1. Definition and invocation of functions
How 1.1 functions are defined
1. Function Declarations function Keyword (named function)
2. Function expression (anonymous function)
3. new Function()
var fn=new Function('Parameter 1', 'Parameter 2', 'Parameter 3'..., 'Function Body')
- Function Inside parameters must be in string format
- The third method is inefficient and not easy to write, so it is less used
- All functions are Function Instances (Objects)
- Functions also belong to objects
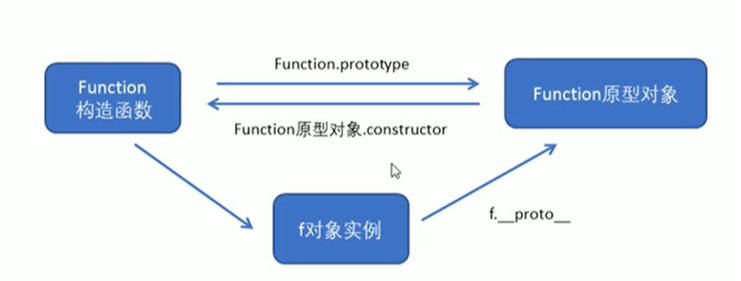
<body>
<script>
// Function Definition
// 1. Custom functions (named functions)
function fn(){};
// 2. Function expressions (anonymous functions)
var fun=function(){};
// 3. Use new Function('Parameter 1','Parameter 2','Function Body')
var f=new Function('a','b','console.log(a+b)');
f(1,2);
// 4. All functions are instances of Function (objects)
console.dir(f);
console.log(f instanceof Object);
</script>
</body>
Call method of 1.2 function
1. General function
2. Method of object
3. Constructor
4. Bind Event Function
5. Timer Functions
6. Execute function immediately
<body>
<script>
// Call method of function
// 1. General functions
function fn(){
console.log('Peak of life!!!!!!');
}
fn();
fn.call();
// 2. Methods of Objects
var o={
sayHi:function(){
console.log('Peak of life!!!!');
}
}
o.sayHi();
// 3. Constructors
function Star(){};
new Star();
// 4. Binding event functions
btn.onclik=function(){}; // You can call this function by clicking the button
// 5. Timer function
setInterval(function(){},1000); // This function is called automatically once a second by the timer
// 6. Execute the function immediately
(function(){
console.log('Peak of life!!!!');
})();
// Immediate execution of a function is called automatically
</script>
</body>
2. this
Direction of this within 2.1 function
These ones here this The direction is determined when we call a function, and how we call it depends this The directions are different.
Usually pointing to our caller.
Call Method | this points to |
---|
Common function calls | window |
constructor call | Method inside the instance object prototype object also points to the instance object |
Object Method Call | Object to which the method belongs |
Event Binding Method | Bind Event Object |
Timer Functions | window |
Execute function immediately | window |
<body>
<button>click</button>
<script>
// Different ways of calling functions determine the direction of this
// 1. Common function this points to window
function fn(){
console.log('Of ordinary functions this'+this);
}
window.fn();
// 2. Object's method this points to object o
var o={
sayHi:function(){
console.log('Object method this: '+this);
}
}
o.sayHi();
// 3. The constructor this points to the ldh instance object, and the this inside the prototype object also points to the ldh instance object
function Star(){};
Star.prototype.sing=function(){
}
var ldh=new Star();
// 4. The bound event function this points to the button object btn, the caller of the function
var btn=document.querySelector('button');
btn.onclick=function(){
console.log('Bound to event functions this: '+this);
};
// 5. Timer function this also points to window
window.setTimeout(function(){
console.log('Timer's this: '+this);
},1000);
// 6. Execute function this immediately or point to window
(function(){
console.log('Execute the function immediately this: '+this);
})();
</script>
</body>
javascript There are some function methods specifically provided to help us handle function interiors more elegantly this Directional problems, often with bind(),
call(), apply()Three methods.
2.1.1 call() method
call() Method calls an object.Simply understood as the way a function is called, but it can change its this Point.
fun.call(thisArg, arg1, arg2,...)
