This paper uses Processing to complete a case of 3D random rendering. Because the final animation effect is like a swarm of geese hovering and flying, I named it the "swarm experiment".
To simplify the explanation, we first use a cube rotation to illustrate the code as follows:
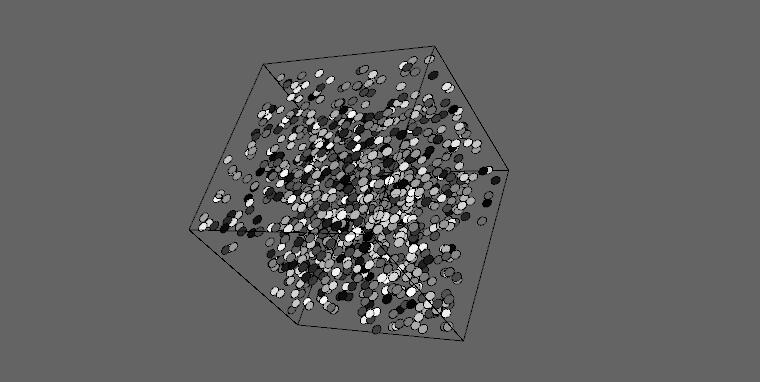
float angle=0; void setup() { size(500, 500, P3D); smooth(); } void draw() { background(100); translate(width/2, height/2, 0); rotateY(angle/6); rotateX(angle/6); angle+=0.05; noFill(); box(200, 200, 200); }
The results are as follows:
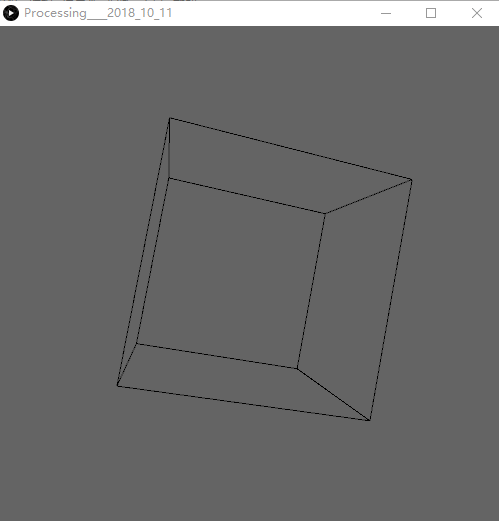
In the above case, the frame of the cube is used as the coordinate range, filling in the circle (that is, our geese). The final code is as follows:
float angle=0; void setup() { size(500, 500, P3D); smooth(); } void draw() { background(100); translate(width/2, height/2, 0); rotateY(angle/6); rotateX(angle/6); angle+=0.05; noFill(); box(200, 200, 200); translate(0, 0, -100); for (int i=0; i<=1000; i++) { drawCircle(10, random(-100, 100), random(-100, 100)); } } void drawCircle(int r, float x, float y) { translate(0, 0, 0.2);//Note here that 0.2 * 1000 of the cycle above = 200 of the edge length on the Z axis fill(random(255)); ellipse(x, y, r, r); }
The results are as follows:
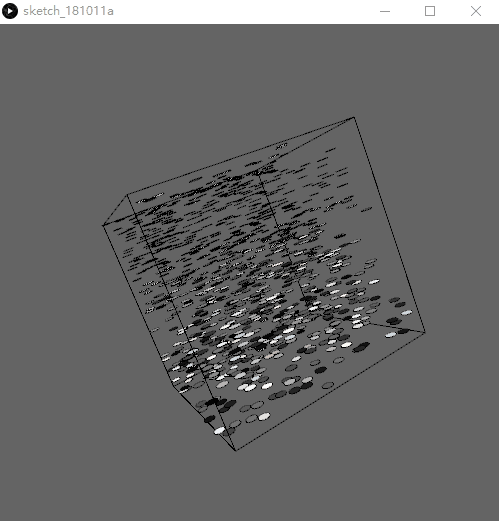
