catalogue
1, Operator
1. Arithmetic operator
Mathematical operators are also called arithmetic operators, including: addition+ Subtract multiply * divide / remainder% (also called modulo)
The arithmetic operator is relatively simple, which is + - * learned in primary school/ . It should be noted that% modulo is often used to find out whether a number can be divided
Priority summary of operators: multiply and divide before addition and subtraction. If there are parentheses, calculate the inside of the parentheses first.
Find the area of a circle
// Prepare a variable to receive user input radius let r = prompt('Please enter the radius of the circle:') // Prepare a variable to receive the area calculation result of the circle. Pay attention to the operation on the right side of the equal sign, and then assign it to the right side of the equal sign let s = 3.14 * r * r // Template string used for page printing document.write(`The area of the circle is: ${s}`)
2. Assignment operator
The '=' sign is the assignment operator. Note that the '=' equal sign in JavaScript is different from the equal sign in mathematics
In js' = 'is to assign the value on the right of the equal sign to the variable on the left of the equal sign. In js' = =' is the same as' = 'in mathematics
Other assignment operators are:
- +=
- -=
- *=
- /=
- %=
//How to add one to a variable before? <script> let num = 1 num = num + 1 console.log(num); // 2 </script> //You can now assign values directly <script> let num = 1 num += 1 console.log(num); // 2 </script>
3. Unary operator
There are many kinds of operators in js, including unary operator, binary operator and ternary operator.
Every time we add one, we have to write it again. It's troublesome. Is there any simple way?
Autoincrement: Symbol:++ Let the variable add 1
Self subtraction: Symbol:-- Let the variable subtract 1
Usage scenario: it is often used to count multiple operations
Usage:
Pre auto increment:
<script> let num = 1 ++num </script>
Adding 1 to num is equivalent to num += 1
Post auto increment:
<script> let num = 1 num++ </script>
Adding 1 to num is equivalent to num += 1
There is no difference between pre auto increment and post auto increment The difference is that the rules involved in arithmetic operations are different
Pre auto increment: automatically increment 1 first, and then participate in the operation (+ + add before)
<script> let num = 1 console.log(++i + 2); //The result is 4 // Note: at this time, i is 2 plus 2 </script>
Post auto increment: first participate in the operation, and then auto increment 1 (+ + add after)
<script> let num = 1 console.log(i++ + 2); //The result is 3 // Note that i + + first line operation is self adding 1 console.log(i) //And i is 2 </script>
Self subtraction homology
Generally, it is used alone in development, and it is rarely calculated together, while the post self addition is more
Interview questions:
let i = 1 console.log(i++ + ++i + i) // i + + is 1 and participates in the operation 1 + //At this time, i increases by 1 to 2 + + i, and + + i increases by 1 to 2 + 1 = 3, which is 1 + 2 + 1 //Finally, i increases by 1 to 3, which is 1 + (2 + 1) + 3 //The output is 7
4. Comparison operator
Comparison operator:
- >: is the left greater than the right
- <: is the left smaller than the right
- >=: is the left greater than or equal to the right
- < =: is the left less than or equal to the right
- ==: are the left and right sides equal
- ===: whether the left and right types and values are equal
- !==: Are the left and right sides not
The result of the comparison operator is Boolean, and only two false and true will be obtained
Details:
- String comparison is the ASCII code corresponding to the character being compared
- NaN is not equal to any value, including itself
- The comparison between different types will be implicitly converted to Boolean and then compared
- ===And== Use more and = = use less
5. Logical operators
Symbol | name | Everyday reading | characteristic | Pithy formula |
&& | Logic and | also | Both sides of the symbol are true, and the result is true | One false is false |
|| | Logical or | perhaps | Both sides of the symbol are false, and the result is false | One truth is one truth |
! | Logical non | Reverse | True becomes false, false becomes true | True becomes false, false becomes true |
Short circuit between logic and & & and logic or 𞓜
&&: when the left side of the symbol is false, the result is false, regardless of the expression on the right
||: when the left side of the symbol is true, the result is true, regardless of the expression on the right
Reason: the result of the whole formula can be judged through the left, so there is no need to care about the right
6. Operator priority
Logical non precedence in unary operators is high !