<body>
<script>
// To change this point within a function, js provides three methods: call() apply() bind()
// 1. call()
var o={
name:'andy'
}
function fn(a,b){
console.log(this);
console.log(a+b);
}
fn.call(o,1,2);
// call() The first can call a function, and the second can change the this point within the function
// The primary role of call() is to implement inheritance
function Father(uname,age,sex){
this.uname=uname;
this.age=age;
this.sex=sex;
}
function Son(uname,age,sex){
Father.call(this,uname,age,sex);
}
var son=new Son('Lau Andy',18,'male');
console.log(son);
</script>
</body>
2.1.2 apply() method
apply() Method calls a function.Simply understood as the way a function is called, but he can change the function's this Point.
fun.apply(thisArg, [argsArray])
- thisArg: stay fun Specified when the function runs this value
- argsArray: The value passed must be included in the array
- The return value is the return value of the function because it is the calling function
<body>
<script>
// To change this point within a function, js provides three methods: call() apply() bind()
// 2. apply() application, meaning of application
var o={
name:'andy'
};
function fn(arr){
console.log(this);
console.log(arr); // 'pink'
};
fn.apply(o,['pink']);
// 1. It is also a call to a function, the second can change the this point inside the function
// 2. But his argument must be an array (pseudo array)
// 3. The main application of apply (): for example, we can use apply to maximize with the help of built-in objects in Teaching
// Math.max();
var arr=[1,66,33,55,88];
var arr1=['red','blue'];
var max=Math.max.apply(Math,arr);
var min=Math.min.apply(Math,arr);
console.log(max,min);
</script>
</body>
2.1.3 bind() method
bind() Method does not call a function.But it can change the inside of the function this Point.
fun.bind(thisArg, arg1, arg2,...)
- thisArg: stay fun Specified when the function runs this value
- arg1, arg2: Other parameters passed
- Returns the specified this Copy of original function modified by value and initialization parameters
<body>
<button>click</button>
<button>click</button>
<button>click</button>
<button>click</button>
<script>
// To change this point within a function, js provides three methods: call() apply() bind()
// 2. bind() binding, meaning of binding
var o={
name:'andy'
};
function fn(a,b){
console.log(this);
console.log(a+b);
};
var f=fn.bind(o,1,2);
f();
// 1. Instead of calling the original function, you can change the this point inside the function
// 2. Returns the new function that is generated when the original function changes this
// 3. If there is a function we don't need to call it immediately, but we want to change the this inside the function to point to bind at this time.
// 4. We have a button that will be disabled when we click it and then turned on after 3 seconds
// var btn=document.querySelector('button');
// btn.οnclick=function(){
// this.disabled=true; //This this points to the btn button
// // var that=this;
// setTimeout(function(){
// //that.disabled=false; //this inside the timer function points to window
// this.disabled=false; //The this inside the timer function now points to btn
// }.bind(this), 3000;//This this points to the btn object
// }
var btns=document.querySelectorAll('button');
for(var i=0;i<btns.length;i++){
btns[i].onclick=function(){
this.disabled=true;
setTimeout(function(){
this.disabled=false;
}.bind(this),2000);
}
}
</script>
</body>
Summary of call() apply() bind()
Same:
Can change the internal this point
The difference:
1. call and apply Will call the function and change the this Point.
2. call and apply The parameters passed are different, call Delivery Interpretation arg1,arg2...Form, apply Must be array form[arg]
3. bind Function is not called, function interior can be changed this Point.
Main scenarios:
1. call Inherit often.
2. apply Often related to arrays.. For example, using a mathematical object to achieve the minimum and maximum values of an array
3. bind Function is not called, but it can be flipped this Point to, for example, change the inside of the timer this Point.
3. Strict Mode
3.1 What is a strict model
javascript In addition to providing normal mode, strict mode is also provided(stict mode). ES5 The strict pattern is to use restrictive
javascript One way of doing this is under strict conditions JS Code.
Strict mode IE10 These browsers will only be supported and will be ignored in older browsers.
Strict mode pairs normal javascript Some semantic changes have been made:
1. Eliminated javascript Some improper and inaccurate grammar reduces some strange behavior.
2. Eliminate some unsafe aspects of code operation to ensure code security.
3. Increase compiler efficiency and speed.
4. Disabled in ECMAScript Some grammars that may be defined in future versions of javascript Do a good job of paving.
For example, some reserved words such as: class, enum, export, extends, import, super Cannot make variable name
3.2 Turn on strict mode
Strict mode can be applied to the entire script or to individual functions, so when using it, we can classify the strict mode into two cases: opening strict mode for scripts and opening strict mode for functions.
