Git has dozens of hundreds of commands, but we often use only a few basic commands. If you want to be proficient in using git, you need to remember many commands
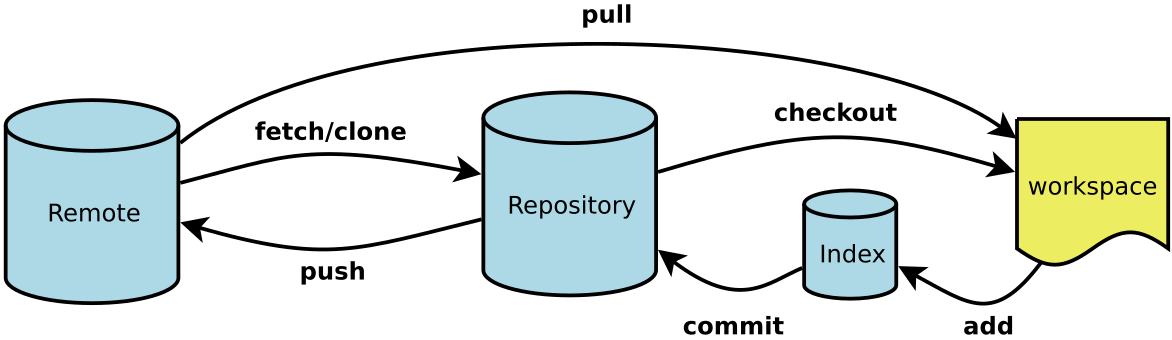
Common basic 6 commands
Workspace: workspace
Index / Stage: staging area
Repository: warehouse area (or local warehouse)
Remote: remote warehouse
1, New code base
# Create a Git code base in the current directory $ git init # Create a new directory and initialize it as Git code base $ git init [project-name] # Download a project and its entire code history $ git clone [url]
2, Configuration
Git's settings file is. gitconfig, which can be in the user's home directory (global configuration) or in the project directory (project configuration).
# Show current Git configuration $ git config --list # Edit Git profile $ git config -e [--global] # Set user information when submitting code $ git config [--global] user.name "[name]" $ git config [--global] user.email "[email address]"
3, Add / remove files
# Add specified file to staging area $ git add [file1] [file2] ... # Add the specified directory to the staging area, including subdirectories $ git add [dir] # Add all files from the current directory to the staging area $ git add . # Confirmation is required before each change is added # For multiple changes of the same file, multiple submissions can be realized $ git add -p # Delete the workspace file and put this deletion in the staging area $ git rm [file1] [file2] ... # Stops tracking the specified file, but it remains in the workspace $ git rm --cached [file] # Rename the file and put it in the staging area $ git mv [file-original] [file-renamed]
4, Code submission
# Submit staging area to warehouse area $ git commit -m [message] # Submit the specified file of staging area to the warehouse area $ git commit [file1] [file2] ... -m [message] # Commit workspace changes since last commit, directly to warehouse $ git commit -a # Show all diff information on submit $ git commit -v # Replace the last commit with a new commit # If there is no new change in the code, it is used to overwrite the commit information of the last commit $ git commit --amend -m [message] # Redo the last commit and include new changes to the specified file $ git commit --amend [file1] [file2] ...
5, Branch
# List all local branches $ git branch # List all remote branches $ git branch -r # List all local and remote branches $ git branch -a # Create a new branch, but still stay in the current branch $ git branch [branch-name] # Create a new branch and switch to it $ git checkout -b [branch] # Create a new branch pointing to the specified commit $ git branch [branch] [commit] # Create a new branch and establish a tracking relationship with the specified remote branch $ git branch --track [branch] [remote-branch] # Switch to the specified branch and update the workspace $ git checkout [branch-name] # Switch to previous branch $ git checkout - # Establish a trace relationship between an existing branch and a specified remote branch $ git branch --set-upstream [branch] [remote-branch] # Merge the specified branch to the current branch $ git merge [branch] # Select a commit to merge into the current branch $ git cherry-pick [commit] # Delete branch $ git branch -d [branch-name] # Delete remote branch $ git push origin --delete [branch-name] $ git branch -dr [remote/branch]
6, Label
# List all tag s $ git tag # Create a new tag in the current commit $ git tag [tag] # Create a new tag in the specified commit $ git tag [tag] [commit] # Delete local tag $ git tag -d [tag] # Delete remote tag $ git push origin :refs/tags/[tagName] # View tag information $ git show [tag] # Submit specified tag $ git push [remote] [tag] # Submit all tag s $ git push [remote] --tags # Create a new branch pointing to a tag $ git checkout -b [branch] [tag]
7, View information
# Show changed documents $ git status # Displays the version history of the current branch $ git log # Show the commit history and the files that change every commit $ git log --stat # Search submission history by keyword $ git log -S [keyword] # Show all changes after a commit, one line for each commit $ git log [tag] HEAD --pretty=format:%s # Display all changes after a commit, and its "submission description" must meet the search criteria $ git log [tag] HEAD --grep feature # Display the version history of a file, including file rename $ git log --follow [file] $ git whatchanged [file] # Display every diff related to the specified file $ git log -p [file] # Show last 5 submissions $ git log -5 --pretty --oneline # Display all submitted users, sorted by submission times $ git shortlog -sn # Show who modified the specified file at what time $ git blame [file] # Show differences between staging and workspace $ git diff # Show differences between staging area and previous commit $ git diff --cached [file] # Shows the difference between the workspace and the latest commit for the current branch $ git diff HEAD # Show differences between two submissions $ git diff [first-branch]...[second-branch] # Show how many lines of code you wrote today $ git diff --shortstat "@{0 day ago}" # Show metadata and content changes for a submission $ git show [commit] # Show files that have changed in a submission $ git show --name-only [commit] # Displays the contents of a file at the time of a submission $ git show [commit]:[filename] # Shows the most recent commits for the current branch $ git reflog
8, Remote synchronization
# Download all changes to remote warehouse $ git fetch [remote] # Show all remote warehouses $ git remote -v # Display information about a remote warehouse $ git remote show [remote] # Add a new remote warehouse and name it $ git remote add [shortname] [url] # Retrieve changes from remote warehouse and merge with local branch $ git pull [remote] [branch] # Upload local designated branch to remote warehouse $ git push [remote] [branch] # Force the current branch to a remote warehouse, even if there is a conflict $ git push [remote] --force # Push all branches to remote warehouse $ git push [remote] --all
9, Undo
# Recover the specified files from the staging area to the workspace $ git checkout [file] # Recover the specified file of a commit to the staging area and workspace $ git checkout [commit] [file] # Recover all files from staging area to workspace $ git checkout . # Resets the specified file for the staging area, consistent with the last commit, but the workspace does not change $ git reset [file] # Reset staging area and workspace to match last commit $ git reset --hard # Resets the pointer of the current branch to the specified commit, and resets the staging area, but the workspace does not change $ git reset [commit] # Reset the HEAD of the current branch to the specified commit, and reset the staging area and workspace at the same time, consistent with the specified commit $ git reset --hard [commit] # Reset the current HEAD to the specified commit, but leave the staging area and workspace unchanged $ git reset --keep [commit] # Create a new commit to undo the specified commit # All changes in the latter are offset by the former and applied to the current branch $ git revert [commit] # Temporarily remove uncommitted changes and move in later $ git stash $ git stash pop
10, Others
# Generate a compressed package for publishing $ git archive
Reference link
Git branch management strategy - Ruan Yifeng