JSON and AJAX in the Pthon3 Development Advanced-Djamgo Framework
Keywords:
Python
JSON
Javascript
xml
JQuery
Read the catalog
- What is JSON
- What is AJAX
- Common scenarios for AJAX applications
- jQery implements AJAX
- How to set csrf_token for AJAX requests
- AJAX Upload File
- Supplement Django's built-in serializers
1. What is JSON?
- JSON is a string that represents a JavaScript object (JavaScript Object Notation)
- JSON is a lightweight text data exchange format
- JSON Language Independent *
- JSON is self-describing and easier to understand
* JSON uses JavaScript syntax to describe data objects, but JSON is still independent of language and platform.JSON parsers and JSON libraries support many different programming languages.
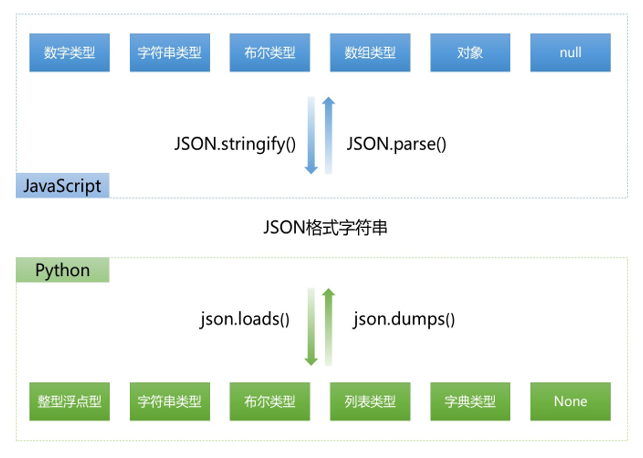
Eligible json objects:
["one", "two", "three"]
{ "one": 1, "two": 2, "three": 3 }
{"names": ["Big Baby", "Two Dolls"] }
[ { "name": "Big Baby"}, {"name": "Two Dolls"} ]
Unqualified json object:
{ name: "Zhang San", 'age': 32 } // Property names must be double quoted
[32, 64, 128, 0xFFF] // Hexadecimal value cannot be used
{ "name": "Zhang San", "age": undefined } // undefined cannot be used
{ "name": "Zhang San",
"birthday": new Date('Fri, 26 Aug 2011 07:13:10 GMT'),
"getName": function() {return this.name;} // Functions and date objects cannot be used
}
1. stringify and parse methods
Two methods for JSON object and string conversion in JavaScript:
JSON.parse(): Used to convert a JSON string to a JavaScript object
JSON.parse('{"name":"duoduo"}'); -----> Object {name:"duoduo"}
JSON.parse('{name:"duoduo"}') ; // error
JSON.parse('[18,undefined]') ; // error
JSON.stringify(): Used to convert JavaScript values to JSON strings.
JSON.stringify({"name":"duoduo"})
Be careful:
1. Single quotation marks are written outside {}, double quotation marks must be used for each dry property name, otherwise an exception will be thrown
a={name:"duoduo"}; //ok
b={'name':'duoduo'}; //ok
c={"name":"duoduo"}; //ok
alert(a.name); //ok
alert(a[name]); //undefined
alert(a['name']) //ok
2. django sends data to js
def login(request):
obj={'name':"duoduo"}
return render(request,'index.html',{"objs":json.dumps(obj)})
#----------------------------------
<script>
var temp={{ objs|safe }}
alert(temp.name);
alert(temp['name'])
</script>
In addition, the ajax technology described below allows js to accept django's corresponding json data.
3. Comparison of JSON and XML
The JSON format was proposed by Douglas Crockford in 2001 to replace the cumbersome XML format.
The JSON format has two distinct advantages: it is easy to write and straightforward; it conforms to the native JavaScript syntax and can be handled directly by the interpretation engine without adding additional parsing code.
As a result, JSON was quickly accepted as a standard format for exchanging data across major websites and was written to ECMAScript 5 as part of the standard.
Both XML and JSON use structured methods to tag data, so here's a simple comparison.
Some provinces and cities in China are represented by XML as follows:

<?xml version="1.0" encoding="utf-8"?>
<country>
<name>China</name>
<province>
<name>Heilongjiang</name>
<cities>
<city>Harbin</city>
<city>Daqing</city>
</cities>
</province>
<province>
<name>Guangdong</name>
<cities>
<city>Guangzhou</city>
<city>Shenzhen</city>
<city>Zhuhai</city>
</cities>
</province>
<province>
<name>Taiwan</name>
<cities>
<city>Taipei</city>
<city>Kaohsiung</city>
</cities>
</province>
<province>
<name>Xinjiang</name>
<cities>
<city>Urumchi</city>
</cities>
</province>
</country>
XML Format Data
Expressed in JSON as follows:

{
"name": "China",
"province": [{
"name": "Heilongjiang",
"cities": {
"city": ["Harbin", "Daqing"]
}
}, {
"name": "Guangdong",
"cities": {
"city": ["Guangzhou", "Shenzhen", "Zhuhai"]
}
}, {
"name": "Taiwan",
"cities": {
"city": ["Taipei", "Kaohsiung"]
}
}, {
"name": "Xinjiang",
"cities": {
"city": ["Urumchi"]
}
}]
}
JSON format data
As you can see from the code at both ends above:
- JSON's simple syntax format and clear hierarchy are significantly easier to read than XML
- In terms of data exchange, JSON uses much fewer characters than XML.
