python connects to Mysql database:
Python Programming can be done using MySQLdb data base Connections and operations such as query/insert/update, but each connection MySQL When database requests are accessed independently, it is a waste of resources, and when the number of accesses reaches a certain number, it will have a great impact on the performance of mysql. Therefore, in actual use, database connection pool technology is usually used to access the database to achieve the purpose of resource reuse.
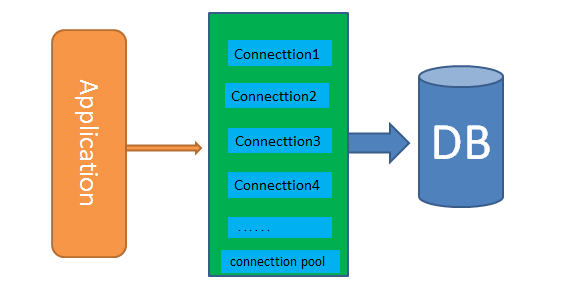
python's database connection pool package DBUtils:
DBUtils is a set of Python database connection pool packages that allow thread-safe wrapping of non-thread-safe database interfaces. DBUtils comes from Webware for Python.
DBUtils provides two external interfaces:
- * PersistentDB: Provides thread-specific database connections and automatically manages connections.
- * PooledDB: Provides shared database connections between threads and automatically manages connections.
Download address: DBUtils After downloading and decompressing, install using the python setup.py install command
Next, use MySQLdb and DBUtils to build your own mysql database connection pool Toolkit
Under the project directory, the new package is named dbConnecttion, and the new module is named MySqlConn. Below is MySqlConn.py. This module creates Mysql's connection pool object and creates common operation methods such as query/insert. This part of the code is implemented as follows:
-
-
-
-
-
-
-
-
-
-
-
import MySQLdb
-
from MySQLdb.cursors import DictCursor
-
from DBUtils.PooledDB import PooledDB
-
-
import Config
-
-
-
-
-
-
class Mysql(object):
-
-
-
-
-
-
__pool = None
-
def __init__(self):
-
-
self._conn = Mysql.__getConn()
-
self._cursor = self._conn.cursor()
-
-
@staticmethod
-
def __getConn():
-
-
-
-
-
if Mysql.__pool is None:
-
__pool = PooledDB(creator=MySQLdb, mincached=1 , maxcached=20 ,
-
host=Config.DBHOST , port=Config.DBPORT , user=Config.DBUSER , passwd=Config.DBPWD ,
-
db=Config.DBNAME,use_unicode=False,charset=Config.DBCHAR,cursorclass=DictCursor)
-
return __pool.connection()
-
-
def getAll(self,sql,param=None):
-
-
-
-
-
-
-
if param is None:
-
count = self._cursor.execute(sql)
-
else:
-
count = self._cursor.execute(sql,param)
-
if count>0:
-
result = self._cursor.fetchall()
-
else:
-
result = False
-
return result
-
-
def getOne(self,sql,param=None):
-
-
-
-
-
-
-
if param is None:
-
count = self._cursor.execute(sql)
-
else:
-
count = self._cursor.execute(sql,param)
-
if count>0:
-
result = self._cursor.fetchone()
-
else:
-
result = False
-
return result
-
-
def getMany(self,sql,num,param=None):
-
-
-
-
-
-
-
-
if param is None:
-
count = self._cursor.execute(sql)
-
else:
-
count = self._cursor.execute(sql,param)
-
if count>0:
-
result = self._cursor.fetchmany(num)
-
else:
-
result = False
-
return result
-
-
def insertOne(self,sql,value):
-
-
-
-
-
-
-
self._cursor.execute(sql,value)
-
return self.__getInsertId()
-
-
def insertMany(self,sql,values):
-
-
-
-
-
-
-
count = self._cursor.executemany(sql,values)
-
return count
-
-
def __getInsertId(self):
-
-
-
-
self._cursor.execute("SELECT @@IDENTITY AS id")
-
result = self._cursor.fetchall()
-
return result[0]['id']
-
-
def __query(self,sql,param=None):
-
if param is None:
-
count = self._cursor.execute(sql)
-
else:
-
count = self._cursor.execute(sql,param)
-
return count
-
-
def update(self,sql,param=None):
-
-
-
-
-
-
-
return self.__query(sql,param)
-
-
def delete(self,sql,param=None):
-
-
-
-
-
-
-
return self.__query(sql,param)
-
-
def begin(self):
-
-
-
-
self._conn.autocommit(0)
-
-
def end(self,option='commit'):
-
-
-
-
if option=='commit':
-
self._conn.commit()
-
else:
-
self._conn.rollback()
-
-
def dispose(self,isEnd=1):
-
-
-
-
if isEnd==1:
-
self.end('commit')
-
else:
-
self.end('rollback');
-
self._cursor.close()
-
self._conn.close()
Configuration file module Cnofig, including database connection information/username password, etc.
-
-
''
-
-
-
-
-
DBHOST = "localhost"
-
DBPORT = 33606
-
DBUSER = "zbc"
-
DBPWD = "123456"
-
DBNAME = "test"
-
DBCHAR = "utf8"
Create a test module to test mysql access using connection pools:
-
-
''
-
-
-
-
from MySqlConn import Mysql
-
from _sqlite3 import Row
-
-
-
mysql = Mysql()
-
-
sqlAll = "SELECT tb.uid as uid, group_concat(tb.goodsname) as goodsname FROM ( SELECT goods.uid AS uid, IF ( ISNULL(goodsrelation.goodsname), goods.goodsID, goodsrelation.goodsname ) AS goodsname FROM goods LEFT JOIN goodsrelation ON goods.goodsID = goodsrelation.goodsId ) tb GROUP BY tb.uid"
-
result = mysql.getAll(sqlAll)
-
if result :
-
print "get all"
-
for row in result :
-
print "%s\t%s"%(row["uid"],row["goodsname"])
-
sqlAll = "SELECT tb.uid as uid, group_concat(tb.goodsname) as goodsname FROM ( SELECT goods.uid AS uid, IF ( ISNULL(goodsrelation.goodsname), goods.goodsID, goodsrelation.goodsname ) AS goodsname FROM goods LEFT JOIN goodsrelation ON goods.goodsID = goodsrelation.goodsId ) tb GROUP BY tb.uid"
-
result = mysql.getMany(sqlAll,2)
-
if result :
-
print "get many"
-
for row in result :
-
print "%s\t%s"%(row["uid"],row["goodsname"])
-
-
-
result = mysql.getOne(sqlAll)
-
print "get one"
-
print "%s\t%s"%(result["uid"],result["goodsname"])
-
-
-
mysql.dispose()
Of course, there are many other parameters to configure:
- dbapi: database interface
- mincached: Number of empty connections opened at startup
- maxcached: Maximum number of connections available in connection pools
- maxshared: Maximum number of shared connections in connection pool
- maxconnections: maximum number of connections allowed
- blocking: blocking when the maximum number is reached
- maxusage: Maximum number of multiplexing times for a single connection
According to their own needs, the above resource parameters should be rationally allocated to meet their actual needs.
So far, the mysql connection pool in python has been implemented, and it will be better to use it directly next time.
Blog address:
data
mining club