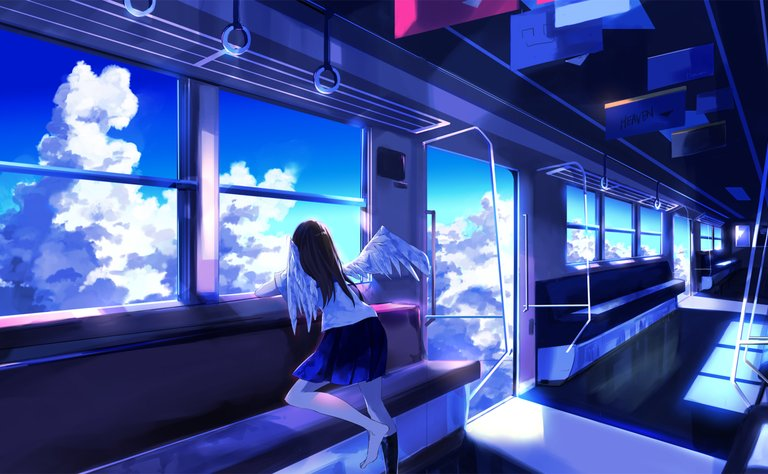
php constants, constants can not be changed, consisting of letters, underscores, and numbers, but numbers can not appear as initials.
bool define ( string $name , mixed $value [, bool $case_insensitive = false ] )
<?php // Case-sensitive constant names define("44", "Welcome to 444"); echo 44; echo '<br>'; echo 55; ?>
<?php // Case-insensitive constant names define("Da", "Welcome to dashu", true); echo da; ?>
String variables are used to store and process text, contain character values, create, use, and store in variables.
<?php $txt="Hello world!"; echo $txt; ?>
Operator (.) is used to connect two string values
strlen() function returns the length of the string
<?php echo strlen("Hello world!"); ?>
The Strpos() function is used to find a character or a specified text in a string.
<?php echo strpos("Hello world!","world"); ?>
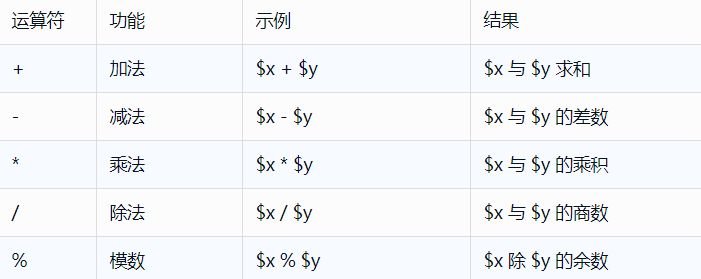

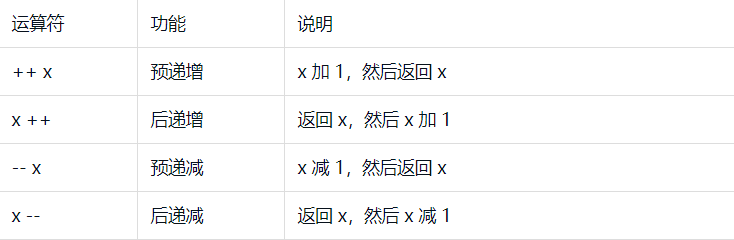
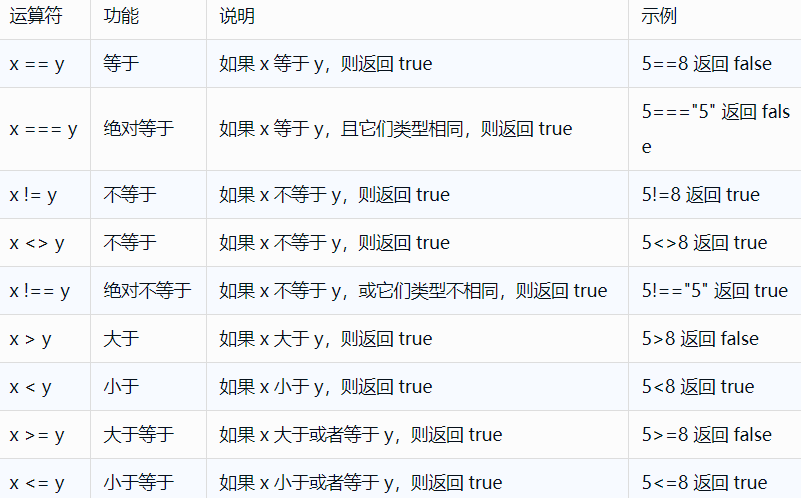
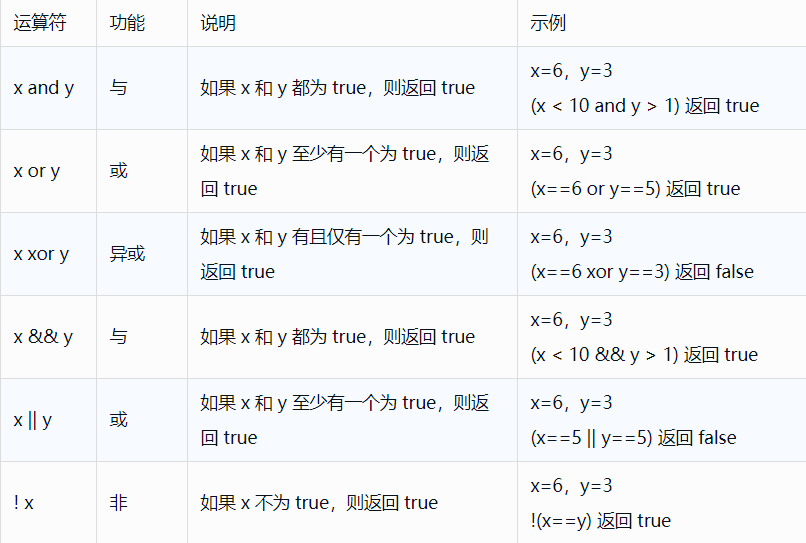
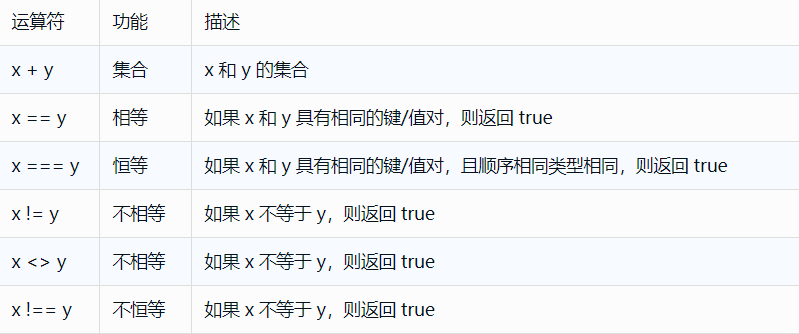
Ternary operator
(expr1) ? (expr2) : (expr3)
PHP conditional statement
