Think of a way to use proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy proxy Pro
Implementation steps
1. Implementing Agent by Modifying and Modifying Configuration in learn Project
Open index.js under config
Add the following code to proxyTable
'/learn': { target: 'http://localhost:8088', changeOrigin: true, pathRewrite: { '^/learn': '/'/ } }
2. Modify api.js
let base = '/learn'
In this way, every request / learn t is equivalent to a request / localhost: 8088
Use login interface as column
import axios from 'axios' let base = '/learn' // Registration interface export const ReginUser = params => { return axios.post(`${base}/regin`, params) } // Logon interface export const LoginUser = params => { return axios.get(`${base}/login`, {params: params}) }
When the proxy is not used, we access the login interface. The actual requested address is locaihost:8080/learn/login.
After this proxy, we access the login interface and the real request address is:
localhost:8088/login
3. Modify main.js
Cancel the use of mock data
// Introduce mock and initialize // import Mock from './data/mock' // Mock.init()
So the rewriting of this project is completed.
4. Write node-server
1. Initialization
npm init
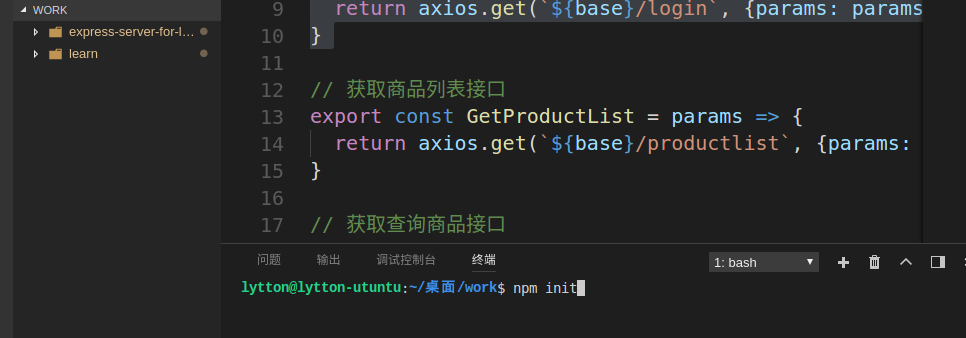
2. Let's talk about the bags that are probably used.
package.json
{ "name": "nodeserver", "version": "1.0.0", "description": "express mongoose node-server", "main": "app.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "start": "nodemon app.js" }, "author": "lytton", "license": "ISC", "dependencies": { "body-parser": "^1.18.2", "express": "^4.16.2", "express-promise-router": "^2.0.0", "formidable": "^1.1.1", "mongoose": "^4.13.7", "morgan": "^1.9.0", "nodemon": "^1.14.1", "shortid": "^2.2.8" } }
Because I use async when I write, please ensure that the installed version of node is at least 7.6, node-v view, if it is below 7.6, please upgrade.

