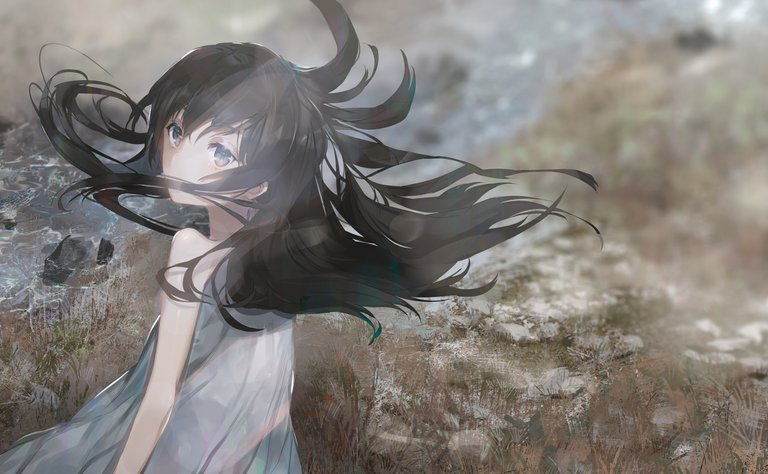
<?php $fileName = "php master.test.php"; //Supplementary program that displays file names (excluding extensions) $start = strrpos($fileName, "."); $newStr = substr($fileName,0, $start); var_dump ( $newStr ); ?>
<?php for($i=100;$i<1000;$i++){ if(($i*$i)%1000==$i) { echo $i; echo "<br/>"; } } ?>
<?php $x = "hello"; switch ($x) { case 1 : echo "Number 1"; break; case 2 : echo "Number 2"; break; case "hello" : echo "hello"; break; default : echo "No number between 1 and 3"; } ?>
<?php $students = array(array("name"=>"Zhang San","age"=>25,"height"=>180),array("name"=>"Li Si","age"=>22,"height"=>170)); echo $students[0]["name"]; echo "<br/>"; echo $students[1]["name"]; ?>
<?php // Show n characters to the right $n = 5; $oldStr = "dsfasfasf"; $rightStr = subStr ( $oldStr, strlen($oldStr) - $n ); var_dump ( $rightStr ); ?>
<html> <body> <form action="welcome.php" method="post"> Name: <input type="text" name="name" /> Age: <input type="text" name="age" /> <input type="submit" /> </form> </body> </html>
Study hard,Make progress every day <br/> <?php echo "hello world"; define("ABC",1000); var_dump(defined("ABC")); echo "<br/>"; echo constant("ABC"); echo PHP_OS; echo "<br/>"; echo PHP_VERSION; echo "<br/>"; echo __FILE__; define('NAME','php'); define('NAME','linux'); echo NAME; ?> <img src="/test/a.jpg"></img>
Modify port number
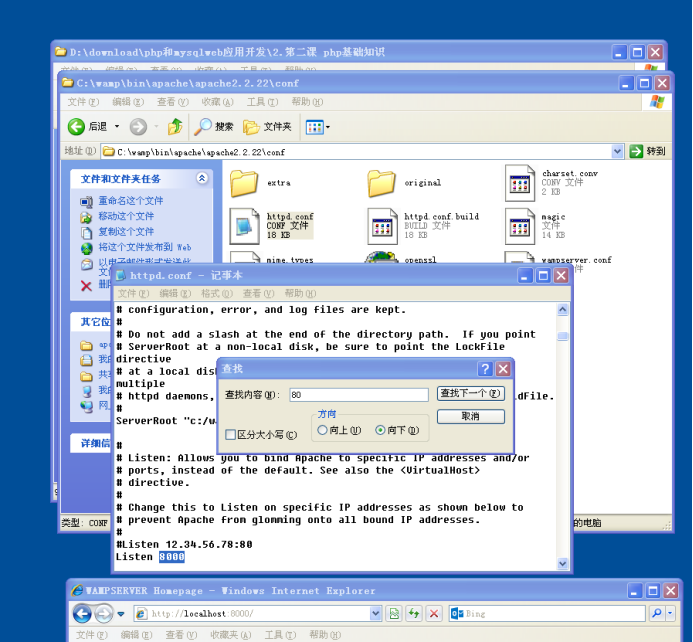
Find the process number through the network command netstat-aon
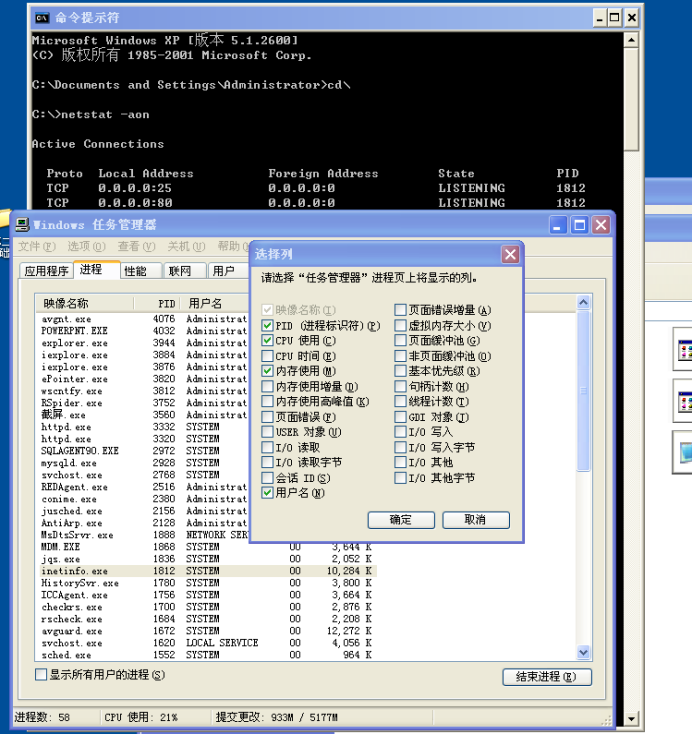
php learning
