https://www.youtube.com/playlist?list=PLsyeobzWxl7qbvNnJKjYbkTLn2w3eRy1Q
First, you can write method body in the interface
When a new method is added to the interface, a method body can be brought along.
Benefits:
When the new interface is released, the classes that previously implemented the interface need not be changed.
Namely, there is no need to implement the newly added method.
Syntax: Use default keywords
- interface A{
- void show();
- default void sayHello(){
- System.out.println("Hello, World!");
- }
- }
Be careful:
1. When implementing multiple interfaces, there is implementation of method bodies with duplicate names in the interfaces.
If two interfaces with the same method name are implemented simultaneously,
You need to provide your own implementation of this method. Only then can the conflict be resolved.
sayHello() duplicates, class C needs to implement its own sayHello()
- interface A{
- void showA();
- default void sayHello(){
- System.out.println("Hello, A!");
- }
- }
- interface B{
- void showB();
- default void sayHello(){
- System.out.println("Hello, B!");
- }
- }
- class C implements A,B{
- public void sayHello(){
- System.out.println("Hello, C!");
- }
- public static void main(String[] args){
- C c = new C();
- c.sayHello(); // Hello, C!
- }
- }
Priority of Class Method over Interface Method
The implementation of method sayHello() is defined in both interface A and class B.
Class C prefers the method of class B.
- interface A{
- void showA();
- default void sayHello(){
- System.out.println("Hello, A!");
- }
- }
- class B{
- public void sayHello(){
- System.out.println("Hello, B!");
- }
- }
- class C extends B implements A{
- public static void main(String[] args){
- C c = new C();
- c.sayHello(); // Hello, B!
- }
- }
3. Methods in java.lang.Object cannot be overridden in interfaces
- interface A {
- // can't define a equals method in interface.
- default public boolean equals(){
- }
- }
4. static methods can be defined in interfaces
- interface A{
- void showA();
- static void sayHello(){
- System.out.println("Hello, A!");
- }
- }
- class C {
- public static void main(String[] args){
- A.sayHello(); // Hello, A!
- }
- }
Functional Programming VS. Object Oriented Programming
Functional Programming with Java 8
https://www.youtube.com/watch?v=LcfzV38YDu4
Functional Programming refers to the use of Lambda expressions for interfaces with only one method.
A good example of an interface with only one method is the java.lang.Comparable interface.
It has only one method: compareTo(Object obj)
3. Lambda Expression (-> Look sideways)
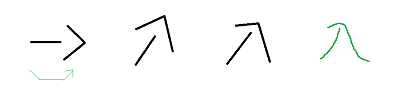
Lambda Expression VS. Anonymous inner class
For implementation classes with only one method interface, when writing implementation classes, you only need to provide (parameter + Lambda + method body).
- interface A{
- void show();
- }
- public class LambdaDemo{
- public static void main(String[] args){
- A obj;
- // old
- obj = new A(){
- public void show(){
- System.out.println("Hello");
- }
- }
- // java 8
- obj = () -> {
- System.out.println("Multiple");
- System.out.println("Lines ... ");
- }
- // or
- obj = () -> System.out.println("Single line.");
- }
- }
Explain:
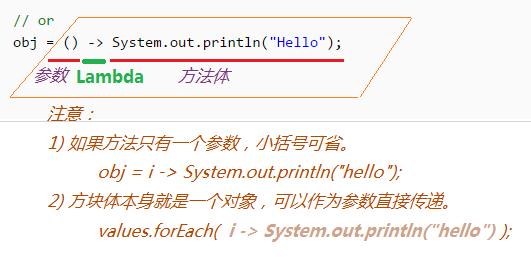
4. Adding forEach() method to java.lang.Iterable interface
NOTE:
1. Before java 8, there was only one way for the java.lang.Iterable interface:
java.util.Iterator<T> iterator()
2. The java.util.Collection interface inherits the java.lang.Iterable interface.
- import java.util.Arrays;
- import java.util.List;
- import java.util.function.Consumer;
- class IConsumer implements Consumer<Integer>{
- @Override
- public void accept(Integer t) {
- System.out.println(t);
- }
- }
- public class Java8ForEach {
- public static void main(String[] args) {
- List<Integer> list =Arrays.asList(1,2,3,5,6);
- // normal loop
- for(Integer i : list){
- System.out.println(i);
- }
- // Java 8 forEach - normal
- Consumer<Integer> action = new IConsumer();
- list.forEach(action);
- // Java 8 forEach - use lambda expressions.
- // see how we do it in one liner
- list.forEach(each -> System.out.println(each));
- }
- }
V. Streaming API
Java collections got a new package java.util.Stream.
classes in this package provide a stream API.
And supports functional-style operations on streams of elements.
Stream API enables bulk operations like sequential or parallel map-readuce on Collections.
- //Java 7 or earlier:
- public List listFilter(List<Integer> bigList){
- List<Integer> filteredList = new ArrayList<>();
- for (Integer p : bigList) {
- if (p > 40) {
- filteredList.add(p);
- }
- }
- return filteredList;
- }
- //Java 8:
- public List listFilter(List<integer> bigList){
- return bigList
- .stream()
- .filter(p -> p > 40)
- .collect(Collectors.toList());
- }
-
So, if you know, in this world, it's an information age, we have a concept of "Big Data", "Haddoop", we have to process huge amount of data.
1) In stream we have two types of methods:
1. Intermediate method. Like: filter(), map()
lazy, it can't give result immediately until you call a terminate method.
2. Terminate method. Like: findFirst(), forEach()
Example Given:
- List<Integer> values = Arrays.asList(4,5,6,7,8);
- values.stream().filter(i->{
- Sysout.out.println("Hello");
- return true;
- });
2) Stream is once used, it can't be reused:
- Stream<Integer> s = values.stream();
- s.forEach(Sysout.out::println); // works
- s.forEach(Sysout.out::println); // throws Exception
More:
New features of Java 9
http://programtalk.com/java/java-9-new-features/
Quote:
Java 8 Stream API Features Lambda Expression
https://www.youtube.com/playlist?list=PLsyeobzWxl7otduRddQWYTQezVul0xIX6
http://programtalk.com/java/java-8-new-features/