3.2.1 Turn on strict mode for scripts
To turn on strict mode for the entire script, you need to put a specific statement before all the statements" use strict";(or 'use strict').
<script>
"use strict";
console.log("This is a strict mode.");
</script>
because"use strict"Quoted, so older browsers would ignore him as a normal line of string.
<body>
<!--For the entire script(Script Label)Turn on strict mode-->
<script>
'use strict';
</script>
<script>
(function(){
'use strict';
})();
</script>
</body>
3.2.2 Turn on strict mode for functions
To turn on strict mode for a function, you need to " use strict";(or'use strict';)Declarations precede all statements in the body.
<body>
<!--Turn on strict mode for a function-->
<script>
// Just turn on strict mode for the fn function at this point
function fn(){
'use strict';
// The following code executes in strict mode
}
function fun(){
// The code inside executes in normal mode
}
</script>
</body>
3.4 Changes in Strict Mode
Strict pattern pairs javascript Has made some changes in its grammar and behavior.
3.4.1 Variable Provisions
1. In normal mode, if a variable is assigned without a declaration, the default is a global variable.Strict mode prohibits this use.
Variables must be used first var Command declaration before use.
2. Deleting declared variables is strictly prohibited.For example: delete x; The grammar is wrong.
<body>
<script>
'use strict';
// 1. Our variable names must be declared before they can be used
// num=10;
// console.log(num);
var num = 10;
console.log(num);
// 2. We cannot delete declared variables at will
delete num; // Errors will occur
</script>
</body>
this Pointing Problem in 3.4.2 Strict Mode
1. Previously in Global Scope Functions this point window Object.
2. In strict mode, in functions in global scope this yes undefined.
3. Previous constructors are not added new It can also be called when a normal function, this Points to a global object.
4. In strict mode, if the constructor is not added new Called, this Errors will be reported.
5. new The instantiated constructor points to the object instance created.
6. timer this Or point to window.
7. Event, object or point to caller.
<body>
<script>
'use strict';
// 3. this is undefined in the global scope function in strict mode
function fn(){
console.log(this); // undefined
}
fn();
// 4. In strict mode, this will error if the constructor is not called with a new call
function Star(){
this.sex='male';
}
// Star();
var ldh=new Star();
console.log(ldh.sex);
// 5. Timer this or pointing to window
setTimeout(function(){
console.log(this);
},2000);
</script>
</body>
3.4.3 Functional Variation
1. Functions cannot have parameters with duplicate names.
2. Functions must be declared in a new version at the top level javascript Will introduce a block-level scope ( ES6 Has been introduced).In order to keep up with the new version,
Declaring a function in the code block of a non-function is not allowed.
More stringent mode requirements refer to: https://developwe.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Strict_mode
// 6. Duplicate names are not allowed for parameters inside functions in strict mode
// function fn(a,a){
// console.log(a+a);
// }
// fn(1,2); //Errors will occur
4. Higher Order Functions
A higher-order function is a function that operates on other functions, receiving functions as parameters or outputting functions as return values.
<script>
function fn(callback){
callback&&callback();
}
fn(function(){alert('hi')});
</script>
<script>
function fn(){
return function(){}
}
fn();
</script>
here fn Is a higher-order function
Functions are also a data type that can be passed as parameters to another parameter, most typically as callback functions.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
</head>
<body>
<div></div>
<script>
// Higher-order function-function can be passed as a parameter
function fn(a,b,callback){
console.log(a+b);
callback && callback();
}
fn(1,2,function(){
console.log('I was last called');
});
$("div").animate({
left:500
},function(){
$("div").css("backgroundColor","purple");
})
</script>
</body>
</html>
5. Closure
5.1 Variable Scope
Variables can be divided into two types depending on scope: global and local variables
1. Global variables can be used inside a function
2. Local variables cannot be used outside a function
3. When the function is executed, local variables in this scope are destroyed
5.2 What is a closure
closure(closure) A function that has access to variables in the scope of another function.-----javascript Advanced Programming
A simple understanding is that one scope can access local variables within another function.
<body>
<script>
// A closure is a function that has access to variables in the scope of another function.
