Description of the problem: Write a function to find the longest common prefix string amongst an array of strings.
Analysis:
The title is to find the longest prefix of a set of strings, such as {"abcd", "abdf","abf"}. The first two "ab" of the three strings are the same, and the longest prefix is ab.
Disintegration idea: The longest identical prefix must be less than or equal to the shortest string length, so first find the shortest string and use it as the object of comparison. If the string on a bit is different from the string being compared, the comparison ends, and the character before the bit of the string being compared is the longest identical prefix of the string group.
Two special situations need to be considered in this question:
(1) The string group is empty. At this point, return directly to "".
(2) A string group has only one string. In this case, the longest prefix is the string itself.
Code:
#include <stdio.h> #include <string.h> #include <stdlib.h> char* longestCommonPrefix(char** strs, int strsSize); int main() { char * strs[] = { "abc","abd" }; int strsize = sizeof(strs) / sizeof(char *); printf("strsize=%d\n", strsize); char *CommonPrefix; //char **str = &strs[0]; int i=0; for ( i = 0; i < strsize;i++) { printf("strsize[%d]=%d\n",i, strlen(strs[i])); printf("strs[%d]=%s\n", i, strs[i]); } CommonPrefix=longestCommonPrefix(strs,strsize); printf("longestCommonPrefix=%s\n",CommonPrefix); system("pause"); return 0; } char* longestCommonPrefix(char** strs, int strsSize) { if (strs == NULL || *strs == NULL) { return ""; } if (strsSize==1) return *strs; int i,j,x=0; int minprefix=strlen(strs[0]); //strs is a secondary pointer, that is, a pointer. Its essence is a pointer array, that is, an array whose elements are pointers. The array name represents the address of the first element of the array, and the first element is a pointer. Therefore, the array name of the pointer array is a secondary pointer. char temp; char *result; for(i=1;i<strsSize;i++) //Find the shortest string and record its length { if(minprefix>strlen(strs[i])) { minprefix=strlen(strs[i]); x=i; } } for (j=0;j<minprefix;j++) //Use the shortest string length as the upper limit of comparison digits { temp=*(strs[x]+j); //Baseline the shortest string for(i=0;i<strsSize;i++) { if(*(strs[i]+j)!=temp) //If one character is found to be different, the comparison ends break; } if(i!=strsSize) //At least two corresponding bits in each string are different, ending the loop break; } if(j>=0) { result = (char* ) malloc(sizeof(char) *(j+1)); strncpy(result,strs[x],j+1); //Return the same first j bit result[j]='\0'; } return result; }
char* longestCommonPrefix(char** strs, int strsSize) { if (strs == NULL || *strs == NULL) { return ""; } int len = strlen(strs[0]); int max = 0; char *res; while (max < len) { for (int i = 1; i < strsSize; i++) { if (strs[i][max] != strs[0][max]) //Compare bit by bit until different bits are found { len = max; break; } } if (max < len) max++; //Discovery of dislocation } res = (char*)malloc(sizeof(char)*(max+1)); for (int i = 0; i < max; i++) //Return the same pre-max bit { res[i] = strs[0][i]; } res[max] = '\0'; return res; }
Secondary Pointer and Two-Dimensional Array
char *string[] ={"abc","abcd","acf"};
char string[3][4]={"abc","abcd","acf"};
First of all, although the array name of a two-dimensional array can be regarded as a pointer, it is not possible to assign the array name of a two-dimensional array to a second-level pointer, that is, the following code
int main(void) { int arr[3][3] = {{1,2,3},{4,5,6},{7,8,9}}; int **p = arr; return 0; }
The above code will report an error when it is executed, because the data types of the two are different, so they cannot be assigned.
The array name of a two-dimensional array points to a one-dimensional array, that is, to the array type, but the second-level pointer points to a first-level pointer, that is, to the pointer type, so they cannot assign values to each other.
The relationship between pointers and two-dimensional arrays is described in detail below.
Declare a two-dimensional array
int a[3][4] = {{1,2,3,4},{5,6,7,8},{9,10,11,12}}; for (i=0;i<3;i++) { for(j=0;j<4;j++) printf("%p ->%d\n",&arr[i][j],arr[i][j]); }
000000000062FE10 ->1 000000000062FE14 ->2 000000000062FE18 ->3 000000000062FE1C ->4 000000000062FE20 ->5 000000000062FE24 ->6 000000000062FE28 ->7 000000000062FE2C ->8 000000000062FE30 ->9 000000000062FE34 ->10 000000000062FE38 ->11 000000000062FE3C ->12
A two-dimensional array a can be seen as a[0],a 1 a[2] is a one-dimensional array of three elements, A is its array name; a[0],a 1 a[2] is also a one-dimensional array, whose array names are a[0],a 1,a[2],a[0],a1 a[2] denotes the address of the first element of each array, respectively.
arr[0]=000000000062FE00 ->1 arr[1]=000000000062FE10 ->5 arr[2]=000000000062FE20 ->9
Because the array name is the pointer, a[0],a 1 a[2] is a first-order pointer, and its type is int. A is an array of three first-order pointers.
