[.NET] Step by step build a simple MVC website - BooksStore
Keywords:
ASP.NET
SQL
Database
Java
PHP
Step by step build a simple MVC website - BooksStore
brief introduction
The main functions and knowledge points are as follows:
Classification, product browsing, shopping cart, settlement, CRUD management, paging and unit testing.
[Remarks] The project is developed using VS2015 + C.
I. Creating Project Architecture
1. Create a new solution "BooksStore" and add the following items:
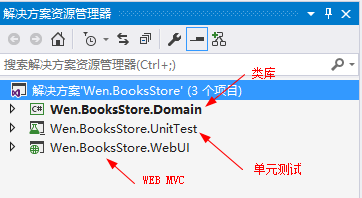
BooksStore.Domain: Class libraries, storage domain models and logic, using EF;
BooksStore.WebUI: Web applications that store views and controllers as display layers;
BoosStore.Unit Test: Unit test, which tests the two projects mentioned above.
Web MVC is an empty MVC project:
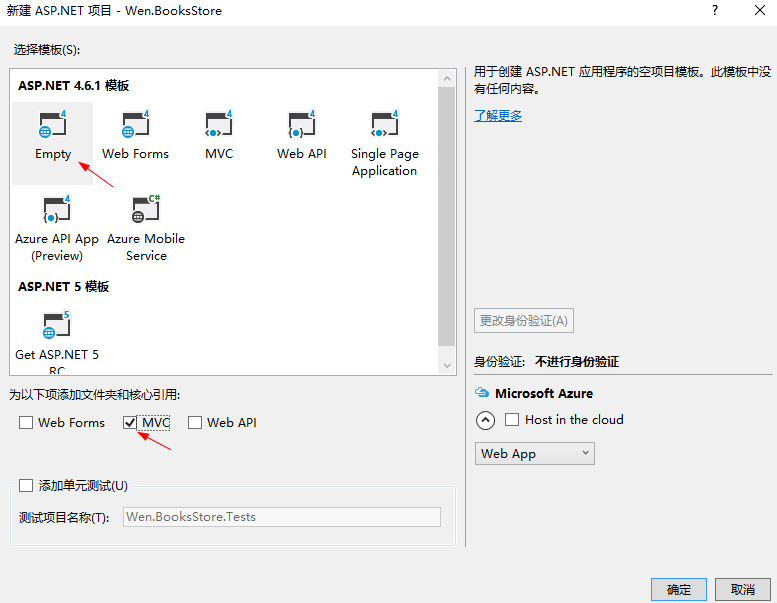
2. Add project references (NuGet is required):
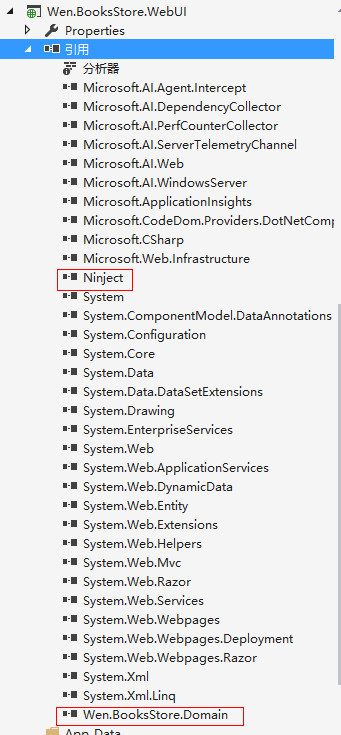
These are libraries and projects that need to be referenced by different projects
3. Setting up DI containers
Through Ninject, we create a class inheritance DefaultController Factory called NinjectController Factory (default controller factory)
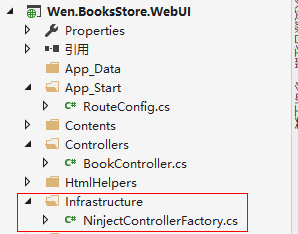
public class NinjectControllerFactory : DefaultControllerFactory
{
private readonly IKernel _kernel;
public NinjectControllerFactory()
{
_kernel = new StandardKernel();
AddBindings();
}
protected override IController GetControllerInstance(RequestContext requestContext, Type controllerType)
{
return controllerType == null
? null
: (IController) _kernel.Get(controllerType);
}
/// <summary>
/// Adding bindings
/// </summary>
private void AddBindings()
{
}
}
Adding a line of code to Global.asax tells MVC to create controller objects with new classes
ControllerBuilder.Current.SetControllerFactory(new NinjectControllerFactory());

public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
RouteConfig.RegisterRoutes(RouteTable.Routes);
ControllerBuilder.Current.SetControllerFactory(new NinjectControllerFactory());
}
}
Global.asax
Creating Domain Model Entities
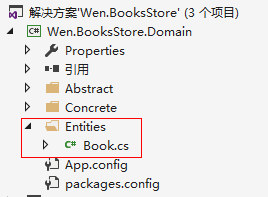
Create an entity class named Book in the figure.
public class Book
{
/// <summary>
/// Identification
/// </summary>
public int Id { get; set; }
/// <summary>
/// Name
/// </summary>
public string Name { get; set; }
/// <summary>
/// describe
/// </summary>
public string Description { get; set; }
/// <summary>
/// Price
/// </summary>
public decimal Price { get; set; }
/// <summary>
/// classification
/// </summary>
public string Category { get; set; }
}
With an entity, we should create a "library" to operate on that entity, and this persistence operation should also be isolated from the domain model, which can be called a repository mode.
