- home page
- special column
- PHP Garden
- Article details
Laravel 5.8 bottom learning note 01 - control inversion and dependency injection
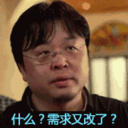
reference material:
Explanation of core concepts of laravel underlying core code analysis
PHP inversion of control (IOC) and dependency injection (DI)
1 - Laravel framework core
advantage
- Integrated composer
- Dependency injection is implemented to better manage class dependencies and facilitate extension (compared with MVC mode)
- Advanced features: console, event, queue, middleware, facade mode
- Core concept: service container serviceProvider
Disadvantages and optimization
shortcoming
- Too many files loaded, slow access
optimization
- Cache profile
- Remove unnecessary loading files (mainly serviceProvider)
- Open Opcache
Framework initiation process (life cycle)
- Reference autoload file
- Build service container
1) Register basic bindings
2) Register service container, event, routing and log services through bind
3) Bind interface through bind - Get Request object
- Logical processing
1) Resolve startup items (basic services), such as routing, exception handling, facade and service container
2) Through the pipeline mode, middleware is used to filter user requests and process business logic - Return Response object
Knowledge points
Singleton mode, observer mode, pipeline mode
Dependency injection, anonymous function, reflection
Predefined interface ArrayAccess
2 - control inversion and dependency injection
inversion of control (IOC)
The dependencies between components are managed from the inside to the outside of the program
Explanation: instead of directly creating class B instances in class A, class B instances are transferred to class a through the IOC container
dependency injection (DI)
Inject component dependencies through external parameters or other forms
Example:
class DbMysql { public function query(){} } class IOC { public $db; public function __construct($dbMysql) { $this->db = $dbMysql; } public function action() { $this->db->query(); } } $db = new DbMysql(); $c = new IOC($db); $c->action();
The IOC class does not need to instantiate DbMysql, but passes in the instance of DbMysql as a parameter and only calls the method of DbMysql. This pattern is called dependency injection.
Putting the instantiation action of class B outside the IOC class is called control inversion.
Reflection mechanism of PHP
In PHP runtime, extend the analyzer to export or propose detailed information about classes, methods, properties and parameters. This function of dynamically obtaining and calling information is called reflection API.
class A { public function __construct(B $b) { } } class B { } //Get the reflection information of the class (all information) $reflector = new ReflectionClass('A'); //Get constructor $constructor = $reflector->getConstructor(); //Get constructor parameters $dependencies = $constructor->getParameters(); //Gets the dependent class name foreach ($dependencies as $dependency){ if(!is_null($dependency->getClass())){ $classname = $dependency->getClass()->name; $p[] = new $classname(); } } //Create a new class instance from the given parameters $a = $reflector->newInstanceArgs($p);
If class B also has dependent classes, it needs to be created recursively
<?php class A { public function __construct(B $b) { $this->b = $b; } public function getB() { $this->b->bMethod(); } } class B { public function __construct(C $c,D $d) { $this->c = $c; $this->d = $d; } public function bMethod() { echo "I am B Methods in bMethod()"; } } class C{ public function __construct() { } public function cMethod(){ echo "I am C Methods in cMethod()"; } } class D{ public function __construct() { } public function dMethod(){ echo "I am D Methods in dMethod()"; } } class Ioc { protected $instances = []; public function __construct() { } public function getInstance($classname){ $reflector = new ReflectionClass($classname); $constructor = $reflector->getConstructor(); $dependencies = $constructor->getParameters(); if(!$dependencies){ return new $classname(); } foreach ($dependencies as $dependency){ if(!is_null($dependency->getClass())){ $instances[] = $this->make($dependency->getClass()->name); } } return $reflector->newInstanceArgs($instances); } public function make($classname){ return $this->getInstance($classname); } } $ioc = new Ioc(); $a = $ioc->make('A'); $a->getB();
summary
The essence of PHP program running: including files and obtaining instantiated objects.
Traditional framework: manage class dependencies through include/require.
Laravel: through namespace and use, it realizes the automatic loading mechanism, finds the file where the class is located, and then obtains the instantiated object of the class through reflection.
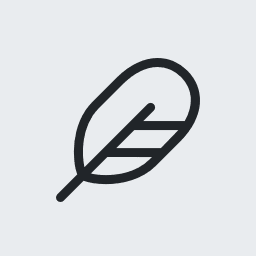