<?php $i = 0; do { echo $i; } while ($i > 0); ?>
for (expression 1; expression 2; expression 3){ Code snippets to execute }
<?php for ($i = 1; $i <= 10; $i++) { if($i == 4){ break; } echo 'After breaking up'.$i.'Years, I forgot all about you<br />'; } ?>
<?php for($i=0; $i<100; $i++) { echo 'No.'. $i .'11<br />'; if($i == 17){ goto end; } } end: echo '22'; ?>
<?php goto wan; echo '11'; wan: echo '12'; ?>
<?php function test( $arg = 10){ echo $arg; } test(); test(88); ?>
<?php function test( $a , $b = 20 , $c = 30){ echo $a + $b + $c; } test( 1 , 2 , 3 ); ?>
<?php $hello = 10; function demo( $hello ){ $hello = 250; echo $hello + $hello; } demo($hello); echo $hello; ?>
<?php function demo(){ echo 111; return; echo 222; } demo(); ?>
<?php function php_cn(){ $foo = 5; $bar = 6; $result = $foo + $bar; return $result; } $piao = php_cn(); echo $piao; ?>
<?php function demo(){ echo 'King Gekko'; } function test(){ echo 'Stew mushrooms with chicken'; } $fu = 'demo'; $fu(); ?>
<?php $hello = 'world'; $world = 'Hello'; //The output is: Hello echo $$hello; ?>
Anonymous functions for php custom functions
Anonymous means no name.
Anonymous functions, that is, functions without function names.
Anonymous function of variable function
<?php $greet = function($name) { echo $name.',Hello'; }; $greet('11'); $greet('22'); ?>
Internal function refers to the declaration of a function within a function.
<?php function hello(){ $GLOBALS['que'] = '12'; echo '3<br />'; } hello(); echo $que; ?>
<?php $hello = 10; echo $GLOBALS['hello'].'<br />'; $GLOBALS['hello'] = 'I love you'; echo $hello; ?>
<?php $one = 10; function demo(){ $two = 100; $result = $two + $GLOBALS['one']; return $result; } //You will find that the result is 110 echo demo(); ?>
<?php function hello(){ $GLOBALS['que'] = '111'; echo 'You adjusted the function hello<br />'; } hello(); echo $que; ?>
<?php $a = 10; $b = &$a; $a = 100; echo $a.'---------'.$b; ?>
<?php $foo = 100; //Note: An amp ersand is added before $n function demo(&$n){ $n = 10; return $n + $n; } echo demo($foo).'<br />'; //You'll get a $foo value of 10 echo $foo; ?>
Common functions in php mathematics
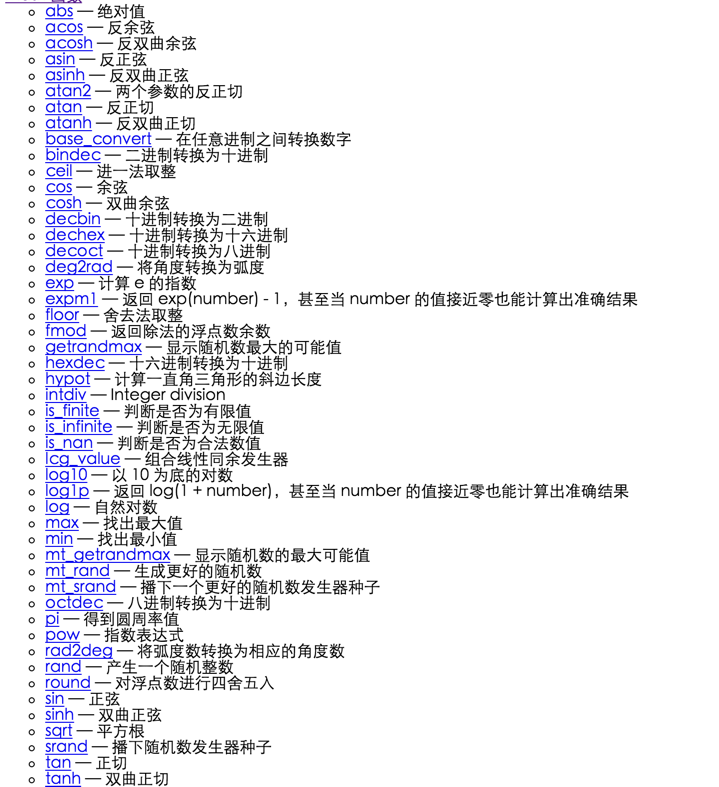
Get Period Time Information Function
1. Time Zone
2. Universal Time 3.unix timestamp
The function to set the time zone is:
1).date_default_timezone_get()
2).date_default_timezone_set()
<?php echo date_default_timezone_get (); ?>
<?php //Define the time zone constant and you can put it in the configuration file later define('TIME_ZONE','Asia/shanghai'); //Execute Function date_default_timezone_set(TIME_ZONE); echo date('Y-m-d H:i:s'); ?>
time() Gets the current unix timestamp
<?php $time=time(); print_r( $time); ?>
<?php echo date('Y year m month d day'); ?>
<?php echo date('Y-m-d H:i:s'); ?>
getdate gets the current system time
microtime(), which returns the current Unix timestamp and microseconds
Common PHP functions:
trim() removes spaces or other predefined characters at both ends of a string
rtrim() removes spaces or other predefined characters to the right of a string
ltrim() removes spaces or other predefined characters to the left of the string
The directory part of the dirname() loop path
str_pad() fills the string with the specified length
str_repeat() reuses the specified string
str_split() splits a string into an array
strrev() invert string
wordwrap() wraps a string at a specified length
str_shuffle() randomly shuffles all characters in a string
parse_str() parses a string into a variable
number_format() formats numbers by grouping them in thousands
strtolower() string lowercase
strtoupper() string uppercase
ucfirst() string initial capitalization