Then, create a folder named Abstract in the root directory. As the name implies, some abstract classes, such as interfaces, should be placed. First, we define an interface that operates on the Book repository:
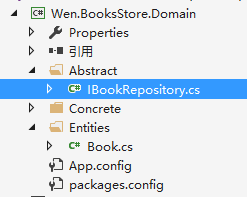
public interface IBookRepository
{
IQueryable<Book> Books { get; }
}
Here, I intend to use simple EF to operate on the database, so I need to download EF's class library through Nuget.
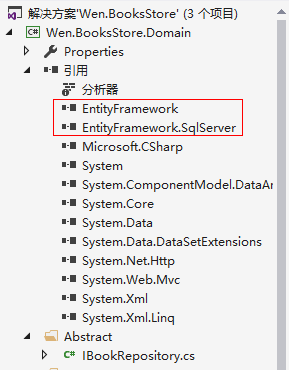
Since the interface class has been defined before, the next step is to define the class that implements the interface.
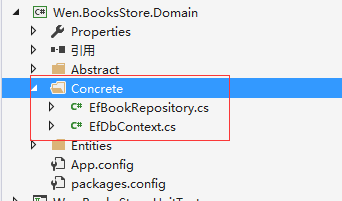
public class EfDbContext : DbContext
{
public DbSet<Book> Books { get; set; }
}
public class EfBookRepository : IBookRepository
{
private readonly EfDbContext _context = new EfDbContext();
public IQueryable<Book> Books => _context.Books;
}
Now it's only time to create a new table in the database.
CREATE TABLE Book
(
Id INT IDENTITY PRIMARY KEY,
Name NVARCHAR(100),
Description NVARCHAR(MAX),
Price DECIMAL,
Category NVARCHAR(50)
)
And insert test data:

INSERT INTO dbo.Book
(
Name ,
Description ,
Price ,
Category
)
VALUES (
N'C#From entry to proficiency' , -- Name - nvarchar(100)
N'Good book-C#From entry to proficiency' , -- Description - nvarchar(max)
50 , -- Price - decimal
N'.NET' -- Category - nvarchar(50)
)
INSERT INTO dbo.Book
(
Name ,
Description ,
Price ,
Category
)
VALUES (
N'ASP.NET From entry to proficiency' , -- Name - nvarchar(100)
N'Good book-ASP.NET From entry to proficiency' , -- Description - nvarchar(max)
60 , -- Price - decimal
N'.NET' -- Category - nvarchar(50)
)
INSERT INTO dbo.Book
(
Name ,
Description ,
Price ,
Category
)
VALUES (
N'Multithreading from entry to proficiency' , -- Name - nvarchar(100)
N'Good book-Multithreading from entry to proficiency' , -- Description - nvarchar(max)
65 , -- Price - decimal
N'.NET' -- Category - nvarchar(50)
)
INSERT INTO dbo.Book
(
Name ,
Description ,
Price ,
Category
)
VALUES (
N'java From Initial to Abandoned' , -- Name - nvarchar(100)
N'Good book-java From Initial to Abandoned' , -- Description - nvarchar(max)
65 , -- Price - decimal
N'java' -- Category - nvarchar(50)
)
INSERT INTO dbo.Book
(
Name ,
Description ,
Price ,
Category
)
VALUES (
N'sql From Initial to Abandoned' , -- Name - nvarchar(100)
N'Good book-sql From Initial to Abandoned' , -- Description - nvarchar(max)
45 , -- Price - decimal
N'sql' -- Category - nvarchar(50)
)
INSERT INTO dbo.Book
(
Name ,
Description ,
Price ,
Category
)
VALUES (
N'sql From entry to exit' , -- Name - nvarchar(100)
N'Good book-sql From entry to exit' , -- Description - nvarchar(max)
45 , -- Price - decimal
N'sql' -- Category - nvarchar(50)
)
INSERT INTO dbo.Book
(
Name ,
Description ,
Price ,
Category
)
VALUES (
N'php From entry to exit' , -- Name - nvarchar(100)
N'Good book-php From entry to exit' , -- Description - nvarchar(max)
45 , -- Price - decimal
N'php' -- Category - nvarchar(50)
)
test data
After doing the preheating operation, you may want to display it in the form of interface immediately. Don't worry. First, use unit test to check whether our operation on the database is normal. After reading the data simply, check whether the connection is successful.
After installation, an app.config configuration file will be generated, requiring an additional line of connection strings.
Right-click inside the method body and you can see an option to "run the test" when you try to click it:
Next, we need to formally display the information we want from the page.
We need to customize a Details method for subsequent interaction with the interface.
Next, create a View view View.
Change the default routing mechanism to let him jump to the page by default.
The results are roughly as follows (because of the addition of a little CSS style, the effect may be slightly different), and the results are consistent.