3. Write app.js
/** * author: lytton * date: 2017-12-21 * email: lzr3278@163.com */ const express = require('express') const logger = require('morgan') const mongoose = require('mongoose') const bodyParser = require('body-parser') // Connect to database mongodb mongoose.Promise = global.Promise mongoose.connect('mongodb://localhost/learn', {useMongoClient: true}) const connection = mongoose.connection; connection.on('error',err => { if(err){ console.log(err); } }); connection.on('open', () => { console.log('opened MongoDB'); }); // routes const route = require('./routes/route') // app const app = express() // middlewares // Log record app.use(logger('dev')) // body-parser app.use(bodyParser.json()) // routes const base = '' app.use(base, route) // catch 404 err and then to err handler app.use((req, res, next) => { const err = new Error('Not Found 404') err.status = 404 next(err) }) // err handler app.use((err, req, res, next) => { const error = app.get('env') === 'development' ? err : {} const status = err.status || 500 res.status(status).json({ error: { message: error.message } }) console.error(err) }) // listen port const port = app.get('port') || 8088 app.listen(port, () => { console.log('your server are listening at localhost:'+ port) })
4. Write route.js
const express = require('express') const router = require('express-promise-router')() // const router = express.Router() const Controller = require('../controllers/control') // register router.route('/regin') .post(Controller.regin) // Sign in router.route('/login') .get(Controller.login) module.exports = router
5. Write control.js
const {User, Product} = require('../models/model') const formidable = require('formidable') const form = new formidable.IncomingForm() module.exports = { // register regin: async (req, res, next) => { const newuser = new User(req.body) const adduser = await newuser.save() res.status(200).send({ adduser: adduser }) }, // Sign in login: async (req, res, next) => { const user = await User.findOne(req.query) res.status(200).json({ code: 200, msg: 'Login successfully', user: user }) } /** * Callback */ // newuser: (req, res, next) => { // const newuser = req.body // const adduser = new user(newuser) // adduser.save((err, user) => { // if (err) { // next(err) // } else { // res.status(200).json(newuser) // } // }) // } /** * Promise */ // newuser: (req, res, next) => { // const newuser = req.body // const adduser = new user(newuser) // adduser.save().then(newuser => { // res.status(200).json(newuser) // }).catch(err => { // next(err) // }) // } /** * async */ // newuser: async (req, res, next) => { // const newuser = new User(req.body) // const adduser = await newuser.save() // res.status(200).json(adduser) // } }
6. Write model.js
const mongoose = require('mongoose') const shortid = require('shortid') const Schema = mongoose.Schema UserSchema = new Schema({ _id: { type: String, 'default': shortid.generate }, username: String, password: String, email: String, tel: Number, avatar: { type: String, 'default': 'http://diy.qqjay.com/u2/2014/1027/4c67764ac08cd40a58ad039bc2283ac0.jpg' }, date: Date, name: String }) const User = mongoose.model('User', UserSchema) productsSchema = new Schema({ _id: { type: String, 'default': shortid.generate }, type: String, name: String, prods: [{ type: Schema.Types.ObjectId, ref: 'Prods' }] }) const Product = mongoose.model('Product', productsSchema) prodsSchema = new Schema({ _id: { type: String, 'default': shortid.generate }, name: String, price: Number, desc: String, selling: Boolean, info: String }) const Prods = mongoose.model('Prods', prodsSchema) sendsSchema = new Schema({ _id: { type: String, 'default': shortid.generate }, sendname: String, sendaddr: String, sendtel: Number, recepname: String, recepaddr: String, receptel: Number, sendprod: String, sendmsg: String, sendprice: Number, sendcode: Number, sendstauts: String, sender: { type: Schema.Types.ObjectId, ref: 'User' } }) const Sends = mongoose.model('Sends', sendsSchema) module.exports = { User, Product, Prods, Sends }
7. Briefly speaking, understanding
In this way, the whole server can be used almost simply. As a novice, I have some new knowledge to say.
1. The function of nodemon, using the sentence nodemon app.js, is to make the whole server hot overload, that is, to load the modified content without restarting the server.
2. The router used is
const router = require('express-promise-router')()
Instead of
// const router = express.Router()
As we all know, using Promise or async requires catch(err).
promise then().catch(err =>{}),
async try{}.catch(err => {}),
And we use express-promise-router, so that we can automatically catch(err) in the whole process, so as to reduce the amount of code, how to do it, I don't know, please bosses to solve the confusion.