// Closure: we fun this function scope, access another function fn local variable num
function fn(){
var num=10;
// function fun(){
// console.log(num);
// }
// fun();
return function(){
console.log(num);
}
}
var f=fn();
f();
// Be similar to
// var f=function fun(){
// console.log(num);
// }
// The primary role of closures: extends the scope of variables
</script>
</body>
5.5 Closure Cases
1. Cyclic click event.
2. In loop setTimeout().
3. Calculate taxi cases.
Cyclic Click Event
<body>
<ul class="nav">
<li>Durian</li>
<li>Stinky tofu</li>
<li>Big Pig's Foot*</li>
</ul>
<script>
// 1. We can take advantage of dynamically adding attributes
var lis=document.querySelectorAll('li');
for(var i=0;i<lis.length;i++){
lis[i].index=i;
lis[i].onclick=function(){
console.log('++++'+i);
console.log(this.index);
}
}
// 2. Use closures to get the index number of the current small li
for(var i=0;i<lis.length;i++){
(function(i){
console.log('---'+i);
lis[i].onclick=function(){
console.log(i);
}
})(i);
}
</script>
</body>
setTimeout() in a loop
<body>
<ul class="nav">
<li>Durian</li>
<li>Stinky tofu</li>
<li>Big Pig's Foot*</li>
</ul>
<script>
// Print the contents of all li elements after closure application - 3 seconds
var lis=document.querySelector('.nav').querySelectorAll('li');
for(var i=0;i<lis.length;i++){
(function(i){
setTimeout(function(){
console.log(lis[i].innerHTML);
},3000)
})(i);
}
</script>
</body>
Calculate taxi price
<body>
<script>
// The taxi start price is 13 (within three kilometers). After that, no more than one kilometer is added 5 yuan, the user can calculate the taxi price by entering kilometers.
// If there is congestion, the total price is 10 yuan more congestion fee
var car=(function(){
var start=13; // Start price
var total=0; // Total Price
return {
// Normal Price
price: function(n){
if(n<=3){
total=start;
}else{
total=start+(n-3)*5;
}
return total
},
// Traffic jam
yd:function(flag){
return flag?total+10:total;
}
}
})();
console.log(car.price(5)); // 23
console.log(car.yd(true)); // 33
console.log(car.yd(false)); //23
</script>
</body>
Reflection
<body>
<script>
// Think Question 1:
var name="The Window";
var object={
name:"My Object",
getNameFunc:function(){
return function(){
return this.name;
};
}
};
console.log(object.getNameFunc()()); // The window
// var f=object.getNameFunc();
// //Similar to
// var f=function(){
// return this.name;
// }
// fn();
// Think Question 2:
var name="The Window";
var object={
name:"My Object",
getNameFunc:function(){
var that=this;
return function(){
return that.name;
};
}
};
console.log(object.getNameFunc()()); // My object
// var f=object.getNameFunc();
// //Similar to
// var f=function(){
// return that.name;
// }
// fn();
</script>
</body>
5.6 Closure Summary
1. What are closures?
Closure is a function(Local variables where one scope can access another function)
2. What is the function of closures?
Extend the scope of a variable
6. Recursion
6.1 What is recursion?
If a function can call itself internally, it is a recursive function.
Simple understanding: a function calls itself within itself, and this function is a recursive function.
<body>
<script>
// Recursive function: a function calls itself within itself, and this function is a recursive function
var num=1;
function fn(){
console.log('I want to print six sentences');
if(num==6){
return; // Exit criteria must be added to recursion
}
num++;
fn();
}
fn();
</script>
</body>
6.2 Use recursion to solve mathematical problems
1. Seek 1*2*3*4*...*n Factorial.
2. Requesting Fibonacci series.
<body>
<script>
// Using recursive function to find Fibonacci (Rabbit) 1,1,2,3,5,8,13,21...