- The bandwidth used to transmit data can be greatly saved.(
2. What is AJAX
The translation of AJAX (Asynchronous Javascript And XML) into Chinese is "Asynchronous Javascript And XML".Even if you use the Javascript language to interact asynchronously with the server,
The data transferred is XML (of course, the data transferred is not just XML).AJAX is not a new programming language, but a new way to use existing standards.
The best advantage of AJAX is that you can exchange data with the server and update part of the page content without reloading the entire page.
(This feature gives the user the impression that the request and response process is completed unconsciously)
AJAX does not require any browser plug-ins, but requires the user to allow JavaScript to execute on the browser.
- Synchronous interaction: After a client makes a request, it waits for the server to respond before making a second request;
- Asynchronous interaction: After a client makes a request, it can make a second request without waiting for the server to respond.
Example
The page enters two integers, transfers the results to the back end through AJAX, and returns them.

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="x-ua-compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>AJAX Local refresh instance</title>
</head>
<body>
<input type="text" id="i1">+
<input type="text" id="i2">=
<input type="text" id="i3">
<input type="button" value="AJAX Submit" id="b1">
<script src="/static/jquery-3.2.1.min.js"></script>
<script>
$("#b1").on("click", function () {
$.ajax({
url:"/ajax_add/",
type:"GET",
data:{"i1":$("#i1").val(),"i2":$("#i2").val()},
success:function (data) {
$("#i3").val(data);
}
})
})
</script>
</body>
</html>
Code for HTML

def ajax_demo1(request):
return render(request, "ajax_demo1.html")
def ajax_add(request):
i1 = int(request.GET.get("i1"))
i2 = int(request.GET.get("i2"))
ret = i1 + i2
return JsonResponse(ret, safe=False)
views.py

urlpatterns = [
...
url(r'^ajax_add/', views.ajax_add),
url(r'^ajax_demo1/', views.ajax_demo1),
...
]
urls.py
3. Common scenarios of AJAX application
Search engines automatically prompt for keywords based on keywords entered by users.Another important scenario is the duplication of the user name at the time of registration.
Actually, AJAX technology is used here!When the input of a file box changes, use AJAX technology to send a request to the server, which then responds to the results of the query to the browser.
Finally, the results returned by the backend are shown.
- The page did not refresh during the whole process, it just refreshed part of the page!
- When the request is sent, the browser can do other things without waiting for the server to respond!
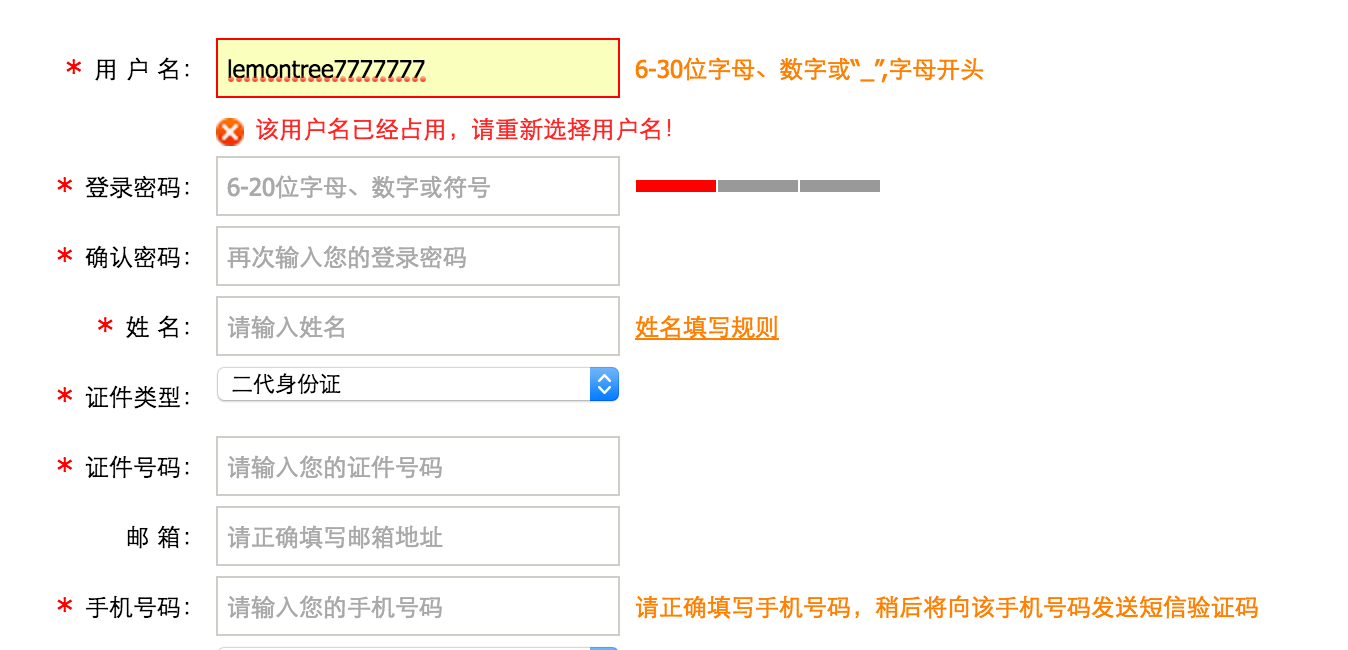
When the user name is entered and the cursor is moved to another form item, the browser uses AJAX technology to make a request to the server, which queries whether a user named lemontree7777777 exists.