5, test
1. Open mongodb first
sudo service mongod start
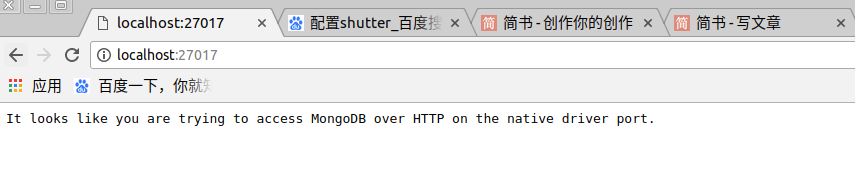
2. Start the server we wrote and make sure it succeeds
npm run start
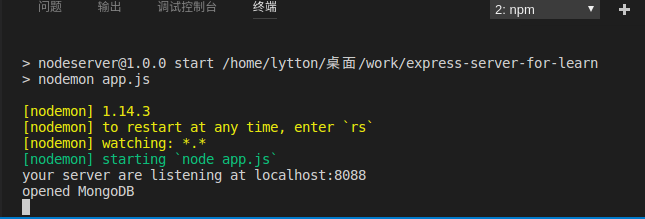
3. Start our project learn
npm run dev
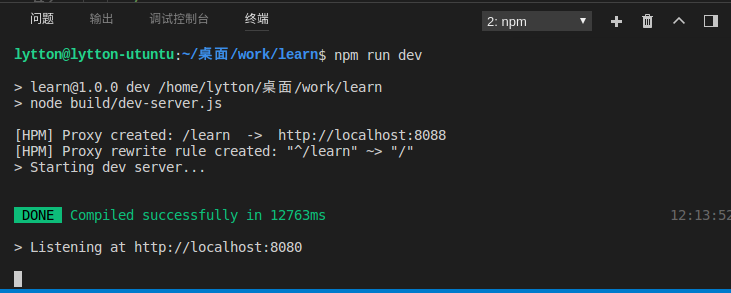
4. Start testing
Open robo3t first and look at the user in the database.
