This article is mainly about the binary de edge and de line noise reduction of verification code pictures.
It's hot recently. People are also a little tired and don't type too many words.
First install opencv: (click the link to view) https://blog.csdn.net/weixin_43582101/article/details/88660570
I drew a picture myself (the picture data below is written according to this picture) Picture name: 1234567.png:
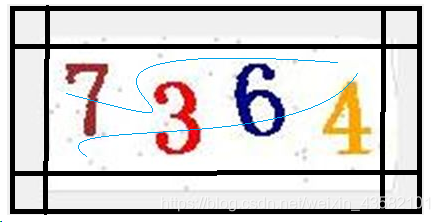
Read in picture 1234567.png
import cv2 im = cv2.imread('1234567.png')
Use cvtColor method to convert color space into black and white
im = cv2.cvtColor(im, cv2.COLOR_BGR2GRAY)
You can save it to see:
cv2.imwrite('33333.png',im)
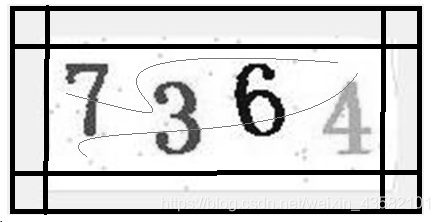
Then we remove the border first: What principle is to change the pixels of all coordinates in the border range into 255 white. This depends on the border value of your picture. It depends on the situation.
def clear_border(img): h, w = img.shape[:2] # h height, w width for y in range(0, w): for x in range(0, h): if y < 50 or y > w - 61: # Turn the pixel coordinates [0,0] to [height, 50] and [0, width - 50] to [height, width] within 50 into white img[x, y] = 255 if x < 60 or x > h - 60: # Above img[x, y] = 255 cv2.imwrite('22222.png',img) return img
Look at 22222.png:
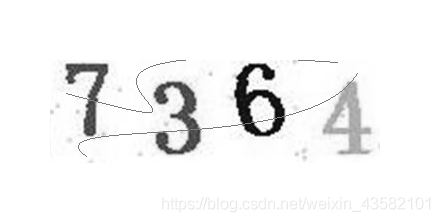
The frame is gone.
Now let's remove the offline: that is, noise reduction of interference line
This principle is to judge whether the pixel is white. If it indicates that it is an interference line, it will also turn it white.
def interference_line(img): h, w = img.shape[:2] # opencv matrix points are inverse # img[1,2] 1: height of picture, 2: width of picture for y in range(1, w - 1): for x in range(1, h - 1): count = 0 if img[x, y - 1] > 245: count = count + 1 if img[x, y + 1] > 245: count = count + 1 if img[x - 1, y] > 245: count = count + 1 if img[x + 1, y] > 245: count = count + 1 if count > 2: img[x, y] = 255 #Judge how much white there is in a circle. If it exceeds 2, it will turn white. cv2.imwrite('44444.png',img) return img
Take a look at 44444.png:
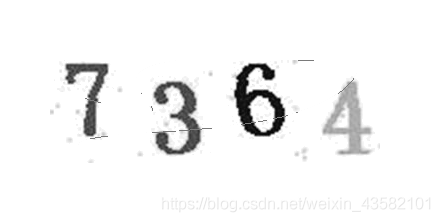
The line is almost gone. But there are some interference points or something.
Point noise reduction:
If you want to reduce noise, you can write it according to the situation, or you can use the encapsulated method.
Median filter medianBlur:
The basic idea of this median filter is to replace the gray value of the pixel with the median of the gray value in the neighborhood of the pixel, so that the surrounding pixel values are close to the real value, so as to eliminate the isolated noise points.
import numpy image = cv2.imread('44444.png') result = numpy.array(image) ss = cv2.medianBlur(result,5) cv2.imwrite('66666.png',ss)
Take a look at 66666.png
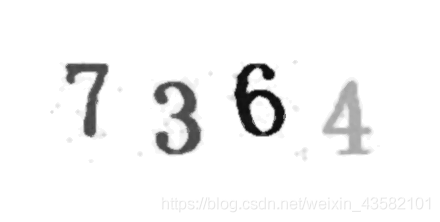
Is it much better. You can also call this method again.
Gaussian filter: Gaussian blur function:
Gaussian filtering needs to pay more attention to the pixels around a pixel. Therefore, the values of these surrounding points can be recalculated by assigning weights. This can be solved by the weight scheme of Gaussian function (bell function, i.e. horn number)
# Replace each pixel with the average of the pixels around that pixel image1 = cv2.imread('66666.png') result = cv2.blur(image1,(5,5)) gaussianResult = cv2.GaussianBlur(result,(5,5),1.5) cv2.imwrite('77777.png',gaussianResult)
Look at 77777.png again
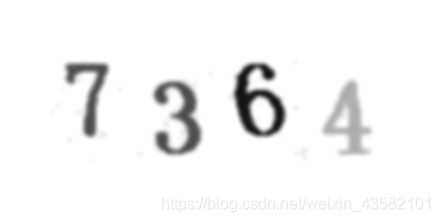
All right, let's get here first. In fact, there are not many things. If you want to study the principle of noise reduction, you can contact me for further study.
Complete process code:
import cv2 import numpy def cvt(img): img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) return img def clear_border(img): h, w = img.shape[:2] # h height, w width for y in range(0, w): for x in range(0, h): if y < 50 or y > w - 61: # Turn the pixel coordinates [0,0] to [height, 50] and [0, width - 50] to [height, width] within 50 into white img[x, y] = 255 if x < 60 or x > h - 60: # Above img[x, y] = 255 cv2.imwrite('22222.png',img) return img def interference_line(img): h, w = img.shape[:2] # opencv matrix points are inverse # img[1,2] 1: height of picture, 2: width of picture for y in range(1, w - 1): for x in range(1, h - 1): count = 0 if img[x, y - 1] > 245: count = count + 1 if img[x, y + 1] > 245: count = count + 1 if img[x - 1, y] > 245: count = count + 1 if img[x + 1, y] > 245: count = count + 1 if count > 2: img[x, y] = 255 #If you judge that a circle is white, it turns white. return img def dian_medianBlur(image): result = numpy.array(image) image = cv2.medianBlur(result,5) return image def dian_GaussianBlur(image): result = cv2.blur(image,(5,5)) gaussianResult = cv2.GaussianBlur(result,(5,5),1.5) return gaussianResult if __name__ == '__main__': img = cv2.imread('1234567.png') image = dian_GaussianBlur(dian_medianBlur(interference_line(clear_border(cvt(img))))) cv2.imwrite('88888.png',image)