1. Words written in the front
I still want to learn Python well. I have learned the basic grammar part for a long time. With a little penetration of the application part, I can go to data analysis, crawler, web, machine learning and other aspects, and have a long way to go.

OK, no more nonsense, start programming.



2. Build project structure
For a good project, the structure and context must be clear, so that the team cooperation can be better coordinated. Although my structure is not very good every time, I try to develop in this direction. As shown below:
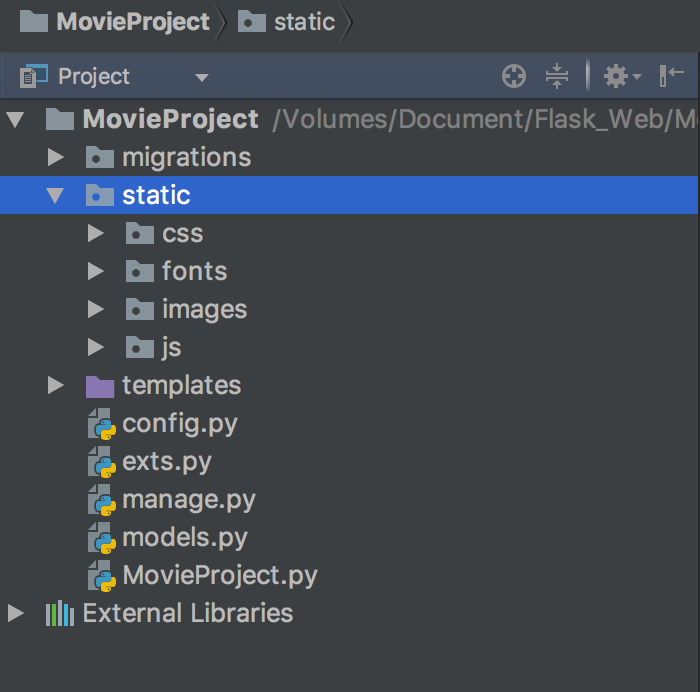
3. Write configuration file and command line operation file
The packages needed are: Flask script, flask Sqlalchemy, flask migrate, etc. now write the configuration files such as database, and then write the manage command line operation file, as follows:
import os DEBUG = True SECRET_KEY = os.urandom(24) DIALECT = 'mysql' DRIVER = 'pymysql' HOST = '127.0.0.1' PORT = '3306' USERNAME = 'root' PASSWORD = 'root' DATABASE = 'movie_demo3' SQLALCHEMY_DATABASE_URI = '{}+{}://{}:{}@{}:{}/{}'.format(DIALECT, DRIVER, USERNAME, PASSWORD, HOST, PORT, DATABASE) SQLALCHEMY_TRACK_MODIFICATIONS = False