So what is the difference between a and a[0],a+1 and a[0]+1?
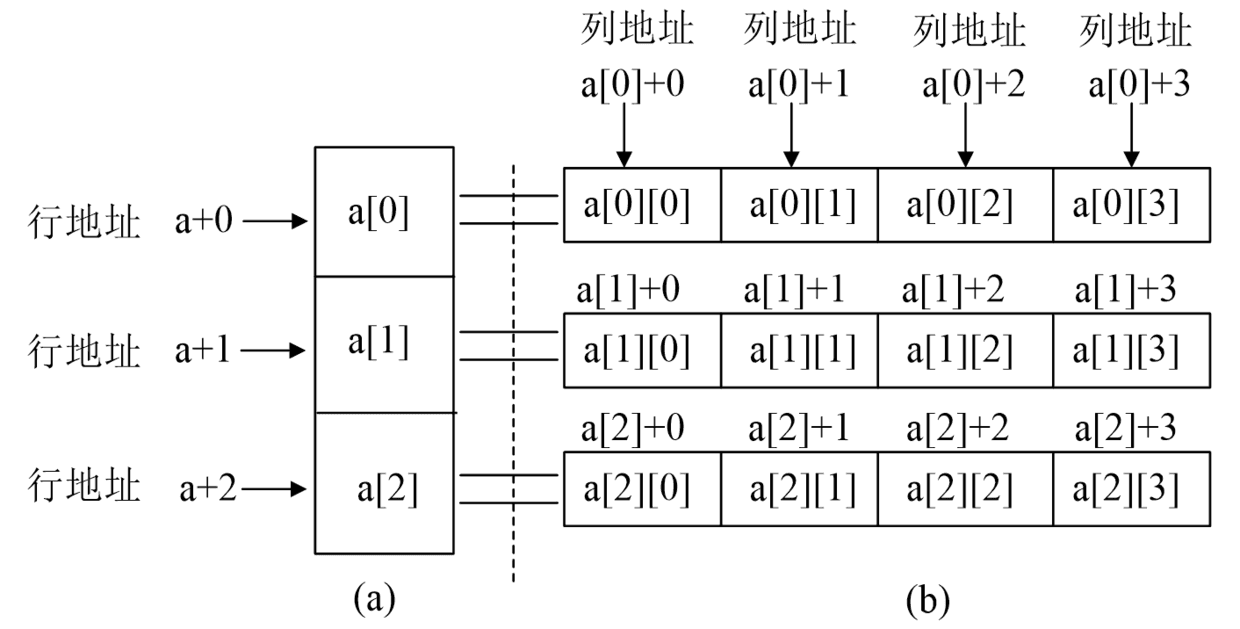
a+1 spans one line of a two-dimensional array, so a,a+1 and a+2 are line addresses.
Compared with a[0], a[0]+1 crosses only one element of a two-dimensional array, so it is a column address.
From this we can see that:
(1)a+i is the address of line i, i.e. * (a + i) = a[i]. So a[i][j] is equivalent to (*(a+i)[j];
(2)a[i]+j is the address of column J in line i, so * (a[i]+j) is equivalent to a[i][j].
The above equivalence relations are summarized as follows:
a[i][j] ←→ (*(a+i))[j] ←→ *(a[i]+j) ←→ *(*(a+i)+j)
To understand the relationship between pointers and two-dimensional arrays, we need to first understand the elements represented by various array names.
The array name represents the address of the first element of the array, that is, arr=&arr[0][0];
Two-dimensional arrays are also stored in memory in the form of one-dimensional arrays, except that the elements of each one-dimensional array are one-dimensional arrays, so two-dimensional array names can be regarded as pointers to row arrays.
For the storage of two-dimensional arrays, they are arranged in the form of row after column. Each row is regarded as a one-bit array. That two-dimensional array is an array composed of multiple one-bit arrays, that is, two-dimensional array is an array of arrays.
