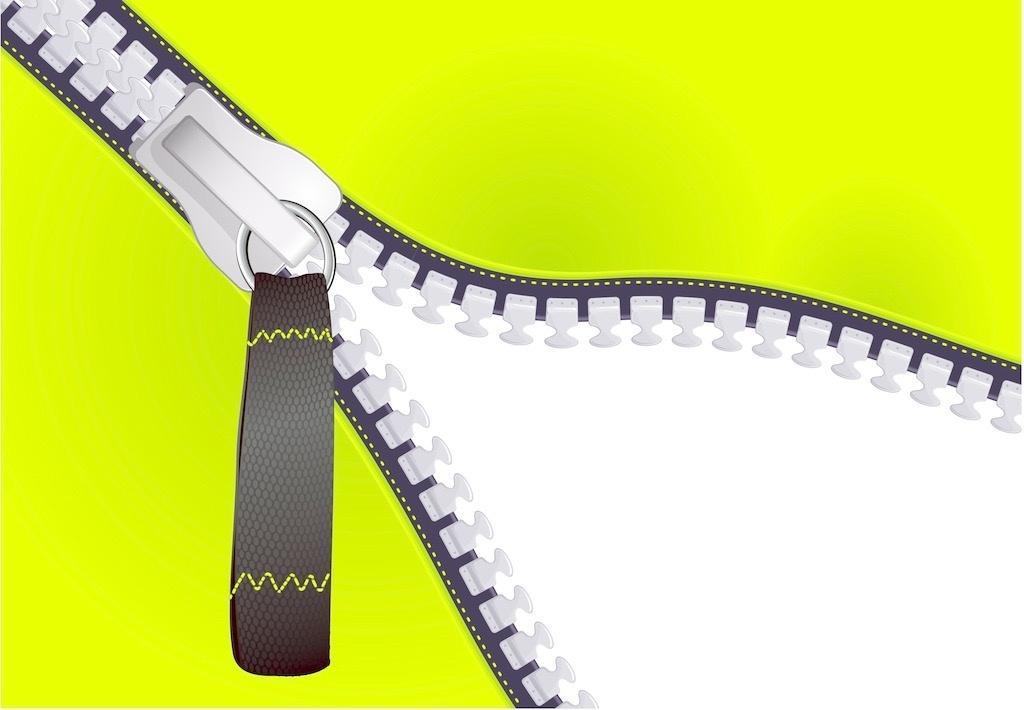
Links: https://leetcode.com/problems/merge-two-sorted-lists/#/description
Difficulty: Easy
Title: 21. Merge Two Sorted Lists
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists.
Merge two ordered links and return them as a new list. The new list should be joined together by splicing the nodes of the first two lists.
Idea 1: Create a new temporary list with header pointer pointing to 0, compare the current values of l1 and l2, point the next node of temporary list to smaller nodes, move the pointer of l1 or L2 back one bit, then move down one by one until l1 or L2 is empty, then point the next node of temporary list to the last non-empty list and return to the second node of temporary list (the first node is 0). .
Reference Code 1 (Java)
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode(int x) { val = x; } * } */ public class Solution { public ListNode mergeTwoLists(ListNode l1, ListNode l2) { ListNode newList = new ListNode(0); ListNode nextList = newList; while(l1 != null && l2 != null){ if(l1.val < l2.val){ nextList.next = l1; l1 = l1.next; }else{ nextList.next = l2; l2 = l2.next; } nextList = nextList.next; } if(l1 != null){ nextList.next = l1; }else{ nextList.next = l2; } return newList.next; } }
Idea 2: Use recursive method. A temporary list is constructed. When the value of l1 current node is greater than that of l2 current node, we assign the smaller value of l2 to the next node of the temporary list, and compare the value of l2 next node with that of l1 current node next time, and then recurse.
Reference Code 2 (Java)
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode(int x) { val = x; } * } */ public class Solution { public ListNode mergeTwoLists(ListNode l1, ListNode l2) { if (l1 == null) return l2; if (l2 == null) return l1; if (l1.val > l2.val) { ListNode tmp = l2; tmp.next = mergeTwoLists(l1, l2.next); return tmp; } else { ListNode tmp = l1; tmp.next = mergeTwoLists(l1.next, l2); return tmp; } } }