Question:
Given a binary tree, find the leftmost value in the last row of the tree. Example 1: Input:

Output: 1 Example 2: Input:
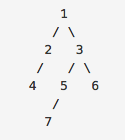
Output: 7 Note: You may assume the tree (i.e., the given root node) is not NULL.
General idea:
Give a binary tree and find the leftmost node value in the bottom row of the tree. Example 1: Input:

Output: 1 Example 2: Input:
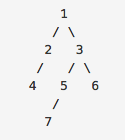
Output: 7 Note: you can assume that the tree (given the root node, for example) is not empty.
Idea:
This question can actually be broken down into two questions:
- Find the bottom row of the binary tree;
- Find the leftmost node value in the bottom row.
It should be noted that the leftmost node value is not necessarily a left node, but may also be a right child node value on the leftmost.
Remember when we were Portal: LeetCode note: 102. Binary Tree Level Order Traversal In, it is required to output the binary tree layer by layer. In the same way, we use BFS breadth first traversal method and queue to ensure that all node values of the lowest layer are found. Then, we only need to use a tag to record the value of the leftmost node every time we comb the nodes of one layer. In this way, when it is determined that it is the last layer and there is no next layer, What we record is the leftmost node value of the lowest layer.
Code (Java):
/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */ public class Solution { public int findBottomLeftValue(TreeNode root) { Queue<TreeNode> queue = new LinkedList<TreeNode>(); queue.offer(root); int result = root.val; boolean has = false; while (!queue.isEmpty()) { int levelNum = queue.size(); for (int i = 0; i < levelNum; i++) { if (queue.peek().left != null) { queue.offer(queue.peek().left); if (!has) { result = queue.peek().left.val; has = true; } } if (queue.peek().right != null) { queue.offer(queue.peek().right); if (!has) { result = queue.peek().right.val; has = true; } } queue.poll(); } has = false; } return result; } }
outside the box:
My method is actually slow. Let's take a look at the following method:
public class Solution { public int findBottomLeftValue(TreeNode root) { return findBottomLeftValue(root, 1, new int[]{0,0}); } public int findBottomLeftValue(TreeNode root, int depth, int[] res) { if (res[1]<depth) {res[0]=root.val;res[1]=depth;} if (root.left!=null) findBottomLeftValue(root.left, depth+1, res); if (root.right!=null) findBottomLeftValue(root.right, depth+1, res); return res[0]; } }
The first advantage of this method is that the code is much simpler than me... In fact, his method is similar to my first idea. He uses DFS to pass it back and look down, and records the depth of the currently found node. He uses an int array res. the first element of the array records the node value, and the second element records the depth of the node. Only when you enter a deeper layer and the node value has not been recorded in this layer, record the first node value found, which is actually the leftmost node value. After finding it, mark the depth as the current depth, and then all the node values found later will not be recorded unless a deeper node is found. In this way, keep updating the found node values according to the depth, and finally find the leftmost node value of the deepest layer.
Collection: https://github.com/Cloudox/LeetCode-Record