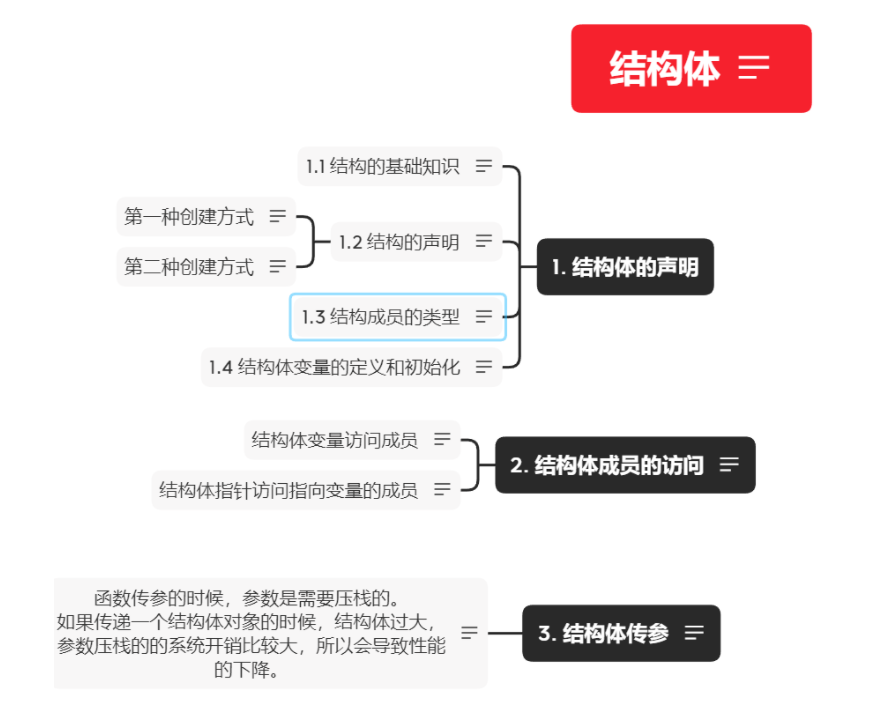
1. Declaration of structure
1.1 basic knowledge of structure
The objects we see in life are complex objects, such as describing a cat or a book. We can't use simple type or floating point type to describe these types
1.2 declaration of structure
struct tag { member-list; }variable-list;
Let's say I want to describe a pokemon
I need to describe it from a variety of characteristics, such as name, Atlas number, height or weight
First creation method
struct Pokemon { char name[20];//name int id;//Atlas No float height; //height float weight;//weight char fighting_type[20]; //attribute char species[15]; //type };
int main() { struct Pokemon pikachu;//Take this structure to create a variable. The variable type is this structure Pokemon return 0; }
The second creation method
struct Pokemon { char name[20];//name int id;//Atlas No float height; //height float weight;//weight char fighting_type[20]; //attribute char species[15]; //type }pokemon1,pokemon2;//overall situation
The difference between the two creation methods is that the structure variables created by the second creation method are global
1.3 types of structure members
The members of a structure can be scalars, arrays, pointers, or even other structures
1.4 definition and initialization of structure variables
Like arrays, structures are initialized, defined in the order and type previously defined
struct Pokemon pikachu = { "Pikachu",25,0.4,6.0,"electric","mouse pokemon" };
2. Access of structure members
I've written it before, so I won't write it in detail
- Structure variable access member
Members of structure variables are accessed through the point operator (.). The point operator accepts two operands.
struct S s; strcpy(s.name, "zhangsan");//Use. To access the name member s.age = 20;//Use. To access age members
Or output
printf("name = %s id = %d height = %.1f weight = %.1f\n", pikachu.name, pikachu.id, pikachu.height, pikachu.weight);
- Structure pointers access members that point to variables
Sometimes what we get is not a structure variable, but a pointer to a structure (- >).
struct Pokemon* ps = &pikachu;//Structure pointer printf("name = %s id = %d height = %.1f weight = %.1f\n", ps->name, ps->id, ps->height, ps->weight); printf("name = %s id = %d height = %.1f weight = %.1f\n", (*ps).name, (*ps).id, (*ps).height, (*ps).weight);
3. Structure transmission parameters
When we need to pass parameters to Print the array, which Print function will be better
struct S { int arr[1000]; float f; char ch[100]; }; void print1(struct S tmp) { int i = 0; for (i = 0; i < 10; i++) { printf("%d ", tmp.arr[i]); } printf("\n"); printf("%f\n", tmp.f); printf("%s\n", tmp.ch); } void print2(struct S* ps) { int i = 0; for (i = 0; i < 10; i++) { printf("%d ", ps->arr[i]); } printf("\n"); printf("%f\n", ps->f); printf("%s\n", ps->ch); } int main() { struct S s = { {1,2,3,4,5,6,7,8,9,10}, 5.5f, "Hello there" }; print1(s); print2(&s); return 0; }
print1 is a value transfer parameter, so a temporary copy will be created, but re creating a space will cause a waste of space. If the content contained in the structure is large enough, it will lead to problems
print2 is address passing, so you don't need to create an entire structure, just a pointer. When the structure is large enough, the efficiency of saving space will be very high.
The following is a professional explanation:
- When a function passes parameters, the parameters need to be pressed on the stack.
If the structure is too large when passing a structure object, the system overhead of parameter stack pressing is relatively large, which will lead to performance degradation.
When the program is running, the process of function stack is
For example, execute such a piece of code
int Add(int x, int y) { int z = 0; z = x + y; return z; } int main() { int a = 10; int b = 20; int c = Add(a, b); printf("%d\n", c); return 0; }
The process of program execution is to create from bottom to top and from first to last in the stack according to the code order of the main function
Summary:
Generally, it is a better choice if we use the address when passing the parameter structure
summary
The old fellow must have a good commentary.