In the previous article, we used app.config to define routing matching rules
<script>
var app = angular.module("app", ["ngRoute"]);
app.config(["$routeProvider", function ($routeProvider) {
$routeProvider
.when("/index",
{
template: "<p>Home page out!</p>"
})
.when("/info",
{
template: "<p>I'm your personal information.</p>"
})
.when("/change",
{
template: "<p>Want to modify your personal information?</p>"
})
.otherwise({
redirectTo: "/index"
});
}]);
</script>
In the "when" method, "template" can write Html tags directly. When triggered, HTML tags in the template are automatically embedded in the ng-view view view, but this is not recommended.
We can use "template Url" to specify files that contain html tags, and we can also specify corresponding "controller" controllers.
when("/change",
{
templateUrl: "changeInfo.html",
controller : "changeController"
})
ChangeeInfo. HTML file, note that div does not need to declare the controller controller controller again (<div ng-controller= "change controller"> (</div>), which has been declared in when.
<div>
<p> Want to modify your personal information? </p>
</div
Like the template just stored in a separate "changeInfo.html" file, to achieve splitting. We can also modularize projects.
The effect is as follows
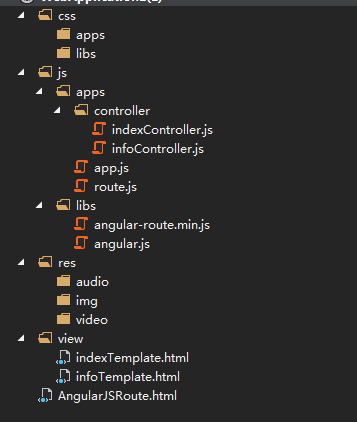
Let's look at the Angular JSRoute. HTML code
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" ng-app="app">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>AngularJS route</title>
</head>
<body>
<ul>
<li>
<a href="#Index > Home Page</a>
</li>
<li>
<a href="#Info "> Information</a>
</li>
</ul>
<!-- view -->
<div style="width: 300px; height: 300px; border: 1px #00bfff solid;" ng-view>
</div>
<script src="js/libs/angular.js"></script>
<script src="js/libs/angular-route.min.js"></script>
<!-- Initialization -->
<script src="js/apps/app.js"></script>
<!-- Routing configuration -->
<script src="js/apps/route.js"></script>
<!-- Controller -->
<script src="js/apps/controller/indexController.js"></script>
<script src="js/apps/controller/infoController.js"></script>
</body>
</html>
Next comes the routing route.js file
app.config(["$routeProvider", function ($routeProvider) {
$routeProvider
.when("/index",
{
templateUrl: "view/indexTemplate.html",
controller : "indexController"
})
.when("/info",
{
templateUrl: "view/infoTemplate.html",
controller: "infoController"
})
.otherwise({
redirectTo: "/index"
});
}]);
Operation effect
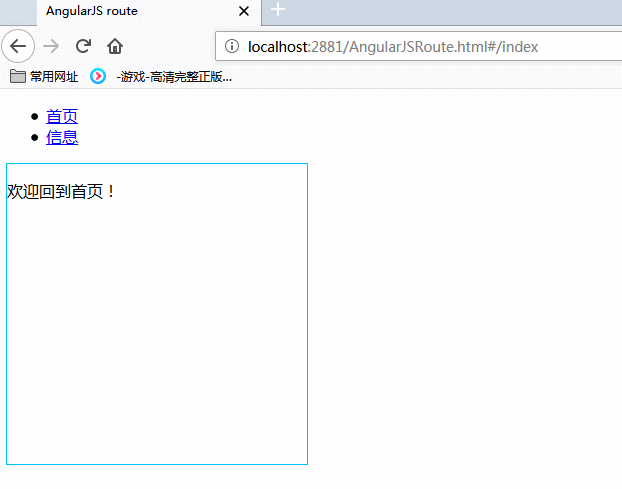
It has become very simple and suitable for team modular development. That's great!