- home page
- Special column
- full stack dev stills
- Article Details
React Native Pit Filling Tour--Using react-redux hooks
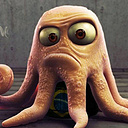
React hooks come out and open a door to front-end wheels. Tools from various Hooks fly all over the sky. react-redux also follows this trend.
The project code is in Here
First and foremost. If you don't know if you want to use redux, it's best not to.
The main problem redux solves is state management from a unified source.
- The global state is stored in the store. store.getState() gets the state tree.
- To the store, sending an action generates a new state through reducer. store.dispatch({type:SOME_ACTION, data: {}).
- Subscribe to a store, or a new state. store.subscribe() => store.getState()).
React-redux mainly solves Article 2. Use the connect method to inject both States and actions into the component as props.
For example, there is now a Counter component. increment acts as an action creator. The state is value++. Then it can be written as:
function Counter(props) { // ... return (<Button onClick={() => props.increment()}>+</Button>) } const mapStateToProps = (state) => ({ value: state.value, }); const mapActionToProps = (dispatch) => ({ increment: dispatch(increment()), }) connect(mapStateToProps, mapActionToProps)(Counter);
Probably that's what it is like above. With react-redux hooks, the picture turns around. The whole is much clearer. Of course, there's no shortage of help from the redux toolkit.
To achieve a complete application of redux, you need to create store s for Redux and other previous configurations. To reduce these tedious configurations, Redux has developed a toolkit set of tools. These tools are available in the project code and can be used as a reference. They are not described here.
It looks like this after using react-redux. We didn't do much introducing action creator and reducer earlier. Suddenly it doesn't show the benefits of simplification. One thing is, actions and reducers are usually placed in different places. They aren't very convenient to use.
In the new implementation, these are all in the slice file for unified management. They are also much better to maintain.
import { createSlice } from '@reduxjs/toolkit'; export const counterSlice = createSlice({ name: 'counter', initialState: { value: 0, }, reducers: { increment: state => { state.value += 1; }, decrement: state => { state.value -= 1; }, incrementByAmount: (state, action) => { state.value += action.payload; }, }, }); // Action creators are generated for each case reducer function export const { increment, decrement, incrementByAmount } = counterSlice.actions;
This is a benefit of using the redux toolkit. The action part is rarely handled. It will automatically generate an action creator.
The connect method for react-redux is also omitted. The alternatives are the useSelector and useDispatch methods. In the ReduxCounter component:
// ... const ReduxCounter: React.FC<ReduxCounterProps> = props => { // ... const count = useSelector((state: RootState) => { // 1 return state.counter.value; }); const dispatch = useDispatch(); // 2 const onAdd = () => dispatch(increment()); //3 return ( <View> <Text>{count}</Text> </View> <View style={styles.buttonWrapper}> <TouchableHighlight style={styles.button} onPress={onAdd}> <View style={styles.buttonInsider}> <Text>+</Text> </View> </TouchableHighlight> ); }; export { ReduxCounter };
// 1. Use useSelector to get the state of the state tree corresponding to this component
// 2. Use useDispatch to get dispatch method to send action
// 3. Call the dispatch method to send an increment() action creator generated action while processing the add click.
finish
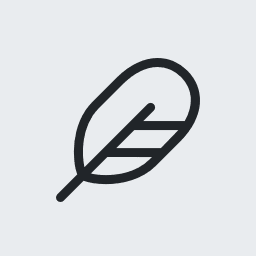
full stack dev stills
Time will not wait for me
0 Comments
Time will not wait for me