Introduction to ES6
ECMAScript 6, short for ES6, is the next generation standard of JavaScript language, which was officially released in June 2015. Its goal is to enable JavaScript language to be used to write complex large-scale applications and become an enterprise-level development language.
The relationship between ECMAScript and JavaScript: The former is the syntax specification of the latter, and the latter is an implementation of the former.
Babel: Convert ES6 code to ES5 code http://babeljs.io/
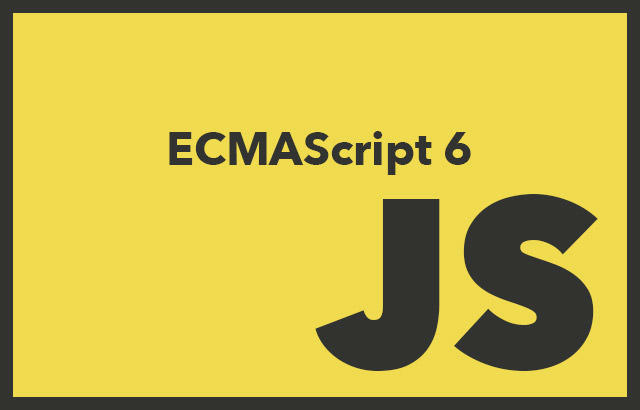
New features
let,const
Variables defined by let will not be elevated by variables, const constants can not be modified, let and const are block-level scopes
Before ES6, js had no concept of block-level scope {}. (Functional scope, global scope, eval scope)
After ES6, let and const appeared, and js also had the concept of block-level scope. Front-end knowledge is changing with each passing day.~
Variable promotion: Before ES6, the var keyword declared variables. Wherever a declaration is made, it is deemed to be declared at the top of a function; not within a function, that is, at the top of a global scope. This will lead to some misunderstandings. For example:
console.log(a); // undefined
var a = 'hello';
# The above code is equivalent to
var a;
console.log(a);
a = 'hello';
# and let It won't be elevated by variables.
console.log(a); // a is not defined
let a = 'hello';
Constants defined by const cannot be modified
var name = "bai";
name = "ming";
console.log(name); // ming
const name = "bai";
name = "ming"; // Assignment to constant variable.
console.log(name);
import,export
Import import module and export export module
// All imports
import people from './example'
// Import the entire module as a single object, and all exports of the module will exist as attributes of the object.
import * as example from "./example.js"
console.log(example.name)
console.log(example.getName())
// In the import section, when introducing non-default, use curly brackets
import {name, age} from './example'
// Export default, with and only with one default
export default App
// Partial Derivation
export class App extend Component {};
class,extends,super
The most troublesome parts of ES5: prototypes, constructors, inheritance, with ES6 we no longer bother!
ES6 introduces the concept of Class.
class Animal {
constructor() {
this.type = 'animal';
}
says(say) {
console.log(this.type + ' says ' + say);
}
}
let animal = new Animal();
animal.says('hello'); //animal says hello
class Cat extends Animal {
constructor() {
super();
this.type = 'cat';
}
}
let cat = new Cat();
cat.says('hello'); //cat says hello
The above code first defines a "class" with class, and you can see that there is a constructor method inside, which is the construction method, and this keyword represents the instance object. Simply put, the methods and attributes defined within the constructor are the instance objects themselves, while the methods and attributes defined outside the constructor can be shared by all the power objects.
Classes can be inherited through extends keywords, which is much clearer and more convenient than ES5 through modifying the prototype chain to achieve inheritance. A Cat Class is defined above, which inherits all the properties and methods of the Animal Class through the extends keyword.
The super keyword refers to an instance of the parent class (that is, the parent's this object). The subclass must call the super method in the constructor method, otherwise an error will be reported when a new instance is created. This is because the subclass does not have its own this object, but inherits the parent's this object and processes it. If you don't call the super method, the subclass won't get this object.
The inheritance mechanism of ES6 is essentially to create the instance object this of the parent class (so the super method must be called first) and then modify this with the constructor of the subclass.
