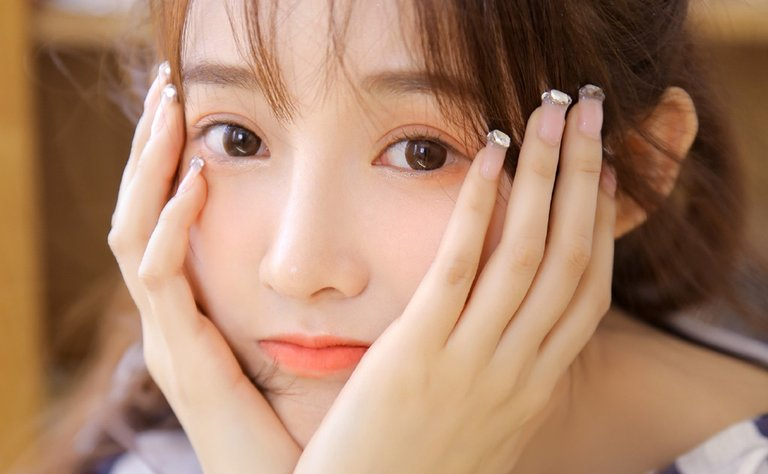
CSS helper
Added advanced functions such as variable, nesting, mixing, import, etc.
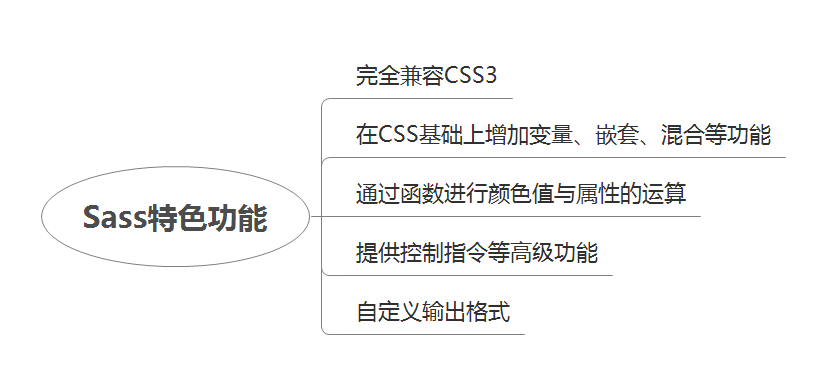
# Convert Sass to SCSS $ sass-convert style.sass style.scss # Convert SCSS to Sass $ sass-convert style.scss style.sass
Using variables
Variable declaration
Sass variable declaration is similar to CSS property declaration:
$highlight-color: #F90;
$nav-color: #F90; nav { $width: 100px; width: $width; color: $nav-color; } //After compilation nav { width: 100px; color: #F90; }
Variable reference
$highlight-color: #F90; .selected { border: 1px solid $highlight-color; } //After compilation .selected { border: 1px solid #F90; }
$highlight-color: #F90; $highlight-border: 1px solid $highlight-color; .selected { border: $highlight-border; } //After compilation .selected { border: 1px solid #F90; }
$link-color: blue; a { color: $link_color; } //After compilation a { color: blue; }
Nested CSS rules
#content article h1 { color: #333 } #content article p { margin-bottom: 1.4em } #content aside { background-color: #EEE }
#content { article { h1 { color: #333 } p { margin-bottom: 1.4em } } aside { background-color: #EEE } } /* After compilation */ #content article h1 { color: #333 } #content article p { margin-bottom: 1.4em } #content aside { background-color: #EEE }
Parent selector identifier&
sass will not work properly in the following situations:
article a { color: blue; :hover { color: red } }
article a { color: blue; &:hover { color: red } }
Nesting of group selectors
.button, button { margin: 0; } .container h1, .container h2, .container h3 { margin-bottom: .8em } .container { h1, h2, h3 {margin-bottom: .8em} }
article { ~ article { border-top: 1px dashed #ccc } > section { background: #eee } dl > { dt { color: #333 } dd { color: #555 } } nav + & { margin-top: 0 } }
SASS import SASS file
Sass's @ import rule imports related files when generating css files
$link-color: blue; $link-color: red; a { color: $link-color; } .blue-theme {@import "blue-theme"} //The result is exactly the same as writing the file "blue theme. SCSS" directly in the. Blue theme selector. .blue-theme { aside { background: blue; color: #fff; }
/*Comment syntax for... * /
Sass mixer
Mixer is defined with @ mixin identifier
@mixin rounded-corners { -moz-border-radius: 5px; -webkit-border-radius: 5px; border-radius: 5px; } notice { background-color: green; border: 2px solid #00aa00; @include rounded-corners; } //sass final generation: .notice { background-color: green; border: 2px solid #00aa00; -moz-border-radius: 5px; -webkit-border-radius: 5px; border-radius: 5px; }
Place @ include where it is called
Selector inheritance
Through @ extend syntax
//Inherit styles through selectors .error { border: 1px solid red; background-color: #fdd; } .seriousError { @extend .error; border-width: 3px; }
Using sass to write clear, non redundant, semantic css
Please praise! Because your approval / encouragement is the biggest driving force of my writing!
Welcome to your attention. Da Shu Xiao Sheng The short book!
This is a blog with quality and attitude
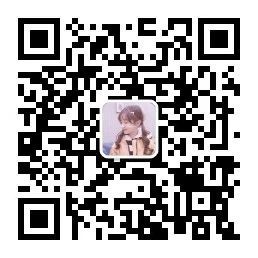