Note: Use redis as session storage
Getting cookie s using dynamic js addresses introduced into sso servers
After the application server obtains the special identity provided by the single point server, such as session ID or other,
Go to redis directly through identification, or submit (through rpc) to a single server to query for login information results
Server code example
const Koa = require('koa'); const Router = require('koa-router'); const bodyParser = require('koa-bodyparser'); const app = new Koa(); const router = new Router(); app.use(bodyParser()); //====session s===== var session = require('koa-generic-session'); var redisStore = require('koa-redis'); app.keys = ['keys', 'c29tZSBzZWNyZXQgaHVycg']; app.use(session({//Configure session store: redisStore({}), cookie: { path: '/', httpOnly: true, maxAge: 1 * 60 * 60 * 1000, rewrite: true, signed: true } })); //====session e===== router.get('/login', function* (next) {//Login page this.session=null;//delete cookie this.body=` <form action="/login" method="post"> <p>User name: <input type="text" name="name" /></p> <p>Password: <input type="text" name="pwd" /></p> <input type="submit" value="Submission" /> </form> `; }).post('/login', function* (next) {//Submit login data var sinfo = JSON.stringify(this.request.body);//<== Get post data this.session.sinfo =sinfo;//<==== save session to simulate successful login this.redirect('/');//<==== jump to the page you want }); router.get('/', function* (next) { if(this.session&&this.session.sinfo){//Determine whether cookie s exist this.body=`Already logged in `; }else{ this.redirect('/login');//<==== jump to the page you want } }); router.get('/sso.js', function* (next) { //Dynamic js if(this.session&&this.session.sinfo&&this.session.sinfo.length>0){ this.body=`var kosid='${this.sessionId}';`;//The example is written to session Id, which is the key stored in redis }else{ this.body=`window.location.href="http://sso.com/login";`; } }); app.use(router.routes()).use(router.allowedMethods()); app.listen(8087);
Application code example:
const Koa = require('koa'); const Router = require('koa-router'); const bodyParser = require('koa-bodyparser'); const app = new Koa(); const router = new Router(); app.use(bodyParser()); //===== session s============= You can use ordinary session directly. app.keys = ['c29tZSBzZWNyZXQgaHVycg%3D%3D']; var CONFIG = { key: 'koa:sess', /** (string) cookie key (default is koa:sess) */ maxAge: 2000, /** (number) maxAge in ms (default is 1 days) */ overwrite: true, /** (boolean) can overwrite or not (default true) */ httpOnly: true, /** (boolean) httpOnly or not (default true) */ signed: true, /** (boolean) signed or not (default true) */ }; app.use(session(CONFIG, app)); //====session e===== /* //====session s===== Or the same. var session = require('koa-generic-session'); var redisStore = require('koa-redis'); app.keys = ['keys', 'c29tZSBzZWNyZXQgaHVycg']; app.use(session({//Configure session store: redisStore({}), cookie: { path: '/', httpOnly: true, maxAge: 1 * 60 * 60 * 1000, rewrite: true, signed: true } })); //====session e===== */ router.get('/', function* (next) { this.body=` <script type="text/javascript" src="http://cdn.bootcss.com/jquery/3.2.1/jquery.min.js"></script> <script type="text/javascript" src="http://cdn.bootcss.com/jquery-cookie/1.4.1/jquery.cookie.min.js"></script> <script src='http://sso.com:8087/sso.js'></script> <script> $.cookie("sid",kosid); if(kosid){ document.write('key:',kosid); }else{ document.write('Not logged in'); } console.log("this",document.cookie); </script>`; return; }); app.use(router.routes()).use(router.allowedMethods()); app.listen(8088);
Modifying hosts files under windows system
(Generally under C: Windows System32 drivers etc)
Add to:
127.0.0.1 sso.com 127.0.0.1 testsso.com
Browser access:
http://sso.com:8087
http://testsso.com:8088
Effect picture:
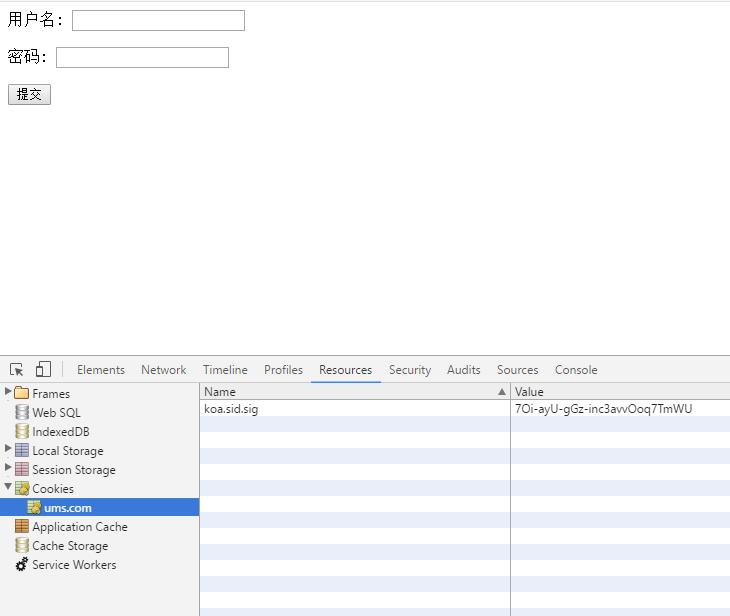
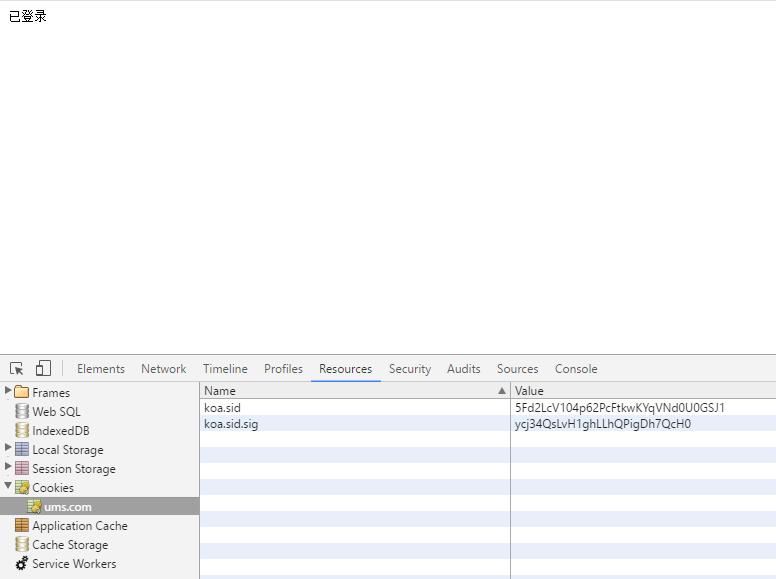
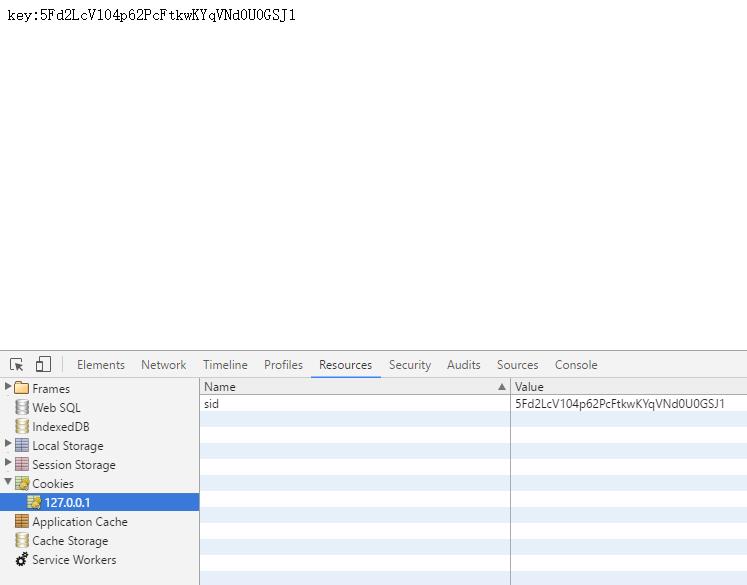
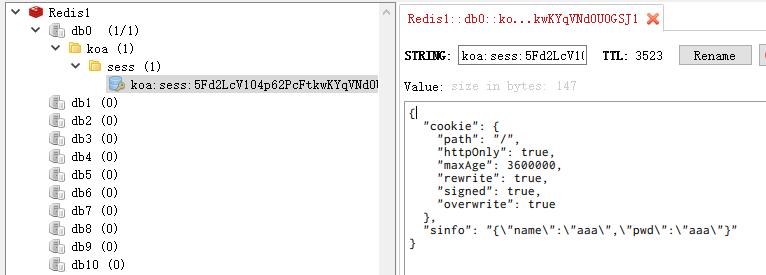
The actual situation may not be so simple, for example, we can get browser information, timestamp, create UUID, and so on in the way of URL reference combined with cookie to judge the legitimacy of login users. Wait...