For two-dimensional array names, it can be regarded as a pointer to row arrays, that is, the elements of two-dimensional arrays are row arrays. Therefore, for two-dimensional arrays, the change of addition and subtraction is based on the size of row arrays, that is, arr points to arr[0], and arr+1 points to arr. 1 This row array. The first address of each row array is de-referenced, i.e. the first address of arr is equal to that of arr[0], (arr+1) is the first address of the first row array, and arr 1 It's equal. If you want to take the address of the second element in line 1, it's arr. 1 +2, because arr 1 Represents a one-dimensional array, so its element is a number. When it is added or subtracted, it moves by the size of the element. For example, +1 means the first element in the array, and + 3 means the three elements in the array (starting with 0). because
(arr+1) and arr 1 Equal, so the address of the second element in the first line can also be expressed as (arr+1)+2, which is de-referenced to get the specific value of the array element, that is ((arr+1)+2).
So there are the following formulas. If a two-dimensional array has N elements in each row and the name of the two-dimensional array is arr, then the address of the j element in line I is * (arr + i) +j, which can also be expressed as arr[i]+j. The value of an element can be expressed as *(*(arr+i)+j), or as arr[i][j].
For the above two-dimensional array arr, although arr[0], arr are the first address of the array, but they point to different objects. Ar [0] is the name of the one-dimensional array. It points to the first element of the arr[0], and its value is the address of the first element of the arr[0]. The value of arr[0], which is the first element value of the arr[0] array, is calculated by "*", and the result is an array element value, that is, the first element value of the arr[0] array (in this case, the first element value of the arr[0] array). Thus, * arr[0] and arr[0][0] are the same values.
Ar (arr+0) is the name of a two-dimensional array. It points to the first element of the element it belongs to, and every element of the two-dimensional array is a one-dimensional array. Therefore, arr points to the first element of the two-dimensional array arr, arr[0], and the address of arr[0] is obtained by its * operation. To get the value of arr[0][0], another * operation is needed.
*arr=&arr[0]; **arr=*(&arr[0])=arr[0][0]
printf("arr=%p ->%d\n",arr,**arr); //Ar[0][0] is obtained by applying two solutions. printf("*arr=%p->%d\n",*arr,**arr) ; printf("arr[0]=%p ->%d\n",arr[0],*(arr[0])); //arr[0][0] address printf("%p\n",&arr[0][0]); printf("%p\n",&arr[0]); printf("%p\n",*(arr+2)+3); //&arr[2][3] printf("%p\n",arr[2]+3); printf("%p ->%d\n",*(arr+2)+3,*(*(arr+2)+3)); printf("%p ->%d\n",&arr[2][3],arr[2][3]);
arr=000000000062FE00 ->1 *arr=000000000062FE00->1 arr[0]=000000000062FE00 ->1 000000000062FE00 000000000062FE00 000000000062FE2C 000000000062FE2C 000000000062FE2C ->12 000000000062FE2C ->12
Therefore, when the array name is the most pointer, the moving unit is "row", so arr+i points to the array of the first row, that is, to arr[i]. The first address of one-dimensional array arr[0], i.e. \ arr and arr[0] are the same value but point to different types of arr (arr+1 and arr[0]+1 can see their differences). When the pointer P is defined with int p, the pointer P is an int data, which is added or subtracted. It moves an int size instead of an address. Therefore, it is correct to assign P with arr[0], but wrong to assign P with arr.
* Use array pointers to manipulate secondary pointers*
And int (ptr)[4],ptr denotes a pointer to five int arrays, which is added or subtracted. The variable is the size of the array multiplied by the size of the data type. PTR + 1 = & PTR + 4 sizeof (int);
Therefore, int (ptr)[4] can be initialized with arr, because PTR is a data that points to four int elements, while arr points to the address of its first element, and its first element is a data that contains four int elements.
