1. What are the built-in types in JS?
Seven. They are boolean, number, string, object, undefined, null and symbol.
2. Is NaN an independent type?
no NaN is of type number.
3. How to judge which type?
Object.prototype.toString.call(), returned as [object Type].
Now let's verify it.
Object.prototype.toString.call(NaN); // "[object Number]" Object.prototype.toString.call('1'); // "[object String]" Object.prototype.toString.call([1,2]); // "[object Array]"
Why use Object.prototype.toString.call() instead of typeof. Because it has some limitations. For example, when we judge the Array type, we print out the object instead of the Array we want.
typeof [1,2]; // "object" typeof new Number(1); // "object"
OK, since Object.prototype.toString.call() is so easy to use, we might as well encapsulate a method.
function getType(v) { return Object.prototype.toString.call(v).match(/\[object (.+?)\]/)[1].toLowerCase(); } getType(1); // "number"
4. How to perform type conversion between built-in types?
First, let's look at a few examples.
First example:
const a = 1; const b = '1'; if(a==b){ console.log('true') //true }
Second example:
const x = 1+'1'; console.log(x); // 11 const y = 1*'1'; console.log(y); // 1
The third example:
const array = []; if (array) { console.log('true'); // Return true } else { console.log('false'); }
The fourth example:
if([] == false) { console.log('true'); //Return true } else { console.log('false'); }
If you don't believe the operation of the fourth example, let's actually operate it. Sure enough, return 'true'.
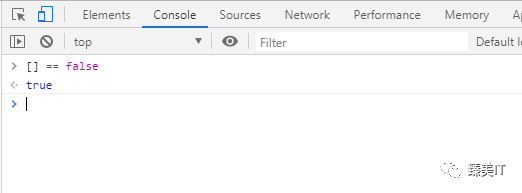
Let's not worry about why it returns true. We need to know these two points.
Four arithmetic transformations:
- When "adding" with a string is encountered, it will be converted into a string first, and then added.
- When "subtraction", "multiplication" and "division" are encountered, they will be converted into numbers before operation.
Contrast conversion (= =):
- Convert Boolean value and string to number;
- Object to string (most);
- null,undefined,symbol,NaN;
Let's take a look at the third point in comparative transformation.
- null == undefined, but not equal to other.

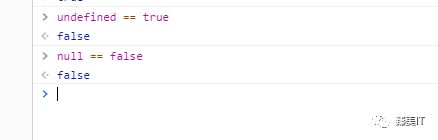
- symbol is not equal to other.
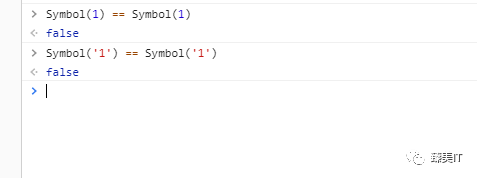
- NaN is not equal to others. The key is that it is not equal to itself.
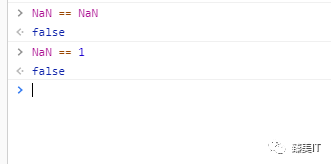
All right, let's get to the point. Why does the fourth example return true. Because [] is converted to a string first (as mentioned in the comparison conversion). Use the toString() method to convert, that is' ', then' 'to the number 0, and finally false to 0. 0 and 0 are of course equal.
Having said so much, let's talk about the data type conversion of objects in detail.
Object data type conversion
- valueOf
- toString
- Symbol.toPrimitive
Let's start with an example:
let obj = { valueOf(){ return 1 }, toString(){ return 'character' } }; console.log(obj+1);
Do you think it will be [object Object]1. I tell you, no! But 2. Because valueOf and toString are built-in methods of objects. We just customized it here. Let's first prove whether it is really a built-in method.

The valueOf method converts the original value, that is, itself. The toString method is converted to characters.
By the way, why 2? This is the flexibility of object data conversion, which will adapt to its own environment. To convert itself, here we use obj+1, which gives priority to the addition of numbers. Then let's change to alert(obj).
let obj = { valueOf(){ return 1 }, toString(){ return 'character' } }; alert(obj);
Characters will be printed here. Because the alert method takes precedence over the character type.
