jQuery's get(), map(), map().get().join() simple application
Keywords:
Javascript
JQuery
1 .get()
Gets the DOM element specified by the selector
grammar
$(selector). get(index)
parameter
Specify which matching element to get (get by index number, starting from 0)
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="/jquery/jquery.js"></script>
<script type="text/javascript">
$(document).ready(function() {
$("#bt1").click(function() {
var x = $("p").get(0);
$("div").text(x.nodeName + ": " + x.innerHTML);
});
$("#bt2").click(function() {
var x = $("p").get(1);
$("div").text(x.nodeName + ": " + x.innerHTML);
});
});</script>
</head>
<body>
<p>Test 01</p>
<p>Test 02</p>
<button id="bt1">Get the first p DOM element</button>
<button id="bt2">Get the second p DOM element</button>
<div></div>
</body>
</html>
1.2 effect
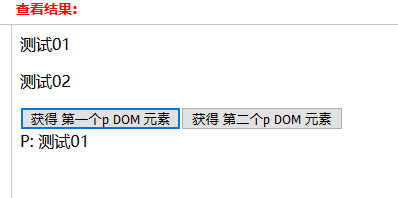
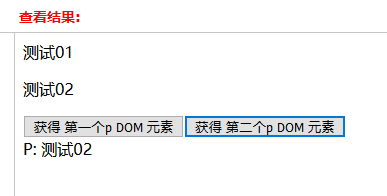
2 .map()
Each element is passed to the current matching set through a function to generate a new jQuery object containing the return value
grammar
.map(callback(index,domElement)))
parameter
Function object called for each element in the current collection
Detailed description
Because the return value is an array encapsulated by jQuery, get() is used to process the returned object to get the basic array
You can get comma separated text information through. get().join('), which can also be defined as other symbols you want to use
<!DOCTYPE html>
<html>
<head>
<style>p { color:red; }</style>
<script type="text/javascript" src="/jquery/jquery.js"></script>
</head>
<body>
<p><b>Values:</b></p>
<form>
<input type="text" name="name" value="admin" />
<input type="text" name="password" value="password" />
<input type="text" name="url" value="http://baidu.com" />
</form>
<button id="bt1">Get all input Element value</button>
<script type="text/javascript">
$("#bt1").click(function() {
var values = $("input").map(function() {
return $(this).val();
}).get().join(",");
$("p").append(values);
})
</script>
</body>
</html>
2.2 effect
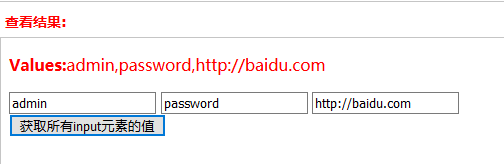
Posted by pedroz on Sun, 15 Dec 2019 09:17:00 -0800