int (*ptr)[4]=arr; // Row address printf("arr=%p->%d\n",&arr[0][0],arr[0][0]); //Head element address printf("ptr=%p->%d\n",ptr,**ptr); printf("ptr+1=%p->%d\n",ptr+1,**(ptr+1)); //Line Address plus 1 Moving 4 int Sizes printf("*(ptr+1)+1=%p->%d\n",*(ptr+1)+1,*(*(ptr+1)+1)); //
Operation results: arr=000000000062FE00->1 ptr=000000000062FE00->1 ptr+1=000000000062FE10->5 *(ptr+1)+1=000000000062FE14->6
Complete procedure:
#include<stdio.h> int main() { int i,j=0; int arr[3][4] = {{1,2,3,4},{5,6,7,8},{9,10,11,12}}; //Print the values and addresses of line 2, element 3 in different forms //According to the rule deduced, the following is the rule. //The address of the element int *p; // p+1 Moves the size of an int type, column address arr+1 ; //Move an int a[4] type size, line address // p=arr; // error, type mismatch p=*arr; // Legitimate, equal to p=*(a+0) p=arr[0]; // Lawfully, arr[0] represents the address of the first element arr[0][0] of the array arr[0]. p= & arr[0][0] for(i=0;i<3;i++) printf("arr[%d]=%p ->%d\n",i,arr[i],*(arr[i])); int (*ptr)[4]=arr; printf("arr=%p->%d\n",&arr[0][0],arr[0][0]); printf("ptr=%p->%d\n",ptr,**ptr); printf("ptr+1=%p->%d\n",ptr+1,**(ptr+1)); printf("*(ptr+1)+1=%p->%d\n",*(ptr+1)+1,*(*(ptr+1)+1)); printf("arr=%p ->%d\n",arr,**arr); //Ar[0][0] is obtained by applying two solutions. printf("*arr=%p->%d\n",*arr,**arr) ; printf("arr[0]=%p ->%d\n",arr[0],*(arr[0])); //arr[0][0] address printf("\n"); printf("%p\n",&arr[0][0]); printf("%p\n",&arr[0]); printf("%p\n",*(arr+2)+3); //&arr[2][3] printf("%p\n",arr[2]+3); //The value of this element is 12. printf("%p ->%d\n",*(arr+2)+3,*(*(arr+2)+3)); printf("%p ->%d\n",&arr[2][3],arr[2][3]); printf("\n"); for (i=0;i<3;i++) { for(j=0;j<4;j++) printf("%p ->%d\n",&arr[i][j],arr[i][j]); } return 0; }
The results are as follows:
ptr=000000000062FE00->1 ptr+1=000000000062FE10->5 *(ptr+1)+1=000000000062FE14->6 arr=000000000062FE00 ->1 *arr=000000000062FE00->1 arr[0]=000000000062FE00 ->1 000000000062FE00 000000000062FE00 000000000062FE2C 000000000062FE2C 000000000062FE2C ->12 000000000062FE2C ->12 000000000062FE00 ->1 000000000062FE04 ->2 000000000062FE08 ->3 000000000062FE0C ->4 000000000062FE10 ->5 000000000062FE14 ->6 000000000062FE18 ->7 000000000062FE1C ->8 000000000062FE20 ->9 000000000062FE24 ->10 000000000062FE28 ->11 000000000062FE2C ->12
Pointer array
char *strs[]={"abc","def","acfg"};
The pointer array is an array whose elements are pointers. The array name represents the address of the first element of the array, and the first element is a pointer. Therefore, the array name of the pointer array is a secondary pointer.

int douptr(char** a,int size) { char **p=a; int c=strlen(*p+2); printf("a=%p\n",a); printf("p=%p\n",p); printf("%s\n",*p); printf("%c",**p); } int main() { int i,j=0; char *strs[] = {"abc","def","acfg"}; int size=sizeof(arr)/sizeof(char *); //Array size printf("arr=%p\n",arr); //Array Address, Array Name Represents the First Element Address printf("&arr[0]=%p\n",&arr[0]); //The address of the first element of the array printf("&arr[1]=%p\n",&arr[1]); printf("&arr[2]=%p\n",&arr[2]); printf("&arr[0]=%p\n",arr); //The address of the first element of the array printf("arr[0]->%s\n",*arr); //The first element of the array points to the content printf("arr[1][1]=%c\n",*(*(arr+1)+1)) ; //The second element points to the second element of the array. printf("arr[1][1]=%c\n",arr[1][1]); // printf("size=%d\n",size); douptr(arr,size); return 0; }
Result:
arr=000000000062FE30 &arr[0]=000000000062FE30 &arr[1]=000000000062FE38 &arr[2]=000000000062FE40 &arr[0]=000000000062FE30 arr[0]->abc arr[1][1]=e arr[1][1]=e size=3 a=000000000062FE30 p=000000000062FE30 abc a
Reference
1 https://www.oschina.net/question/253203_243676
[2] https://ybzbxcc.github.io/2016/08/06/LeetCode-14-Longest-Common-Prefix/
[3] http://ssspure.blog.51cto.com/8624394/1694224
[4] https://v.qq.com/x/cover/3dwhoro4c9voh54/h14042xdbdp.html
[6] http://ssspure.blog.51cto.com/8624394/1694224
[7] #leetcode——Longest Common Prefix
Label (Space Separation): Unclassified
Description of the problem: Write a function to find the longest common prefix string amongst an array of strings.