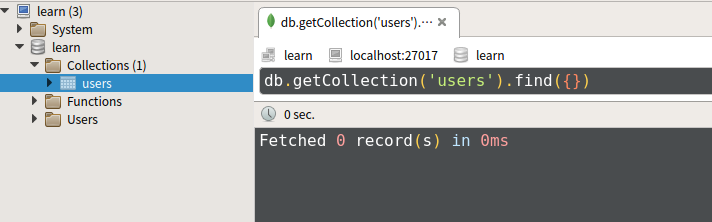
no data
Register a user
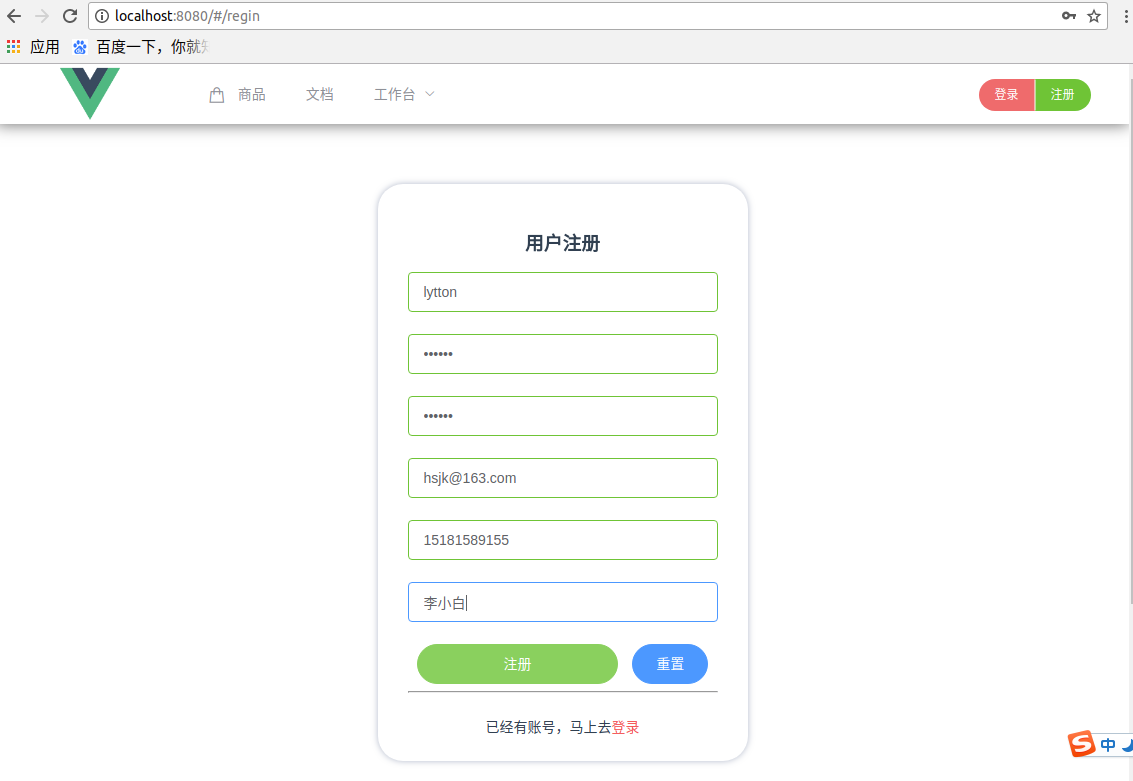
Successfully returned
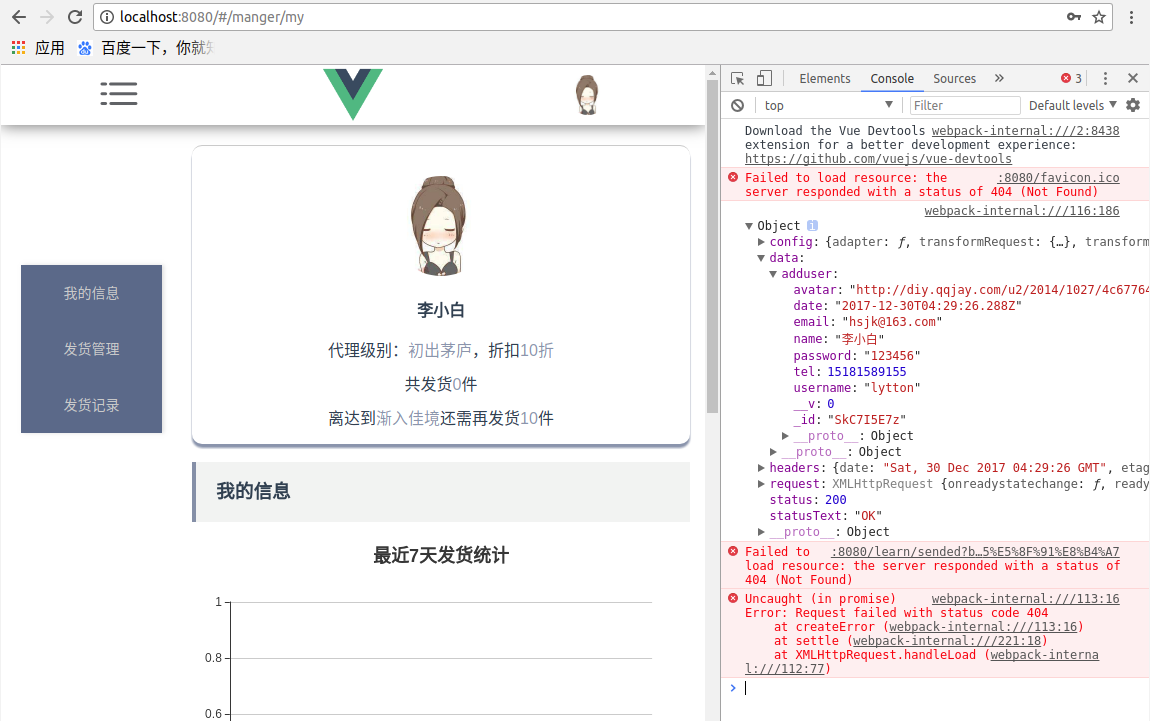
There was an error because the page also requested other data, but did not write the interface well, so there was an error.
Look at the database again.
At this point, the data has been successfully inserted.
