In the last few sections, we implemented the basic linked list structure, and at the bottom of the previous section we gave the source code of the linked list. Let's stick it up here once, poke it hard.
Before we start the implementation of the stack, let's look at the addition, deletion and lookup operations of the linked list only in the head. The time complexity is O(1). Based on these advantages of the linked list, we implement the stack on this basis.
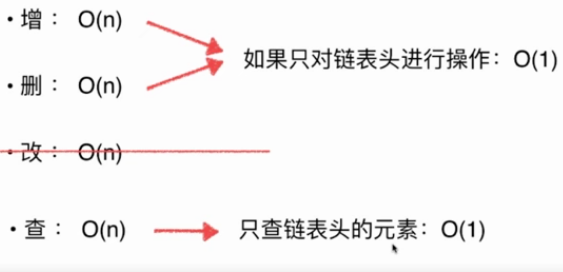
Preface: Before writing this section, we have implemented a stack based on static arrays. Go to view . Here we implement a linked list-based stack.
1. Copy the linked list class to the Stack package:
When implementing a stack based on static arrays, we have created a new package. At this time, we copy the linked list classes we have implemented into the package. The directory structure is as follows:
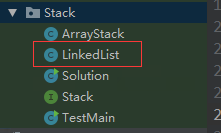
2. implementation stack
A new LinkedListStack class is created to implement the Stack interface. The relevant code is as follows:
Stack interface:
package Stack; public interface Stack<E> { //Number of elements in stack int getSize(); //Is the number of elements in the stack empty? boolean isEmpty(); //Enter stack void push(E e); //Stack out E pop(); //View stack top elements E peek(); }
LinkedListStack class:
package Stack; public class LinkedListStack<E> implements Stack<E> { private LinkedList<E> list; public LinkedListStack() { list = new LinkedList<E>(); } //Number of elements in stack @Override public int getSize() { return list.getSize(); } //Is the stack empty? @Override public boolean isEmpty() { return list.isEmpty(); } //Adding elements to the stack @Override public void push(E e) { list.addFirst(e); } //Delete the first element from the stack @Override public E pop() { return list.removeFirst(); } //View the first element in the stack @Override public E peek() { return list.getFirst(); } //Mainly for easy output to object information @Override public String toString() { StringBuilder res = new StringBuilder(); res.append("Stack: top "); res.append(list); return res.toString(); } }
3. Add test code
In order to simplify the test, we have established the main() method in the LinkedListStack class, which has implemented the Stack interface. The code in main is as follows:
//test public static void main(String[] args) { LinkedListStack<Integer> stack = new LinkedListStack<Integer>(); for (int i = 0; i < 5; i++) { stack.push(i); System.out.println(stack); } System.out.println("Out of the stack an element:"); stack.pop(); System.out.println(stack); }
The result is:
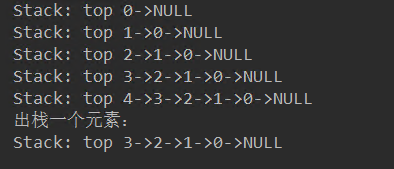
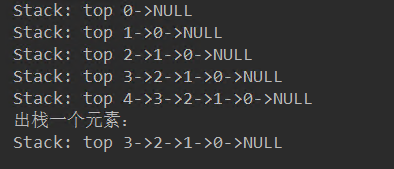
About this section, if you think it's okay and passable, remember to give a recommendation, oh ~, thank you!!