The final server returns true to indicate that a user named lemontree77777 already exists and the browser displays "User name registered!" when it gets the result.
- The page did not refresh during the whole process, but only partially refreshed.
- After the request is sent, the browser can do other things without waiting for the server to respond.
Summary:
- AJAX uses JavaScript technology to send asynchronous requests to the server;
- AJAX requests do not have to refresh the entire page;
- AJAX performance is high because the server response content is no longer the entire page but part of the page;
4. AJAX implemented by jQuery
The most basic example of jQuery sending an AJAX request is:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="x-ua-compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>ajax test</title>
<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<button id="ajaxTest">AJAX test</button>
<script>
$("#ajaxTest").click(function () {
$.ajax({
url: "/ajax_test/",
type: "POST",
data: {username: "duoduo", password: 123456},
success: function (data) {
alert(data)
}
})
})
</script>
</body>
</html>
views.py:
def ajax_test(request):
user_name = request.POST.get("username")
password = request.POST.get("password")
print(user_name, password)
return HttpResponse("OK")
$.ajax parameters
A key-value pair in the data parameter that needs to be converted to a string type if the value is not a string.
$("#b1").on("click", function () {
$.ajax({
url:"/ajax_add/",
type:"GET",
data:{ "i1":$("#i1").val(),
"i2":$("#i2").val(),
"hehe": JSON.stringify([1, 2, 3])},
success:function (data) {
$("#i3").val(data);
}
})
})
JS implements AJAX

var b2 = document.getElementById("b2");
b2.onclick = function () {
// Native JS
var xmlHttp = new XMLHttpRequest();
xmlHttp.open("POST", "/ajax_test/", true);
xmlHttp.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
xmlHttp.send("username=duoduo&password=123456");
xmlHttp.onreadystatechange = function () {
if (xmlHttp.readyState === 4 && xmlHttp.status === 200) {
alert(xmlHttp.responseText);
}
};
};
Native JS implementation
5. How to set csrf_token for AJAX requests
Mode 1
Send in data by getting the csrfmiddlewaretoken value in the hidden input tag.
$.ajax({
url: "/cookie_ajax/",
type: "POST",
data: {
"username": "duoduo",
"password": 123456,
"csrfmiddlewaretoken": $("[name = 'csrfmiddlewaretoken']").val()
// Use JQuery to take out the value of csrfmiddlewaretoken and stitch it into data
},
success: function (data) {
console.log(data);
}
})
Mode 2
Sent in the request header by getting a string from the returned cookie.
Note: A jquery.cookie.js plug-in needs to be introduced.
$.ajax({
url: "/cookie_ajax/",
type: "POST",
headers: {"X-CSRFToken": $.cookie('csrftoken')}, // Get csrf_token from Cookie and set ajax request header
data: {"username": "duoduo", "password": 123456},
success: function (data) {
console.log(data);
}
})
Or write a getCookie method yourself:
function getCookie(name) {
var cookieValue = null;
if (document.cookie && document.cookie !== '') {
var cookies = document.cookie.split(';');
for (var i = 0; i < cookies.length; i++) {
var cookie = jQuery.trim(cookies[i]);
// Does this cookie string begin with the name we want?
if (cookie.substring(0, name.length + 1) === (name + '=')) {
cookieValue = decodeURIComponent(cookie.substring(name.length + 1));
break;
}
}
}
return cookieValue;
}
var csrftoken = getCookie('csrftoken');
It's too cumbersome to write this every time. You can request uniform settings for ajax using the $.ajaxSetup() method.
