1, Function realization requirements
Implementation: in the waterfall flow page layout implemented by RecyclerView, click any commodity to jump to its commodity details page.
2, Concrete implementation
The file directory is as follows:
2.1 detail page design after jump
Now only jump implementation is considered. Taking the first product details page as an example, the design is as follows:
If parameter passing is considered, the function can be called:
Pass: intent.putExtra()
Received: getStringExtra(), getIntExtra()
Next, you need to add an Activity on the jump details page before you can use intent to jump between activities. On the R.layout. Page, replace the page with the xml file name of the corresponding detail page of the design.
2.2 add an item click event in the adapter
In the onBindViewHolder function in the Adapter, add listening for click events
// item click event holder.itemView.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent=new Intent(context,pic1Activity); context.startActivity(intent); } });
Code parsing:
① The itemView of the function name refers to the whole content of a single item. When the whole is clicked, an event will be triggered. If you want to click only some controls (pictures and text) in the item, you can change it to:
ImageView img1 TextView txt1 holder.img1.setOnClickListener(); holder.txt1.setOnClickListener();
② Intent usage: used to start another component in an App or a component of another App in a component. Usually, the components are Activity, Service and Broadcast. Here, we use intent to jump to the Activity on the details page after clicking on the shop page.
Function: Intent(context,pic1Activity.class)
The input parameter is (Activity of the current page, class file of the page after jump)
2.3 solve the problem of jumping to different detail pages
In the Adapter, an array of type class is created to store the class files of activities of different jump pages. The purpose is to change the position of pic1Activity in intent = new intent (context, pic1Activity). Therefore, the onBindViewHolder code can be modified as follows:
// Add the following code inside the MyAdapter function and outside the onBindViewHolder function private Class[] jumpActivity={pic1Activity.class,pic2Activity.class}; private int i=0; // Add the following code to the onBindViewHolder function body holder.itemView.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent=new Intent(context,i++); context.startActivity(intent); } });
2.4 solve the problem that the jump cannot be repeated
After the above design runs, it will be found that all pictures can only be clicked once to their own details page, because when variable i traverses the entire Class array, all listening and jumping of the whole page will stop. Therefore, we use the getAdapterPosition() function to obtain the index of a single item on the entire RecyclerView page, so that the variable i can always be re assigned, so as to realize repeated jump. Therefore, the code can be changed to:
// Add the following code inside the MyAdapter function and outside the onBindViewHolder function private Class[] jumpActivity={pic1Activity.class,pic2Activity.class}; // Add the following code to the onBindViewHolder function body int i = holder.getAdapterPosition(); holder.itemView.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent=new Intent(context,jumpActivity[i]); context.startActivity(intent); } });
3, Effect display
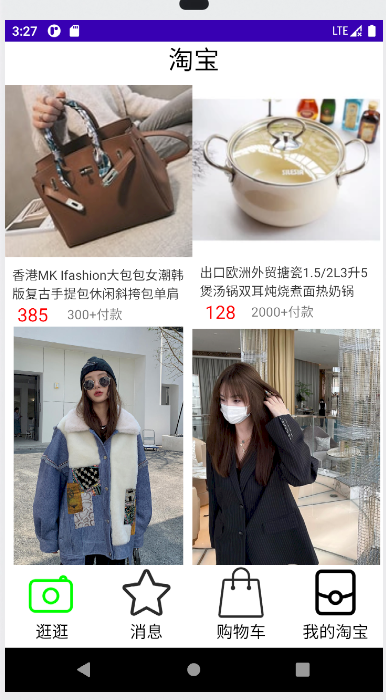
Click the first two pictures to jump:
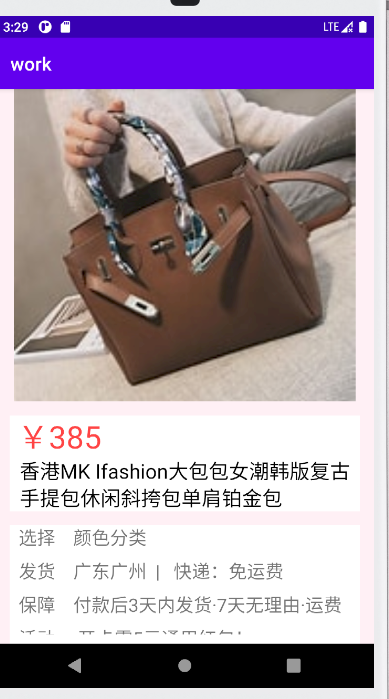
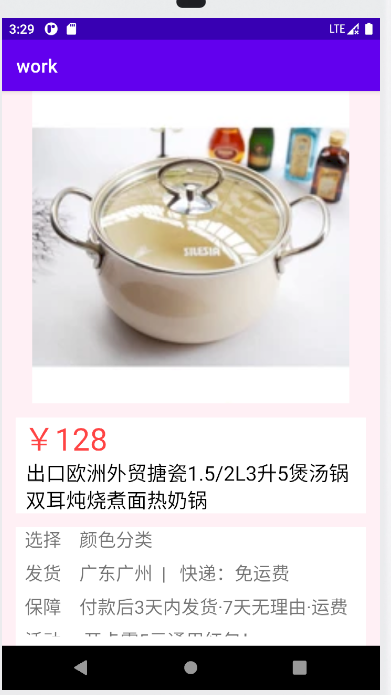