Two way linked list
1, Double linked list structure
Node structure of double linked list
typedef struct DualNode { ElemType data; struct DualNode *prior; //Precursor node struct DualNode *next; //Successor node } DualNode, *DuLinkList;

Since a single linked list can have a circular linked list, a two-way linked list can also have one.
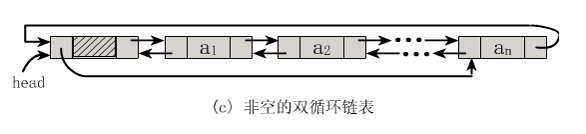
Since this is a two-way linked list, for a certain node p in the linked list, its predecessor node of its successor node is itself.
2, Insert operation of double linked list
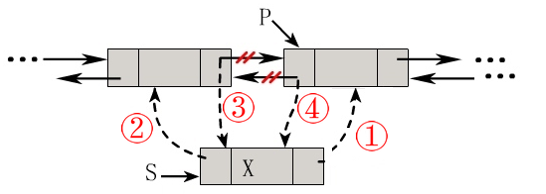
Code implementation:
s->next = p; s->prior = p->prior; p->prior->next = s; p->prior = s;
The key is to avoid conflicts during the exchange process. For example, if the fourth step is executed first, then p - > prior will become s in advance, making the insertion work wrong.
3, Delete operation of double linked list
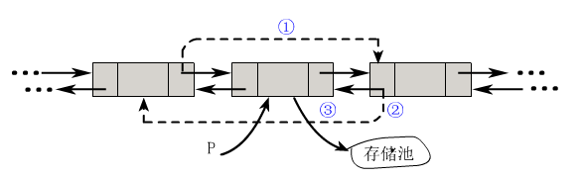
Code implementation:
p->prior->next = p->next; p->next->prior = p->prior; free(p);
Bidirectional linked list can effectively improve the time performance of the algorithm, that is to say, space is used in exchange for time.
4, Practice of double linked list
It is required to input a number to make the arrangement of 26 letters change, for example, user input 3, output result:
DEFGHIJKLMNOPQRSTUVWXYZABC
At the same time, it needs to support negative numbers, such as user input-3 and output results:
XYZABCDEFGHIJKLMNOPQRSTUVW
Code implementation:
#include <stdio.h> #include <stdlib.h> #define OK 1 #define ERROR 0 typedef char ElemType; typedef int Status; typedef struct DualNode{ ElemType data; struct DualNode *prior; struct DualNode *next; }DualNode,*DuLinkList; Status InitList(DuLinkList *L){ DualNode *p,*q; int i; *L = (DuLinkList)malloc(sizeof(DualNode)); if(!(*L)){ return ERROR; } (*L)->next = (*L)->prior = NULL; p = (*L); for(i=0;i<26;i++){ q = (DualNode *)malloc(sizeof(DualNode)); if(!q){ return ERROR; } q->data = 'A'+i; q->prior = p; q->next = p->next; p = q; } p->next = (*L)->next; (*L)->next->prior = p; return OK; } void Caesar(DuLinkList *L,int i){ if(i>0){ do{ (*L) = (*L)->next; }while(--i); } if(i<0){ do{ (*L) = (*L)->next; }while(++i); } } int main(){ DuLinkList L; int i,n; InitList(&L); printf("Please enter an integer:"); scanf("%d",&n); printf("\n"); Caesar(&L,n); for(i=0;i<26;i++){ L = L->next; printf("%c",L->data); } printf("\n"); return 0; }