Logical and have higher priority than logical or
2, Statement
1. Expressions and statements
Difference: expression is the result of calculation; A statement is to do something
For example, the expression: 1 + 2
Statement: alert()
2. Branch statement
Three flow control statements of program control:
- Sequential statement (the execution order of code from top to bottom is not much discussed)
- Branch statement
- Circular statement
Branching statements allow us to execute code selectively
1.if branch statement
if branch statements are divided into single branch, double branch and multi branch
Single branch syntax:
if (condition) { Execute code }
The code in the braces is executed only when it is true in the braces
When the parentheses are not Boolean, they are not implicitly converted to Boolean
Case:
Requirements: when the score is greater than or equal to 700, you will be admitted
// Declare a variable to receive data entered by the user let num = +prompt('Please enter your college entrance examination results:') // If judgment statement if the number of inputs is greater than or equal to 700, the code block is executed if (num >= 700) { document.write('Congratulations on your admission!') }
Double branch statement
if (condition) { Code executed when conditions are met } else { Code executed without conditions }
Case: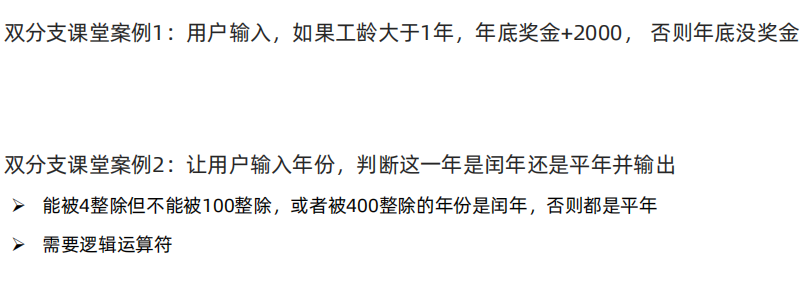
//Case 1 <script> //Declare variables to receive data let age = prompt('Please enter your length of service:') let money = 15000 // Judgment conditions if (age >= 1) { document.write(`What's your salary ${money + 2000}`) } else if (age < 0) { document.write('Please enter a correct number') } else { document.write(`What's your salary ${money}`) } </script>
Case 2 // Declare a variable to receive the data entered by the user. Note that it should be implicitly converted to numeric let year = +prompt('Please enter a year') // Judge whether the condition of leap year is met. The result is true and the output is leap year. The result is a false output year if (year % 4 === 0 & year % 100 !== 0 || year % 400 === 0) { document.write(`You entered ${year}It's a leap year`) } else { document.write(`You entered ${year}It's a normal year`) }
Multi branch statement
interpretation:
- Judge condition 1 first. If condition 1 is met, code 1 will be executed, and others will not be executed
- If not, judge condition 2 downward. If condition 2 is met, code 2 will be executed, and others will not be executed
- If still not satisfied, continue to judge, and so on
- If the above conditions are not met, execute the code n in else
- Note: N conditions can be written, but the demonstration here only writes
2. Ternary operator
Similar to if double branch statement, can I dictate that the condition is true? If it is true, take the colon before it and if it is false, take the colon after it
Case:
Judge the size of two numbers
// Declare two edge quantities to receive the data entered by the user. At this time, pay attention to the implicit conversion of digital type let num1 = +prompt('Please enter the first number') let num2 = +prompt('Please enter the second number') // Declare a variable to receive the result of the comparison let res = num1 > num2 ? num1 : num2 document.write(`You entered ${res} Relatively large`)
Digital zero filling
// Prepare Popup let num = prompt('Please enter a number') // Ternary expression let result = num < 10 ? '0' + num : num document.write(`The number after zero is ${result}`) // Judge whether the number is less than 0. If it is less than 0, fill in zero // if statement if (num < 10) { document.write(`${'0' + num}`) } else { document.write(num) }
3.switch statement
interpretation:
- Find the case value that is congruent with the data in the parentheses and execute the corresponding code
- If there is no congruent = = =, execute the code in default
- Example: if the data is congruent with the value 2, the generation is executed
matters needing attention:
It is generally used for equivalence judgment. Unsuitable interval judgment
It also needs to be used with the break keyword. Failure to break will cause case penetration
Case: simple calculator
// Declare three variables to receive the data entered by the user. Be careful to implicitly convert to type number let num1 = +prompt('Please enter the first number') let num2 = +prompt('Please enter the second number') let operator = prompt('Please enter+ - * / Any operator in') switch (operator) { case '+': document.write(`You entered an addition operation and the result is ${num1 + num2}`) break case '-': document.write(`You entered a subtraction operation and the result is ${num1 - num2}`) break case '*': document.write(`You entered a multiplication operation and the result is ${num1 * num2}`) break case '/': document.write(`You entered a division operation and the result is ${num1 / num2}`) break default: alert('Please enter the correct number')
3, Circulation
1.
2.while loop
1. Syntax:
while (Cycle condition) { Code to repeat(Circulatory body) }
interpretation:
Similar to the if statement, the code will be executed only when the condition in parentheses is true
After the code in the while braces is executed, it will not jump out, but continue to return to the braces to judge whether the conditions are met. If so, execute the code in the braces, and then return to the
Judge the conditions in parentheses until the conditions in parentheses are not met, that is, jump out
2. Precautions:
The essence of a cycle is to take a variable as the starting value, then continuously produce changes and slowly approach the termination condition.
The cycle needs to have three elements:
1. Variable starting value
2. Termination condition (if there is no termination condition, the loop will be executed all the time, resulting in an endless loop)
3. Variable variation (self increasing or self decreasing)
Case:
Case 1 // Declare variable start value let i = 1 let sum = 0 // Cycle condition while (i <= 100) { document.write(i + '<br>') // Variable update sum += i i++ } Case 2 // Declare variable start value let i = 1 let sum = 0 // Cycle condition while (i <= 100) { // Variable update sum += i i++ } document.write(sum) Case 3 // Declare variable start value let i = 1 let sum = 0 while (i < 100) { // The condition for judging whether it is an even number is that it can divide by two if (i % 2 === 0) { sum += i } i++ } console.log(sum)