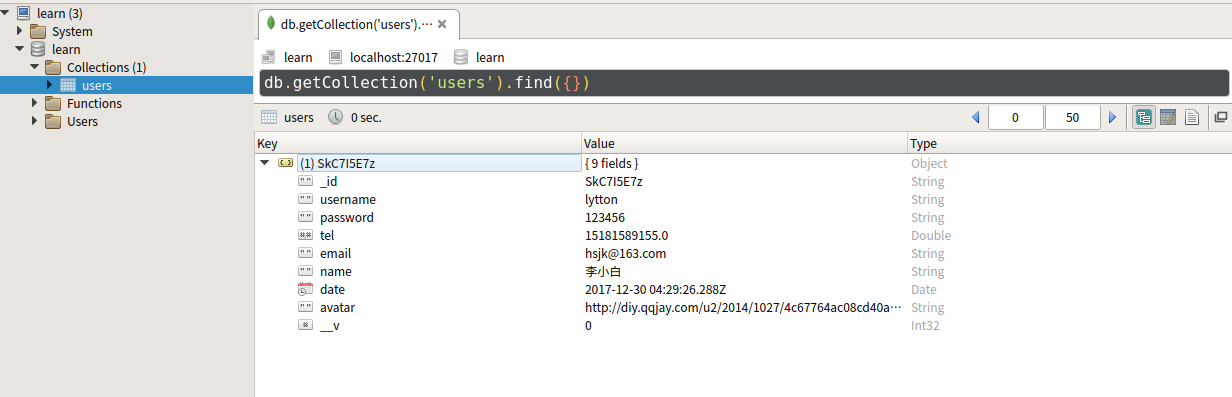
Look at our server again.
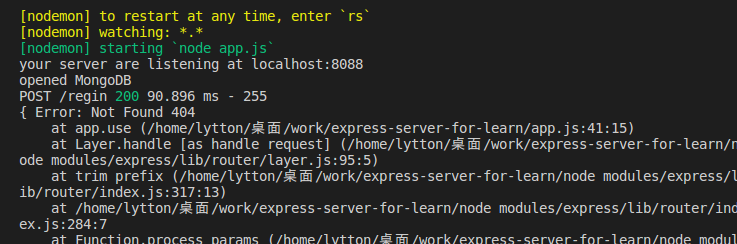
You can see that the request for registration is successful, the error is to request other data error, because there is no write interface!!!
Test the login function again
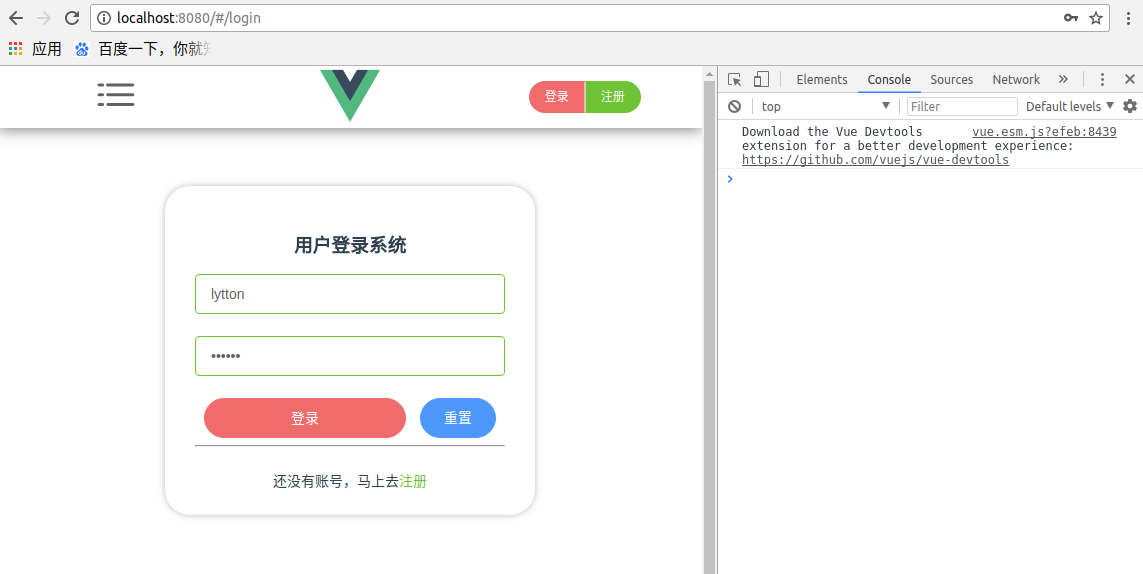
Similarly, it succeeded.
