list interface
The list interface in the standard STL library is as follows:
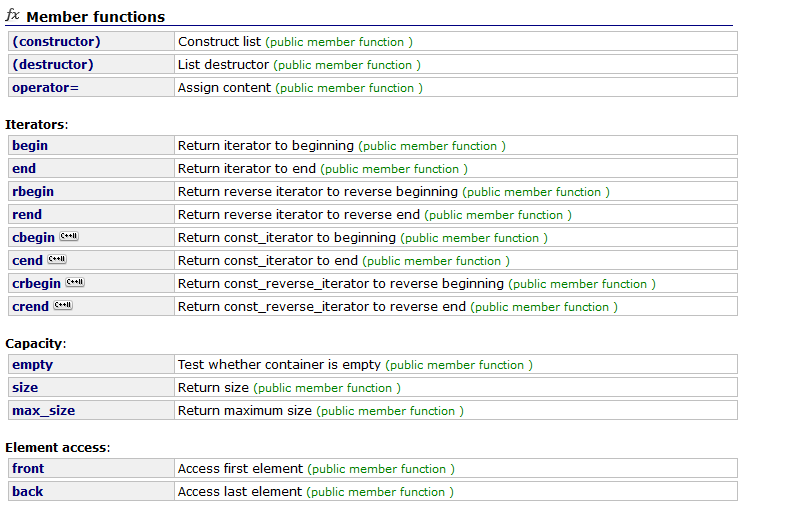

List is part of the C++ Standard Template Library. In fact, the list container is a two-way linked list that can efficiently insert and delete elements.
Before using list container, you must add < vector > header file: # include < list >;
List belongs to the content of STD naming domain, so it needs to be qualified by naming: using std::list; global namespace can also be used directly: using namespace std;
1. Constructor, copy constructor
int main()
{
list<int> l1; //Empty list
list<int> l2(3); //Create a list of elements with three default values
list<int> l3(5,2); //Create a linked list of five elements with all values
list<int> l4(l3); //Create a copy list
list<int> l5(++l3.begin(),--l3.end()); //// c5 contains elements of a region of c1 [_First, _Last].
list<int>::iterator it1;//Use iterators to traverse elements in l1 objects
list<int>::iterator it2;//Use iterators to traverse elements in l2 objects
list<int>::iterator it3;//Use iterators to traverse elements in l3 objects
list<int>::iterator it4;//Use iterators to traverse elements in l4 objects
list<int>::iterator it5;//Use iterators to traverse elements in l5 objects
it1 = l1.begin();
while (it1 != l1.end())
{
cout << *it1 << " ";
++it1;
}
cout << endl;
it2 = l2.begin();
while (it2 != l2.end())
{
cout << *it2 << " ";
++it2;
}
cout << endl;
it3 = l3.begin();
while (it3 != l3.end())
{
cout << *it3 << " ";
++it3;
}
cout << endl;
it4 = l4.begin();
while (it4 != l4.end())
{
cout << *it4 << " ";
++it4;
}
cout << endl;
it5 = l5.begin();
while (it5 != l5.end())
{
cout << *it5 << " ";
++it5;
}
cout << endl;
return 0;
}
2. Iterator member functions
begin() Returns an iterator pointing to the first element of the list.
end() returns an iterator that points to the last element in the list.
c.begin() Returns an iterator pointing to the first element of the list. (c++11 standard)
c.end() returns an iterator that points to the last element in the list. (c++11 standard)
it4 = l4.begin(); while (it4 != l4.end()) { *it4 = 1; cout << *it4 << " "; ++it4; } cout << endl; The results show that: 1 > f: program stl interface stl interface source. cpp(35): error C3892: it4: constants cannot be assigned
rbegin() returns the first element of the reverse list, the last data of the c-linked list.
rend() returns the next position of the last element of the reverse list, that is, the first data of the c-linked list goes forward.
The result is 4321.void Test1() { list<int>l1; l1.push_back(1); l1.push_back(2); l1.push_back(3); l1.push_back(4); list<int>::reverse_iterator it = l1.rbegin(); while (it != l1.rend()) { cout << *it << " "; ++it; } }
c.rbegin() returns the first element of the reverse list, the last data of the C list. (c++11)
c.rend() returns the next position of the last element of the reverse list, that is, the first data of the C-linked list moves forward. (c++11)
Similar to rbegin() and rend() are just not assignable.
