Today, I uploaded the Demo I made in these two days to github and generated a sample project: Vue mobile Demo. I hope it can help the novice.
Let's briefly introduce the content of this project
Basic situation
This project is based on the van demo project, which adds many practical functions, involving Vue + van T + Axios + mockjs, etc. it is a good starting point for novice development. It includes four pages of homepage / application / query / setting, which are switched by the bottom navigation bar.
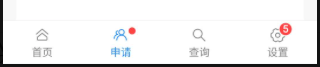
The application page consists of four stages, one master page and four components
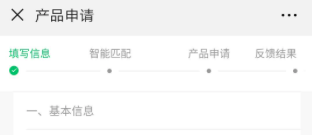
Functional characteristics
Design of single page components (Foot.vue, stored in components directory)
<template> <div class="footer"> <van-tabbar v-model="active"> <van-tabbar-item icon="home-o" to="home">home page</van-tabbar-item> <van-tabbar-item icon="friends-o" to="info" dot>Apply</van-tabbar-item> <van-tabbar-item icon="search" to="search">query</van-tabbar-item> <van-tabbar-item icon="setting-o" to="user" info="5">Set up</van-tabbar-item> </van-tabbar> </div> </template> <script> import { Tabbar, TabbarItem } from 'vant'; export default { name: 'apo-foot', components: { [Tabbar.name]: Tabbar, [TabbarItem.name]: TabbarItem }, data () { return { active: 0 } } } </script>
Progress process components
<template> <div class="info"> <div class="fixed"> <van-steps :active="active"> <van-step>Fill in information</van-step> <van-step>Intelligent matching</van-step> <van-step>Product application</van-step> <van-step>Feedback results</van-step> </van-steps> </div> <div class="step-mask"></div> <div :is="currentView"></div> <div class="van-hairline--top"></div> <van-row> <van-col offset="6" span="12"> <van-button type="primary" size="large" :text="buttonTip" @click="nextStep" ></van-button> </van-col> </van-row> </div> </template>
List components
<template> <div class="products"> <van-radio-group v-model="radio"> <van-list v-model="loading" :finished="finished" finished-text="No more" @load="onLoad"> <van-radio v-for="item in list" :key="item.id" :name="item.id" > <apo-cell :newsData="item" :key="item.id" /> </van-radio> </van-list> </van-radio-group> </div> </template>
Mock request
const Mock = require('mockjs'); const Random = Mock.Random; const produceNewsData = function() { let articles = []; for (let i = 0; i < 10; i++) { let newArticleObject = { id: i, title: Random.csentence(5, 30), thumbnail_pic_s: Random.dataImage('300x250', 'mock picture'), author_name: Random.cname(), date: Random.date() + ' ' + Random.time() } articles.push(newArticleObject) } return { articles: articles } } Mock.mock('/news/index', 'post', produceNewsData);
Axios request
import axios from 'axios' import vue from 'vue' axios.defaults.headers.post['Content-Type'] = 'application/x-www-form-urlencoded' axios.interceptors.request.use(function(config) { return config; }, function(error) { return Promise.reject(error); }) axios.interceptors.response.use(function(response) { return response; }, function(error) { return Promise.reject(error); }) export function fetch(url, params) { return new Promise((resolve, reject) => { axios.post(url, params) .then(response => { resolve(response.data); }) .catch((error) => { reject(error); }) }) } export default { getNews(url, params) { return fetch(url, params); } }
Cross domain request broker
devServer: { open: process.platform === 'darwin', host: '0.0.0.0', port: 8080, https: false, hotOnly: false, proxy: 'proxy_url' }
For more information, please refer to github project: https://github.com/SagittariusZhu/vue-mobile-demo