Overview of TCP
TCP (Transmission Control Protocol) is a connection-oriented, reliable, byte-stream-based transport layer communication protocol.
TCP has the following characteristics:
1) Abstraction of Service Model of Telephone System
2) Every complete data transmission must go through the process of establishing connection, using connection and terminating connection.
3) reliable

To ensure the reliability of data transmission, the error retransmitting and the corresponding confirmation should be given for each data received.
C/S Architecture of TCP Programming
The development of network programming based on TCP is divided into two parts: server side and client side. The common core steps and processes are as follows:
3. TCP Client Programming
For the TCP client programming process, it is a bit similar to the call process:
1. Find a cell phone to talk to (socket()
2. Dial the other party's number and make sure that the other party is the person you are looking for.
3. Active chat (send() or write()
4. Or, receive a call from the other party (recv() or read())
5. After the communication, the two sides said goodbye and hung up (close()
Required header file: #include <sys/socket.h>
int socket(int family,int type,int protocol);
Functions:
Create a socket for network communication
socket (Descriptor)
Detailed usage, please see "socket" word
Introduction.
Parameters:
family: This example writes AF_INET, representing IPv4
type: This example writes SOCK_STREAM, representing TCP data streams
Protocol: Write 0 here, set to 0 to indicate the default protocol
Return value:
Failure < 0
int connect( int sockfd, const struct sockaddr *addr, socklen_t
len );
Functions:
Actively establishing a connection with the server is somewhat similar. We call people and actively dial their phone number. What is the process, please?<The relationship among connect(), listen() and accept()>.
Parameters:
sockfd: Socket returned by socket()
addr: Connected server address structure
len: Address structure length
Return value:
Success: 0
Failure: -1
The connect() function is equivalent to dialing a number, only dialing the number and making sure that the other party is the person you are looking for.( The three handshake ) In order to carry out the next communication.
ssize_t send(int sockfd, const void* buf, size_t
nbytes, int flags);
Functions:
To send data, when the last parameter is 0, write() can be used instead (send is equivalent to write). Note: TCP protocol cannot be used to send 0-length packets. If the data is not sent successfully, the kernel will automatically resend it.
Parameters:
sockfd: Connected socket
buf: Address to send data
nbytes: Size of slow data sent (in bytes)
flags: Socket flag (often 0)
Return value:
Success: Number of bytes sent successfully
Failure < 0
Here we communicate with the ubuntu client program in the virtual machine through the network debugging assistant of Windows. Network Debugging Assistant Download Please Click Access Password
d5f3.
Windows network debugging assistant serves as TCP server and receives requests from clients. The configuration of debugging assistant is as follows:
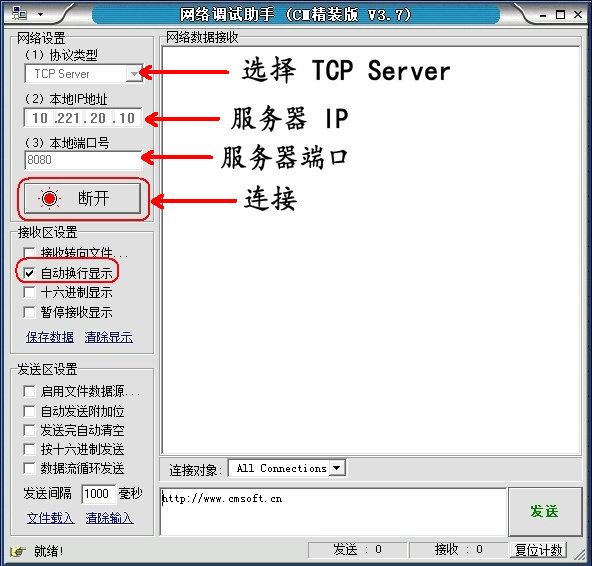
3.1 - Example 1: tcp client sends data: Running in code ubuntu
-
#include <stdio.h>
-
#include <unistd.h>
-
#include <string.h>
-
#include <stdlib.h>
-
#include <arpa/inet.h>
-
#include <sys/socket.h>
-
#include <netinet/in.h>
-
int main(int argc, charchar *argv[])
-
{
-
unsigned short port = 8080;
-
charchar *server_ip = "10.221.20.24";
-
-
if( argc > 1 )
-
{
-
server_ip = argv[1];
-
}
-
if( argc > 2 )
-
{
-
port = atoi(argv[2]);
-
}
-
-
int sockfd;
-
sockfd = socket(AF_INET, SOCK_STREAM, 0);
-
if(sockfd < 0)
-
{
-
perror("socket");
-
exit(-1);
-
}
-
-
-
struct sockaddr_in server_addr;
-
bzero(&server_addr,sizeof(server_addr));
-
server_addr.sin_family = AF_INET;
-
server_addr.sin_port = htons(port);
-
inet_pton(AF_INET, server_ip, &server_addr.sin_addr.s_addr);
-
-
-
int err_log = connect(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
-
if(err_log != 0)
-
{
-
perror("connect");
-
close(sockfd);
-
exit(-1);
-
}
-
-
char send_buf[512] = {0};
-
printf("send data to %s:%d\n",server_ip,port);
-
while(1)
-
{
-
printf("send:");
-
fgets(send_buf,sizeof(send_buf),stdin);
-
send_buf[strlen(send_buf)-1]='\0';
-
send(sockfd, send_buf, strlen(send_buf), 0);
-
}
-
-
close(sockfd);
-
-
return 0;
-
}
Operation results:
3.2 - Example 2: The tcp client receives data and the code runs under ubuntu
recv() is used to receive data from tcp.