if (condition) { //The code to be executed when the condition is established; } if (condition) { //The code executed when the condition is established; } else { //Code executed when conditions are not established; } if (condition) { if Code executed when the condition is set; } elseif (condition) { elseif Code executed when the condition is set; } else { //Code executed when conditions are not established; } <?php switch (n) { case label1: break; case label2: break; default: } ?>
PHP cycle
while (The condition is true.) { } do { } while (The condition is true.); for (initial value; condition; increment) { } foreach ($array as $value) { }
PHP function
<?php function functionName() { // Code to execute } ?>
PHP default parameter values
<?php function aa($aaa=50) { echo "The is : $aaa<br>"; } aa(); // The default value 50 will be used ?>
PHP function return value
<?php function add($x,$y) { $total=$x+$y; return $total; } echo "1 + 5= " . add(1,5); ?>
PHP array
array(); $name=array("a","b","c");
Get the length of the array
<?php $name=array("a","b","c"); echo count($name); ?>
Traversing index arrays
<?php $name=array("a","b","c"); $arrlength=count($name); for($x=0;$x<$arrlength;$x++) { echo $name[$x]; echo "<br>"; } ?>
Traversing associative arrays
<?php $age=array("a"=>"aa","b"=>"bb","c"=>"cc"); foreach($age as $x=>$x_value){ echo "Key=" . $x . ", Value=" . $x_value; echo "<br>"; } ?>
<?php $aaa = array ( "course"=>array ( "c", "https://a/list" ), "class"=>array ( "b", "https://a.com" ), "coding"=>array ( "a", "https://aa.com" ) ); print("<pre>"); // Formatting output arrays print_r($aaa); print("</pre>"); ?>