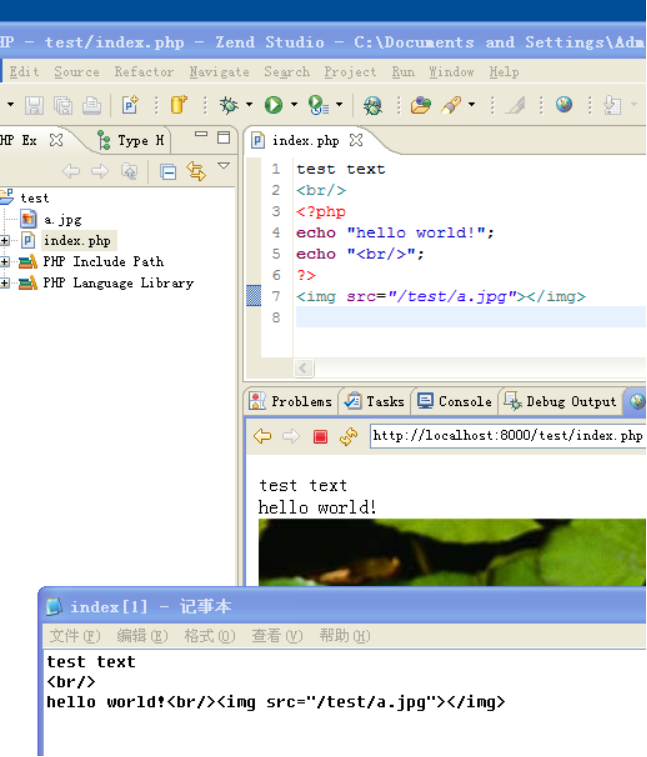
Constants are usually composed of uppercase letters and can only be defined once
bool define ( string name, mixed value [, bool case_insensitive] ) bool defined ( string name ) <?php echo "Chinese Test<br/>"; print "hello world!"; echo "<br/>"; echo "<img src='/test/a.jpg'></img>"; define("PI",3.14); var_dump(defined("Pi")); echo PHP_OS; echo "<br/>"; echo PHP_VERSION; echo "<br/>"; echo __FILE__; ?>
Naming rules for constants and variables:
Start with letters, underscores, followed by letters, numbers, and underscores
Name variables starting with $and assign them before using them
The same variable can store either numbers or strings, that is, any type of data
Variables do not need to be of a specified data type, but must be assigned before they can be used
Find the length of the string: int strlen (string character name)
Find the first occurrence of substring location: int strpos
Find the last occurrence of the substring location: int strrpos
Find a substring of n characters to the right of a string
Substr (original string,-n)
Show file names with extensions removed
$dotpos = strpos($fileName,"."); echo substr($fileName,0, $dotpos);
$y = $x++ Amount to{$y=$x; $x=$x+1;} $y = $x-- Amount to{$y=$x; $x=$x-1;} $y = ++$x Amount to{$x=$x+1; $y=$x;} $y = $x-- Amount to{$x=$x-1; $y=$x;}
For expression: A && B, if A is false, the value of expression B is no longer calculated For expression: A || B, if A is true, the value of expression B is no longer calculated