Analysis:
The title is to find the longest prefix of a set of strings, such as {"abcd", "abdf","abf"}. The first two "ab" of the three strings are the same, and the longest prefix is ab.
Disintegration idea: The longest identical prefix must be less than or equal to the shortest string length, so first find the shortest string and use it as the object of comparison. If the string on a bit is different from the string being compared, the comparison ends, and the character before the bit of the string being compared is the longest identical prefix of the string group.
Two special situations need to be considered in this question:
(1) The string group is empty. At this point, return directly to "".
(2) A string group has only one string. In this case, the longest prefix is the string itself.
Code:
#include <stdio.h> #include <string.h> #include <stdlib.h> char* longestCommonPrefix(char** strs, int strsSize); int main() { char * strs[] = { "abc","abd" }; int strsize = sizeof(strs) / sizeof(char *); printf("strsize=%d\n", strsize); char *CommonPrefix; //char **str = &strs[0]; int i=0; for ( i = 0; i < strsize;i++) { printf("strsize[%d]=%d\n",i, strlen(strs[i])); printf("strs[%d]=%s\n", i, strs[i]); } CommonPrefix=longestCommonPrefix(strs,strsize); printf("longestCommonPrefix=%s\n",CommonPrefix); system("pause"); return 0; } char* longestCommonPrefix(char** strs, int strsSize) { if (strs == NULL || *strs == NULL) { return ""; } if (strsSize==1) return *strs; int i,j,x=0; int minprefix=strlen(strs[0]); //strs is a secondary pointer, that is, a pointer. Its essence is a pointer array, that is, an array whose elements are pointers. The array name represents the address of the first element of the array, and the first element is a pointer. Therefore, the array name of the pointer array is a secondary pointer. char temp; char *result; for(i=1;i<strsSize;i++) //Find the shortest string and record its length { if(minprefix>strlen(strs[i])) { minprefix=strlen(strs[i]); x=i; } } for (j=0;j<minprefix;j++) //Use the shortest string length as the upper limit of comparison digits { temp=*(strs[x]+j); //Baseline the shortest string for(i=0;i<strsSize;i++) { if(*(strs[i]+j)!=temp) //If one character is found to be different, the comparison ends break; } if(i!=strsSize) //At least two corresponding bits in each string are different, ending the loop break; } if(j>=0) { result = (char* ) malloc(sizeof(char) *(j+1)); strncpy(result,strs[x],j+1); //Return the same first j bit result[j]='\0'; } return result; }
char* longestCommonPrefix(char** strs, int strsSize) { if (strs == NULL || *strs == NULL) { return ""; } int len = strlen(strs[0]); int max = 0; char *res; while (max < len) { for (int i = 1; i < strsSize; i++) { if (strs[i][max] != strs[0][max]) //Compare bit by bit until different bits are found { len = max; break; } } if (max < len) max++; //Discovery of dislocation } res = (char*)malloc(sizeof(char)*(max+1)); for (int i = 0; i < max; i++) //Return the same pre-max bit { res[i] = strs[0][i]; } res[max] = '\0'; return res; }
Secondary Pointer and Two-Dimensional Array
char *string[] ={"abc","abcd","acf"};
char string[3][4]={"abc","abcd","acf"};
First of all, although the array name of a two-dimensional array can be regarded as a pointer, it is not possible to assign the array name of a two-dimensional array to a second-level pointer, that is, the following code
int main(void) { int arr[3][3] = {{1,2,3},{4,5,6},{7,8,9}}; int **p = arr; return 0; }
The above code will report an error when it is executed, because the data types of the two are different, so they cannot be assigned.
The array name of a two-dimensional array points to a one-dimensional array, that is, to the array type, but the second-level pointer points to a first-level pointer, that is, to the pointer type, so they cannot assign values to each other.
The relationship between pointers and two-dimensional arrays is described in detail below.
Declare a two-dimensional array
int a[3][4] = {{1,2,3,4},{5,6,7,8},{9,10,11,12}}; for (i=0;i<3;i++) { for(j=0;j<4;j++) printf("%p ->%d\n",&arr[i][j],arr[i][j]); }
000000000062FE10 ->1 000000000062FE14 ->2 000000000062FE18 ->3 000000000062FE1C ->4 000000000062FE20 ->5 000000000062FE24 ->6 000000000062FE28 ->7 000000000062FE2C ->8 000000000062FE30 ->9 000000000062FE34 ->10 000000000062FE38 ->11 000000000062FE3C ->12
A two-dimensional array a can be seen as a[0],a 1 a[2] is a one-dimensional array of three elements, A is its array name; a[0],a 1 a[2] is also a one-dimensional array, whose array names are a[0],a 1,a[2],a[0],a1 a[2] denotes the address of the first element of each array, respectively.
arr[0]=000000000062FE00 ->1 arr[1]=000000000062FE10 ->5 arr[2]=000000000062FE20 ->9
Because the array name is the pointer, a[0],a 1 a[2] is a first-order pointer, and its type is int. A is an array of three first-order pointers.