// ES5
var Shape = function(id, x, y) {
this.id = id,
this.move(x, y);
};
Shape.prototype.move = function(x, y) {
this.x = x;
this.y = y;
};
var Rectangle = function id(ix, x, y, width, height) {
Shape.call(this, id, x, y);
this.width = width;
this.height = height;
};
Rectangle.prototype = Object.create(Shape.prototype);
Rectangle.prototype.constructor = Rectangle;
var Circle = function(id, x, y, radius) {
Shape.call(this, id, x, y);
this.radius = radius;
};
Circle.prototype = Object.create(Shape.prototype);
Circle.prototype.constructor = Circle;
// ES6
class Shape {
constructor(id, x, y) {
this.id = id this.move(x, y);
}
move(x, y) {
this.x = x this.y = y;
}
}
class Rectangle extends Shape {
constructor(id, x, y, width, height) {
super(id, x, y) this.width = width this.height = height;
}
}
class Circle extends Shape {
constructor(id, x, y, radius) {
super(id, x, y) this.radius = radius;
}
}
arrow functions
Fast Writing of Functions. function keywords are not required to create functions, return keywords are omitted, and this keywords in the current context are inherited.
// ES5
var arr1 = [1, 2, 3];
var newArr1 = arr1.map(function(x) {
return x + 1;
});
// ES6
let arr2 = [1, 2, 3];
let newArr2 = arr2.map((x) => {
x + 1
});
Small details of arrow function: When your function has only one parameter, parentheses can be omitted; when you have only one expression in the function, you can omit {}.
let arr2 = [1, 2, 3];
let newArr2 = arr2.map(x => x + 1);
This object in JavaScript language has always been a headache. Running the above code will cause errors, because this in setTimeout points to global objects.
class Animal {
constructor() {
this.type = 'animal';
}
says(say) {
setTimeout(function() {
console.log(this.type + ' says ' + say);
}, 1000);
}
}
var animal = new Animal();
animal.says('hi'); //undefined says hi
Solution:
// Traditional method 1: pass this to self, and then use self to refer to this
says(say) {
var self = this;
setTimeout(function() {
console.log(self.type + ' says ' + say);
}, 1000);
}
// Traditional method 2: using bind(this), i.e.
says(say) {
setTimeout(function() {
console.log(this.type + ' says ' + say);
}.bind(this), 1000);
}
// ES6: Arrow function
// When we use the arrow function, the this object in the function body is the object in which we define it.
says(say) {
setTimeout(() => {
console.log(this.type + ' says ' + say);
}, 1000);
}
template string
It solves the pain point of ES5 in string function.
First use: string splicing. Expressions are embedded in strings for splicing and defined by `and ${}.
// es5
var name1 = "bai";
console.log('hello' + name1);
// es6
const name2 = "ming";
console.log(`hello${name2}`);
Second use: In ES5, we use backslash to do multi-line string splicing. ES6 inverse quotation mark `directly.
// es5
var msg = "Hi \
man!";
// es6
const template = `<div>
<span>hello world</span>
</div>`;
In addition: include repeat
// Include: Determine whether to include and then return the Boolean value directly
let str = 'hahah';
console.log(str.includes('y')); // false
// repeat: Gets the string repeated n times
let s = 'he';
console.log(s.repeat(3)); // 'hehehe'
destructuring
Simplify the extraction of information from arrays and objects.
Before ES6, we got object information one by one.