The date function is used to format a date into a specified format
Construct the array: $names = array("a","b","c"); Access array elements: $names[0], $names[1], $names[2].
Function explode, which splits a string into an array of substrings
Associated Array
Method: "Key"=>Value
data type
Boolean type
integer type
float
String type
Pseudo-type
mixed,number,void,callback
<?php // Ask for 1!+2!.... +10! // Declare a control variable, initialize $i = 1; // Declare a variable to store sums $sum = 0; // Declare a variable to store n!, initialize to 1; $rank = 1; // Calculate the factorial of $i, add up immediately while ( $i <= 10 ) { // Calculate $i!= ($i-1)!*$i $rank *= $i; // accumulation $sum += $rank; // Changing the value of a circular variable $i ++; } echo $sum . "<br/>"; // Ask for 1!+2!.... +10! $x = 1; $sum2 = 0; while ( $x <= 10 ) { // Calculate $x! $rank2 = 1; $y = 1; while ( $y <= $x ) { $rank2 *= $y; $y ++; } // accumulation $sum2 += $rank2; // Changing the value of a circular variable $x ++; } echo $sum2 . "<br/>"; // Find prime numbers between 1.....100 // 8 = 2*47 is a prime number because 7/27/3 7/4....7/6, 7 cannot be divisible for($i = 2; $i <= 100; $i ++) { // Assume a prime number $isPrime = true; //The divisor does not need to be less than $i-1, to sqrt($i) for($j = 2; $j <= sqrt($i); $j ++) { if ($i % $j == 0) { $isPrime = false; break; } } //Verify that assumptions have not been modified if ($isPrime == true) { echo $i . " "; } } $arr=array("one", "two", "three"); //Take out each element of the array in turn and place it in $aa foreach ($arr as $aa){ echo $aa. " "; } echo "<br/>"; // Ask for 1!