So what is the difference between a and a[0],a+1 and a[0]+1?
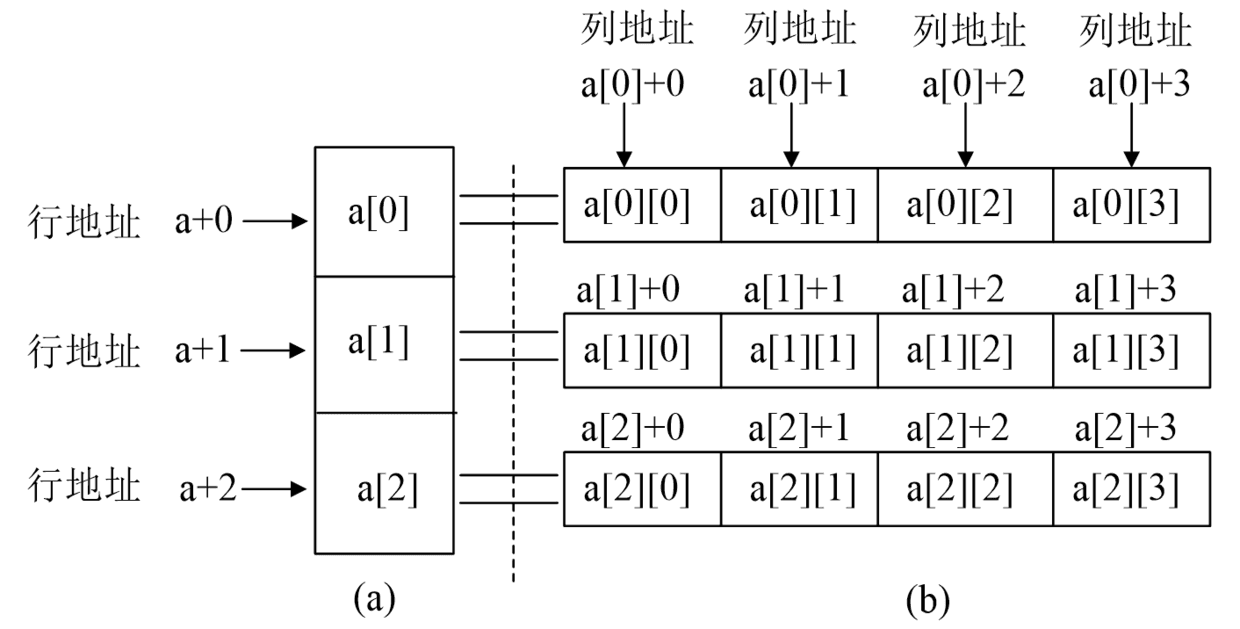
a+1 spans one line of a two-dimensional array, so a,a+1 and a+2 are line addresses.
Compared with a[0], a[0]+1 crosses only one element of a two-dimensional array, so it is a column address.
From this we can see that:
(1)a+i is the address of line i, i.e. * (a + i) = a[i]. So a[i][j] is equivalent to (*(a+i)[j];
(2)a[i]+j is the address of column J in line i, so * (a[i]+j) is equivalent to a[i][j].
The above equivalence relations are summarized as follows:
a[i][j] ←→ (*(a+i))[j] ←→ *(a[i]+j) ←→ *(*(a+i)+j)
To understand the relationship between pointers and two-dimensional arrays, we need to first understand the elements represented by various array names.
The array name represents the address of the first element of the array, that is, arr=&arr[0][0];
Two-dimensional arrays are also stored in memory in the form of one-dimensional arrays, except that the elements of each one-dimensional array are one-dimensional arrays, so two-dimensional array names can be regarded as pointers to row arrays.
For the storage of two-dimensional arrays, they are arranged in the form of row after column. Each row is regarded as a one-bit array. That two-dimensional array is an array composed of multiple one-bit arrays, that is, two-dimensional array is an array of arrays.
