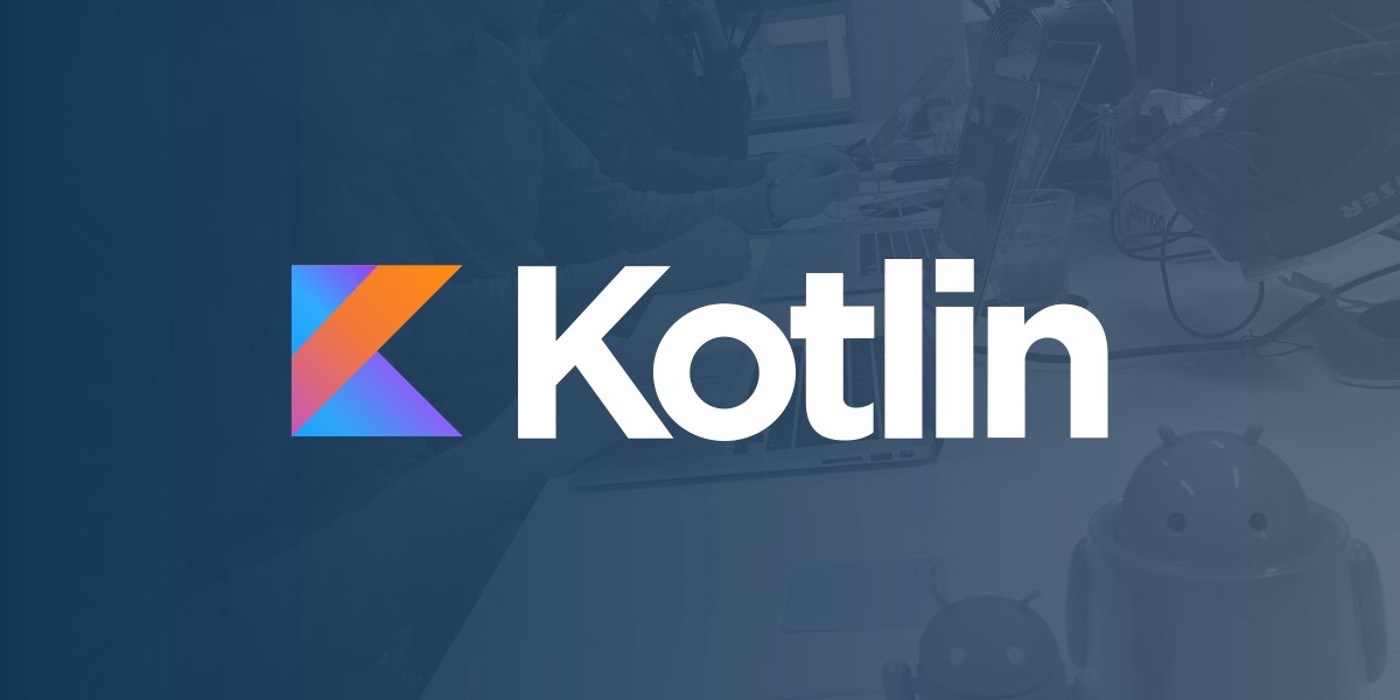
data class Tut( var title:String, var courses:Int, var author:String, var category:String )
Define the data class Tut to describe the course object, including some features of the course.
var tuts = listOf<Tut>( Tut("angular base",10,"zidea","web"), Tut("vue base",10,"rob","web"), Tut("golang base",30,"zidea","web"), Tut("rust base",120,"tina","native"), Tut("kotlin base",50,"jerry","mobie"), Tut("groovy base",60,"tony","tool") ) var webTuts = ArrayList<Tut>() for(tut in tuts){ if(tut.category == "web"){ webTuts.add(tut) } } for(tut in webTuts){ println(tut.component1()) }
Doing applications, especially web applications, mostly deals with data, gets data, finds data and updates data. Here we use list to simulate a table, which contains some course records for our use. Then we use the idea of java to find the data we want, that is, to find the course of category for the web.
fun getWebTuts(tuts:List<Tut>):ArrayList<Tut>{ var webTuts = ArrayList<Tut>() for(tut in tuts){ if(tut.category == "web"){ webTuts.add(tut) } } return webTuts }
Further organized as a getWebTuts method, we can also extend the value of category as a parameter to be passed into the function for use.
var webTuts = getWebTuts(tuts) for(tut in webTuts){ println(tut.component1()) }
Well, there's a new demand for courses with less than 100 hours.
fun getTutlessThen100(tuts:List<Tut>):ArrayList<Tut>{ var result = ArrayList<Tut>() for(tut in tuts){ if(tut.courses < 100){ result.add(tut) } } return result }
var webTuts = getWebTuts(tuts) for(tut in webTuts){ println(tut.component1()) } var coursesLessThen100Tuts = getTutlessThen100(tuts) for(tut in coursesLessThen100Tuts){ println(tut.title) }
How can we liberate ourselves from endless demands?
Behavior parameterization
- Pass the filter behavior (function) as a parameter into the filter
- Clean Code to Improve Efficiency
demand
- Find out the courses with the largest number of courses
println(tuts.maxBy { it.courses })
Tut(title=rust base, courses=120, author=tina, category=native)
println(tuts.filter { (it.courses == 10) and (it.category == "web") })
[Tut(title=angular base, courses=10, author=zidea, category=web), Tut(title=vue base, courses=10, author=rob, category=web)]
Map
println(tuts.map { "${it.title} by ${it.author}" })
Any
Determine whether there is a data in the set
println(tuts.any { it.courses == 120 })
Count
println(tuts.count { it.courses > 10 }) #### groupBy ```kotlin println(tuts.groupBy { it.category })
{web=[Tut(title=angular base, courses=10, author=zidea, category=web), Tut(title=vue base, courses=10, author=rob, category=web), Tut(title=golang base, courses=30, author=zidea, category=web)], native=[Tut(title=rust base, courses=120, author=tina, category=native)], mobie=[Tut(title=kotlin base, courses=50, author=jerry, category=mobie)], tool=[Tut(title=groovy base, courses=60, author=tony, category=tool)]}
println(tuts.groupBy { it.category }.get("web")?.forEach{ println("${it.title}") })