ucwords() string The first character of each word is capitalized
str_shuffle() randomly shuffles all characters in a string
parse_str() parses a string into a variable
php date validation function
Chedate can determine whether an output date is valid.
The syntax format of the function is as follows:
bool checkdate ( int $month , int $day , int $year )
Get Localized Timestamp Function
The mktime() function can obtain a localized timestamp for a date and time
<?php //now is the current time echo strtotime("now")."<br />"; //10 September 2000 echo strtotime("10 September 2000")."<br />"; //Current time plus one day echo strtotime("+1 day")."<br />"; //Current time plus one week echo strtotime("+1 week")."<br />"; //Current time plus 2 days a week, 4 hours, 2 seconds echo strtotime("+1 week 2 days 4 hours 2 seconds")."<br />"; //Next Thursday echo strtotime("next Thursday")."<br />"; //Last Monday echo strtotime("last Monday")."<br />"; ?>
<?php //start time $time_start = microtime(true); //10,000 cycles for($i = 0 ; $i < 10000 ; $i++){ } //Finishing time $time_end = microtime(true); $time = $time_end - $time_start; echo "This script executes at $time seconds\n"; ?>
PHP Arrays and Data Structure
<?php $minren = array( 'Yang Mi', 'Wang Rodan', 'Liu Yifei', 'Huang Shengyi', 'Fan Bingbing' ); $minren[5] = 'Master Fan'; $minren[2] = 'Yifei'; echo '<pre>'; var_dump($minren); echo '</pre>'; ?>
Int count (mixed $variable)
<?php list($one , $two , $three) = array('Zhang San' ,'Li Si' ,'King Five'); //Again: single quotes do not resolve variables, so the output is the string $one echo '$one----'.$one.'<br />'; echo '$two----'.$two.'<br />'; echo '$three----'.$three.'<br />'; ?>
array_shift pops up the first element in the array array_unshift pushes elements at the beginning of the array array_push pushes an element at the end of the array array_pop pops up the last element at the end of the array Current reads out the value of the current position of the pointer Key Reads the key at the current position of the pointer next pointer down prev moves up reset pointer to start end Pointer to end
array_rand() randomly extracts one or more elements from the array, noting the key name array_unique() deletes duplicate values and returns the remaining array sort() Sorts the values of a given array in ascending order without preserving the key name rsort() reverse-sorts the array without preserving the key name asort() sorts arrays and maintains indexed relationships arsort() sorts arrays in reverse order to maintain indexed relationships ksort() key name sort array krsort() sorts arrays in reverse order by key natsort() uses a natural order algorithm to sort elements in an array natcasesort() natural sort, case-insensitive array_filter() removes empty or predefined elements from the array extract changes key to variable name and value to variable value
range() creates and returns an array of elements containing the specified range. compact() creates an array of variables with parameters array_fill() generates an array with a given value array_chunk() splits an array into a new array block array_merge() combines two or more numbers into an array array_slice() takes a value from the array based on a condition and returns it array_diff() returns the difference set array of two arrays array_search() searches for a given value in an array and returns the corresponding key name if successful array_splice() removes part of the array and replaces it with other values array_sum() calculates the sum of all the values in the array in_array() checks if a value exists in the array array_key_exists() checks if the given key name or index exists in the array shuffle() shuffles the array, preserving key values count() counts the number of cells in an array or the number of attributes in an object array_flip() returns an array with inverted key values array_keys() returns all keys of an array, forming an array array_values() returns all the values in the array to form an array array_reverse() returns an array of elements in reverse order
