When we visit the website, we sometimes pop up a small window with a piece of prompt message written on it. If you don't click "OK", you can't do anything to the web page. This small window is implemented by using alert.
Note: the alert pop-up message dialog box contains a OK button.
Syntax:
Alert (string or variable);
Be careful:
1. No other operation can be performed before clicking "OK" button in the dialog box.
2. Message dialog box can be used to debug programs.
3. The output of alert can be a string or a variable, similar to document.write.
- <title>alert</title>
- <script type="text/javascript">
- function rec(){
- var mychar="I love JavaScript";
- alert(mychar);
- }
- </script>
- </head>
- <body>
- <input name="button" type="button" onClick="rec()" value="Click me and a dialog box will pop up" />
- </body>
Confirm (confirm message dialog box)
The confirm message dialog is usually used to allow the user to make a selection action, such as: "are you right?" And so on. Pop up dialog box (including a OK button and a cancel button).Syntax:
confirm(str);
Parameter Description:
str: text to display in the message dialog Return value: Boolean value
Return value:
Returns true when the user clicks the "OK" button Return to false when the user clicks "Cancel"
Note: the return value can be used to judge what button the user clicked
- <script type="text/javascript">
- function rec()
- {
- var mymessage=confirm("You like it javascript Do you?");
- if(mymessage==true)
- {
- document.write("Good, come on!");
- }
- else if(mymessage==false)
- {
- document.write("To learn javascript,And you have to learn");
- }
- }
- </script>
- </head>
- <body>
- <input type="button" name="button" value="Click me to open the confirmation dialog box" onclick="rec()" />
- </body>
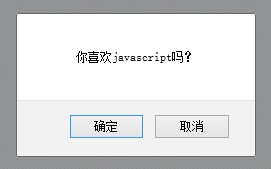
- <script type="text/javascript">
- function resc()
- {
- var myname=prompt("Please enter your name");
- if(myname!=null)
- {
- document.write("Hello"+myname);
- }
- else
- {
- document.write("Hello"+Friend);
- }
- }
- </script>
- <body>
- <input type="button" name="button" value="Click me to open the question dialog box" onclick="resc()" />
- </body>
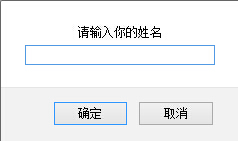
- <html>
- <head>
- <script type="text/javascript">
- function show_alert(){
- alert('first line\n Second elements');
- }
- function show_confirm(){
- var result = confirm('Delete or not!');
- if(result){
- alert('Delete successfully!');
- }else{
- alert('Do not delete!');
- }
- }
- function show_prompt(){
- var value = prompt('Enter your name:', 'Default name');
- if(value == null){
- alert('You canceled the entry!');
- }else if(value == ''){
- alert('The name is blank, please re-enter!');
- show_prompt();
- }else{
- alert('Hello,'+value);
- }
- }
- </script>
- </head>
- <body>
- <input id="alert_button" type="button" value="alert" onclick="show_alert()" >
- <input id="confirm_button" type="button" value="confirm" onclick="show_confirm()" >
- <input id="prompt_button" type="button" value="prompt" onclick="show_prompt()" >
- </body>
- </html>