PHP Array Sorting
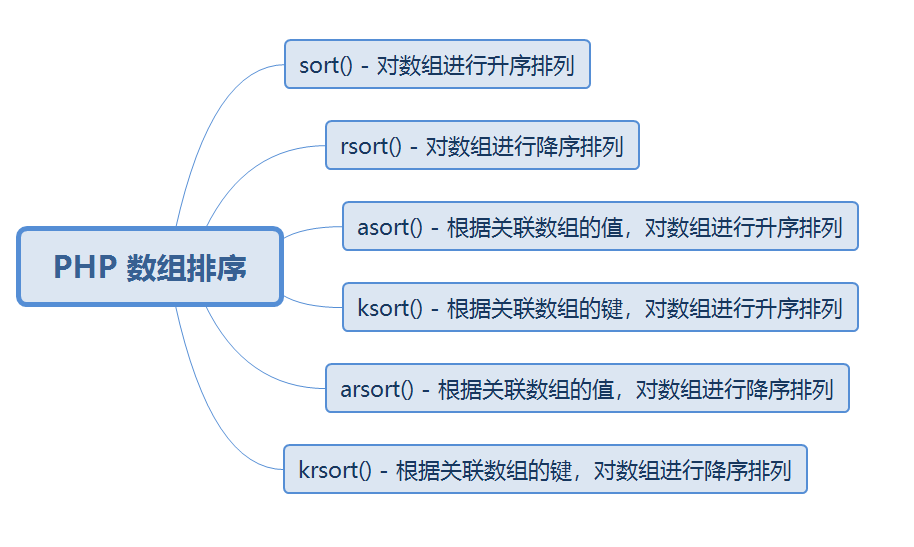
PHP Global Variables

// GLOBALS global variable <?php $x = 2; $y = 3; function add() { $GLOBALS['z'] = $GLOBALS['x'] + $GLOBALS['y']; } add(); echo $z; ?>
Global variable $_SERVER
$_REQUEST is used to collect data submitted by HTML forms
Global variable $_POST
Global variable $_GET
PHP form, _ POST is used to collect form data
PHP drop-down menu
<?php $q = isset($_GET['q'])? htmlspecialchars($_GET['q']) : ''; ?>
PHP drop-down menu multiple selection (multiple= "multiple")
PHP form validation
The htmlspecialchars() function converts special characters into HTML entities
<script>location.href('http://www.aaa.com')</script>
PHP Date Function
string date ( string $format [, int $timestamp ] )
date() formatted date
d -- represents the day in the month (01-31), m -- represents the month (01-12), Y -- represents the year (four digits), and 1 -- represents a day in the week.
Get time zone
<?php date_default_timezone_set("Asia/Shanghai"); echo "current time is " . date("h:i:sa"); ?>
The readfile() function reads the file and writes it to the input buffer
The first parameter of the fopen() function contains the name of the opened file, and the second parameter specifies the mode of opening the file.
