As more and more scripts and installation tools are written, desktops and folders are becoming more and more messy.
Just a few days ago, I deleted one of my projects because of an accident, so I decided to tidy up the computer.
But I forgot the location of many of my scripts and tools, so I decided to write a small tool to quickly start some of the commonly used scripts or tools.
Python is chosen here because Python is cross-platform, so my Linux computer can also be used, and simple.
Note: Use of tutorials python3 and PyQt5 To write
If you use Python 2.7, you can use this tutorial for reference. PyQt4 To write
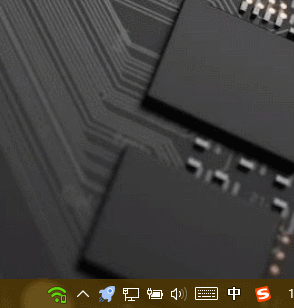
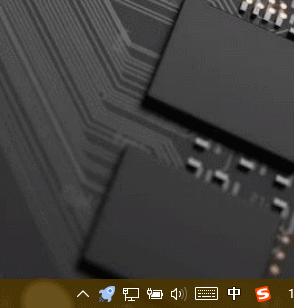
1. preparation
First
Install PyQt5
pip3 install PyQt5
Then create the directory
Create a new folder to store items
The folder example structure is as follows:
/main #Home Folder Name Customization ├─main.pyw #Main startup program ├─conf.py #Used for custom menu configuration ├─/icons #Used to store icon folders └─/scripts #Used to store script folders can be omitted
The file with.pyw suffix refers to the pythonw.exe file in the Python environment variable directory by default. If it is opened by console program, you need to specify the opening mode manually.
About icons you can go to http://www.iconfont.cn/ download
2. code
2.1. Let's create a notification bar to display:
############## ## main.pyw ## ############## from PyQt5.QtWidgets import QDialog, QSystemTrayIcon, QMenu ,QAction,QApplication from PyQt5.QtGui import QIcon import sys class main(QDialog): def __init__(self): super().__init__() self.loadMenu() self.initUI() def loadMenu(self): menuItems = [] # Menu list menuItems.append({"text": "start-up", "icon": "./icons/set.png", "event": self.show, "hot": "D"}) menuItems.append({"text": "Sign out", "icon": "./icons/close.png", "event": self.close, "hot": "Q"}) self.trayIconMenu = QMenu(self)# create menu #Traverse the text, icons, hotkeys, and click events displayed by the bindings #Hotkeys may not work. I just want to show the effect here. for i in menuItems: tmp = QAction(QIcon(i["icon"]), i["text"],self, triggered=i["event"]) tmp.setShortcut(self.tr(i["hot"])) self.trayIconMenu.addAction(tmp) def initUI(self): self.trayIcon = QSystemTrayIcon(self) # <=== Create notification bar tray icon self.trayIcon.setIcon(QIcon("./icons/menu2.png"))#<=== Set tray icon self.trayIcon.setContextMenu(self.trayIconMenu)#<==== Create a right-click connection menu self.trayIcon.show()#<====== Display tray self.setWindowIcon(QIcon("./icons/menu2.png")) #<=== Set the form icon self.setGeometry(300, 300, 180, 300) # <=== Set the opening position and width of the form self.setWindowTitle('Text') # self.show()#<===== Display Form # self.hide()#<===== Hide Form # Forms are not displayed by default # Rewrite the form closure event to hide when it clicks close def closeEvent(self, event): if self.trayIcon.isVisible(): self.trayIcon.hide() if __name__ == '__main__': app = QApplication(sys.argv) ex = main() sys.exit(app.exec_())
Effect:
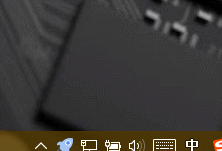
2.2. If the notification bar displays successfully, the next configuration is very simple, just bind the display and events.
############## ## conf.pyw ## ############## import os import time def PrScrn():#Call the dll example time.sleep(0.5) os.popen('rundll32 .\\script\\screenshot\\PrScrn.dll PrScrn') def Open360Wifi():#Open an application example os.popen('"C:\\Program Files (x86)\\360\\360AP\\360AP.exe" /menufree') def OpenRegedit():#Call command example os.popen('regedit') def Ifconfig(): os.system('''ipconfig & pause''') menuItems=[ #... Examples.... {"text":"screenshot","icon":"./icons/cut.png","event":PrScrn,"hot":"Alt+P"}, {"text":"360Wifi","icon":"./icons/wifi.png","event":Open360Wifi,"hot":"Alt+W"}, {"text":"registry","icon":"./icons/regedit.png","event":OpenRegedit,"hot":"Alt+R"}, {"text":"ifconfig","icon":"./icons/ip.png","event":Ifconfig,"hot":"Alt+R"} ]
2.3. Read configuration and display
Reading is very simple:
from PyQt5.QtWidgets import QDialog, QSystemTrayIcon, QMenu ,QAction,QApplication from PyQt5.QtGui import QIcon import sys import conf#<===== Import conf class main(QDialog): def __init__(self): super().__init__() self.loadMenu() self.initUI() def loadMenu(self): menuItems =conf.menuItems #<========= menu list #... slightly. #... slightly. if __name__ == '__main__': app = QApplication(sys.argv) ex = main() sys.exit(app.exec_())
2.4. Use Forms
Let me give you a simple example:
from PyQt5.QtWidgets import QDialog, QAction, QApplication, QListWidget, QVBoxLayout, QSystemTrayIcon, QMenu, QListWidgetItem from PyQt5.QtGui import QIcon import sys import conf class main(QDialog): def __init__(self): super().__init__() self.loadMenu() self.initUI() #Omit... def loadList(self): lv = QListWidget() for i in range(len(conf.menuItems)): itm = conf.menuItems[i] if not 'icon' in itm.keys(): itm["icon"] = None if not 'event' in itm.keys(): itm["event"] = self.show if not 'hot' in itm.keys(): itm["hot"] = 'None' qlv = QListWidgetItem(QIcon(itm["icon"]), self.tr(itm["text"]+" ("+itm["hot"]+")")) qlv.event = itm["event"] # qlv.clicked.connect(self.close) lv.insertItem(i + 1, qlv) lv.itemDoubleClicked.connect(self.dbclickItem) self.layout.addWidget(lv) def dbclickItem(self, item): item.event() #Omit... #Omit...
Effect:
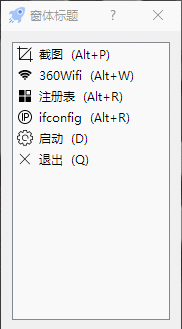
Well, that's all for the tutorial. If you want to boot up and boot yourself, you can put main.pyw create shortcuts into the boot folder.
For example, Windows 10 is usually located in C: ProgramData Microsoft Windows Start Menu Programs StartUp
The complete code is in the link: https://pan.baidu.com/s/1mjBbpnM Password: x6dr
For screenshots, you can refer to: Implementing Shortcut Tool under windows