One problem to note here is that the code translate(0, 0, 0.2) above pays attention to 0.2* 1000 = 200 on the Z axis of the loop above. If translate (0, 0, random (200) is used directly, errors will occur because the translate effect in the loop is superimposed. Or alternatively, return to the original coordinate system at the end of the loop, code as follows:
void myLine() { float aa=random(200); translate(0,0,aa); stroke(random(255)); line(random(-100,100),random(-100,100),random(-100,100),random(-100,100)); translate(0,0,-aa); //Here we return to the original coordinate system. }
Remark the auxiliary border, the effect is as follows:
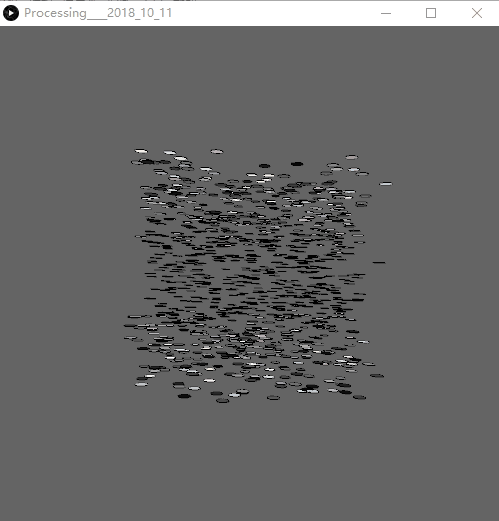
Change the color, the effect is as follows:
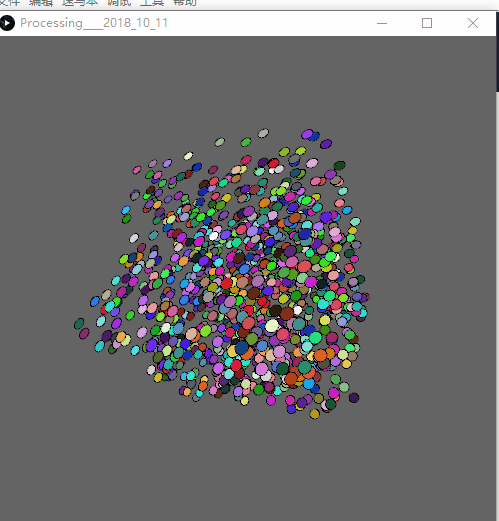
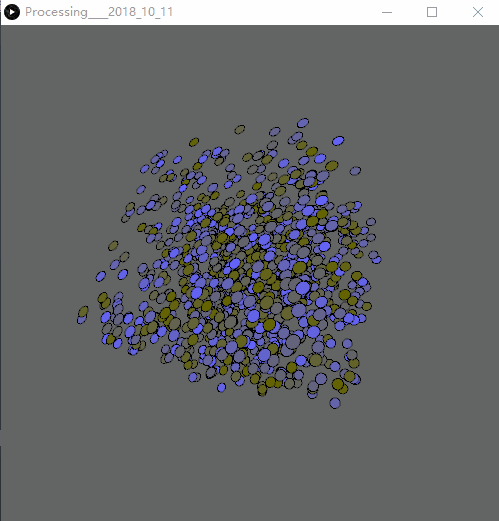
"Big Goose" try to change to a straight line.
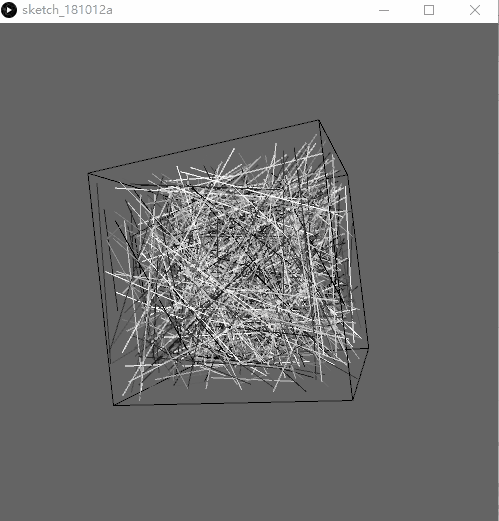
float angle=0; void setup() { size(500, 500, P3D); } void draw() { background(100); translate(width/2, height/2, 0); rotateX(angle/4); rotateZ(angle/4); rotateY(angle/4); angle+=0.1; noFill(); stroke(0); box(200); translate(0,0,-100); for(int i=0;i<1000;i++){ myLine(); } } void myLine() { translate(0,0,0.2); stroke(random(255)); line(random(-100,100),random(-100,100),random(-100,100),random(-100,100)); }
Extension:
Add the following code to draw Circle () or myLine() to try.
rotateX(random(PI)); rotateY(random(PI)); rotateZ(random(PI));