Atoms are the smallest units in a regular representation
\d matches a 0-9 \D All characters except 0-9 \w a-zA-Z0-9_ \W All Characters except 0-9A-Za-z_ \s Matches all whitespace characters\ntr spaces \S Matches all non-whitespace characters [] Atoms of a specified range
<?php //A temporary file was created $handle = tmpfile(); //Write data inside $numbytes = fwrite($handle, 'Write to Temporary File'); //Close the temporary file and it will be deleted fclose($handle); echo 'Written to temporary file'.$numbytes . 'Bytes'; ?>
<?php //Old File Name $filename = 'copy.txt'; //New File Name $filename2 = $filename . '_new'; //Modify the name. copy($filename, $filename2); ?>
<?php $filename = 'demo.txt'; if (file_exists($filename)) { echo '$filename The last time a file was accessed was:' . date("Y-m-d H:i:s", fileatime($filename)); echo '$filename The file was created at: ' . date("Y-m-d H:i:s", filectime($filename)); echo '$filename The modification time of the file is: ' . date("Y-m-d H:i:s", filemtime($filename)); } ?>
Note php.ini file for file upload
<form action="" enctype="multipart/form-data" method="post" name="uploadfile">Upload file:<input type="file" name="upfile" /><br> <input type="submit" value="upload" /></form> <?php //print_r($_FILES["upfile"]); if(is_uploaded_file($_FILES['upfile']['tmp_name'])){ $upfile=$_FILES["upfile"]; //Get the values in the array $name=$upfile["name"];//File name of uploaded file $type=$upfile["type"];//Type of uploaded file $size=$upfile["size"];//Size of uploaded file $tmp_name=$upfile["tmp_name"];//Temporary storage path for uploaded files //Determine if it is a picture switch ($type){ case 'image/pjpeg':$okType=true; break; case 'image/jpeg':$okType=true; break; case 'image/gif':$okType=true; break; case 'image/png':$okType=true; break; } if($okType){ /** * 0:File uploaded successfully <br/> * 1: File size exceeded, set <br/>in php.ini file * 2: Exceeded the value specified by the file size MAX_FILE_SIZE option <br/> * 3: Only part of the file was uploaded <br/> * 4: No files were uploaded <br/> * 5: Upload file size is 0 */ $error=$upfile["error"];//Values returned by the system after uploading echo "================<br/>"; echo "The upload file name is:".$name."<br/>"; echo "The upload file type is:".$type."<br/>"; echo "The upload file size is:".$size."<br/>"; echo "After uploading, the system returns the following values:".$error."<br/>"; echo "The temporary storage path for uploaded files is:".$tmp_name."<br/>"; echo "Start moving uploaded files<br/>"; //Move the temporary uploaded file under the up directory move_uploaded_file($tmp_name,'up/'.$name); $destination="up/".$name; echo "================<br/>"; echo "Upload information:<br/>"; if($error==0){ echo "File upload was successful!"; echo "<br>Picture Preview:<br>"; echo "<img src=".$destination.">"; //Echo "alt=\" picture preview: \r file name: ". $destination." r upload time: \">"; }elseif ($error==1){ echo "Exceeded file size, in php.ini File settings"; }elseif ($error==2){ echo "Exceeded file size MAX_FILE_SIZE Value specified by option"; }elseif ($error==3){ echo "Only part of the file was uploaded"; }elseif ($error==4){ echo "No files uploaded"; }else{ echo "Upload file size is 0"; } }else{ echo "Please upload jpg,gif,png Pictures in equal format!"; } } ?>
