Native javascript implementation magnifier
Keywords:
Web Development
Attribute
IE
Javascript
The effect is as follows
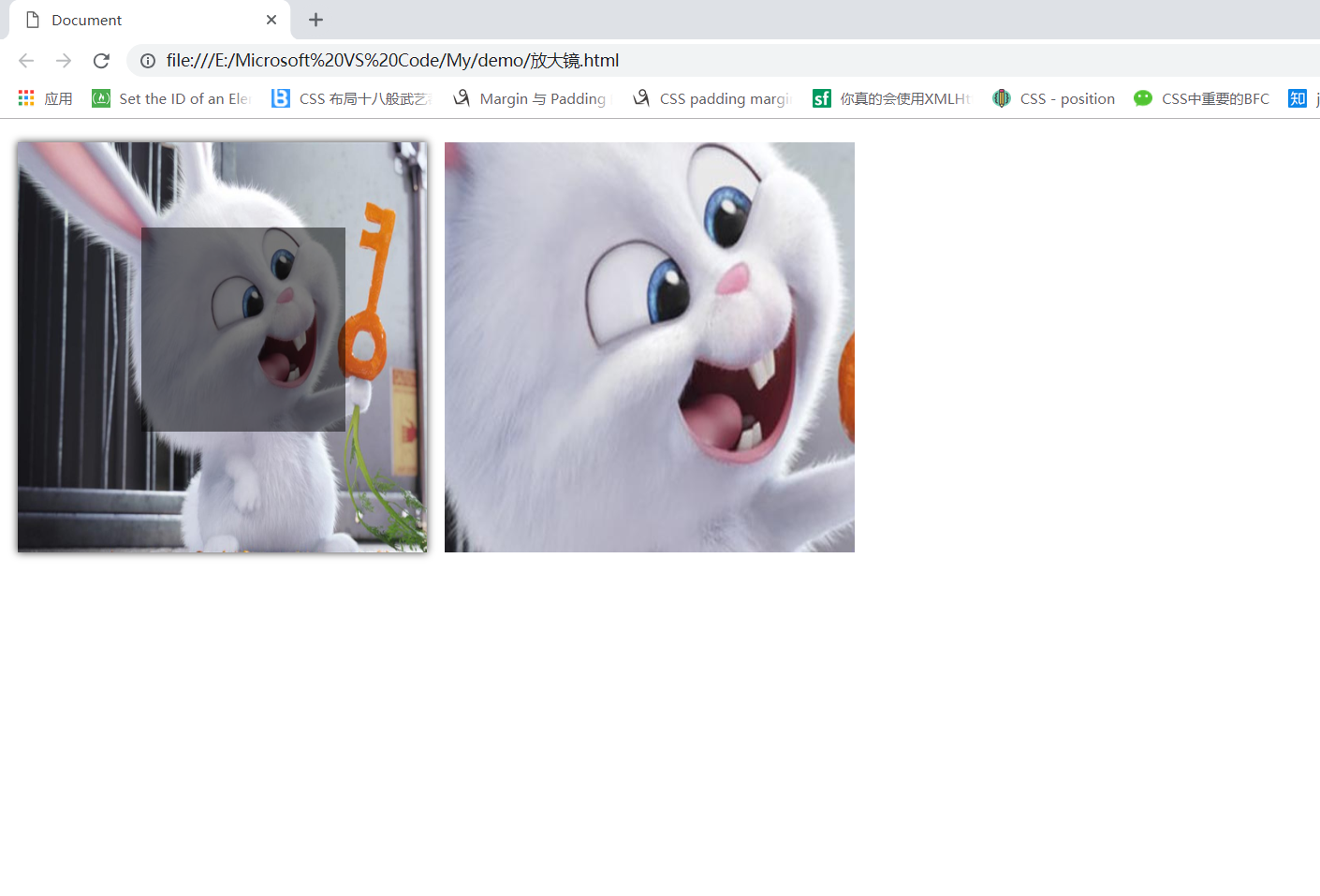
Knowledge Points Used
- offsetTop property:
This attribute can get the distance between the upper and outer edges of the element and the nearest location of the inner wall of the parent element. If the location is not used in the parent element, the distance between the upper and outer edges of the element and the inner wall of the document can be obtained. Location is defined as position attribute values relative, absolute, or fixed.
The return value is an integer in pixels.
- offsetLeft attribute:
This property is the same as offsetTop's principle, but the orientation is different, so we will not introduce it here.
- offsetWidth property:
The width of the element can be obtained by this attribute. The width values include: element content + inner margin + border. Excluding the outer margin and scroll bar section.
The return value is an integer in pixels.
This property is read-only.
- offsetHeight property:
This property can get the height of the element, and the width value includes: element content + inner margin + border. Excluding the outer margin and scroll bar section.
The return value is an integer in pixels.