from flask_script import Manager from flask_migrate import Migrate, MigrateCommand from MovieProject import app from exts import db manager = Manager(app) # Using Migrate to bind app and db migrate = Migrate(app, db) # Add the command of migration script to manager manager.add_command('db', MigrateCommand) if __name__ == '__main__': manager.run()
4. Write database model
Here mainly write the movie data you want to store, and store the prepared data in the form of class, as follows:
from exts import db class Recommend(db.Model): __tablename__ = 'recommend' id = db.Column(db.Integer, primary_key=True, autoincrement=True) title = db.Column(db.String(100), nullable=False) image = db.Column(db.Text, nullable=False) url = db.Column(db.Text, nullable=False) class Action(db.Model): __tablename__ = 'action' id = db.Column(db.Integer, primary_key=True, autoincrement=True) title = db.Column(db.String(100), nullable=False) image = db.Column(db.Text, nullable=False) url = db.Column(db.Text, nullable=False) class Fiction(db.Model): __tablename__ = 'fiction' id = db.Column(db.Integer, primary_key=True, autoincrement=True) title = db.Column(db.String(100), nullable=False) image = db.Column(db.Text, nullable=False) url = db.Column(db.Text, nullable=False) class War(db.Model): __tablename__ = 'war' id = db.Column(db.Integer, primary_key=True, autoincrement=True) title = db.Column(db.String(100), nullable=False) image = db.Column(db.Text, nullable=False) url = db.Column(db.Text, nullable=False) class Love(db.Model): __tablename__ = 'love' id = db.Column(db.Integer, primary_key=True, autoincrement=True) title = db.Column(db.String(100), nullable=False) image = db.Column(db.Text, nullable=False) url = db.Column(db.Text, nullable=False) class Funny(db.Model): __tablename__ = 'funny' id = db.Column(db.Integer, primary_key=True, autoincrement=True) title = db.Column(db.String(100), nullable=False) image = db.Column(db.Text, nullable=False) url = db.Column(db.Text, nullable=False)
5. Finally, write the main program according to the template you want to render
I am here This website Some rendering templates are found above, and the main program is as follows:
from flask import Flask, render_template, request, redirect, url_for import config from exts import db from models import Recommend, Action, Fiction, War, Love, Funny app = Flask(__name__) app.config.from_object(config) db.init_app(app) @app.route('/', methods=['GET','POST']) def