- Introducing Prototype Patterns
- Examples of prototype patterns
- Why do we need to use prototype patterns
Introducing Prototype Patterns
If the reader is familiar with javascript, the word prototype should not be unfamiliar.
The prototype is used to generate instances, but it does not use classes to generate instances, but through instances to generate instances.
Why do we need to use classes to generate instances?
Associated with browsers, if we generate a button instance, this button instance through a series of operations, carrying a variety of information, such as button plus color, background image, plus text, plus events and so on. If we need an instance exactly the same as this button instance at this time, it is not enough just to come out through a new button class, because we have to do a series of operations on it, so the process of generating a exactly the same instance is very complex, so at this time we think that we can directly according to this instance, and then generate a What about the exact same example?
In fact, this is the basic idea of prototype pattern, which generates new instances according to instance prototype and instance pattern.
After introducing the basic ideas, we will take an example to understand the prototype example.
We implement a section that prints strings in boxes or displays them with underlines.
To implement the prototype pattern in Java, that is, the replication of instances, we can directly use the clone method, and need to implement the cloneable interface.
Examples of prototype patterns
First we define an interface, Product interface, which is the interface of replication function and inherits cloneable interface.
public interface Product extends Cloneable { public abstract void use(String s); public abstract Product createClone(); }
createClone is a method for replicating instances, and use is a method for using instance methods. These methods are all implemented by subclasses.
We implement a specific subclass, MessageBox, whose function is to create patterns that add box-like boundaries to characters.
This class implements the product interface, and createClone is used to replicate itself and generate a new identical instance, the idea of prototype pattern. The use method displays the results.
public class MessageBox implements Product { private char decochar; public MessageBox(char decochar) { this.decochar = decochar; } public void use(String s) { int length = s.getBytes().length; for (int i = 0; i < length + 4; i++) { System.out.print(decochar); } System.out.println(""); System.out.println(decochar + " " + s + " " + decochar); for (int i = 0; i < length + 4; i++) { System.out.print(decochar); } System.out.println(""); } public Product createClone() { Product p = null; try { p = (Product)clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return p; } }
Similarly, we implement the UnderlinePen class that can underline strings
This class also implements the product interface and can replicate itself to generate a new instance.
public class UnderlinePen implements Product { private char ulchar; public UnderlinePen(char ulchar) { this.ulchar = ulchar; } public void use(String s) { int length = s.getBytes().length; System.out.println("\"" + s + "\""); System.out.print(" "); for (int i = 0; i < length; i++) { System.out.print(ulchar); } System.out.println(""); } public Product createClone() { Product p = null; try { p = (Product)clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return p; } }
Finally, we create a new management class Manager to manage the creation, replication and use of these objects.
There is a map field in the Manager class to store product objects. The create method finds an instance of the object in map according to the name of the object, and then calls the createClone method of the instance to copy an instance.
public class Manager { private HashMap showcase = new HashMap(); public void register(String name, Product proto) { showcase.put(name, proto); } public Product create(String protoname) { Product p = (Product)showcase.get(protoname); return p.createClone(); } }
Let's test:
public class Main { public static void main(String[] args) { Manager manager = new Manager(); UnderlinePen upen = new UnderlinePen('~'); MessageBox mbox = new MessageBox('*'); MessageBox sbox = new MessageBox('/'); manager.register("strong message", upen); manager.register("warning box", mbox); manager.register("slash box", sbox); Product p1 = manager.create("strong message"); p1.use("Hello, world."); Product p2 = manager.create("warning box"); p2.use("Hello, world."); Product p3 = manager.create("slash box"); p3.use("Hello, world."); } }
Operation results:
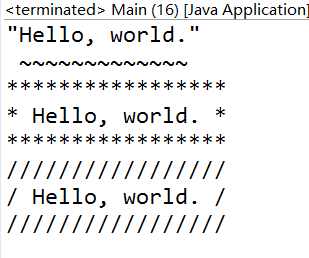
The above code is a simple class diagram:
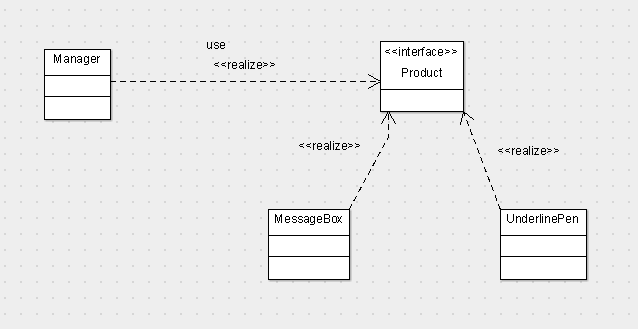
Summary
Let's summarize the Prototype prototype pattern
First, we give the class diagram of Prototype prototype pattern.
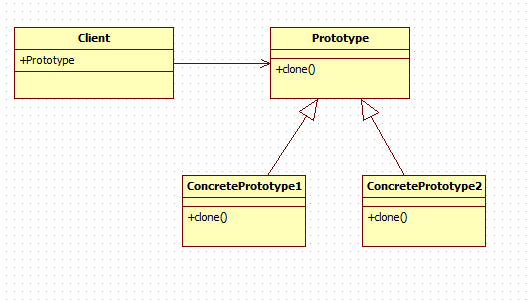
Usually there is a prototype interface or class that defines the clone method, just like the Product interface in this example, and then there are concrete prototype instances to implement or inherit from the interface, such as the UnderlinePen class and MessageBox in this example.
Here we have basically finished the prototype model.
Why do we need to use prototype patterns
But the reader may still have questions. We can't just use class new to give an instance. Why is it so complicated?
new Sth();
At the beginning of this article, we give examples of prototype patterns that need to be used. Now let's talk about them again with the example program.
-
There are so many types of objects that they cannot be integrated into one class.
For example, some examples use'*'or'/' as underscores.
This example is relatively simple, generating only three styles, but want to do, no matter how many styles can be implemented, but if you implement a class for each style, it will become very complex. The number of classes will be very large. -
It is difficult to generate instances from classes
This may not be felt in this case. You can imagine developing an application that users can use the mouse to operate, similar to the graphics editor. If we want to create an instance created by a series of mouse clicks with users, it will become very complicated obviously that we can not generate instances according to classes, but we can adopt the prototype mode to generate instances according to instances and copy them directly. Just give an example.