The fread() function reads open files
fclose() function is used to close open files
The fgets() function is used to read a single line from a file
The fgetc() function is used to read a single character from a file
The fopen() function is also used to create files
The fwrite() function is used to write files
Create a file upload form
<html> <body> <form action="upload_file.php" method="post" enctype="multipart/form-data"> <label for="file">Filename:</label> <input type="file" name="file" id="file" /> <br /> <input type="submit" name="submit" value="Submit" /> </form> </body> </html>
Create upload scripts
<?php if ($_FILES["file"]["error"] > 0) { echo "Error: " . $_FILES["file"]["error"] . "<br />"; } else { echo "Upload: " . $_FILES["file"]["name"] . "<br />"; echo "Type: " . $_FILES["file"]["type"] . "<br />"; echo "Size: " . ($_FILES["file"]["size"] / 1024) . " Kb<br />"; echo "Stored in: " . $_FILES["file"]["tmp_name"]; } ?>
$_FILES["file"]["name"]
Name of uploaded file
$_FILES["file"]["type"]
Types of uploaded files
$_FILES["file"]["size"]
The size of the uploaded file in bytes
$_FILES["file"]["tmp_name"]
The name of the temporary copy of the file stored on the server
$_FILES["file"]["error"]
Error code transmitted from file
Upload restriction
<?php if ((($_FILES["file"]["type"] == "image/gif") || ($_FILES["file"]["type"] == "image/jpeg") || ($_FILES["file"]["type"] == "image/pjpeg")) && ($_FILES["file"]["size"] < 20000)) { if ($_FILES["file"]["error"] > 0) { echo "Error: " . $_FILES["file"]["error"] . "<br />"; } else { echo "Upload: " . $_FILES["file"]["name"] . "<br />"; echo "Type: " . $_FILES["file"]["type"] . "<br />"; echo "Size: " . ($_FILES["file"]["size"] / 1024) . " Kb<br />"; echo "Stored in: " . $_FILES["file"]["tmp_name"]; } } else { echo "Invalid file"; } ?>
Save uploaded files
<?php if ((($_FILES["file"]["type"] == "image/gif") || ($_FILES["file"]["type"] == "image/jpeg") || ($_FILES["file"]["type"] == "image/pjpeg")) && ($_FILES["file"]["size"] < 20000)) { if ($_FILES["file"]["error"] > 0) { echo "Return Code: " . $_FILES["file"]["error"] . "<br />"; } else { echo "Upload: " . $_FILES["file"]["name"] . "<br />"; echo "Type: " . $_FILES["file"]["type"] . "<br />"; echo "Size: " . ($_FILES["file"]["size"] / 1024) . " Kb<br />"; echo "Temp file: " . $_FILES["file"]["tmp_name"] . "<br />"; if (file_exists("upload/" . $_FILES["file"]["name"])) { echo $_FILES["file"]["name"] . " already exists. "; } else { move_uploaded_file($_FILES["file"]["tmp_name"], "upload/" . $_FILES["file"]["name"]); echo "Stored in: " . "upload/" . $_FILES["file"]["name"]; } } } else { echo "Invalid file"; } ?>
PHP cookie
Cookie s are often used to identify users
setcookie(name, value, expire, path, domain);
<?php setcookie("user", "dashucoding", time()+3600); ?> <html> <body> </body> </html>
The $_COOKIE variable is used to retrieve the value of the cookie
<?php //Output cookie value echo $_COOKIE["user"]; //View all cookie s print_r($_COOKIE); ?>
Use the isset() function to confirm that cookie s are set
<html> <body> <?php if (isset($_COOKIE["user"])) echo "Welcome " . $_COOKIE["user"] . "!<br />"; else echo "Welcome!<br />"; ?> </body> </html>
delete cookie
<?php // Set cookie expiration time to last 1 hour setcookie("user", "", time()-3600); ?>
Browsers do not support cookie s
Transfer information from one page to another in an application
<html> <body> <form action="welcome.php" method="post"> //Name: <input type="text" name="name"/> //Age: <input type="text" name="age"/> <input type="submit" /> </form> </body> </html>
<html> <body> Welcome <?php echo $_POST["name"]; ?>.<br /> You are <?php echo $_POST["age"]; ?> years old. </body> </html>
The PHP session variable is used to store information about user sessions or to change user session settings
Working mechanism
Create a unique id (UID) for each visitor and store variables based on the UID. UIDs are stored in cookie s or transmitted through URL s.