Steps for uploading php files
<form action="" enctype="multipart/form-data" method="post" name="uploadfile">Upload file:<input type="file" name="upfile" /><br> <input type="submit" value="upload" /></form> <?php //print_r($_FILES["upfile"]); if(is_uploaded_file($_FILES['upfile']['tmp_name'])){ $upfile=$_FILES["upfile"]; //Get the values in the array $name=$upfile["name"];//File name of uploaded file $type=$upfile["type"];//Type of uploaded file $size=$upfile["size"];//Size of uploaded file $tmp_name=$upfile["tmp_name"];//Temporary storage path for uploaded files //Determine if it is a picture switch ($type){ case 'image/pjpeg':$okType=true; break; case 'image/jpeg':$okType=true; break; case 'image/gif':$okType=true; break; case 'image/png':$okType=true; break; } if($okType){ /** * 0:File uploaded successfully <br/> * 1: File size exceeded, set <br/>in php.ini file * 2: Exceeded the value specified by the file size MAX_FILE_SIZE option <br/> * 3: Only part of the file was uploaded <br/> * 4: No files were uploaded <br/> * 5: Upload file size is 0 */ $error=$upfile["error"];//Values returned by the system after uploading echo "================<br/>"; echo "The upload file name is:".$name."<br/>"; echo "The upload file type is:".$type."<br/>"; echo "The upload file size is:".$size."<br/>"; echo "After uploading, the system returns the following values:".$error."<br/>"; echo "The temporary storage path for uploaded files is:".$tmp_name."<br/>"; echo "Start moving uploaded files<br/>"; //Move the temporary uploaded file under the up directory move_uploaded_file($tmp_name,'up/'.$name); $destination="up/".$name; echo "================<br/>"; echo "Upload information:<br/>"; if($error==0){ echo "File upload was successful!"; echo "<br>Picture Preview:<br>"; echo "<img src=".$destination.">"; //Echo "alt=\" picture preview: \r file name: ". $destination." r upload time: \">"; }elseif ($error==1){ echo "Exceeded file size, in php.ini File settings"; }elseif ($error==2){ echo "Exceeded file size MAX_FILE_SIZE Value specified by option"; }elseif ($error==3){ echo "Only part of the file was uploaded"; }elseif ($error==4){ echo "No files uploaded"; }else{ echo "Upload file size is 0"; } }else{ echo "Please upload jpg,gif,png Pictures in equal format!"; } } ?>
php file upload form considerations
<html> <head> <meta charset="utf-8" /> <title>Single file upload</title> </head> <body> <form action="file.php" method="post" enctype="multipart/form-data"> <input type="file" name="file"> <input type="submit" value="upload"> </form> </body> </html>
<?php if ($_FILES['file']['error'] > 0) { switch ($_FILES['file']['error']) { //Error code is not zero, an error occurred during file upload case '1': echo 'File too large'; break; case '2': echo 'File exceeds specified size'; break; case '3': echo 'Only some files were uploaded'; break; case '4': echo 'File not uploaded'; break; case '6': echo 'The specified folder could not be found'; break; case '7': echo 'File Write Failure'; break; default: echo "Upload error<br/>"; } } else { $MAX_FILE_SIZE = 100000; if ($_FILES['file']['size'] > $MAX_FILE_SIZE) { exit("File exceeds specified size"); } $allowSuffix = array( 'jpg', 'gif', ); $myImg = explode('.', $_FILES['file']['name']); $myImgSuffix = array_pop($myImg); if (!in_array($myImgSuffix, $allowSuffix)) { exit("File suffix name does not match"); } $allowMime = array( "image/jpg", "image/jpeg", "image/pjpeg", "image/gif", ); if (!in_array($_FILES['file']['type'], $allowMime)) { exit('The file format is incorrect, please check'); } $path = "upload/images/"; $name = date('Y') . date('m') . date("d") . date('H') . date('i') . date('s') . rand(0, 9) . '.' . $myImgSuffix; if (is_uploaded_file($_FILEs['file']['tmp_name'])) { if (move_uploaded_file($_FILEs['file']['tmp_name'], $path . $name)) { echo "Upload Successful"; } else { echo 'Upload failed'; } } else { echo 'Not uploading files'; } } ?>