3.Capacity: Some Approaches to Capacity
empty();
bool empty() const
size();
size_type size() const
max_size():
size_type max_size() const
resize(size_type new_size, const T& x);// New size and assign the last number x
resize(size_type new_size);//New size
void Test2()
{
list<int>l1(3);
cout << l1.empty()<<endl;//Judging that the list is empty
cout << l1.size()<<endl;//Number of nodes
cout << l1.max_size()<<endl; //Return
l1.resize(5);// Make the list a node
cout << l1.size() << endl;
l1.resize(6, 6);//Turning a linked list into a node with a value
cout << l1.size() << endl;
list<int>::iterator it5;
it5 = l1.begin();
while (it5 != l1.end())
{
cout << *it5 << " ";
++it5;
}
cout << endl;
}
4.element access
reference front() ;// Return * begin()
const_reference front() const ;
reference back() ; //Return *(- end())
const_reference back() const ;
5.push_back pop_back push_front pop_front
void Test3() { // push_back pop_back list<int> l4; l4.push_back(1); l4.push_back(2); l4.push_back(3); l4.push_back(4); l4.pop_back(); l4.pop_back(); l4.pop_back(); list<int>::iterator it1=l4.begin(); while (it1!=l4.end()) { cout<<*it1<<" "; ++it1; } cout<<endl; //push_front pop_front list<int> l5; l5.push_front(5); l5.push_front(6); l5.push_front(7); l5.pop_back(); l5.pop_back(); list<int>::iterator it2=l5.begin(); while (it2!=l5.end()) { cout<<*it2<<" "; ++it2; } cout<<endl; }
6.insert erase clear swap
Iterator insert (iterator position, const T&x); insert element num at position.
Insert (iterator position, size_type n, const T&x); // insert n elements num at position
Insert (iterator position, Input Iterator first, Input Iterator last); // Insert elements with intervals [first, last] in position
Iterator erase(iterator position); //delete position
Iterator erase(iterator first, iterator last); //delete elements with intervals [first,last)
void Test4() { list<int>l1; list<int>l2; list<int>l3; l1.insert(l1.begin(), 1); l2.insert(l2.begin(), 8, 2); l3.insert(l3.begin(), ++l2.begin(), --l2.end()); list<int>::iterator it1; it1 =l1.begin(); while (it1 !=l1.end()) { cout << *it1 << " "; ++it1; } cout << endl; list<int>::iterator it2; it2 = l2.begin(); while (it2 != l2.end()) { cout << *it2 << " "; ++it2; } cout << endl; list<int>::iterator it3; it3= l3.begin(); while (it3 != l3.end()) { cout << *it3 << " "; ++it3; } cout << endl; //erase l2.erase(l2.begin()); l3.erase(++l3.begin(), l3.end()); list<int>::iterator it4; it4= l2.begin(); while (it4 != l2.end()) { cout << *it4 << " "; ++it4; } cout << endl; list<int>::iterator it5; it5= l3.begin(); while (it5 != l3.end()) { cout << *it5 << " "; ++it5; } cout << endl; //clear l3.clear(); list<int>::iterator it6; it6= l3.begin(); while (it6 != l3.end()) { cout << *it6 << " "; ++it6; } cout << endl; //swap l1.swap(l2); list<int>::iterator it7; it7= l2.begin(); while (it7 != l2.end()) { cout << *it7 << " "; ++it7; } cout << endl; list<int>::iterator it8; it8= l1.begin(); while (it8 != l1.end()) { cout << *it8 << " "; ++it8; } cout << endl; }
7.splice
void Test5() { list<int> l1; list<int> l2; list<int> l3(l2); l1.insert(l1.begin(),5,8); l2.insert(l2.begin(),9,10); l1.splice(l1.begin(),l2); //Connect l2 to the begin position of l1 and release l2 l3.splice(l1.begin(),l2,l2.begin(),l2.end());// Connect the element in the [begin, end] position of L2 to the begin position of l1 and release the element in the [begin, end] position of l2. list<int>::iterator it1; it1 =l1.begin(); while (it1 !=l1.end()) { cout << *it1 << " "; ++it1; } cout << endl; list<int>::iterator it2; it2 = l2.begin(); while (it2 != l2.end()) { cout << *it2 << " "; ++it2; } cout << endl; list<int>::iterator it3; it3 =l1.begin(); while (it3 !=l1.end()) { cout << *it3 << " "; ++it3; } cout << endl; }
8.sort and unique
9.mergevoid Test6() { //sort list<int> l1; l1.push_back(1); l1.push_back(2); l1.push_back(5); l1.push_back(4); l1.push_back(5); l1.push_back(4); l1.push_back(3); l1.sort(); list<int>::iterator it1; it1 =l1.begin(); while (it1 !=l1.end()) { cout << *it1 << " "; ++it1; } cout << endl; //reverse l1.reverse(); list<int>::iterator it2; it2 = l1.begin(); while (it2 != l1.end()) { cout << *it2 << " "; ++it2; } cout << endl; //unique l1.unique(); list<int>::iterator it3; it3 = l1.begin(); while (it3 != l1.end()) { cout << *it3 << " "; ++it3; } cout << endl;}
void Test7() { //void merge(list<T, Allocator>& x); list<int>l1(2, 1); list<int>l2(2, 2); l1.merge(l2);//Merge all elements of l2 into l1 list<int>::iterator it1 = l1.begin(); while (it1 != l1.end()) { cout << *it1 << " "; it1++; } cout << endl;}
10.remove
void Test8() { list<int> l1(5,1); l1.remove(1);//Delete data with node 1 list<int>::iterator it1 = l1.begin(); while (it1 != l1.end()) { cout << *it1 << " "; it1++; } cout << endl; }
11. Operator overloading
operator==
operator!=
operator<
operator<=
operator>
operator>=