- home page
- special column
- c#
- Article details
NETCORE 3.1 uses the Jwt protection api
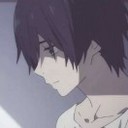
abstract
This article demonstrates how to provide jwt to effective users and how to use this token in webapi to authenticate users through JwtBearerMiddleware middleware.
What is the difference between authentication and authorization?
First, we should make clear the difference between authentication and Authorization to avoid confusion. Authentication is the process of confirming who you are, and Authorization revolves around what you are allowed to do, that is, permissions. Obviously, before confirming what users are allowed to do, you need to know who they are. Therefore, when Authorization is required, users must be authenticated in some way.
What is JWT?
According to the definition of Wikipedia, JSON WEB Token (JWT) is a JSON based token used to declare a claim on the network. JWT usually consists of three parts: header, payload and signature.
The header information specifies the signature algorithm used by the JWT:
header = '{"alg":"HS256","typ":"JWT"}'
HS256 indicates that HMAC-SHA256 is used to generate a signature.
The message body contains the intent of JWT:
payload = '{"loggedInAs":"admin","iat":1422779638}'//iat represents the time when the token was generated
The unsigned token is spliced by the header information encoded by base64url and the message body (separated by "."), and the signature is calculated by the private key:
key = 'secretkey' unsignedToken = encodeBase64(header) + '.' + encodeBase64(payload) signature = HMAC-SHA256(key, unsignedToken)
Finally, the signature encoded by base64url (also separated by ".") on the tail of the unsigned token is JWT:
token = encodeBase64(header) + '.' + encodeBase64(payload) + '.' + encodeBase64(signature) # The token looks like this: eyjhbgcioijiuzi1niiinr5cci6ikpxvcj9.eyjsb2dnzwrjbkfzijoiywrtaw4ilcjpyxqioje0mji3nzk2mzh9.gzslasys8exbxln_ oWnFSRgCzcmJmMjLiuyu5CSpyHI
JWT is often used to protect the resource s of the server. The client usually sends the JWT to the server through the HTTP Authorization header. The server uses its saved key to calculate and verify the signature to determine whether the JWT is trusted:
Authorization: Bearer eyJhbGci*...<snip>...*yu5CSpyHI
preparation
Create a webapi project using vs2019 and install the nuget package
Microsoft.AspNetCore.Authentication.JwtBearer
Startup class
ConfigureServices add authentication service
services.AddAuthentication(options => { options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme; options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme; options.DefaultScheme = JwtBearerDefaults.AuthenticationScheme; }).AddJwtBearer(options => { options.SaveToken = true; options.RequireHttpsMetadata = false; options.TokenValidationParameters = new TokenValidationParameters() { ValidateIssuer = true, ValidateAudience = true, ValidAudience = "https://xx", ValidIssuer = "https://xx", IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("SecureKeySecureKeySecureKeySecureKeySecureKeySecureKey")) }; });
Configure configure authentication Middleware
app.UseAuthentication();// Authentication Middleware
Create a token
Add a login model named LoginInput
public class LoginInput { public string Username { get; set; } public string Password { get; set; } }
Add an authentication controller named AuthenticateController
[Route("api/[controller]")] public class AuthenticateController : Controller { [HttpPost] [Route("login")] public IActionResult Login([FromBody]LoginInput input) { //Verify user name and password from database //Verification passed, otherwise Unauthorized is returned //Create claim var authClaims = new[] { new Claim(JwtRegisteredClaimNames.Sub,input.Username), new Claim(JwtRegisteredClaimNames.Jti,Guid.NewGuid().ToString()) }; IdentityModelEventSource.ShowPII = true; //The signature key can be placed in a json file var authSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("SecureKeySecureKeySecureKeySecureKeySecureKeySecureKey")); var token = new JwtSecurityToken( issuer: "https://xx", audience: "https://xx", expires: DateTime.Now.AddHours(2), claims: authClaims, signingCredentials: new SigningCredentials(authSigningKey, SecurityAlgorithms.HmacSha256) ); //Return token and expiration time return Ok(new { token = new JwtSecurityTokenHandler().WriteToken(token), expiration = token.ValidTo }); } }
Add api resources
Use the default controller WeatherForecastController
- Add an Authorize tag
- Adjust the route to: [Route("api/[controller])] code as follows
[Authorize] [ApiController] [Route("api/[controller]")] public class WeatherForecastController : ControllerBase
Now that all the code is ready, let's run the test
Run project
Simulation using postman
- It is found that 401 is not authenticated when returned. Get the token below
- Obtain token through user and password
- If our credentials are correct, we will return a token and expiration date, and then use the token for access
- Request using token
- Request status 200 now!
Original text:[ https://www.cnblogs.com/cheng...]()