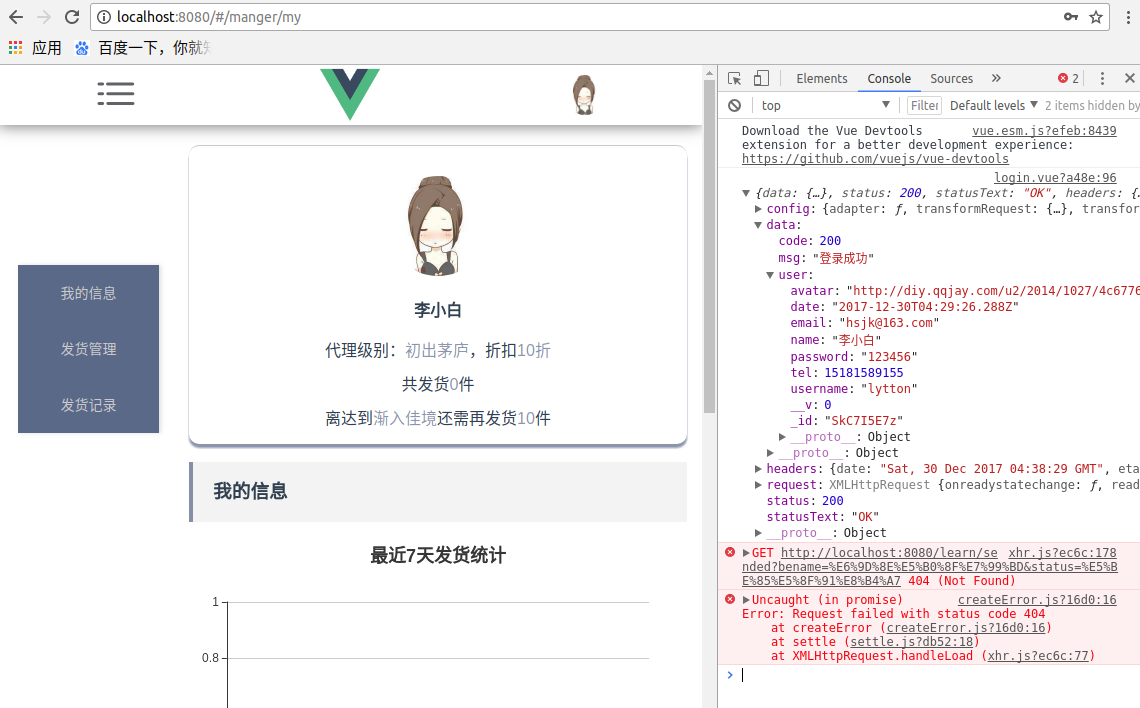
Look at server.
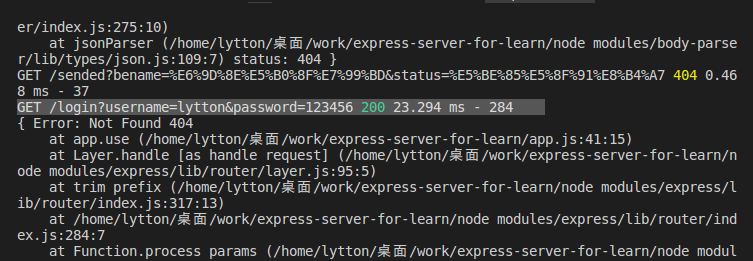
The login function was also successful
Follow up plan
Synchronize and improve the functions written today
github address
learn: https://github.com/lyttonlee/learn
server: https://github.com/lyttonlee/express-server-for-learn