For two-dimensional array names, it can be regarded as a pointer to row arrays, that is, the elements of two-dimensional arrays are row arrays. Therefore, for two-dimensional arrays, the change of addition and subtraction is based on the size of row arrays, that is, arr points to arr[0], and arr+1 points to arr. 1 This row array. The first address of each row array is de-referenced, i.e. the first address of arr is equal to that of arr[0], (arr+1) is the first address of the first row array, and arr 1 It's equal. If you want to take the address of the second element in line 1, it's arr. 1 +2, because arr 1 Represents a one-dimensional array, so its element is a number. When it is added or subtracted, it moves by the size of the element. For example, +1 means the first element in the array, and + 3 means the three elements in the array (starting with 0). because
(arr+1) and arr 1 Equal, so the address of the second element in the first line can also be expressed as (arr+1)+2, which is de-referenced to get the specific value of the array element, that is ((arr+1)+2).
So there are the following formulas. If a two-dimensional array has N elements in each row and the name of the two-dimensional array is arr, then the address of the j element in line I is * (arr + i) +j, which can also be expressed as arr[i]+j. The value of an element can be expressed as *(*(arr+i)+j), or as arr[i][j].
For the above two-dimensional array arr, although arr[0], arr are the first address of the array, but they point to different objects. Ar [0] is the name of the one-dimensional array. It points to the first element of the arr[0], and its value is the address of the first element of the arr[0]. The value of arr[0], which is the first element value of the arr[0] array, is calculated by "*", and the result is an array element value, that is, the first element value of the arr[0] array (in this case, the first element value of the arr[0] array). Thus, * arr[0] and arr[0][0] are the same values.
Ar (arr+0) is the name of a two-dimensional array. It points to the first element of the element it belongs to, and every element of the two-dimensional array is a one-dimensional array. Therefore, arr points to the first element of the two-dimensional array arr, arr[0], and the address of arr[0] is obtained by its * operation. To get the value of arr[0][0], another * operation is needed.
*arr=&arr[0]; **arr=*(&arr[0])=arr[0][0]
printf("arr=%p ->%d\n",arr,**arr); //Ar[0][0] is obtained by applying two solutions. printf("*arr=%p->%d\n",*arr,**arr) ; printf("arr[0]=%p ->%d\n",arr[0],*(arr[0])); //arr[0][0] address printf("%p\n",&arr[0][0]); printf("%p\n",&arr[0]); printf("%p\n",*(arr+2)+3); //&arr[2][3] printf("%p\n",arr[2]+3); printf("%p ->%d\n",*(arr+2)+3,*(*(arr+2)+3)); printf("%p ->%d\n",&arr[2][3],arr[2][3]);
arr=000000000062FE00 ->1 *arr=000000000062FE00->1 arr[0]=000000000062FE00 ->1 000000000062FE00 000000000062FE00 000000000062FE2C 000000000062FE2C 000000000062FE2C ->12 000000000062FE2C ->12
Therefore, when the array name is the most pointer, the moving unit is "row", so arr+i points to the array of the first row, that is, to arr[i]. The first address of one-dimensional array arr[0], i.e. \ arr and arr[0] are the same value but point to different types of arr (arr+1 and arr[0]+1 can see their differences). When the pointer P is defined with int p, the pointer P is an int data, which is added or subtracted. It moves an int size instead of an address. Therefore, it is correct to assign P with arr[0], but wrong to assign P with arr.
* Use array pointers to manipulate secondary pointers*
And int (*ptr)[4],ptr denotes a pointer to four int arrays, which is added or subtracted. The variable is the size of the array multiplied by the size of the data type. PTR + 1 = & PTR + 4 sizeof (int);
Thus, int (*ptr)[4] can be initialized with arr, because PTR is a data that points to four int elements, and arr points to the address of its first element, and its first element is a data that contains four int elements.
