Android Design Support Library Series First bullet, suspension button
Floating action button (FAB) is simply a circular button, which is suspended on the UI and surrounded by shadows. It can be used to implement some advanced operations, such as adding new entries or attachments to mail, etc.
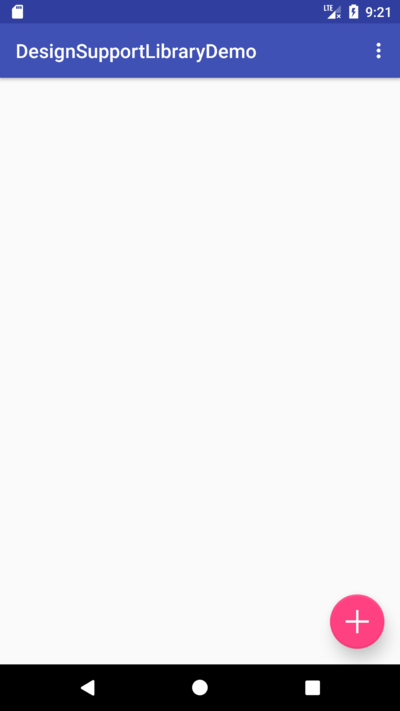
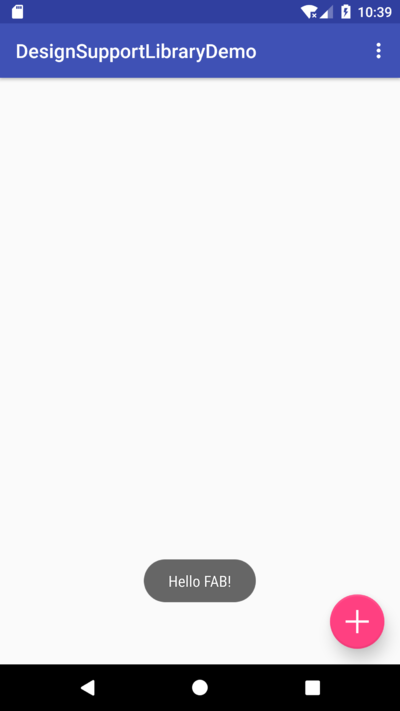
In essence, FAB is a control inherited from ImageView, which supports all the properties of ImageView and ImageButton.
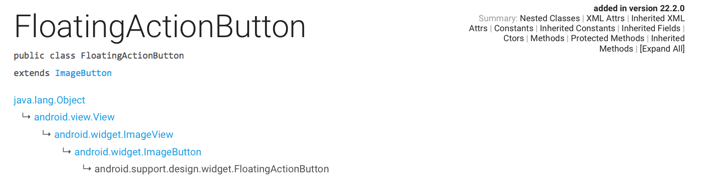
To use FAB, first add dependency packages:
//At the time of writing, the latest version was 25.3.1. compile 'com.android.support:design:25.3.1'
Step one:
By adding FAB to the layout file, we assume that we have declared the namespace xmlns:app=" http://schemas.android.com/apk/res-auto.
<FrameLayout android:id="@+id/layoutInner" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.design.widget.FloatingActionButton android:id="@+id/btnFloatingAction" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="bottom|right" /> </FrameLayout>
It should be noted that because FAB is often suspended in the entire UI interface, it is usually placed in a single FrameLayout, which will cover the UI interface below, so that the location of FAB can be easily set.
Introduction to FAB Attributes
- Background fill color: By default, FAB fill color is defined by color Accent in style.xml. If necessary, attribute ** app:backgroundTint ** can be set to customize background fill color.
- Size: FAB has three sizes: Normal 56dp, Mini 40dp and adaptive size (based on screen size). Users can set it by app:fabSize.fabSize
- Ripple color: app:rippleColor attribute is used to control the color that produces ripple effect when FAB is pressed.
- Shadow size: app:elevation attribute is used to control the size of FAB shadow in normal state, default 6dp;
- Press Shadow: app:pressedTranslationZ property is used to control the FAB's shadowing when press, default 12dp;
The second step
According to the introduction of attributes, improve the FAB settings:
android:id="@+id/btnFloatingAction" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="bottom|right" android:src="@drawable/ic_plus" app:fabSize="normal" app:rippleColor="@android:color/background_dark"/>
The third step
Set the click event in Activity:
FloatingActionButton btnFab = (FloatingActionButton) findViewById(R.id.btnFloatingAction); btnFab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(MainActivity.this, "Hello FAB!", Toast.LENGTH_SHORT).show(); } });
The settings of Button click events are the same as those of general Button clicks, which are very useful.
Issues needing attention
The effect of FAB rendering is not the same on Android 4.4 and devices over 4.4:
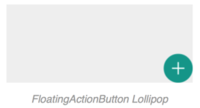
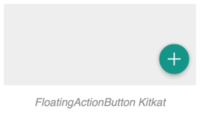
As can be seen from the comparison chart above, the FAB on Android 4.4 has margins and default shadows, but the 4.4 + device has no borders and devices.
Resolving margins
For the end of the border problem, you can set the border distance for 4.4 + devices, while for 4.4 and previous devices, the border distance is set to 0.
- values/dimens.xml
<dimen name="fab_margin_right">0dp</dimen> <dimen name="fab_margin_bottom">0dp</dimen>
- values-v21/dimens.xml
<dimen name="fab_margin_right">16dp</dimen> <dimen name="fab_margin_bottom">16dp</dimen>
In the layout file, you can set the margin of FAB in this way.
<android.support.design.widget.FloatingActionButton ...... ...... android:layout_marginBottom="@dimen/fab_margin_bottom" android:layout_marginRight="@dimen/fab_margin_right"/>
In this way, the device will select the margin value that matches the system version to set it.
Resolving shadows
In order to unify the size of shadows, the app:borderWidth property can be used in the layout file to unify the size of shadows.
<android.support.design.widget.FloatingActionButton ...... ...... app:fabSize="normal" app:borderWidth="0dp" android:layout_marginBottom="@dimen/fab_margin_bottom" android:layout_marginRight="@dimen/fab_margin_right"/>
Animation problem
At first, Rotate Animation could not be applied to FAB, but the problem was solved after attribute animation appeared.
btnFab = (FloatingActionButton) findViewById(R.id.btnFloatingAction); btnFab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(FloatingActionButtonActivity.this, "Hello FAB!", Toast.LENGTH_SHORT).show(); //Set Properties Animation, Rotate ObjectAnimator animator = ObjectAnimator.ofFloat(btnFab,"rotation",0f,360f); animator.setInterpolator(new FastOutLinearInInterpolator()); animator.setDuration(1000).start(); } });
For more information on attribute animation pairs, please read Here
Related reference
https://developer.android.com/reference/android/support/design/widget/FloatingActionButton.html