statement, the program will continue to execute until a break is encountered or the case statement is executed before jumping out of the switch statement.
case followed by integer:
#include <stdio.h> int main() { int day; scanf("%d", &day); switch (day) { case 1: printf("Monday\n"); break; case 2: printf("Tuesday\n"); break; case 3: printf("Wednesday\n"); break; case 4: printf("Thursday\n"); break; case 5: printf("Friday\n"); break; case 6: printf("Saturday\n"); break; case 7: printf("Sunday\n"); break; } return 0; }
Operation results:
Sometimes our needs change:
- Input 1-5 and output "weekday"
- Input 6-7 and output "weekend"
#include <stdio.h> int main() { int day; scanf("%d", &day); switch (day) { case 1: case 2: case 3: case 4: case 5: printf("weekday\n"); break; case 6: case 7: printf("weekend\n"); break; } return 0; }
Operation results:
- default clause in switch statement
- If the expressed value does not match the value of the integer constant expression after all case s, all statements will be skipped. The program will not terminate or report an error, because this situation is not considered an error in C.
- If you do not want to ignore the values of expressions that do not match all labels, you can add a default clause to the switch statement list. When the values of switch expressions do not match the values of all case labels, the statements after this default clause will be executed. Only one default clause can appear in each switch statement. However, it can appear anywhere in the statement list.
- Although the default clause can be written anywhere in the switch statement, it is generally written at the end of all case statements.
#include <stdio.h> int main() { int day; scanf("%d", &day); switch (day) { case 1: case 2: case 3: case 4: case 5: printf("weekday\n"); break; case 6: case 7: printf("weekend\n"); break; default : printf("Input error, please enter 1~7 Number of\n"); } return 0; }
Operation results:
Write a simple calculator
case followed by character:
#include<stdio.h> int main() { int num1=0, num2=0,sum=0; char ch; printf("Please enter two integers and operators to evaluate(+,-,*,/),Do not add spaces when entering\n"); scanf("%d%c%d", &num1, &ch, &num2); switch (ch) { case '+':sum = num1 + num2; printf("%d+%d=%d\n", num1, num2, sum); break; case '-':sum = num1 - num2; printf("%d-%d=%d\n", num1, num2, sum); break; case '*':sum = num1 * num2; printf("%d*%d=%d\n", num1, num2, sum); break; case '/':sum = num1 / num2; printf("%d/%d=%d\n", num1, num2, sum); break; default:printf("Input error, please re-enter as required\n"); break; } return 0; }
Operation results:
summary
Recognition of C language (I) mainly expounds the selection structure (branch statement) in C language in detail.
This series will continue to learn C language and further elaborate the knowledge of C language.