Finally, let's talk about the last attribute Symbol.toPrimitive.
let obj = { valueOf(){ return 1 }, toString(){ return 'character' }, [Symbol.toPrimitive](){ return 10 } } console.log(obj+1) // 11 alert(obj); //10
Whether it is an addition operation or an alert pop-up. Symbol.toPrimitive is executed. Because it has the highest weight.
5. What is this?
this refers to the context that represents the current environment.
6. How to judge the direction of this
- Default (the method points to whoever)
- Show bindings
- Arrow function
- Strict mode
The first case (whose method points to who)
1,
var a = 2; var obj = { a:1, getVal(){ console.log(this.a); } } obj.getVal(); //1
2,
class A { a(){ console.log(this); } }; const f = new A(); f.a(); // A {}
3,
function fun() { console.log(this) }; fun(); //Window, which is equivalent to window.fun().
Second case (display binding)
The call, apply, and bind methods can all display and change the direction of this.
var value = 3; var obj = { value: 1 } function get() { console.log(this.value); } get(); // 3 get.call(obj); // 1 get.apply(obj); // 1 get.bind(obj)(); // 1
The third case (arrow function)
The arrow function's this point is different from the function's this point. It points to its external context.
var value = 3; var obj = { value: 1, get: ()=> { console.log(this.value); } } obj.get(); // 3
The fourth case (~ ~ strict mode ~ ~)
In strict mode, when a method is called directly, the 'this' inside the method will not point to' window '.
'use strict' function fun() { console.log(this) } fun(); //undefined
7. What is prototype?
Prototype is a prototype object. It is unique to a function. It contains properties and methods shared by all instances of a function (class).
function A() {}; A.prototype.get=()=>{ console.log('I am get method'); } var a1 = new A(); a1.get(); // I'm the get method var a2= new A(); a2.get(); // I'm the get method
a1 and a2 are both instances of a, so they both have get method properties on a's prototype object.
8. What's the difference between setting a method on prototype and setting it on this of the constructor?
function A() {}; A.prototype.get = () => { console.log('I am A'); } function B() { this.get = () => { console.log('I am B'); } };
- The method of binding on `prototype` will only store one copy in memory, and each instance will find the method on the constructor based on the prototype chain, and then call it.
- The method bound to the constructor 'this' will be created in memory every time it is instantiated, and it will be created several times if it is' new'.
9. What is__ proto__?
- __ proto__ Is an internal property of the browser, not a js standard property. (usually we don't operate it directly)
- Every object has__ proto__, And points to the prototype of the constructor. (very important, but null and undefined do not have _proto_)
Second, note that the following two examples return true.
function F() {}; const f = new F(); f.__proto__ === F.prototype?console.log('true'):console.log('false'); //true
const n = new Number(1); n.__proto__ === Number.prototype?console.log('true'):console.log('false'); // true
10. The prototype chain of objects?
Prototype chain:
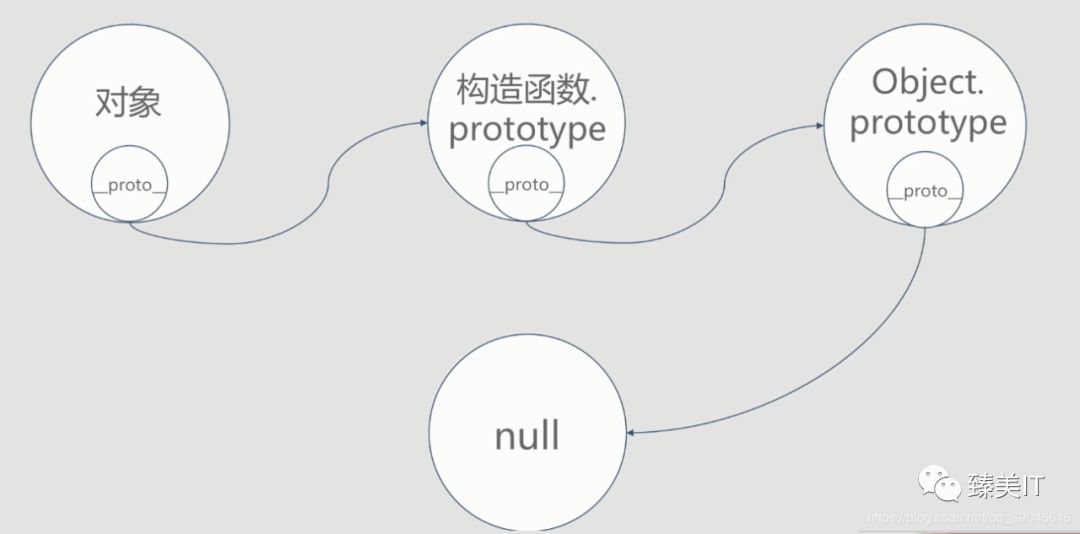
function A() {}; const a = new A(); a.__proto__===A.prototype?console.log('true'):console.log('false');//true console.log(A.prototype); // {constructor:f} A.prototype.get=()=>{ console.log('This is get') } console.log(A.prototype); // {get:f,constructor:f} / / print out as an object // The following two lines of code are examples const obj = new Object({get:()=>{},constructor:()=>{}}) console.log(obj); A.prototype.__proto__===Object.prototype?console.log('true'):console.log('false');//true