- The coordinates of the clientX mouse relative to the upper-left x-axis of the browser (in this case, the effective area of the browser) do not change with the scroll bar.
- The coordinates of the clientY mouse relative to the y axis in the upper left corner of the browser (the effective area of the browser here) do not change with the scroll bar.
Calculation as shown
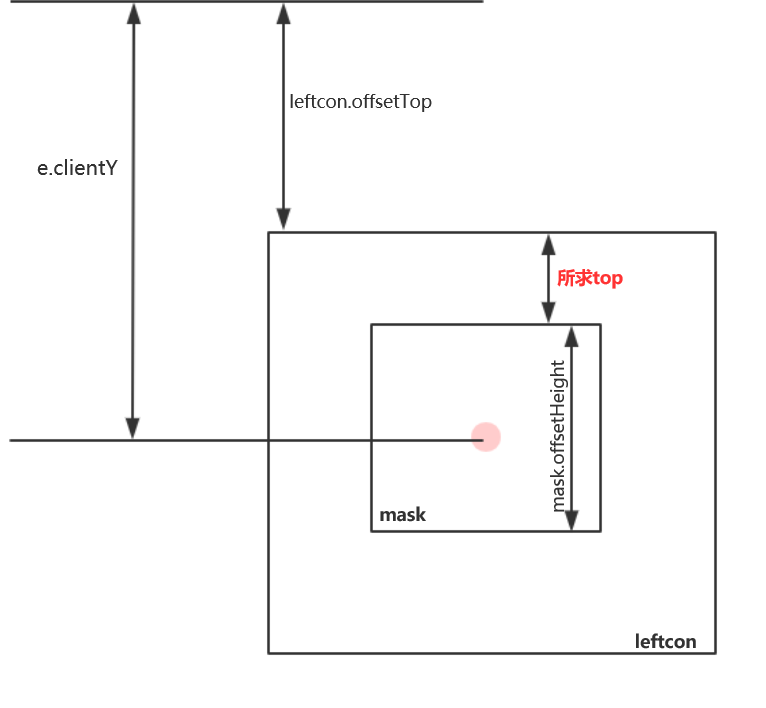
Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title>
</head>
<style>
* {
padding: 0;
margin: 0;
}
.leftcon {
width: 350px;
height: 350px;
position: relative;
float: left;
box-shadow: 0px 0px 10px 0px rgb(65, 64, 64);
margin: 20px 15px;
}
.picl {
display: block;
width: 100%;
height: 100%;
}
.mask {
width: 175px;
height: 175px;
background-color: #000;
opacity: 0.5;
position: absolute;
top: 0;
left: 0;
cursor: move;
display: none;
}
.rightcon {
width: 350px;
height: 350px;
position: relative;
float: left;
overflow: hidden;
margin-top: 20px;
display: none;
}
.picr {
display: block;
width: 200%;
height: 200%;
position: absolute;
left: 0px;
top: 0px;
}
</style>
<body>
<div class="leftcon" id="leftbox">
<img src="pic2.jpg" class="picl">
<div class="mask"></div>
</div>
<div class="rightcon" id="rightbox">
<img src="pic2.jpg" class="picr">
</div>
<script>
var leftcon = document.getElementById('leftbox');
var rightcon = document.getElementById('rightbox');
var mask = document.getElementsByClassName('mask')[0];
var rpic = document.getElementsByClassName('picr')[0];
function getPoint(e) {
var e = e || window.event;
var top = e.clientY - leftcon.offsetTop - mask.offsetHeight / 2;
var left = e.clientX - leftcon.offsetLeft - mask.offsetWidth / 2;
var maxtop = leftcon.offsetHeight - mask.offsetHeight;
var maxleft = leftcon.offsetWidth - mask.offsetWidth;
var mintop = 0;
var minleft = 0;
var mvtop;
var mvleft;
if (top < mintop) {
mask.style.top = mintop + "px";
mvtop = mintop;
} else if (top > maxtop) {
mask.style.top = maxtop + "px";
mvtop = maxtop;
} else {
mask.style.top = top + "px";
mvtop = top;
}
if (left < minleft) {
mask.style.left = minleft + "px";
mvleft = minleft
} else if (left > maxleft) {
mask.style.left = maxleft + "px";
mvleft = maxleft
} else {
mask.style.left = left + "px";
mvleft = left;
}
rpic.style.top = -mvtop * 2 + "px";
rpic.style.left = -mvleft * 2 + "px";
}
leftcon.onmouseover=function(e){
var e = e||window.event;
rightcon.style.display="block";
mask.style.display="block";
getPoint(e);
}
leftcon.onmouseout=function(e){
var e = e||window.event;
rightcon.style.display="none";
mask.style.display="none";
}
leftcon.onmousemove = function(e){
var e=e||window.event;
getPoint(e);
}
</script>
</body>
</html>
Posted by cjacks on Sat, 02 Feb 2019 11:21:16 -0800