algorithm
Like the fast sort algorithm, the merge sort algorithm is based on the divide and conquer algorithm, which divides large-scale problems into smaller sub problems. The content of merging and sorting is to cut the table according to the midpoint, divide it into left and right sub tables, and then continue to divide the left and right sub tables until they cannot be divided. When it is impossible to partition (that is, both the left and right tables contain only one element), merge the left and right tables into an ordered table, and then continue to merge the left and right until the final overall order.
. However, the spatial complexity is o(n) because auxiliary tables are used in merging. In general, merging sorting is not as fast as merging sorting.
Example
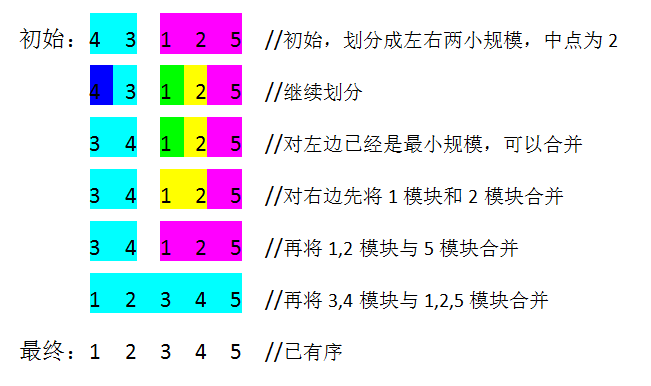
Codes
package com.fairy.InnerSort; import java.util.Scanner; /** * Merge sort * @author Fairy2016 * */ public class MergeSort { static int b[] = new int[100];//Auxiliary array, help merging public static void Merge(int a[], int low, int high, int mid) { int i,j,k; for(i = low; i <= high; i++) { b[i] = a[i]; } //Merge the first half and the second half of mid into an ordered table for(i = low, j = mid + 1, k = i; i <= mid && j <= high; k++) { //Who wants who if(b[i] < b[j]) { a[k] = b[i++]; } else { a[k] = b[j++]; } } //The two parts may not be the same length, redundant direct assignment while(i <= mid) { a[k++] = b[i++]; } while(j <= high) { a[k++] = b[j++]; } } public static void sort(int a[], int low, int high) { if(low < high) { //Divide and conquer algorithm, which is divided into smaller left and right ordered tables, and the two are combined int mid = (low + high)/2; sort(a, low, mid); sort(a, mid+1, high); Merge(a, low, high, mid); } } public static void Print(int a[], int n) { for(int i = 1; i <= n; i++) { System.out.print(a[i]+" "); } } public static void main(String args[]) { int n; int a[]; Scanner scanner = new Scanner(System.in); while(scanner.hasNext()) { n = scanner.nextInt(); if(n > 0) { a = new int[n+1]; for(int i=1; i <= n; i++) { a[i] = scanner.nextInt(); } sort(a, 1, n); Print(a, n); } } scanner.close(); } }