+2!.... +10! function Rank($n){ $rank = 1; for($i=1;$i<=$n;$i++){ $rank *= $i; } return $rank; } $sum = 0; for($i=1;$i<=10;$i++){ $sum += Rank($i); } echo $sum; ?>
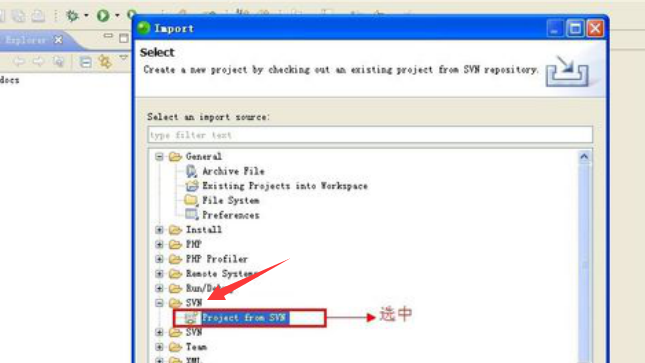
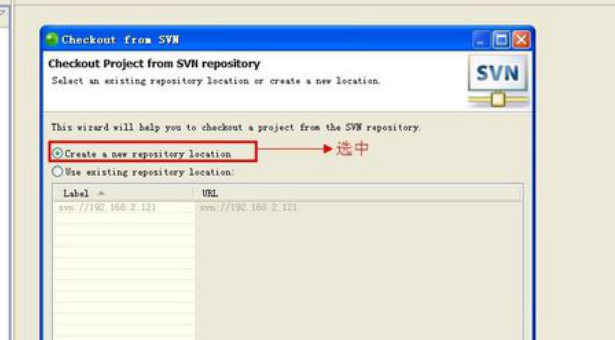
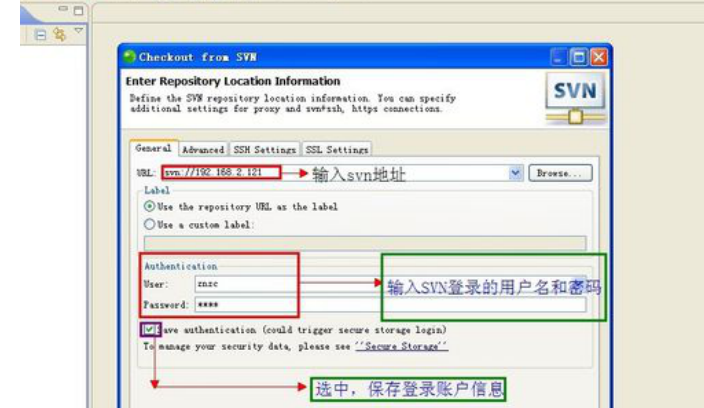
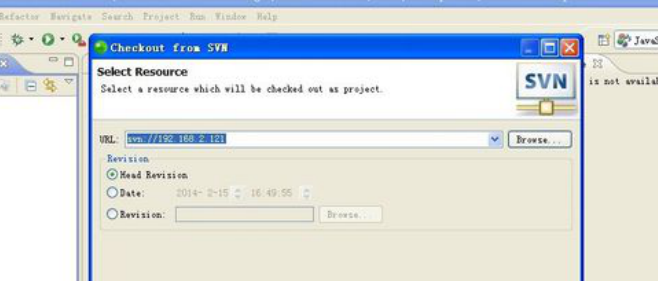
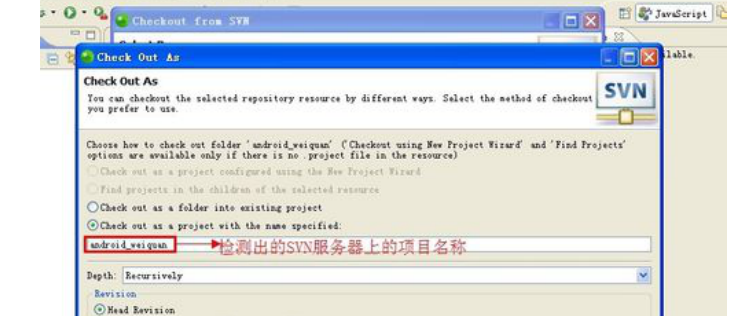
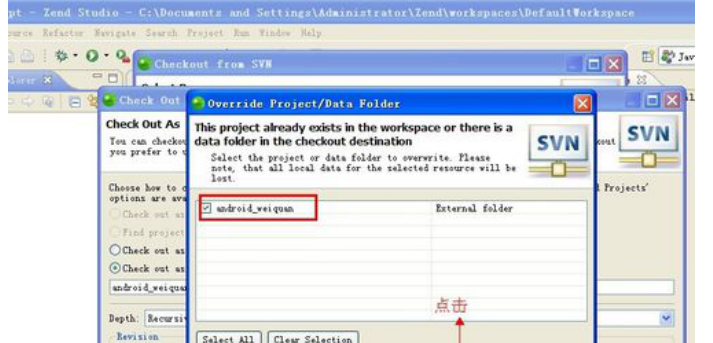
Check in
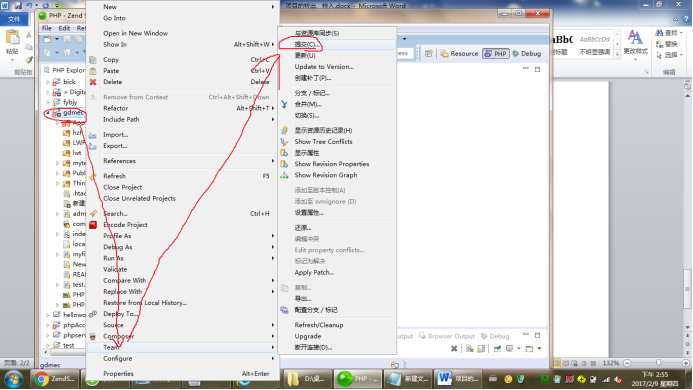
Custom function templates
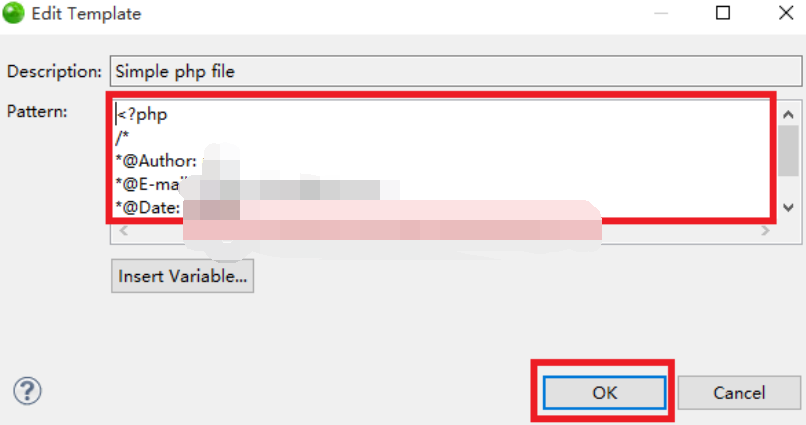
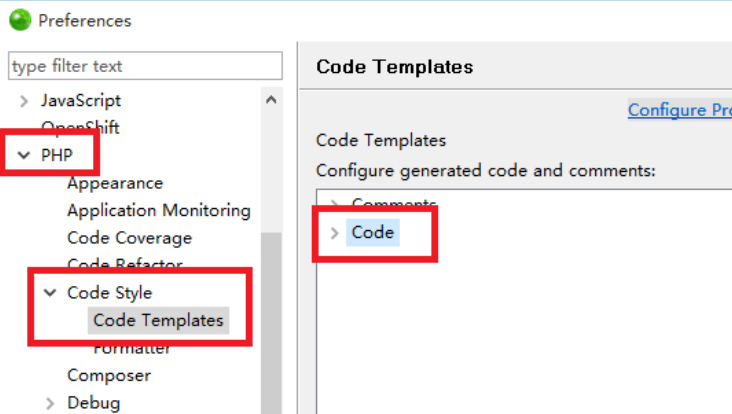
Development environment: wamp3.06 + Zend studio 12
Debugging Configuration
Open PHP configuration file php.ini
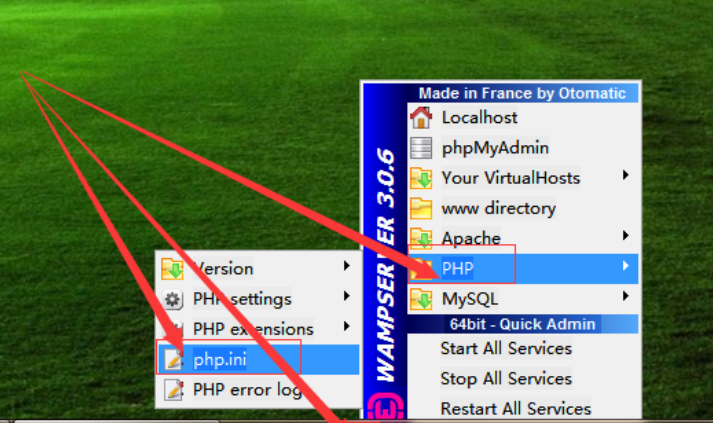
Remove all the comment symbols before xdebug';', that is, use the debugger that comes with wamp
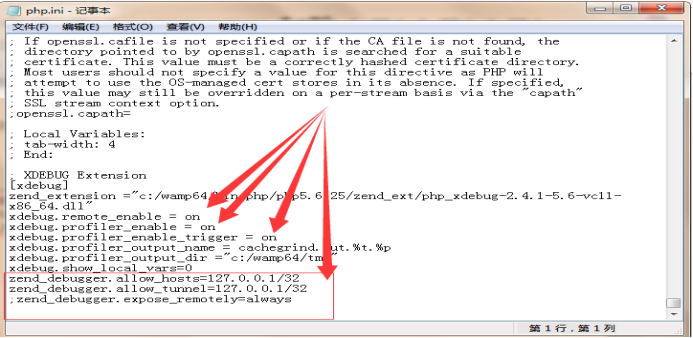

Allow access to server
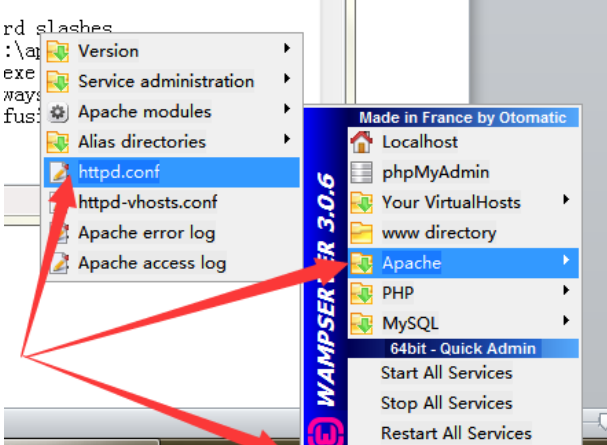
Modify httpd.conf to allow access to the server
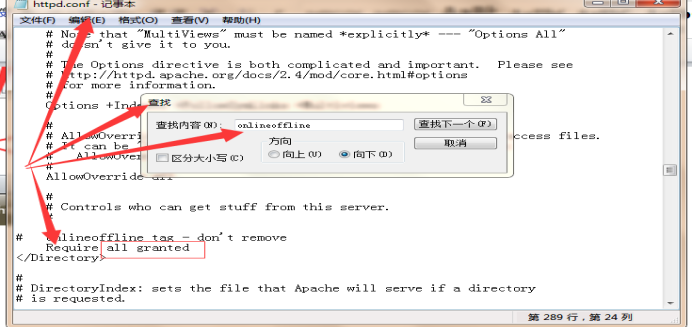
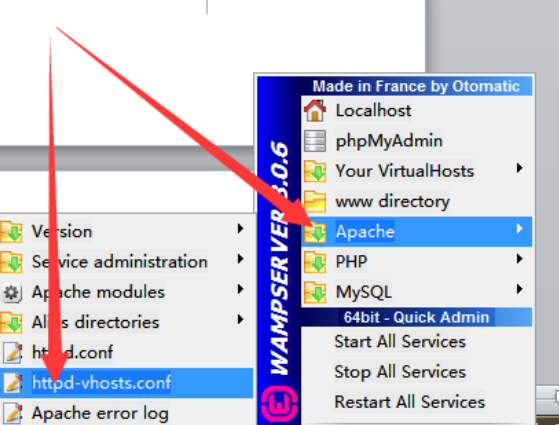
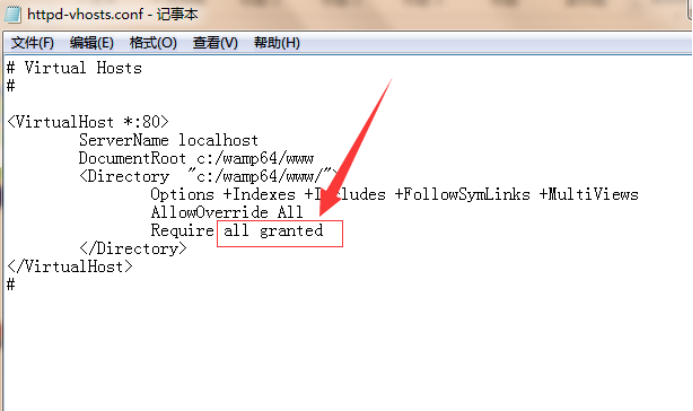
Settings in Zend Studio
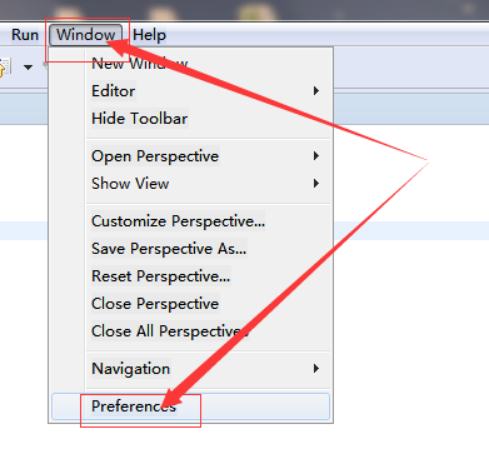
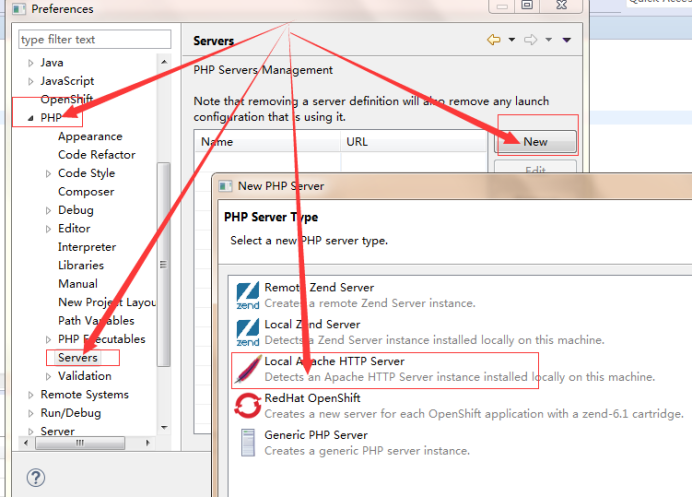
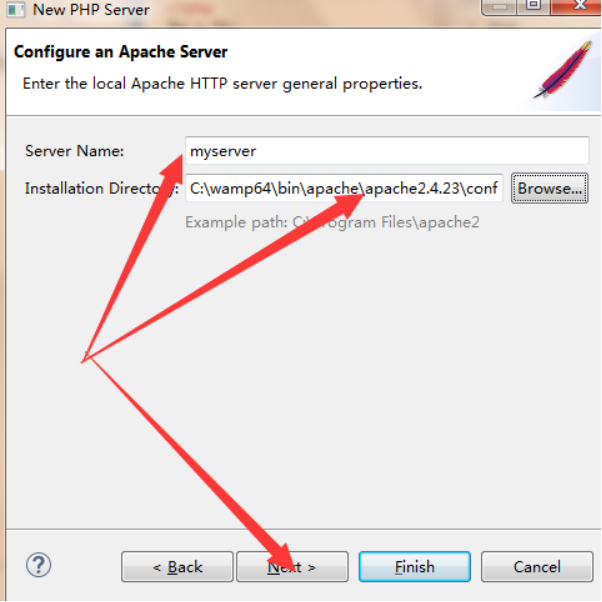
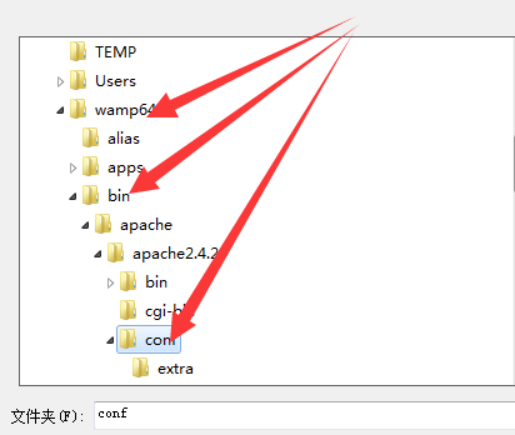
Servers
Configure local Apache HTTP Server
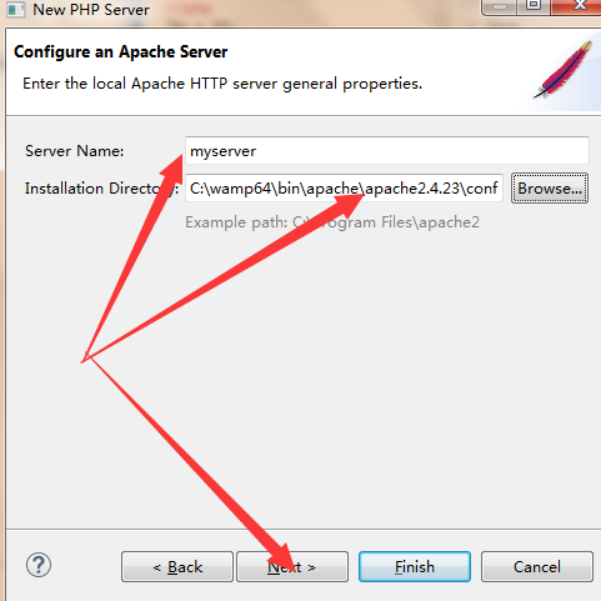
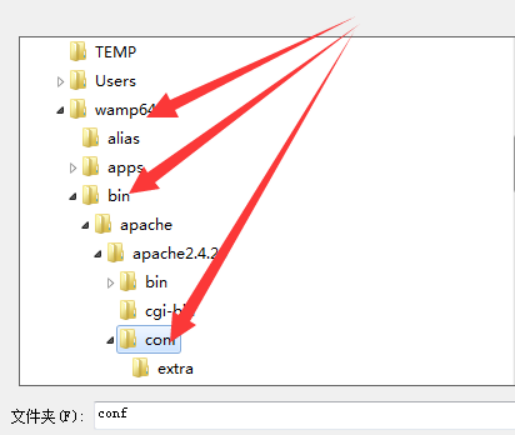
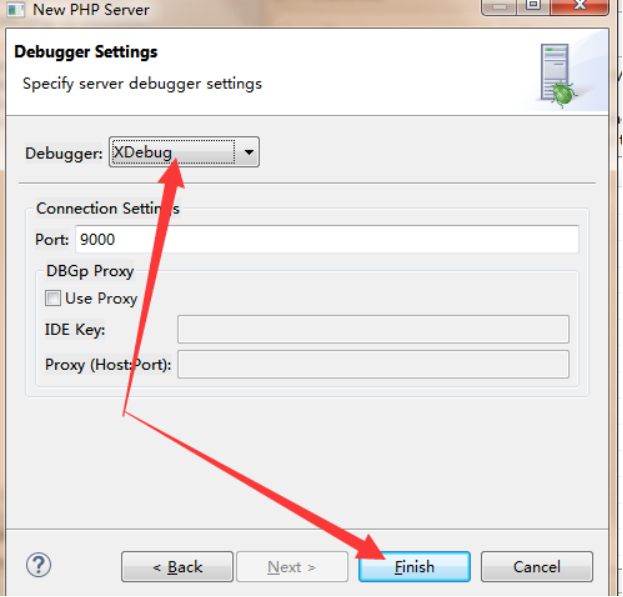