int (*ptr)[4]=arr; // Row address printf("arr=%p->%d\n",&arr[0][0],arr[0][0]); //Head element address printf("ptr=%p->%d\n",ptr,**ptr); printf("ptr+1=%p->%d\n",ptr+1,**(ptr+1)); //Line Address plus 1 Moving 4 int Sizes printf("*(ptr+1)+1=%p->%d\n",*(ptr+1)+1,*(*(ptr+1)+1)); //
Operation results: arr=000000000062FE00->1 ptr=000000000062FE00->1 ptr+1=000000000062FE10->5 *(ptr+1)+1=000000000062FE14->6
Complete procedure:
#include<stdio.h> int main() { int i,j=0; int arr[3][4] = {{1,2,3,4},{5,6,7,8},{9,10,11,12}}; //Print the values and addresses of line 2, element 3 in different forms //According to the rule deduced, the following is the rule. //The address of the element int *p; // p+1 Moves the size of an int type, column address arr+1 ; //Move an int a[4] type size, line address // p=arr; // error, type mismatch p=*arr; // Legitimate, equal to p=*(a+0) p=arr[0]; // Lawfully, arr[0] represents the address of the first element arr[0][0] of the array arr[0]. p= & arr[0][0] for(i=0;i<3;i++) printf("arr[%d]=%p ->%d\n",i,arr[i],*(arr[i])); int (*ptr)[4]=arr; printf("arr=%p->%d\n",&arr[0][0],arr[0][0]); printf("ptr=%p->%d\n",ptr,**ptr); printf("ptr+1=%p->%d\n",ptr+1,**(ptr+1)); printf("*(ptr+1)+1=%p->%d\n",*(ptr+1)+1,*(*(ptr+1)+1)); printf("arr=%p ->%d\n",arr,**arr); //Ar[0][0] is obtained by applying two solutions. printf("*arr=%p->%d\n",*arr,**arr) ; printf("arr[0]=%p ->%d\n",arr[0],*(arr[0])); //arr[0][0] address printf("\n"); printf("%p\n",&arr[0][0]); printf("%p\n",&arr[0]); printf("%p\n",*(arr+2)+3); //&arr[2][3] printf("%p\n",arr[2]+3); //The value of this element is 12. printf("%p ->%d\n",*(arr+2)+3,*(*(arr+2)+3)); printf("%p ->%d\n",&arr[2][3],arr[2][3]); printf("\n"); for (i=0;i<3;i++) { for(j=0;j<4;j++) printf("%p ->%d\n",&arr[i][j],arr[i][j]); } return 0; }
The results are as follows:
ptr=000000000062FE00->1 ptr+1=000000000062FE10->5 *(ptr+1)+1=000000000062FE14->6 arr=000000000062FE00 ->1 *arr=000000000062FE00->1 arr[0]=000000000062FE00 ->1 000000000062FE00 000000000062FE00 000000000062FE2C 000000000062FE2C 000000000062FE2C ->12 000000000062FE2C ->12 000000000062FE00 ->1 000000000062FE04 ->2 000000000062FE08 ->3 000000000062FE0C ->4 000000000062FE10 ->5 000000000062FE14 ->6 000000000062FE18 ->7 000000000062FE1C ->8 000000000062FE20 ->9 000000000062FE24 ->10 000000000062FE28 ->11 000000000062FE2C ->12
Pointer array
char *strs[]={"abc","def","acfg"};
The pointer array is an array whose elements are pointers. The array name represents the address of the first element of the array, and the first element is a pointer. Therefore, the array name of the pointer array is a secondary pointer.
The two-dimensional array name represents the address of the first element, and the two-dimensional array can be understood as a one-dimensional array, and the elements of each one-dimensional array are
A one-dimensional array. Addition and subtraction operations are performed in terms of the size of one-dimensional arrays. Similar to the array pointer, that is, the pointer to the array, the addition and subtraction operations are also carried out in units of the size of the array.

int douptr(char** a,int size) { char **p=a; int c=strlen(*p+2); printf("a=%p\n",a); printf("p=%p\n",p); printf("%s\n",*p); printf("%c",**p); } int main() { int i,j=0; char *strs[] = {"abc","def","acfg"}; int size=sizeof(arr)/sizeof(char *); //Array size printf("arr=%p\n",arr); //Array Address, Array Name Represents the First Element Address printf("&arr[0]=%p\n",&arr[0]); //The address of the first element of the array printf("&arr[1]=%p\n",&arr[1]); printf("&arr[2]=%p\n",&arr[2]); printf("&arr[0]=%p\n",arr); //The address of the first element of the array printf("arr[0]->%s\n",*arr); //The first element of the array points to the content printf("arr[1][1]=%c\n",*(*(arr+1)+1)) ; //The second element points to the second element of the array. printf("arr[1][1]=%c\n",arr[1][1]); // printf("size=%d\n",size); douptr(arr,size); return 0; }
Result:
arr=000000000062FE30 &arr[0]=000000000062FE30 &arr[1]=000000000062FE38 &arr[2]=000000000062FE40 &arr[0]=000000000062FE30 arr[0]->abc arr[1][1]=e arr[1][1]=e size=3 a=000000000062FE30 p=000000000062FE30 abc a
Reference
1 https://www.oschina.net/question/253203_243676
[2] https://ybzbxcc.github.io/2016/08/06/LeetCode-14-Longest-Common-Prefix/
[3] http://ssspure.blog.51cto.com/8624394/1694224
[4] https://v.qq.com/x/cover/3dwhoro4c9voh54/h14042xdbdp.html
[6] http://ssspure.blog.51cto.com/8624394/1694224
[7] http://c.biancheng.net/cpp/html/478.html