After ES6, deconstruction allows us to take data from objects or arrays and store it as variables
// ES5
var people1 = {
name: 'bai',
age: 20,
color: ['red', 'blue']
};
var myName = people1.name;
var myAge = people1.age;
var myColor = people1.color[0];
console.log(myName + '----' + myAge + '----' + myColor);
// ES6
let people2 = {
name: 'ming',
age: 20,
color: ['red', 'blue']
}
let { name, age } = people2;
let [first, second] = people2.color;
console.log(`${name}----${age}----${first}`);
Default function default parameter
// ES5 defines default values for function parameters
function foo(num) {
num = num || 200;
return num;
}
// ES6
function foo(num = 200) {
return num;
}
rest arguments (rest parameters)
Solve the es5 complex arguments problem
function foo(x, y, ...rest) {
return ((x + y) * rest.length);
}
foo(1, 2, 'hello', true, 7); // 9
Spread Operator
First use: assembling arrays
let color = ['red', 'yellow'];
let colorful = [...color, 'green', 'blue'];
console.log(colorful); // ["red", "yellow", "green", "blue"]
Second use: Get other items in an array except for a few items
let num = [1, 3, 5, 7, 9];
let [first, second, ...rest] = num;
console.log(rest); // [5, 7, 9]
object
Object initialization abbreviation
// ES5
function people(name, age) {
return {
name: name,
age: age
};
}
// ES6
function people(name, age) {
return {
name,
age
};
}
Object literal abbreviation (omitting colons and function keywords)
// ES5
var people1 = {
name: 'bai',
getName: function () {
console.log(this.name);
}
};
// ES6
let people2 = {
name: 'bai',
getName () {
console.log(this.name);
}
};
In addition: Object.assign()
The ES6 object provides Object.assign() as a method for shallow replication. Object.assign() can copy the enumerable attributes of any number of source objects to the target object, and then return to the target object. The first parameter is the target object. In a real project, we did not change the source object. Typically, the target object is passed as {}
const obj = Object.assign({}, objA, objB)
// Adding attributes to objects
this.seller = Object.assign({}, this.seller, response.data)
Promise
Write asynchronous code synchronously
// Initiate asynchronous requests
fetch('/api/todos')
.then(res => res.json())
.then(data => ({
data
}))
.catch(err => ({
err
}));
Generators
generator is a function that returns an iterator.
Generator function is also a kind of function, the most intuitive performance is that there is an asterisk * more than ordinary function, in its function body can use the field keyword, interestingly, the function will pause after each field.
Here is a vivid example of life. When we go to the bank to do business, we have to get a queue number from the machine in the lobby. When you get your queue number, the machine will not automatically give you another ticket. That is to say, the ticket machine is "suspended" until the next person wakes up again before voting continues.
Iterator: When you call a generator, it returns an iterator object. This iterator object has a method called next to help you restart the generator function and get the next value. The next method not only returns values, but also returns objects with two attributes: done and value. Value is the value you get, and done is used to indicate whether your generator has stopped providing the value. Continue to use the example of just getting tickets. Each queue number is the value here. Whether the paper printing tickets is used up or not is done here.
// generator
function *createIterator() {
yield 1;
yield 2;
yield 3;
}
// The generator can be called like a normal function, but it returns an iterator
let iterator = createIterator();
console.log(iterator.next().value); // 1
console.log(iterator.next().value); // 2
console.log(iterator.next().value); // 3
Iterators play a very important role in asynchronous programming. Asynchronous invocation is very difficult for us. Our functions do not wait for the asynchronous invocation to complete before execution. You may think of using callback functions. (There are other schemes such as Promise, Async/await, of course.)
Generators allow our code to wait. Nested callback functions are not required. Using generator ensures that the execution of the function is suspended when the asynchronous call is completed before our generator function runs a line of code.
So the problem is that we can't call the next() method manually all the time. You need a method that can call the generator and start the iterator. Like this:
function run(taskDef) {
// taskDef is a generator function
// Create an iterator to make it available elsewhere
let task = taskDef();
// Start the task
let result = task.next();
// Recursively uses functions to maintain calls to next()
function step() {
// If there's more to do
if (!result.done) {
result = task.next();
step();
}
}
// Start the process
step();
}
summary
These are some of the most commonly used grammars in ES6. It can be said that 20% of the grammar accounts for 80% of the daily use of ES6.
Last update time: 2017-06-24 18:52:48
Links to original articles: http://bxm0927.github.io/2017/06/24/es6_study/