Configuration exe file
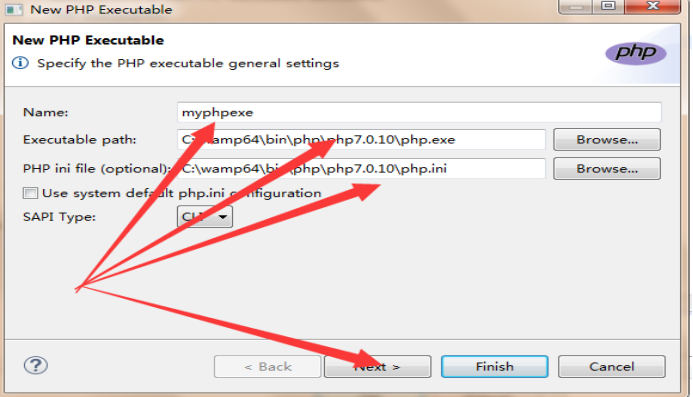
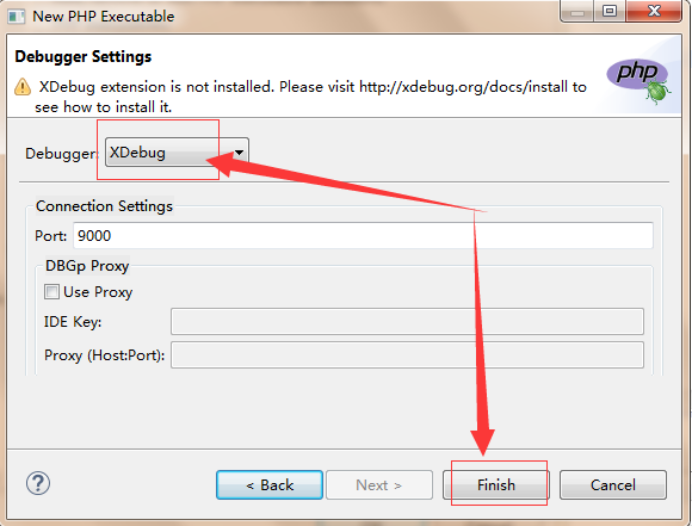
Configure debug
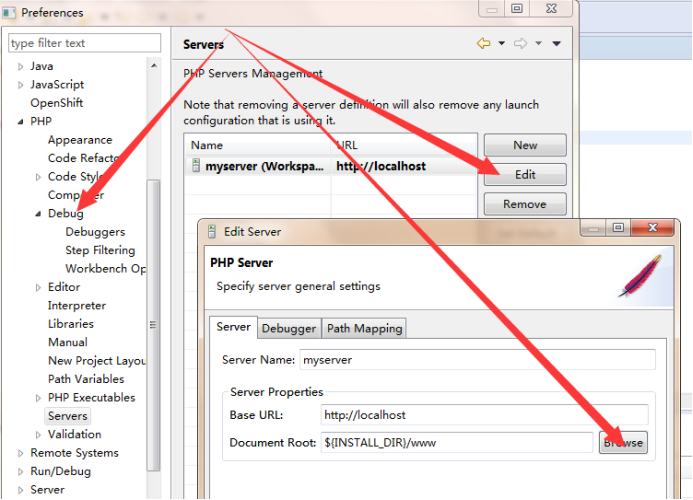
Modify Document Root to
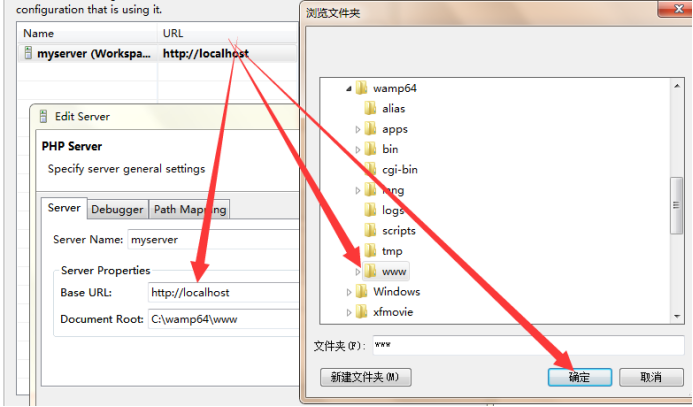
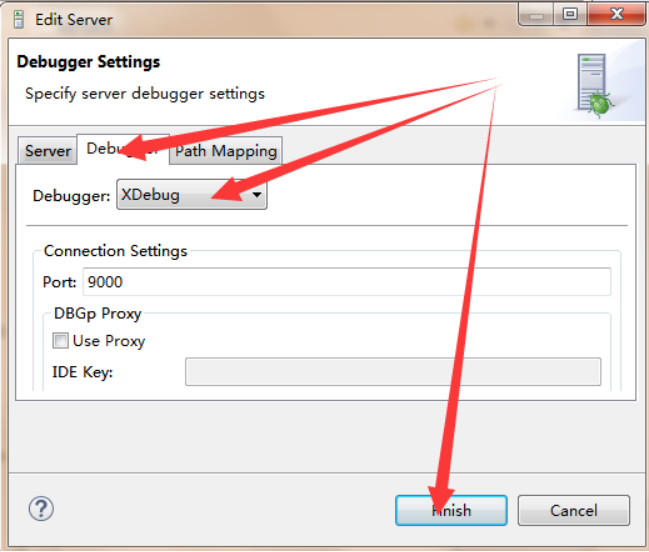
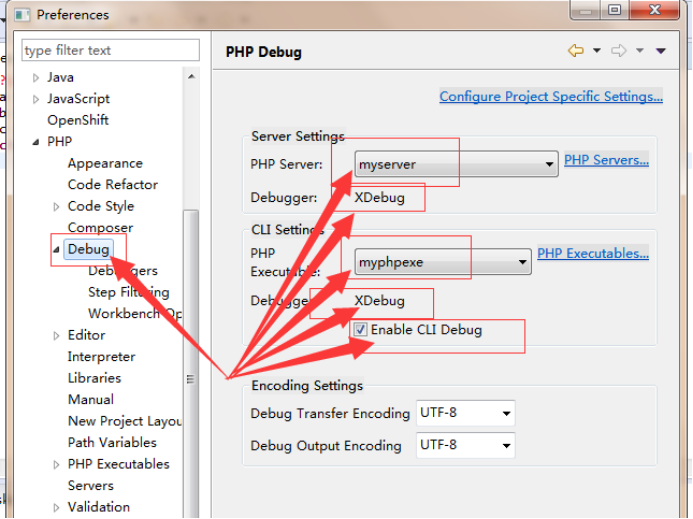
Configure Default Character Set
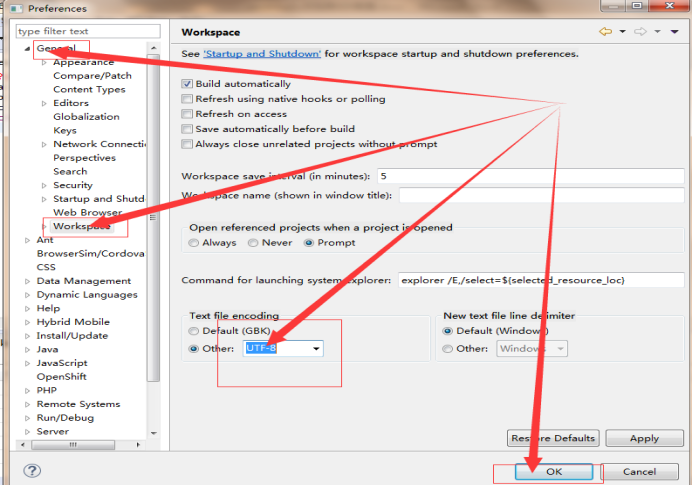
Configure default font size
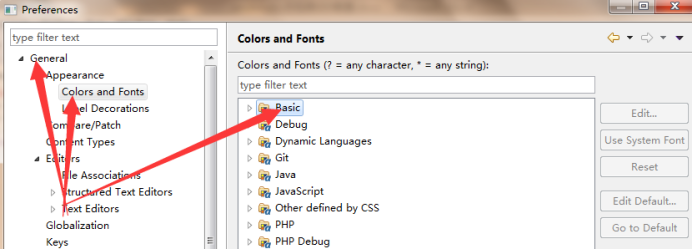
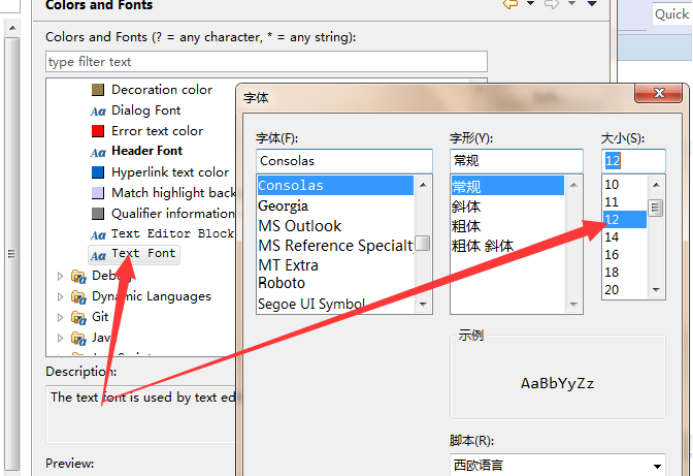
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <?php // if((($_FILES["file"]["type"] == "image/gif") || ($_FILES["file"]["type"] == // "image/jpeg") || ($_FILES["file"]["type"] == "image/pjpeg")) && // ($_FILES["file"]["size"] < 20000)) { // if ($_FILES["file"]["error"] > 0) { // echo "Return Code: " . $_FILES["file"]["error"] . "<br />"; // } // else { if (file_exists ( "upload/" . $_FILES ["file"] ["name"] )) { echo $_FILES ["file"] ["name"] . " already exists. "; } else { $newname = iconv ( "utf-8", "gb2312", $_FILES ["file"] ["name"] ); move_uploaded_file ( $_FILES ["file"] ["tmp_name"], "upload/" . $newname ); // move_uploaded_file($_FILES["file"]["tmp_name"], "upload/" // .$_FILES["file"]["name"]); echo "Stored in: " . "upload/" . $_FILES ["file"] ["name"]; } // } // } else { echo "Invalid file"; } ?> </body> </html>
<html> <body> <?php $week = array("Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"); $day = date("w"); $mydate = date("Hello! Now is Y year n month j day H spot i branch,$week[$day]"); echo $mydate; ?> </body> </html>