index(): return render_template('index.html') @app.route('/detail/<name>', methods=['GET','POST']) def detail(name): page = request.args.get('page', 1, type=int) if name == 'recommend': pagination = Recommend.query.paginate(page, per_page=20, error_out=True) elif name == 'action': pagination = Action.query.paginate(page, per_page=20, error_out=True) elif name == 'fiction': pagination = Fiction.query.paginate(page, per_page=20, error_out=True) elif name == 'war': pagination = War.query.paginate(page, per_page=20, error_out=True) elif name == 'love': pagination = Love.query.paginate(page, per_page=20, error_out=True) elif name == 'funny': pagination = Funny.query.paginate(page, per_page=20, error_out=True) else: return '404' datas = pagination.items print(page) return render_template('detail.html', name=name, datas=datas, pagination=pagination) if __name__ == '__main__': app.run(debug=True)
6. The main interface is shown as follows
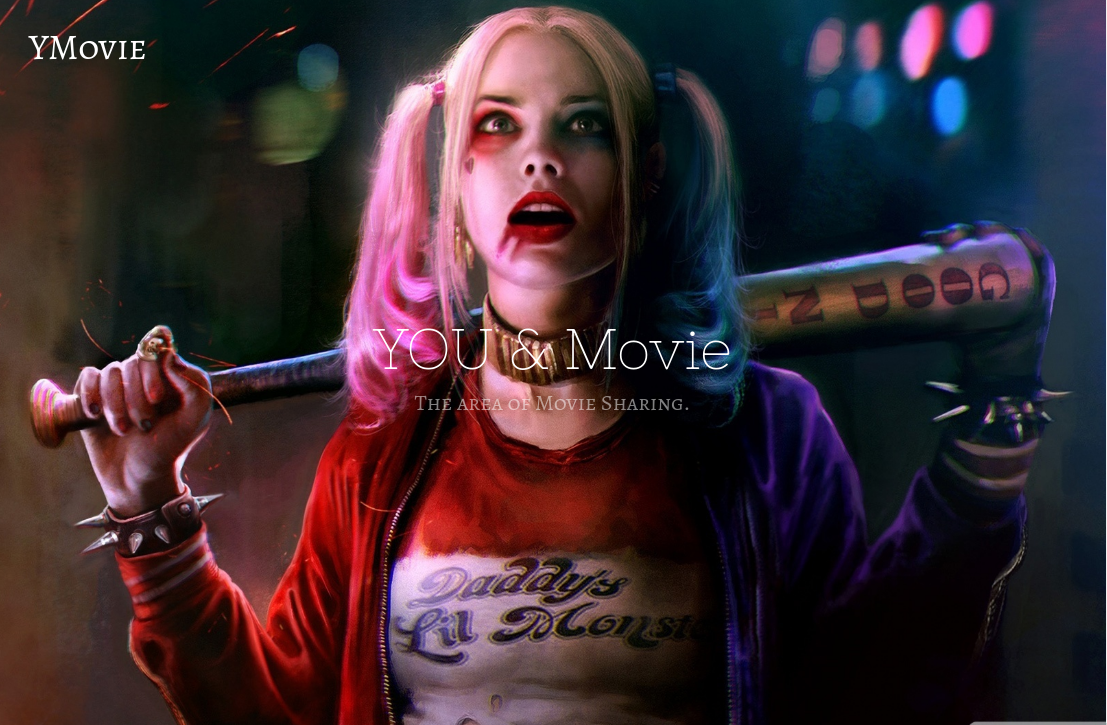
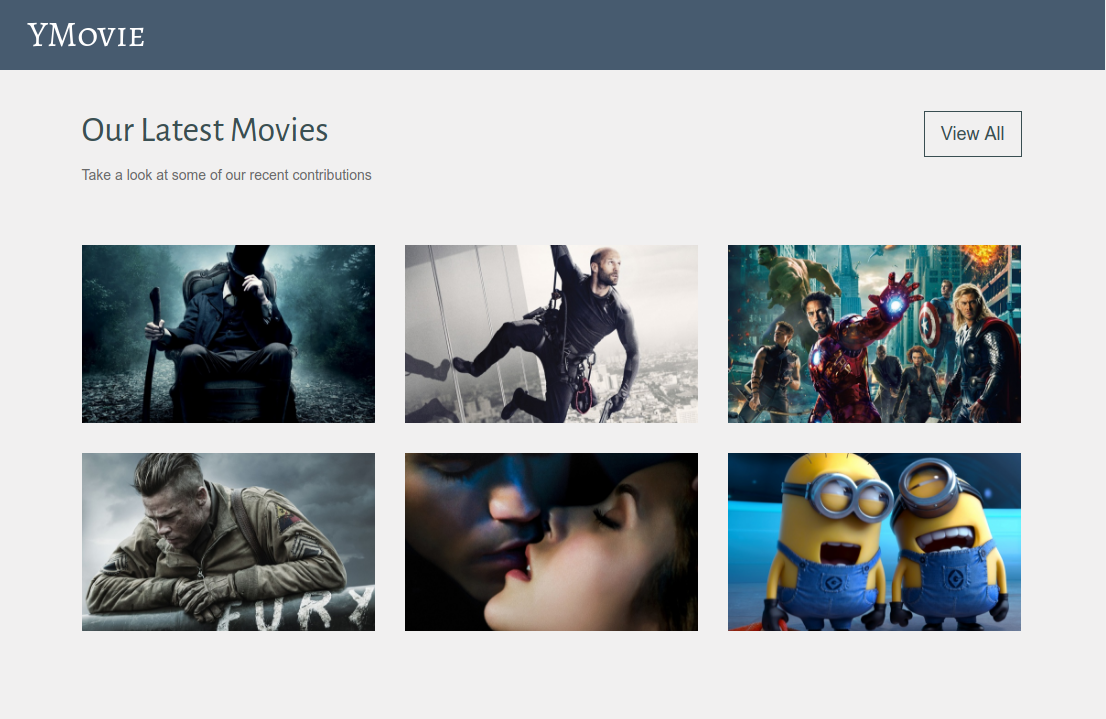
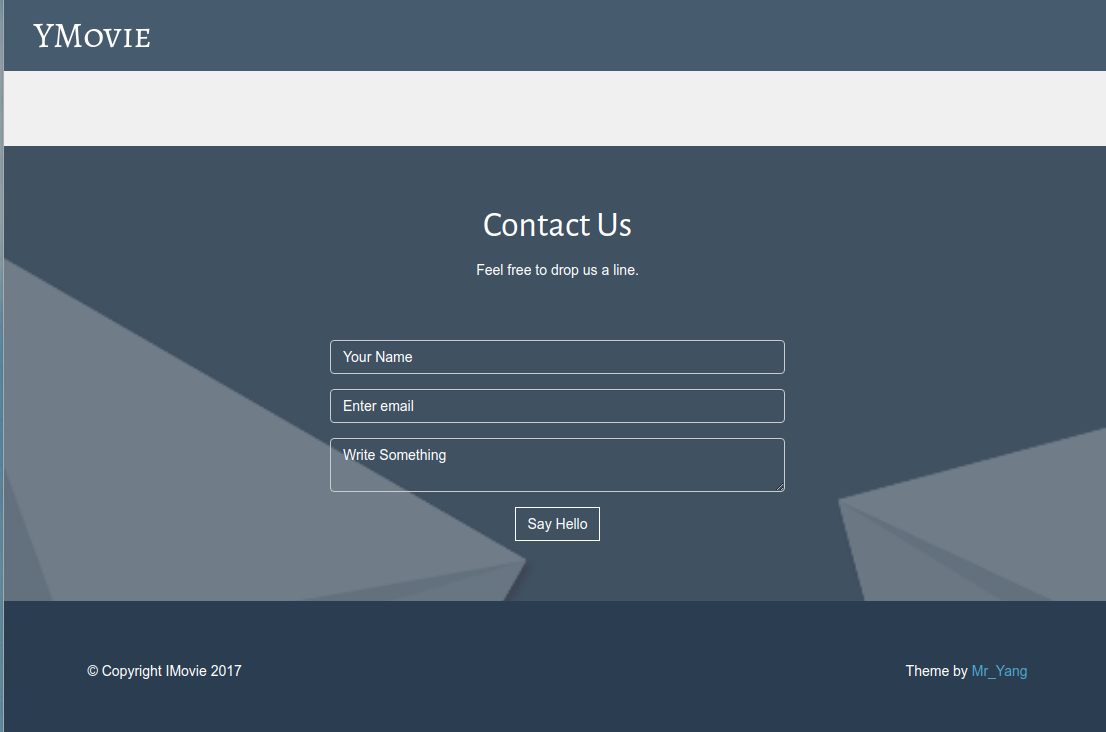
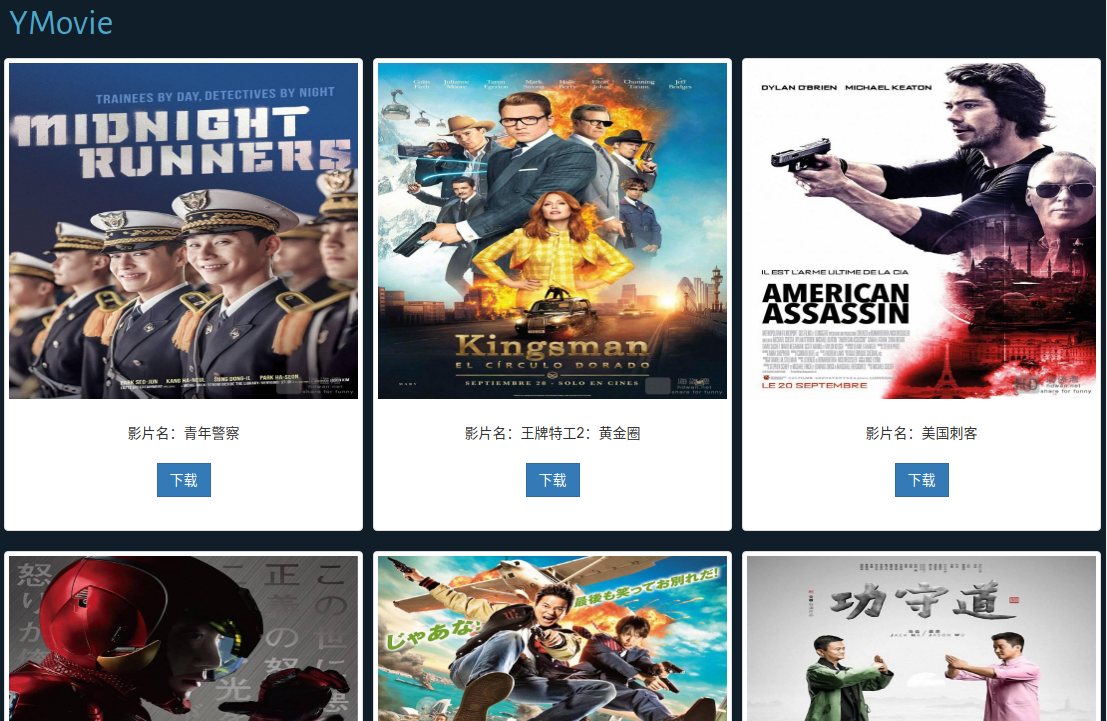
Please refer to Here For the first time, please spray lightly. It will be deployed to Alibaba cloud later.