Demand:
1. Query a user
2. Query multiple users (List)
Step 1: Make a global configuration file under config/SqlMapConfig.xml (note header information)
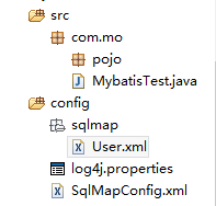
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!-- Configuring data sources --> <!-- and spring After integration environments Configuration will be abolished--> <environments default="development"> <environment id="development"> <transactionManager type="JDBC" /> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver" /> <property name="url" value="jdbc:mysql://localhost:3306/mybatis?characterEncoding=utf-8" /> <property name="username" value="root" /> <property name="password" value="root" /> </dataSource> </environment> </environments> <!-- To configure mapper Mapping file --> <mappers> <mapper resource="sqlmap/User.xml"/> </mappers> </configuration>
Adding config/log4j.properties can print sql statements on the console
Step 2: Create Entity Classes User.java
Step 3: Configure the User.xml mapping file under config/sqlmap/User.xml (note header information)
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <! -- namespace namespace: As a token, but if you use the mapper dynamic proxy method, you need to configure the mapper interface address here - >. <mapper namespace="test"> <!-- Query a record based on the user's ID (return a single record) The select tag represents the sql query, and the content is encapsulated in Mapped Statement. You can call this select tag a Statement. id attribute: the unique identity of the Statement # {}: Represents a placeholder that avoids sql injection ${}: Represents a splicer parameterType: Represents the type of input, that is, what type of placeholder to enter ResultType: The type representing the output result set, and the field name of the select query is the same as the resultType attribute name to map successfully. # {id}:id represents the variable for the parameter input parameter --> <select id="findUserById" parameterType="int" resultType="com.mo.pojo.User"> SELECT * FROM USER WHERE id = #{id} </select> <!-- Query the list of users by name (return to the List collection) Regardless of how many results are returned, resultType specifies the type of a single record mapping java object ${}: Represents sql splicing, which is equivalent to splicing sql strings, and sql injection is unavoidable. ${value}:value represents the variable for the parameter input parameter. With ${}, the variable name must use value. Splice value directly into sql without any modification '%${value}%': Modification can be used in this way Client input sheet sql splices to% sheet% --> <select id="findUserListByName" parameterType="String" resultType="com.mo.pojo.User"> SELECT * FROM USER WHERE username LIKE '%${value}%' </select> </mapper>
Step 4: Test Unit
package com.mo; import java.io.IOException; import java.io.InputStream; import java.util.List; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import org.junit.Test; import com.mo.pojo.User; public class MybatisTest { @Test public void test() throws IOException{ //1. Loading configuration files String resource = "SqlMapConfig.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); //2. Create SqlSessionFactory based on configuration file SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream); //3. Create SqlSession according to SqlSession Factory SqlSession sqlSession = sqlSessionFactory.openSession(); //4. Query returns multiple entries /** * Operating the database through the API of sqlSession * The first parameter is statement: specify the id of the state in the mapper mapping file * When specified, add the space name to which the state belongs * The second is to assign parameters. * * selectOne Returns a single record, and if select returns multiple records (list collection), selectOne will report an error. * * Returning a worthwhile type is the resultType in the mapping file */ User user = sqlSession.selectOne("test.findUserById", 1); List<User> list = sqlSession.selectList("test.findUserListByName","Zhang"); System.out.println(list.size()); System.out.println(user); //5. Close sqlSession sqlSession.close(); } }