// User can enter a number n to calculate the number of rabbits
var i=1,num=1,num1=1,num2=1,n=21;
function sum(){
i++;
console.log(num);
if(num==n){
return i;
};
num=num1+num2;
num1=num2;
num2=num;
sum();
}
sum();
</script>
</body>
6.3 Recursive Request: Return corresponding data objects based on id
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
var data=[{
id:1,
name:'household electrical appliances',
goods:[{
id:11,
gname:'Refrigerator',
goods:[{
id:111,
gname:'Haier',
},{
id:112,
gname:'Beautiful'
}]
},{
id:12,
gname:'Washing machine'
}]
}];
// We want to enter an id number to return a data object
// 1. Use forEach to traverse every object inside
function getID(json,id){
var o={};
json.forEach(function(item){
console.log(item); // Two Array Elements
if(item.id==id){
console.log(item);
o=item;
return item;
// 2. We want to get the underlying data 11 12 using recursive functions
// There should be a goods array inside and the length of the array is not zero
}else if(item.goods && item.goods.length>0){
o=getID(item.goods,id);
}
});
return o;
}
console.log(getID(data,112));
</script>
</body>
</html>
6.4 Shallow and Deep Copies
1. Shallow copies are just one level of copy, and deeper object-level, copy-only references.
2. Deep copies are multiplayer, and data at each level is copied.
3. Object.assign(target,...sources) es6 The new method can be shallowly copied.
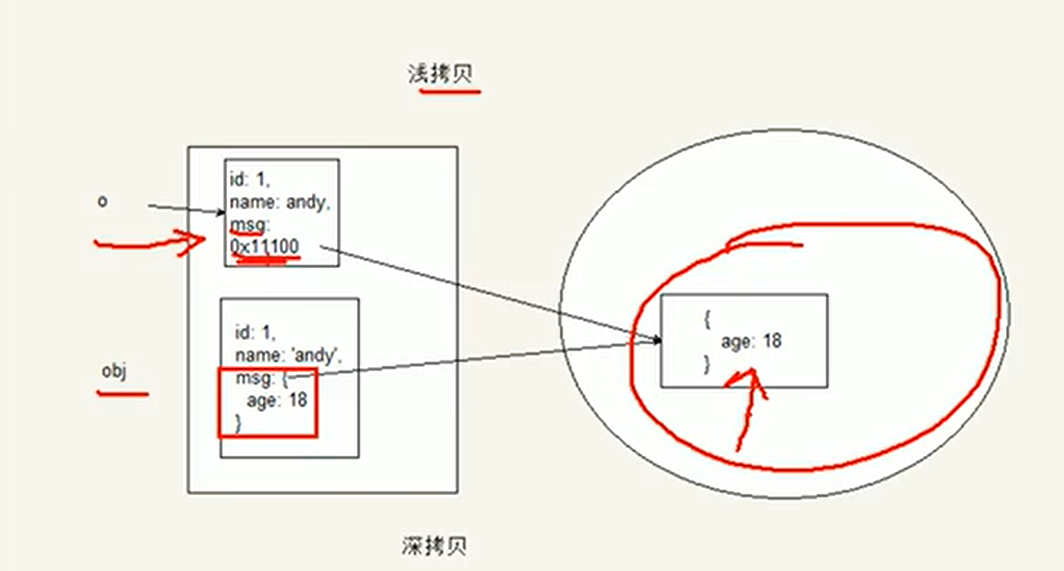
6.4.1 Shallow Copy
<script>
// Shallow copies are only one level of copy, followed by deep object-level copy-only references.
// Deep copies are multiplayer, and data at each level is copied.
var obj={
id:1,
name:'andy',
msg:{
age:18
}
};
var o={};
// for(var k in obj){
// // K is the property name, obj[k] property value
// o[k]=obj[k];
// }
// console.log(o);
// // o.msg.age=20;
// // console.log(obj);
console.log('---------------');
Object.assign(o,obj);
console.log(o); // 20
o.msg.age=20;
console.log(obj); // 20
</script>
</body>
6.4.2 Deep Copy
<body>
<script>
// Deep copies are multiplayer, and data at each level is copied.
var obj={
id:1,
name:'andy',
msg:{
age:18
},
color:['pink','red']
};
var o={};
// Encapsulation function
function deepCopy(newobj,oldobj){
for(var k in oldobj){
// Judging which data type our attribute values belong to
// 1. Get the attribute value oldobj[k]
var item=oldobj[k];
// 2. Determine if the value is an array
if(item instanceof Array){
newobj[k]=[];
deepCopy(newobj[k],item);
}else if(item instanceof Object){
// 3. Determine if this value is an object
newobj[k]=[];
deepCopy(newobj[k],item);
}else{
// 4. Is a simple data type
newobj[k]=item;
}
}
}
deepCopy(o,obj);
console.log(o); // 20
var arr=[];
console.log(arr instanceof Object);
o.msg.age=20;
console.log(obj); // 18
</script>
</body>
7. Regular expressions
1. Overview of Regular Expressions
1.1 What is a regular expression
Regular expressions ( Regular Expression)Is a pattern used to match character combinations in strings.stay JavaScript Medium, regular expressions also
Is the object.