ssize_t recv(int sockfd, void *buf, size_t
nbytes, int flags);
Functions:
Receive network data. By default, if no data is received, this function will block until data arrives.
Parameters:
sockfd: socket
buf: the address of the buffer that receives network data
nbytes: The size of the receiving buffer (in bytes)
flags: socket flag (often 0)
Return value:
Success: Number of bytes successfully received
Failure < 0
test The code is as follows:
-
#include <stdio.h>
-
#include <unistd.h>
-
#include <string.h>
-
#include <stdlib.h>
-
#include <arpa/inet.h>
-
#include <sys/socket.h>
-
#include <netinet/in.h>
-
int main(int argc, charchar *argv[])
-
{
-
unsigned short port = 8080;
-
charchar *server_ip = "10.221.20.24";
-
-
if( argc > 1 )
-
{
-
server_ip = argv[1];
-
}
-
if( argc > 2 )
-
{
-
port = atoi(argv[2]);
-
}
-
-
int sockfd;
-
sockfd = socket(AF_INET, SOCK_STREAM, 0);
-
if(sockfd < 0)
-
{
-
perror("socket");
-
exit(-1);
-
}
-
-
-
struct sockaddr_in server_addr;
-
bzero(&server_addr,sizeof(server_addr));
-
server_addr.sin_family = AF_INET;
-
server_addr.sin_port = htons(port);
-
-
server_addr.sin_addr.s_addr = inet_addr(server_ip);
-
-
-
int err_log = connect(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
-
if(err_log != 0)
-
{
-
perror("connect");
-
close(sockfd);
-
exit(-1);
-
}
-
-
-
printf("send data to %s:%d\n",server_ip,port);
-
-
char send_buf[512] = "this is send data";
-
printf("send data \"%s \" to %s:%d\n",send_buf,server_ip,port);
-
send(sockfd, send_buf, strlen(send_buf), 0);
-
-
char recv_buf[512] = {0};
-
recv(sockfd, recv_buf, sizeof(send_buf), 0);
-
printf("%s\n", recv_buf);
-
-
close(sockfd);
-
-
return 0;
-
}
Operation results:
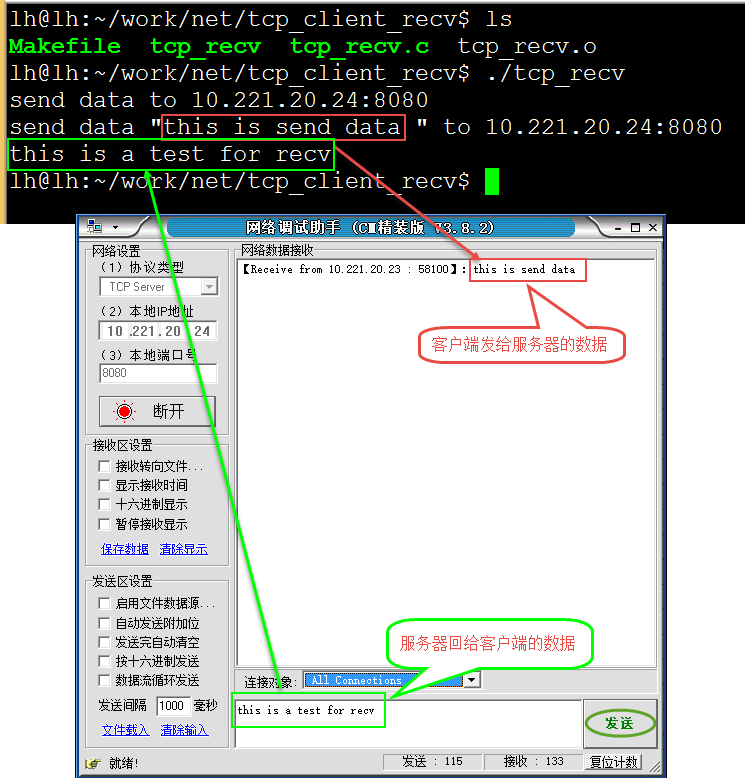