<?php session_start(); if(isset($_SESSION['user'])){ header("Location:main.php");//Automatically jump to main.php }else{ //Get user input $username = $_POST [ 'username' ]; $passcode = $_POST [ 'passcode' ]; $cookie = $_POST [ 'cookie' ]; //Determine if the user exists and the password is correct if ($username =="hhh" && $passcode == "12345") { $_SESSION['user']=$username; header("Location:main.php" );//Automatically jump to main.php } else{ echo "ERROR Incorrect username or password"; } } ?>
<?php header('Content-type:text/html;charset=utf-8'); ?> <html> <head> <meta http-equiv= "Content-Type" content=" text/html; charset=UTF-8"> </head> <body> <?php if (isset ( $_COOKIE ["username"] )) { header ( "location: main1.php" ); } else { $username = $_POST ['username']; $passcode = $_POST ['passcode']; $cookie = $_POST ['cookie']; if ($username == "hhh" && $passcode == "12345") { switch ($cookie) { case 0 : setcookie ( "username", $username ); break; case 1 : setcookie ( "username", $username, time () + 24 * 60 * 60 ); break; case 2 : setcookie ( "username", $username, time () + 30 * 24 * 60 * 60 ); break; case 3 : setcookie ( "username", $username, time () + 365 * 24 * 60 * 60 ); break; } header ( "location: main.php" ); } else { echo ""; } } ?> </body> </html>
Send Mailbox
