Learning C language [8] looking for single dog
Keywords:
C++
Only two numbers in an array appear once, and all other numbers appear twice. Write a function to find the two numbers that only appear once. LeetCode: Once only numbers, title links
Example
Input: [1,2,3,4,5,2,1,3]
Output: 4, 5
Method 1: sort the array first, and then compare the adjacent numbers to see if they are the same. Figure:
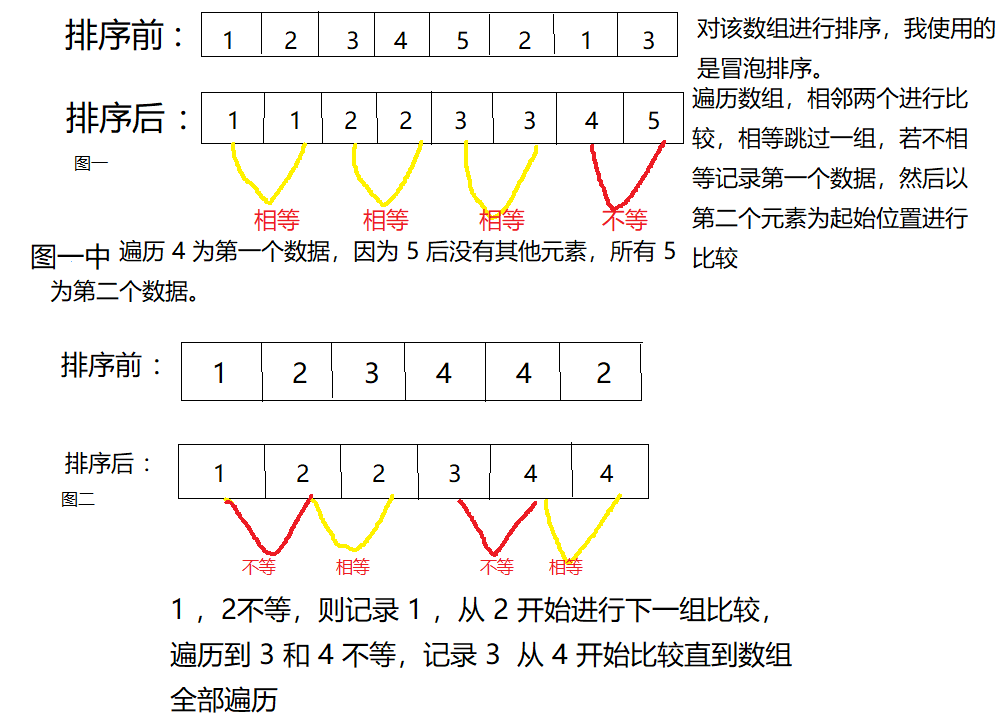
#include<stdio.h>
void Bbsort(int* a,int n)//Bubble sorting
{
int j = 0;
int i = 0;
for (i = 0; i < n; i++)
{
for (j = 0; j < n - i - 1; j++)
{
if (a[j] > a[j + 1])
{
int temp = a[j];
a[j] = a[j+1];
a[j + 1] = temp;
}
}
}
}
void finddog(int* a, int n,int* num )
{
Bbsort(a,n);//Sort first
int i = 0;
int j = 0;
for (i = 0; i < n;)
{
if (a[i] == a[i + 1])//Two adjacent equal, traverse the next group
{
i += 2;//Skip a group
}
else//Unequal, record the first data, the second is the starting position
{
num[j]= a[i];
i++;
j++;
}
}
}
int main()
{
int arr[] = { 1,2,3,4,5,2,1,3 };
int num[2] = {0};
int sz = sizeof(arr) / sizeof(arr[0]);//Number of elements
finddog(arr,sz,num);
printf("%d %d ", num[0],num[1]);
return 0;
}
Method 2: XOR (two numbers, the same is 0, the difference is 1). All elements of the whole array are XOR. The element XOR that appears twice is "0", and the final result cannot be zero (otherwise there is no single dog, it is impossible, I am a single dog). Look for the array element XOR and the binary is 1 Then, the array is divided into two groups: one group has all the binary bits of 1, and all the numbers with the bit of 1 in the array are exclusive or, because the same number of exclusive or in the array is 0, only the number of single dogs with the bit of 1 is left; the other group has all the bits of 0, similarly, all the numbers with the bit of 0 are exclusive or, and only the number of single dogs with the bit of 0 is left.
#include<stdio.h>
void finddog(int a[], int sz, int* num)
{
int i = 0;
int pos = 0;
int ret = 0;
//Traverses the array, resulting in exclusive or of two different numbers.
for (i = 0; i < sz; i++)
{
ret ^= a[i];
}
//Look for a bit of 1 in the XOR result of these two different numbers
for (pos = 0; pos < 32; pos++)
{
if (((ret >> pos) & 1) == 1)//Integer 32-bit, traversing from low to high
{
break; //pos record number with binary bit 1
}
}
for (i = 0; i < sz; i++)
{
//Find the number with pos bit 1 in the array and XOR
if (((a[i] >> pos) & 1) == 1)
{
num[1] ^= a[i];
}
//Find the number of pos bits 0 in the array and XOR
else
{
num[0] ^= a[i];
}
}
}
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 2, 1, 3 };
int num[2] = { 0 };
int sz = sizeof(arr) / sizeof(arr[0]);
finddog(arr, sz, num);
printf("%d %d\n", num[0], num[1]);
return 0;
}
Posted by r4r4 on Sat, 09 May 2020 08:15:00 -0700