<?php session_start(); ?> <html> <body> </body> </html>
Store session variables
<?php session_start(); // Store session data $_SESSION['add']=1; ?> <html> <body> <?php //Retrieving session data echo "Browse volume=". $_SESSION['add']; ?> </body> </html>
<?php session_start(); if(isset($_SESSION['views'])){ $_SESSION['views']=$_SESSION['views']+1;} else{ $_SESSION['views']=1;} echo "Browse:". $_SESSION['views']; ?>
unset() function is used to release the specified session variable
session_destroy() completely destroys session
<?php unset($_SESSION['views']); ?> <?php session_destroy(); ?>
The mail() function is used to send e-mail from scripts
mail(to,subject,message,headers,parameters)
<?php $to = "dashucoding@qq.com"; // Mail Receiver $subject = "Parameter mail"; // Mail title $message = "This is the content of the mail."; // Body $from = "dada@qq.com"; // Email Sender $headers = "From:" . $from; // Header Information Settings mail($to,$subject,$message,$headers); echo "The mail has been sent"; ?>
<html> <body> <?php if (isset($_REQUEST['email'])) //Send mail if you receive mailbox parameters { //Send mail $email = $_REQUEST['email'] ; $subject = $_REQUEST['subject'] ; $message = $_REQUEST['message'] ; mail( "someone@example.com", "Subject: $subject", $message, "From: $email" ); echo "Successful mail delivery"; } else //Display the form if there are no mailbox parameters { echo "<form method='post' action='mailform.php'> Email: <input name='email' type='text' /><br /> Subject: <input name='subject' type='text' /><br /> Message:<br /> <textarea name='message' rows='15' cols='40'> </textarea><br /> <input type='submit' /> </form>"; } ?> </body> </html> // Problematic code
The best way to prevent email injection is to validate the input
<html> <body> <?php function spamcheck($field) { // filter_var() filters e-mail // Using FILTER_SANITIZE_EMAIL $field=filter_var($field, FILTER_SANITIZE_EMAIL); //filter_var() filters e-mail // Using FILTER_VALIDATE_EMAIL if(filter_var($field, FILTER_VALIDATE_EMAIL)) { return TRUE; } else { return FALSE; } } if (isset($_REQUEST['email'])) { // Send mail if you receive mailbox parameters // Judging the Legality of Mailboxes $mailcheck = spamcheck($_REQUEST['email']); if ($mailcheck==FALSE) { echo "illegal input"; } else {//Send mail $email = $_REQUEST['email'] ; $subject = $_REQUEST['subject'] ; $message = $_REQUEST['message'] ; mail("someone@example.com", "Subject: $subject", $message, "From: $email" ); echo "Thank you for using our mail form"; } } else { // Display the form without mailbox parameters echo "<form method='post' action='mailform.php'> Email: <input name='email' type='text' /><br /> Subject: <input name='subject' type='text' /><br /> Message:<br /> <textarea name='message' rows='15' cols='40'> </textarea><br /> <input type='submit' /> </form>"; } ?> </body> </html>
PHP error
<?php if(!file_exists("da.txt")){ die("file does not exist"); }else{ $file=fopen("da.txt","r"); } ?>
afterword
Well, welcome to leave a message in the message area, and share your experience and experience with you.
Thank you for learning today's content. If you think this article is helpful to you, you are welcome to share it with more friends. Thank you.
Thank! Thank you for your kindness! Your sincere appreciation is the greatest driving force for my progress!
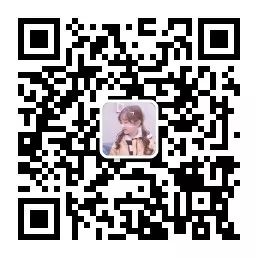