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <html> <body> <?php if (isset($_REQUEST['emailto'])) { $emailto = $_REQUEST['emailto']; $subject = $_REQUEST['subject']; $message = $_REQUEST['message']; if(mail($emailto,$subject,$message,"From:23232323@hzj.com")){ echo "Thank you for using this program!"; }else{ echo "Failed to send successfully!"; } }else{ echo "<form method='post' action='sendemail.php'>EmailTo:<input name='emailto' type='text' /><br />Subject: <input name='subject' type='text' /><br />Message:<br /><textarea name='message' rows='15' cols='40'></textarea><br /><input type='submit' /></form>"; } ?> </body> </html>
The PHP Date() function formats the timestamp into a more readable date and time
grammar
date(format,timestamp)
d - Day of the month (01-31) m - Current month, in numbers (01-12) Y - Current year (four digits)
<?php echo date("Y/m/d"); echo "<br />"; echo date("Y.m.d"); echo "<br />"; echo date("Y-m-d"); ?>
PHP Reference File
include() or require()
They handle errors differently
The include() function generates a warning
The require() function generates a fatal error
PHP File Processing
fopen ( string $filename , string $mode )
Close File
The fclose() function closes an open file
Read files line by line
The fgets() function reads files line by line from the file
PHP file upload
<html> <body> <form action="upload_file.php" method="post" enctype="multipart/form-data"> <label for="file">Filename:</label> <input type="file" name="file" id="file" /> <br /> <input type="submit" name="submit" value="Submit" /> </form> </body> </html>
The enctype property of the <form>tag specifies which content type to use when submitting the form.Use "multipart/form-data" when the form requires binary data, such as file content.