function csrfSafeMethod(method) {
// these HTTP methods do not require CSRF protection
return (/^(GET|HEAD|OPTIONS|TRACE)$/.test(method));
}
$.ajaxSetup({
beforeSend: function (xhr, settings) {
if (!csrfSafeMethod(settings.type) && !this.crossDomain) {
xhr.setRequestHeader("X-CSRFToken", csrftoken);
}
}
});
More details can be found in: Contents about CSRF in the official Djagno documentation
6. AJAX Upload File
// Example upload file
$("#b3").click(function () {
var formData = new FormData();
formData.append("csrfmiddlewaretoken", $("[name='csrfmiddlewaretoken']").val());
formData.append("f1", $("#f1")[0].files[0]);
$.ajax({
url: "/upload/",
type: "POST",
processData: false, // Tell jQuery not to process sent data
contentType: false, // Tell jQuery not to set the Content-Type request header
data: formData,
success:function (data) {
console.log(data)
}
})
})
7. Supplement Django's built-in serializers
def books_json(request):
book_list = models.Book.objects.all()[0:10]
from django.core import serializers
ret = serializers.serialize("json", book_list)
return HttpResponse(ret)
Add a SweetAlert plug-in example
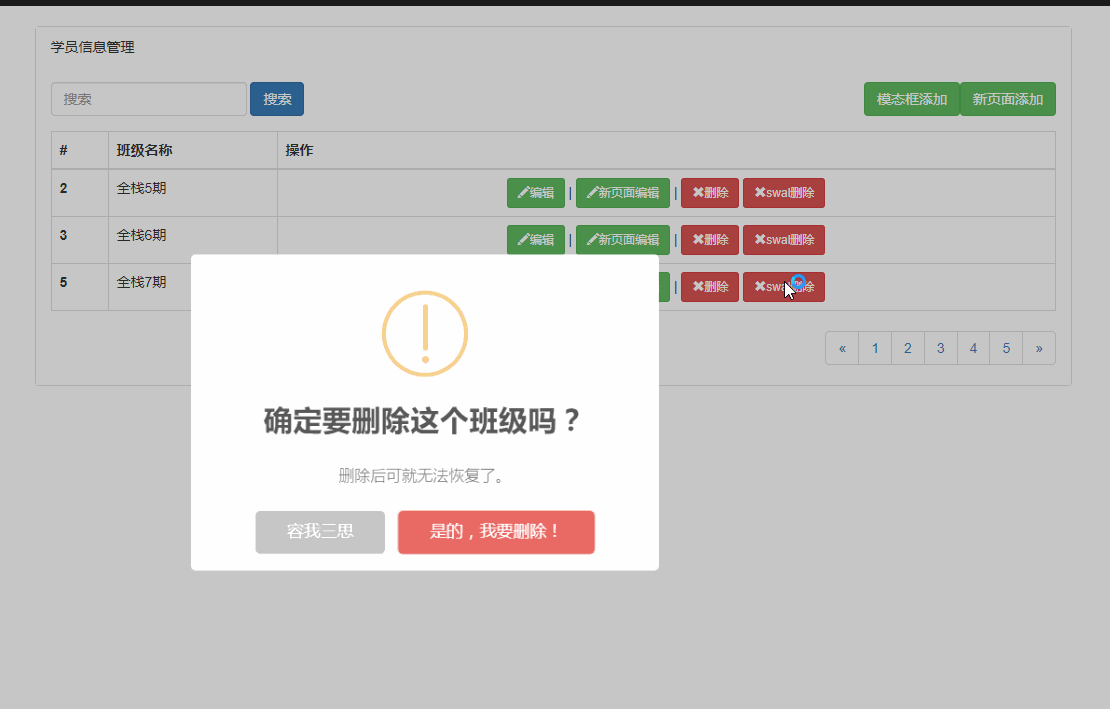
Click Download Bootstrap-sweetalert project.
$(".btn-danger").on("click", function () {
swal({
title: "Are you sure you want to delete it?",
text: "Delete can't find it!",
type: "warning",
showCancelButton: true,
confirmButtonClass: "btn-danger",
confirmButtonText: "delete",
cancelButtonText: "cancel",
closeOnConfirm: false
},
function () {
var deleteId = $(this).parent().parent().attr("data_id");
$.ajax({
url: "/delete_book/",
type: "post",
data: {"id": deleteId},
success: function (data) {
if (data.status === 1) {
swal("Delete succeeded!", "You're ready to run!", "success");
} else {
swal("Delete failed", "You can try again!", "error")
}
}
})
});
})
Posted by MrOnline on Sat, 18 May 2019 07:05:46 -0700