Regular expressions are often used to retrieve and replace text that conforms to a pattern (rule), such as a validation form: User name forms can only be entered in English
Mother, number or underscore. You can enter Chinese in the nickname input box (match).In addition, regular expressions are often used to filter one of the page contents
Some sensitive words (substitution), or get the specific part (extraction) we want from the string, etc.
Regular expressions are also used in other languages, and at this stage we will focus on using JavaScript Regular expressions complete form validation.
Characteristics of 1.2 Regular Expressions
1. Flexibility, logic and functionality are very strong.
2. Complex control of strings can be achieved quickly and in a very simple way.
3. It's obscure for people you've just met.For example:
4. In practice, regular expressions are typically copied directly, but they are required to be used and modified as appropriate.For example, user name:/^[a-z0-9_-]{3,16}$/
2. Use of regular expressions in JavaScript
2.1 Create regular expressions
stay JavaScript You can create a regular expression in two ways.
1. By calling RegExp Constructor creation for objects
var Variable Name = new RegExp(/Expression/);
2. Create by Literal Quantity
var Variable Name = /Expression/;
// An expression placed in the middle of a comment is a regular literal quantity
<body>
<script>
// Use of regular expressions in js
// 1. Create regular expressions using RegExp objects
var regexp = new RegExp(/123/);
console.log(regexp);
// 2. Create regular expressions using literal quantities
var rg=/123/;
console.log(rg);
</script>
</body>
2.2 Testing regular expression test
test() Regular object method that detects whether a string conforms to this rule and returns true or false, Its parameter is a test string.
regexObj.test(str)
1. regexObj Is Written Regular Expression
2. str Text to be tested
3. Is Detection str Does the text conform to the regular representation specification we wrote?
<body>
<script>
// Use of regular expressions in js
// 1. Create regular expressions using RegExp objects
var regexp = new RegExp(/123/);
console.log(regexp);
// 2. Create regular expressions using literal quantities
var rg=/123/;
// 3. The test method is used to detect whether a string meets the specification of a regular expression
console.log(rg.test(123));
console.log(rg.test('abc'));
</script>
</body>
3. Special characters in regular expressions
Composition of 3.1 Regular Expressions
A regular expression can be composed of simple characters.such as/abc/,It can also be a combination of simple and special characters, such as/ab*c/. among
Special characters, also known as metacharacters, are special symbols that have special meaning in regular expressions, such as:^, $, +Wait.
Special characters are very large, you can refer to:
- MDN: https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Guide/Regular_Expressions
- jQuery Manual: Regular Expression Section
- Regular Test Tool: http://tool.oschina.net/regex
3.2 Boundary Character
Boundary characters (placeholders) in regular expressions are used to indicate where a character is, mainly two characters.
Boundary character | Explain |
---|
^ | Text representing the matching beginning of a line (who to start with) |
$ | Text representing the end of a matching line (who ends with) |
If ^ and $are together, they must match exactly.
<body>
<script>
// Boundary character ^$
var rg = /abc/; // Regular expressions do not require quotation marks, whether they are numeric or string
// /abc/Returns true as long as the string contains ABC
console.log(rg.test('abc'));
console.log(rg.test('aabcd'));
console.log('-------------------------');
var reg=/^abc/;
console.log(reg.test('abc')); // true
console.log(reg.test('abcd')); // true
console.log(reg.test('aabcc')); // false
console.log('-------------------------');
var reg1=/^abc$/; // Exact matching requires an abc string to conform to the specification.
console.log(reg1.test('abc')); // true
console.log(reg1.test('abcd')); // false
console.log(reg1.test('aabcd')); // false
console.log(reg1.test('abcabc')); // false
</script>
</body>
3.3 Character Class
A character class indicates that there is a range of characters to choose from, as long as one of them is matched.All optional characters are enclosed in square brackets.