<?php var_dump($_FILES); //Print $_FILES to view array structure ?>
php file upload progress processing
<?php /* Open session.Please note that before session_start(), there should be no action to expect the browser to output content, or this may cause errors. */ session_start(); //ini_get() Gets the value of the environment variable in php.ini $i = ini_get('session.upload_progress.name'); //In ajax, we use the get method, where the variable name is the parameter passed in by the prefix splicing defined in the ini file $key = ini_get("session.upload_progress.prefix") . $_GET[$i]; //Determine if there is information in SESSION to upload files if (!empty($_SESSION[$key])) { //Uploaded Size $current = $_SESSION[$key]["bytes_processed"]; //Total File Size $total = $_SESSION[$key]["content_length"]; //Returns the current percentage of upload progress to ajax. echo $current < $total ? ceil($current / $total * 100) : 100; }else{ echo 100; } ?>
PHP Image Processing
<?php //create picture $img = imagecreatetruecolor(500, 500); //Allocate Colors $red = imagecolorallocate($img, 255, 0, 0); $green = imagecolorallocate($img, 0, 255, 0); $blue = imagecolorallocate($img, 0, 0, 255); $pur = imagecolorallocate($img, 255, 0, 255); $yellow = imagecolorallocate($img, 121, 72, 0); //Fill Background imagefilledrectangle($img, 0, 0, 500, 500, $green); //Draw diagonal lines imageline($img, 0, 0, 500, 500, $red); imageline($img, 500, 0, 0, 500, $blue); //Draw a circle imagefilledellipse($img, 250, 250, 200, 200, $yellow); //Draw a rectangle in the middle of a circle imagefilledrectangle($img, 200, 200, 300, 300, $blue); //Save the picture named haha.jpg imagejpeg($img, 'haha.jpg'); //Destroy resources imagedestroy($img); ?>
php development verification code
<?php check_code(); function check_code($width = 100, $height = 50, $num = 4, $type = 'jpeg') { $img = imagecreate($width, $height); $string = ''; for ($i = 0; $i < $num; $i++) { $rand = mt_rand(0, 2); switch ($rand) { case 0: $ascii = mt_rand(48, 57); //0-9 break; case 1: $ascii = mt_rand(65, 90); //A-Z break; case 2: $ascii = mt_rand(97, 122); //a-z break; } //chr() $string .= sprintf('%c', $ascii); } //background color imagefilledrectangle($img, 0, 0, $width, $height, randBg($img)); //Draw interference elements for ($i = 0; $i < 50; $i++) { imagesetpixel($img, mt_rand(0, $width), mt_rand(0, $height), randPix($img)); } //Write for ($i = 0; $i < $num; $i++) { $x = floor($width / $num) * $i + 2; $y = mt_rand(0, $height - 15); imagechar($img, 5, $x, $y, $string[$i], randPix($img)); } //imagejpeg $func = 'image' . $type; $header = 'Content-type:image/' . $type; if (function_exists($func)) { header($header); $func($img); } else { echo 'Picture type not supported'; } imagedestroy($img); return $string; } //Light background function randBg($img) { return imagecolorallocate($img, mt_rand(130, 255), mt_rand(130, 255), mt_rand(130, 255)); } //Dark words or dots that interfere with these elements function randPix($img) { return imagecolorallocate($img, mt_rand(0, 120), mt_rand(0, 120), mt_rand(0, 120)); } ?>
<?php //Close Error error_reporting(0); //Read a non-existent file, display error //Display error error_reporting(E_ALL & ~ E_NOTICE); ?>
Please compliment!Because your encouragement is my greatest motivation to write!
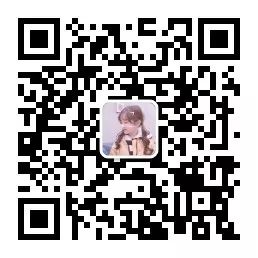
Blow the crowd: 711613774
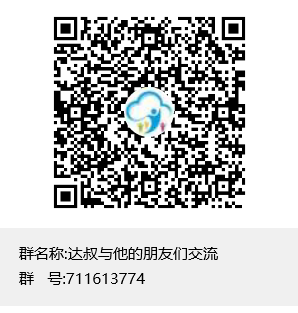