The type="file" attribute of the <input>tag specifies that the input should be processed as a file.
PHP's global array, $_FILES, holds all information about files uploaded to the server
$_FILES['file']['name'] - Name of the file being uploaded $_FILES['file']['type'] - Type of file uploaded $_FILES['file']['size'] - Size of uploaded file $_FILES['file']['tmp_name'] - Name of the temporary copy of the file stored on the server $_FILES['file']['error'] - Error code transmitted from file
"upload_file.php" file
<?php if ($_FILES["file"]["error"] > 0) { echo "Error: " . $_FILES["file"]["error"] . "<br />"; } else { echo "Upload: " . $_FILES["file"]["name"] . "<br />"; echo "Type: " . $_FILES["file"]["type"] . "<br />"; echo "Size: " . ($_FILES["file"]["size"] / 1024) . " Kb<br />"; echo "Stored in: " . $_FILES["file"]["tmp_name"]; } ?>
Save uploaded files
<?php if ((($_FILES["file"]["type"] == "image/gif") || ($_FILES["file"]["type"] == "image/jpeg") || ($_FILES["file"]["type"] == "image/pjpeg")) && ($_FILES["file"]["size"] < 20000)) { if ($_FILES["file"]["error"] > 0) { echo "Return Code: " . $_FILES["file"]["error"] . "<br />"; } else { if (file_exists("upload/" . $_FILES["file"]["name"])) { echo $_FILES["file"]["name"] . " already exists. "; } else { move_uploaded_file($_FILES["file"]["tmp_name"], "upload/" . $_FILES["file"]["name"]); echo "Stored in: " . "upload/" . $_FILES["file"]["name"]; } } } else { echo "Invalid file"; } ?>
coding scheme
<?php header('Content-type: text/html; charset=gbk');?>//Place at the top of the php document
<meta http-equiv= "Content-Type" content=" text/html; charset=UTF-8">
Coding Conversion
string iconv ( string $in_charset , string $out_charset , string $str )
What is a Cookie?
Cookies - Save information on the client
Cookies are often used to identify users.A cookie is a small file that the server leaves on the user's computer.Whenever the same computer requests a page through a browser, it also sends a cookie.With PHP, you can create and retrieve the value of a cookie.
How do I create a cookie?
The setcookie() function is used to set the cookie.
grammar
setcookie(name, value, expire, path, domain);
How do I delete cookie s?
<?php // set the expiration date to one hour ago setcookie("user", "", time()-3600); ?>
Session - Save user information on the server side
The PHP session variable is used to store information about a user session or to change the settings for a user session.
Session works by creating a unique id (UID) for each visitor and storing variables based on it.UIDs are stored in cookie s or are transmitted through URL s.
Session life cycle
The start session_start() function must precede the <html>tag
Store Session Variable
Use PHP $_SESSION variable
<?php session_start(); $_SESSION['views']=1; ?> <?php echo "Pageviews=". $_SESSION['views']; ?>
Isset: Determines whether a variable has been set.
unset(): The function is used to release the specified session variable
The session_destroy() function completely terminates session
PHP mail() function
The PHP mail() function is used to send e-mail messages from scripts
The easiest way is to send a text email
<?php $to = "someone@example.com"; $subject = "Test mail"; $message = "Hello! This is a simple email message."; $from = "someonelse@example.com"; $headers = "From: $from"; mail($to,$subject,$message,$headers); echo "Mail Sent."; ?>
mailform.php
<?php if (isset($_REQUEST['email'])) { $email = $_REQUEST['email'] ; $subject = $_REQUEST['subject'] ; $message = $_REQUEST['message'] ; mail( "someone@example.com", "Subject: $subject", $message, "From: $email" ); echo "Thank you for using our mail form"; } else { echo "<form method='post' action='mailform.php'> Email: <input name='email' type='text' /><br /> Subject: <input name='subject' type='text' /><br /> Message:<br /> <textarea name='message' rows='15' cols='40'> </textarea><br /> <input type='submit' /> </form>"; } ?>
PHP exception handling
Actively throw exception:
throw exception object;
Catch Exceptions
try { This is a statement that may cause an exception. } catch(Exception $e) { Exception handling statement; }
<?php foreach($_COOKIE as $key=>$value){ setCookie($key,"",time()-60); } echo "Delete All cookie!";
End of php Advanced Tutorial!
afterword
Okay, please leave a message in the message area to share your experience and experience.
Thank you for learning today. If you find this article helpful, you are welcome to share it with more friends. Thank you.
Thank!Thank you!Your sincere appreciation is my greatest motivation to move forward!
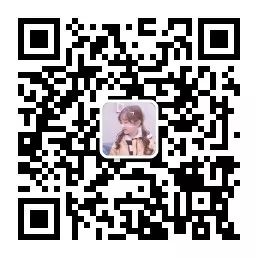