3.3.1 [-] Square Bracket Internal Range Character -
<body>
<script>
// var rg=/abc/;As long as it contains ABC
// Character class: [] means that there is a list of characters to choose from, as long as one of them is matched
var rg=/[abc]/; // Returns true whenever there is a or b or c
console.log(rg.test('andy'));
console.log(rg.test('bady'));
console.log(rg.test('color'));
console.log(rg.test('red'));
console.log('-------------');
var re1=/^[abc]$/; // Three choices, only the letters a or b or c are returned
console.log(re1.test('aa')); // false
console.log(re1.test('a')); // true
console.log(re1.test('b')); // true
console.log(re1.test('c')); // true
console.log(re1.test('abc')); // false
console.log('--------------------');
var reg=/^[a-z]$/; // 26 English letters Any letter returns true
console.log(reg.test('a')); // true
console.log(reg.test('z')); // true
console.log(reg.test('1')); // false
console.log(reg.test('A')); // false
</script>
</body>
3.3.2 Character Combination
/^[a-z0-9]$/.test('a') // true
<body>
<script>
// Character Combination
var reg1=/^[a-zA-Z0-9_-]$/;
// 26 English letters (both upper and lower case) Any letter returns true, -denotes the range of a-Z
console.log(reg1.test('a')); // true
console.log(reg1.test('B')); // true
console.log(reg1.test('8')); // true
console.log(reg1.test('-')); // true
console.log(reg1.test('_')); // true
console.log(reg1.test('!')); // false
</script>
</body>
3.3.3 [^] Negative inside square brackets ^
/^[^abc]$/.test('a') // false
<body>
<script>
// Character Combination
var reg1=/^[^a-zA-Z0-9_-]$/;
// 26 English letters (both upper and lower case) Any letter returns true, -denotes the range of a-Z
// If there is a ^ in the middle bracket to indicate the opposite, don't confuse it with our boundary character ^
console.log(reg1.test('a')); // false
console.log(reg1.test('B')); // false
console.log(reg1.test('8')); // fasle
console.log(reg1.test('-')); // false
console.log(reg1.test('_')); // false
console.log(reg1.test('!')); // true
</script>
</body>
3.4 Quantifier
Quantifiers are used to set the number of times a pattern occurs.
Classifier | Explain |
---|
* | Repeat zero or more times |
+ | Repeat one or more times |
? | Repeat zero or once |
{n} | Repeat n times |
{n,} | Repeat n or more times |
{n,m} | Repeat n to m times |
<body>
<script>
// Quantifier: Used to set the number of times a pattern occurs
// Simple explanation: let the following a character repeat many times
// var reg=/^a$/;
// *Equivalent to >=0 may occur 0 or many times
var reg=/^a*$/;
console.log(reg.test('')); // true
console.log(reg.test('a')); // true
console.log(reg.test('aaa')); // true
console.log("-------------------------");
// +Equivalent to >=1 can occur once or many times
var reg1=/^a+$/;
console.log(reg1.test('')); // fasle
console.log(reg1.test('a')); // true
console.log(reg1.test('aaa')); // true
console.log("-------------------------");
// What?Equivalent to 1 || 0
var reg1=/^a?$/;
console.log(reg1.test('')); // true
console.log(reg1.test('a')); // true
console.log(reg1.test('aaa')); // false
console.log("-------------------------");
// {3} Repeat three times
var reg1=/^a{3}$/;
console.log(reg1.test('')); // fasle
console.log(reg1.test('a')); // false
console.log(reg1.test('aaa')); // true
console.log("-------------------------");
// {3,} greater than or equal to 3 times
var reg1=/^a{3,}$/;
console.log(reg1.test('')); // fasle