11. Prototype chain of constructors?
As we all know, constructors are also objects. We know that there are two ways to create constructors.
1,
function A() {}; console.log(A); //ƒ A() {}
2,
const B = new Function(); console.log(B); //ƒ anonymous() {}
So let's look at a__ Proto = = = function.prototype is established.
function A() {}; A.__proto__===Function.prototype?console.log('true'):console.log('false');//true
Similarly, the prototype chain of the constructor:
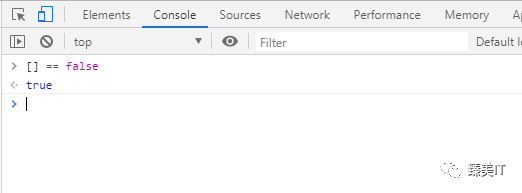
Check it out,
Function.prototype.__proto__===Object.prototype?console.log('true'):console.log('false');//true
So, we have another question? Function.__proto__ To whom?
Function.__proto__===Function.prototype?console.log('true'):console.log('false');//true
Function.prototype is a special built-in object created by the browser itself. Similarly, Object.prototype is also a built-in object created by the browser engine. They all point to null.
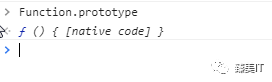
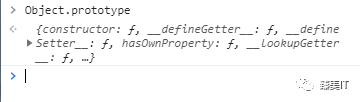
12. What is the constructor on prototype?
The constructor on the prototype object of each function points to the function itself. At present, it has no effect and may be used as instanceof (of course, the pointer of the constructor can also be changed, but it is really useless)
function A() {}; A.prototype.constructor===A?console.log('true'):console.log('false');//true
function A() {}; const a = new A(); console.log(a instanceof A) // true
13. What are the functions of call, apply and bind?
They are all to change the direction of this within the method.
14. What is the difference between call and apply?
- The first parameters of call and apply are the direction of this.
- The rest of the call parameters are a normal parameter list.
- Except for the first parameter, apply accepts only one parameter of array type.
call usage:
const obj = {name:'maomin'}; function get(age,sex) { console.log(` My name: ${this.name} My age: ${age} My gender: ${sex} `) } get.call(obj,18,'male'); //My name: maomin //My age: 18 //My gender: Male
Usage of apply:
const obj = {name:'maomin'}; function get(age,sex) { console.log(` My name: ${this.name} My age: ${age} My gender: ${sex} `) } get.apply(obj,[18,'male']); //My name: maomin //My age: 18 //My gender: Male
15. How is bind different from call and apply?
- The first parameter is the point of this, and the other parameters are a common parameter list. (this is similar to call.)
- The returned function is a function that needs to be called again. (bind can realize curry)
1,
function get(x,y,z) { console.log(x,y,z) } console.log(get.bind(null,'red','yellow','blue')) // ƒ (x,y,z) { // console.log(x,y,z) // } get.bind(null,'red','yellow','blue')(); // Red yellow blue
2,
function get(x,y,z) { console.log(this.name,x,y,z) } get.bind({name:'maomin'},'red','yellow','blue')(); // maomin red yellow blue
Coriolis:
function get(x,y,z) { console.log(this.name,x,y,z) } get.bind({name:'maomin'}).bind(null,'red').bind(null,'yellow').bind(null,'blue')(); // maomin red yellow blue