console.log(reg1.test('aaa')); // false
console.log(reg1.test('aaaa')); // true
console.log("-------------------------");
// {3,5} greater than or equal to 3 times less than or equal to 5 times
var reg1=/^a{3,5}$/;
console.log(reg1.test('')); // fasle
console.log(reg1.test('aa')); // false
console.log(reg1.test('aaaaa')); // true
console.log("-------------------------");
// A quantifier is the number of times a pattern occurs
var reg2=/^[a-zA-Z0-9_-]{6,16}$/;
// Users in this mode can only enter letters, numbers, underscores, dashes,
// But there are boundaries and [], which limits the choice to only one
// Don't have spaces in the middle of {6,16}
// false
console.log(reg2.test('a'));
console.log(reg2.test('8'));
console.log(reg2.test('18'));
console.log(reg2.test('aa'));
console.log('-----------------------');
console.log(reg2.test('andy_red')); // true
console.log(reg2.test('andy-red')); // true
console.log(reg2.test('andy007')); //true
console.log(reg2.test('andy!007')); //false
</script>
</body>
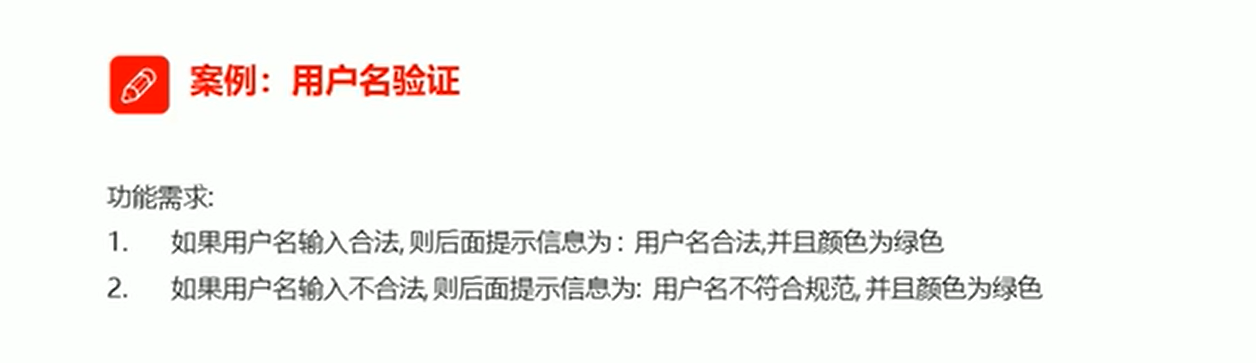
3.5 Bracket Summary
1. Brace quantifier, which indicates the number of repetitions
2. A collection of bracketed characters.Match any character in square brackets.
3. Parentheses denote priority
Can be tested online: https://c.runoob.com/
3.6 Predefined Classes
Predefined classes refer to the abbreviation of some common patterns.
Predefined class | Explain |
---|
\d | Match any number between 0-9, equivalent to [0-9] |
\D | Matches all characters except 0-9, equivalent to [^0-9] |
\w | Match any letter, number, underscore, equivalent to [A-Za-z0-9_] |
\W | Divide by any letter, number, underscore, equivalent to [^A-Za-z0-9_] |
\s | Matches spaces (including line breaks, tabs, space characters, etc.), equivalent to [\t\r\n\v\f] |
\S | Matches a character that is not a space, equivalent to [^\t\r\n\v\f] |
<body>
<script>
// Verification of seat number: National seat number, in two formats: 010-12345678 or 0530-1234567
// Or symbol inside a regular |
var reg=/^\d{3}-\d{8}|\d{4}-\d{7}&/
</script>
</body>
4.1 replace replacement
replace() Method can implement substitution string operation, and the parameter used for substitution can be a string or a regular expression.
stringObject.replace(regexp/substr,replacement)
1. First parameter: substituted string or regular expression
2. Second parameter: string to replace with
3. Return value is a new string replaced
<body>
<script>
// Replace replace
// var str='andy and red'
// // var newStr=str.replace('andy','body');
// var newStr = str.replace(/andy/,'body');
// console.log(newStr);
var text=document.querySelector('textarea');
var btn=document.querySelector('button');
var div=document.querySelector('div');
btn.onclick=function() {
div.innerHTML=text.value.replace(/Passion/,'**');
}
</script>
</body>
4.2 Regular Expression Parameters
/Expression/[switch]
switch(Also known as modifiers)There are three values for which pattern to match:
1. g:Global Matching
2. i:ignore case
3. gi:Global Matching+ignore case
<body>
<script>
// Replace replace
// var str='andy and red'
// // var newStr=str.replace('andy','body');
// var newStr = str.replace(/andy/,'body');
// console.log(newStr);
var text=document.querySelector('textarea');
var btn=document.querySelector('button');
var div=document.querySelector('div');
btn.onclick=function() {
div.innerHTML=text.value.replace(/Passion|gay/g,'**');
}
</script>
</body>